"Game code for C++ often involves utilizing libraries and frameworks for graphics, sound, and input handling to create interactive experiences, such as a simple program that initializes a game window."
Here's a basic example using the SDL library to create a window:
#include <SDL.h>
int main(int argc, char* argv[]) {
SDL_Init(SDL_INIT_VIDEO);
SDL_Window* window = SDL_CreateWindow("Game Window",
SDL_WINDOWPOS_CENTERED, SDL_WINDOWPOS_CENTERED,
800, 600, SDL_WINDOW_SHOWN);
SDL_Delay(3000);
SDL_DestroyWindow(window);
SDL_Quit();
return 0;
}
Setting Up Your Development Environment
Recommended IDEs for C++ Game Development
Choosing the right Integrated Development Environment (IDE) is crucial for efficient coding. Some of the most popular IDEs for C++ game development include:
-
Visual Studio: With its powerful debugging features and extensive library support, Visual Studio is a favorite among C++ developers. It also offers integration with various game engines like Unreal Engine.
-
Code::Blocks: This is an open-source, lightweight IDE that is easy to set up and use, making it an excellent choice for beginners.
-
Eclipse: Known for its flexibility and customization options, Eclipse provides robust support for C++ through its CDT (C/C++ Development Tooling) plugins.
Installing Necessary Libraries
To kickstart your game development journey, it's essential to install libraries that simplify graphics, input handling, and sound. Among these, SDL (Simple DirectMedia Layer) is a popular choice. Below is a quick installation guide:
- Visit the SDL website and download the latest development package.
- Follow the installation instructions for your operating system (Windows, macOS, Linux).
- Once installed, link the SDL library to your IDE project settings.
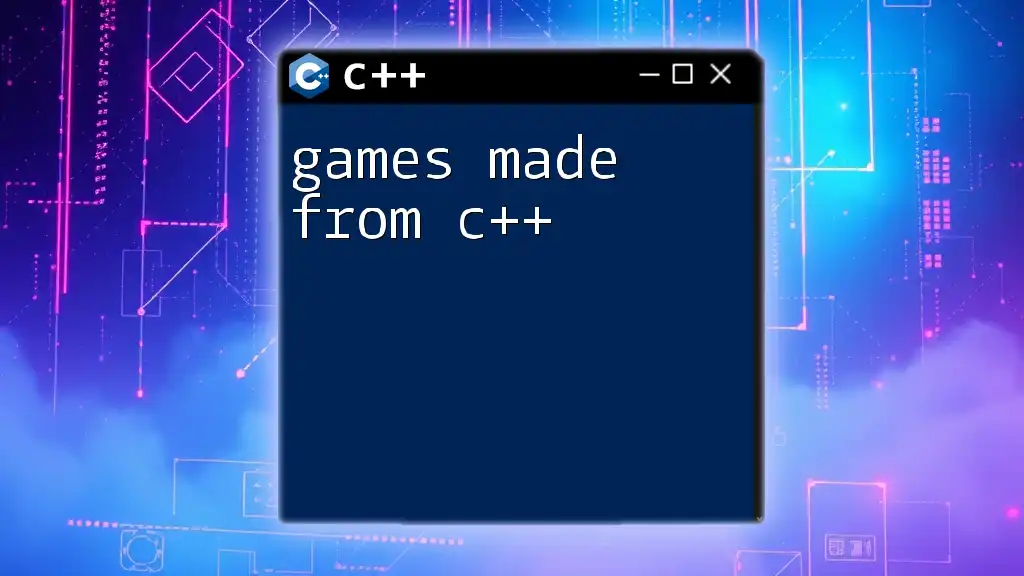
Basics of Game Loops
Understanding the Game Loop
In the context of game code for C++, the game loop is the backbone of any game. It continuously updates the game state and renders frames to the screen, creating a seamless experience for the player. The typical process involves three main components: handling input, updating game logic, and rendering.
Simple Game Loop Example
Here's a simple game loop example in C++:
#include <iostream>
using namespace std;
int main() {
bool isRunning = true;
while (isRunning) {
// Update game logic here
cout << "Game is running..." << endl;
// Control to exit the loop (for this example)
char input;
cin >> input;
if (input == 'q') {
isRunning = false;
}
}
return 0;
}
In this snippet, we see a clear example of a basic game loop. The program continues to print "Game is running..." until the user inputs 'q', at which point the loop terminates. This pattern forms the foundation of dynamic game behavior.
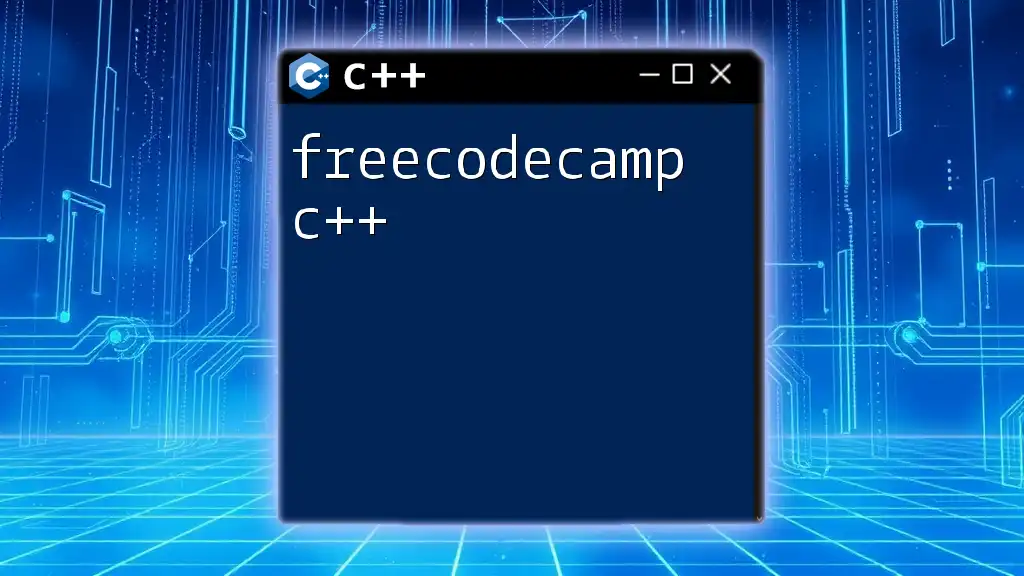
Creating Game Objects
Defining Classes in C++ for Game Entities
Understanding how to define classes in C++ is fundamental for crafting game objects. Object-Oriented Programming (OOP) allows for encapsulation, inheritance, and polymorphism, which can model complex game entities efficiently.
Here's a basic example of a Player class:
class Player {
public:
int health;
int score;
Player() : health(100), score(0) {}
void TakeDamage(int damage) {
health -= damage;
}
};
This class initializes a player with 100 health and a score of zero, providing a method to take damage. By creating and manipulating multiple instances of the Player class, you can easily manage various player states in your game.
Managing Game Objects
In addition to defining the classes of your game entities, you need effective methods for managing them. Consider implementing a game object manager that can:
- Register: Keep track of active game entities.
- Update: Modify their states on each frame within the game loop.
- Delete: Clean up objects that are no longer in use to free resources.
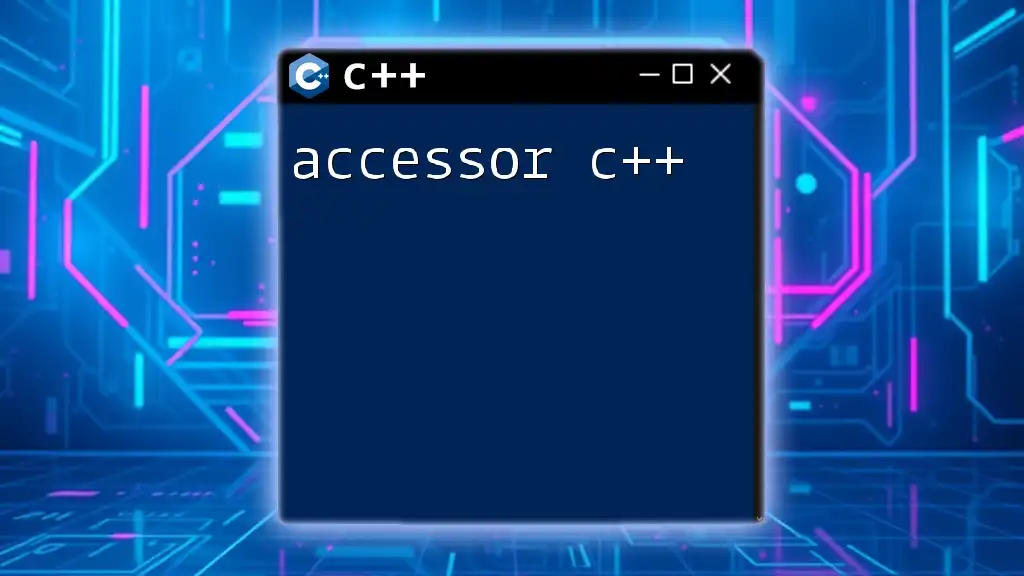
Graphics and Rendering
Using Libraries for Graphics Rendering
To render graphics in C++, libraries like SDL or SFML are commonly used. These libraries offer functionality for drawing shapes, images, and handling visual transformations needed for game design.
Example of Rendering an Object
The following snippet demonstrates how to create a simple window and render a colored rectangle using SDL:
#include <SDL.h>
int main(int argc, char *argv[]) {
SDL_Init(SDL_INIT_VIDEO);
SDL_Window *window = SDL_CreateWindow("Game Window", 100, 100, 640, 480, SDL_WINDOW_SHOWN);
SDL_Renderer *renderer = SDL_CreateRenderer(window, -1, SDL_RENDERER_ACCELERATED);
SDL_SetRenderDrawColor(renderer, 255, 0, 0, 255); // Red color
SDL_RenderClear(renderer); // Clear screen
SDL_RenderPresent(renderer); // Present rendered screen
SDL_Delay(2000); // Display for 2 seconds
SDL_DestroyRenderer(renderer);
SDL_DestroyWindow(window);
SDL_Quit();
return 0;
}
This code initializes SDL, creates a window, and renders a red color for 2 seconds before terminating. It showcases how easy it is to draw graphical components in your game.
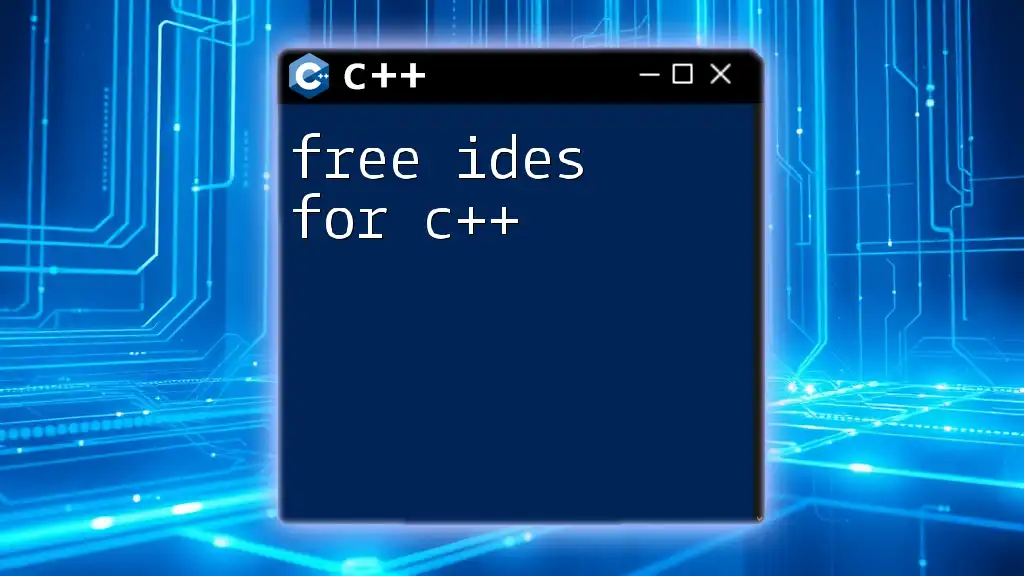
Input Handling
Capturing Player Input
Handling player input properly is crucial for interactive gameplay. SDL provides efficient methods to capture keyboard and mouse events.
Example of Handling Keyboard Input
The following example illustrates a basic input handling routine:
void HandleInput() {
SDL_Event event;
while (SDL_PollEvent(&event)) {
if (event.type == SDL_QUIT) {
// Handle quit
}
if (event.type == SDL_KEYDOWN) {
// Handle key presses
}
}
}
In this snippet, the game continuously checks for events and can respond to quit commands or keystrokes. Reacting to player input enhances the engagement and control within the game.
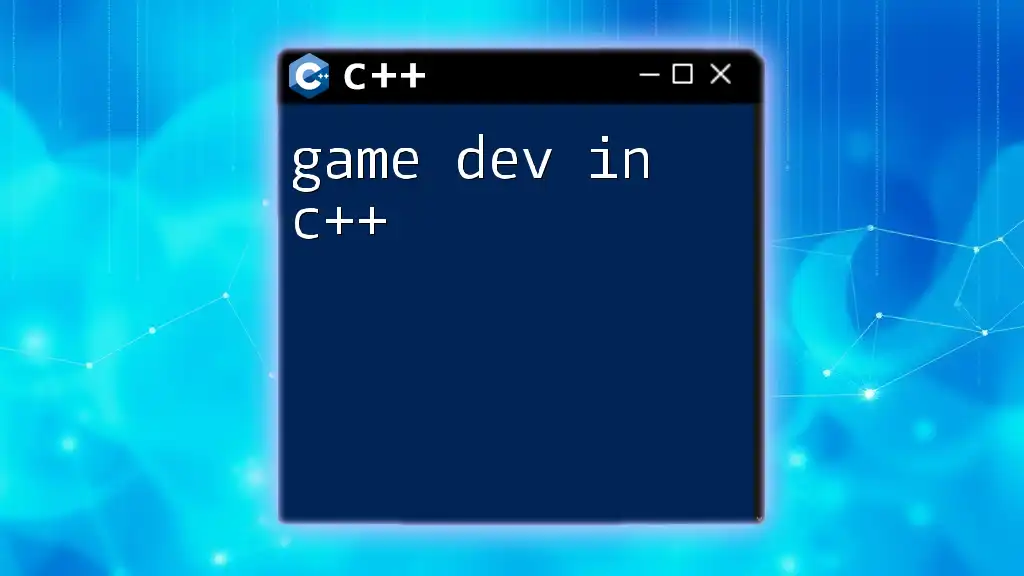
Collision Detection
Understanding Collision in Games
Collision detection is a critical aspect of game design, enabling interactions between game entities, such as players and obstacles. It allows for a more believable game environment and increases player immersion.
Simple Collision Detection Example
Here's a straightforward method for bounding box collision detection:
bool CheckCollision(Player &player, GameObject &object) {
// Simple bounding box collision
return player.x < object.x + object.width &&
player.x + player.width > object.x &&
player.y < object.y + object.height &&
player.y + player.height > object.y;
}
This function checks whether the player's bounding box intersects with another object's bounding box. Effectively implementing collision can enrich the player's gaming experience substantially.
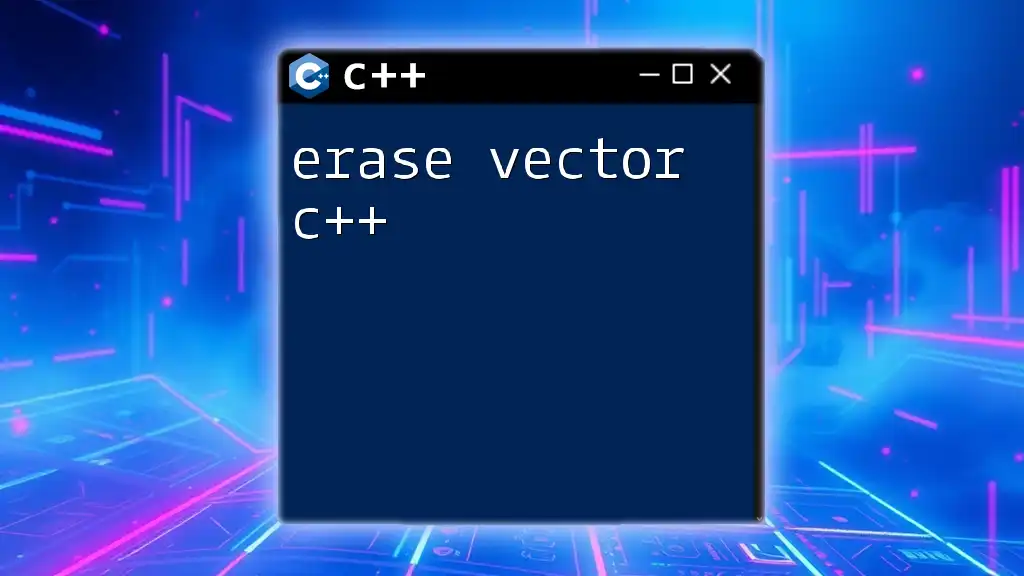
Implementing Sound
Integrating Audio Libraries in C++
Sound effects and background music significantly enhance gameplay. Libraries like SDL_Mixer allow you to integrate audio capabilities with ease.
Playing Sound Effects
The code below showcases how to play a sound effect using SDL_Mixer:
#include <SDL_mixer.h>
Mix_OpenAudio(44100, MIX_DEFAULT_FORMAT, 2, 2048);
Mix_Chunk *sound = Mix_LoadWAV("sound.wav");
Mix_PlayChannel(-1, sound, 0);
This snippet initializes the mixer, loads a sound file, and plays it in the default channel. Sound adds emotional depth to your game and is essential for engaging gameplay experiences.
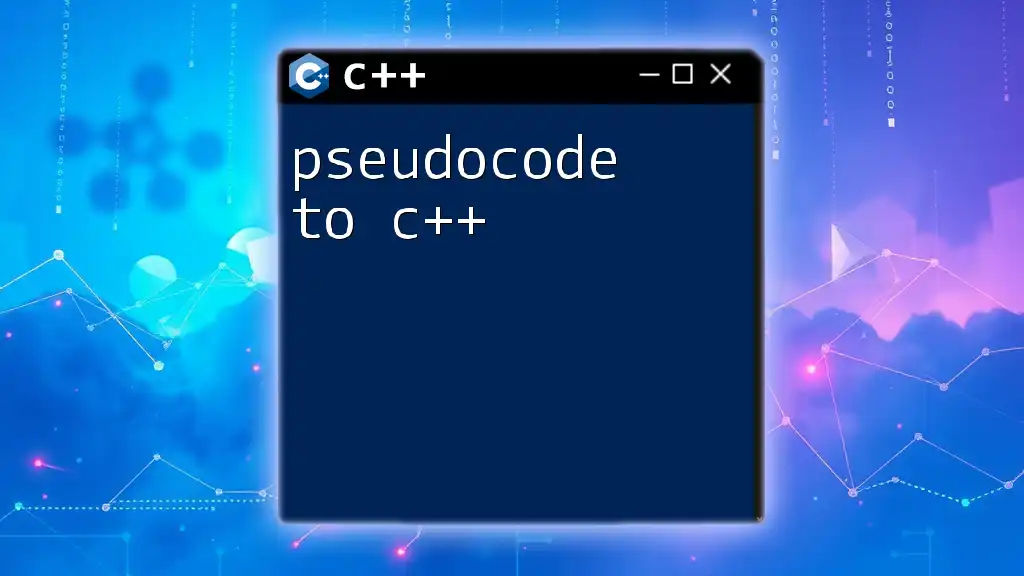
Conclusion
In summary, understanding game code for C++ is integral to creating immersive video games. The importance of a well-structured development environment, a robust game loop, efficient input handling, and dynamic object management cannot be overstated. Dive into creating your small game projects to practice these concepts and continuously evolve your skills in C++ game development.
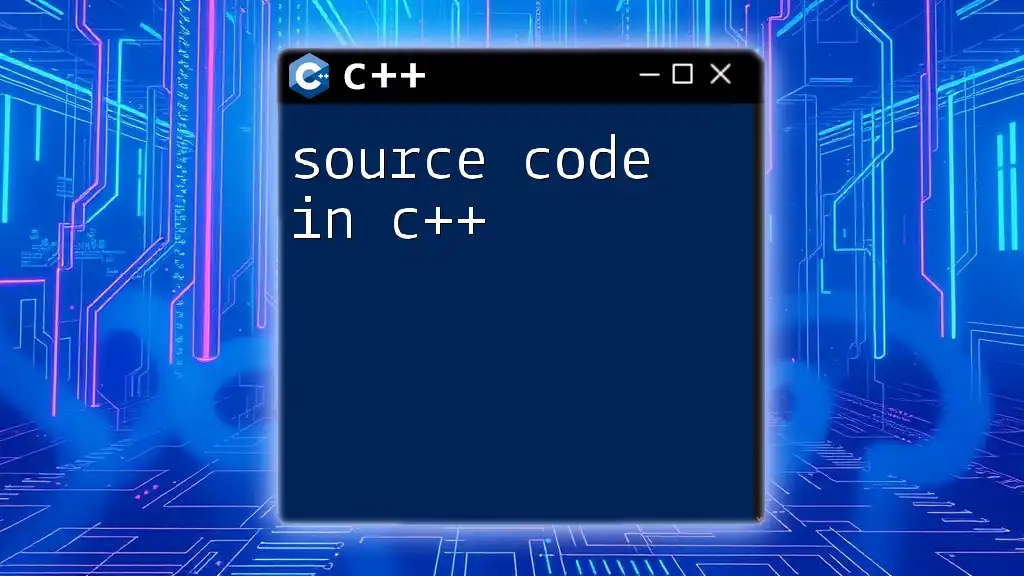
Additional Resources
To further your knowledge in C++ game programming, explore tutorials, online courses, and communities where you can exchange ideas and get feedback. Networking with other developers can open doors to collaboration and learning.
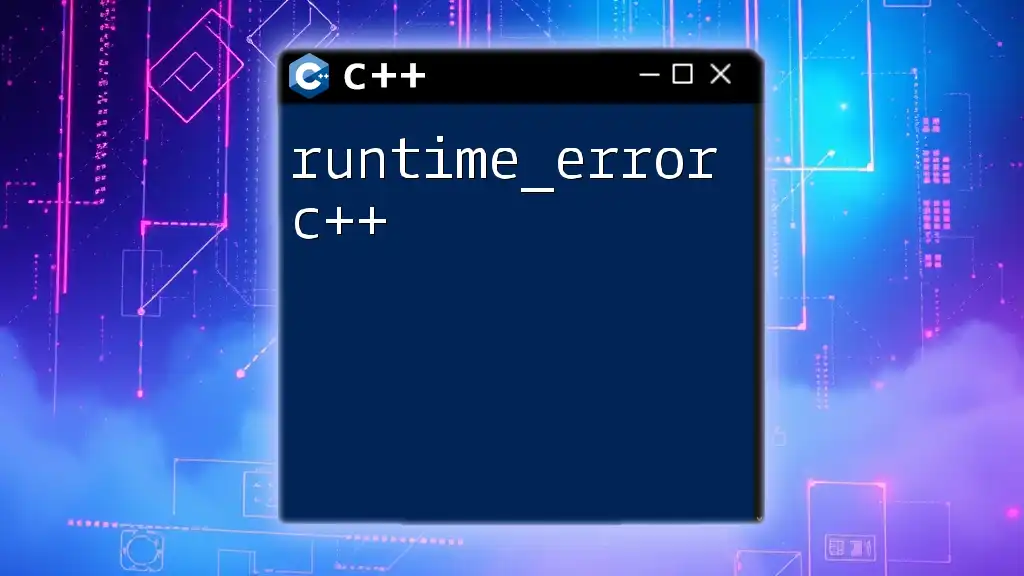
Call to Action
Don't hesitate to share your game projects or ask questions in the comments section below. Stay tuned for more articles to sharpen your C++ skills and enhance your game development journey!