JetBrains IDEs, such as CLion, provide powerful features like code completion, debugging, and integrated testing, making C++ development more efficient and user-friendly.
Here’s an example of a simple C++ program using basic syntax:
#include <iostream>
int main() {
std::cout << "Hello, JetBrains IDE for C++!" << std::endl;
return 0;
}
What is JetBrains IDE for C++?
JetBrains is a renowned company known for creating powerful IDEs tailored for various programming languages. Among these, CLion stands out as the go-to IDE for C++ development. It integrates many features that streamline the programming process, enabling developers to focus on writing code rather than getting bogged down by the complexities of the development environment.
Setting Up JetBrains IDE for C++
Downloading and Installing
To get started with the JetBrains IDE for C++, you'll first need to download CLion. Here’s how to do it:
- Navigate to the [JetBrains website](https://www.jetbrains.com/clion/download/).
- Choose the installer that corresponds to your system (Windows, macOS, Linux).
- Follow the instructions provided for installation, ensuring you meet the minimum system requirements, such as:
- OS: Windows 10 or later, macOS, or a compatible Linux distribution.
- RAM: At least 4 GB (8 GB recommended).
- Disk Space: Minimum of 1.5 GB of free space.
When installing, you'll be given options for configurations such as enabling system PATH for easy command line access. Choose according to your preference.
Configuring Your First C++ Project
Once installation is complete, launching CLion will allow you to create your first C++ project. Here’s how:
- Open CLion and select "New Project".
- Choose C++ Executable and then select the CMake option as the build system.
- Name your project (e.g., `HelloWorld`), and choose a location on your system.
Now, let's create our first C++ program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
To run this project, use the following steps:
- Create a `CMakeLists.txt` file if one isn't created automatically, and ensure it includes:
cmake_minimum_required(VERSION 3.10) project(HelloWorld) set(CMAKE_CXX_STANDARD 11) add_executable(HelloWorld main.cpp)
- Build your project using the green arrow at the top or from the Build menu.
- Click Run to see your "Hello, World!" program in action.
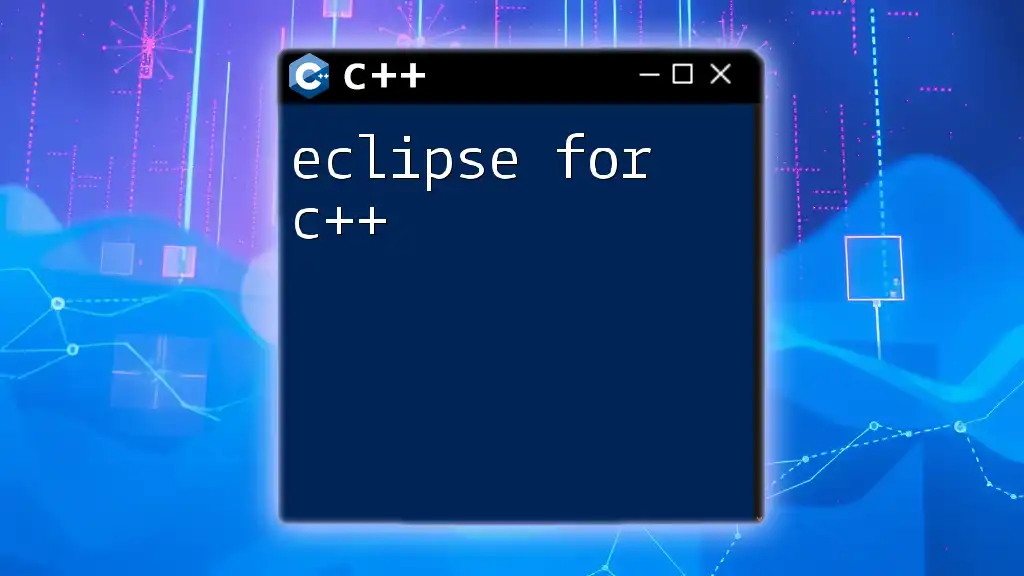
Key Features of JetBrains IDE for C++
Smart Code Completion
One of the shining features of CLion is its smart code completion. The IDE analyzes your code context and suggests relevant completions, significantly speeding up your coding process. This is particularly useful for complex C++ syntax and libraries.
To use this feature, simply start typing a variable or function name, and suggestions will appear in a dropdown menu.
Powerful Refactoring Tools
Refactoring is crucial for maintaining clean code and improving the structure without changing its external behavior.
In CLion, you can:
- Rename variables and functions effectively throughout the project:
- Right-click on the name and select `Refactor` → `Rename` or simply press Shift + F6.
An example of refactoring might be changing a function name from `calculateSum()` to `computeTotal()`. CLion will automatically find every instance of that function in your project.
Debugging Capabilities
Debugging is vital in discovering and correcting errors in your code. CLion shines with its robust built-in debugger.
- Set Breakpoints: Click on the gutter next to the line number where you want execution to pause.
- Run in Debug Mode: Select the Debug configuration from the toolbar.
- Inspect Variables: As your code runs in debug mode, you can hover over variables or use the Variables tab to view current values.
Here’s a quick troubleshooting scenario: suppose you have a loop that isn’t executing as expected. Placing a breakpoint inside the loop and inspecting variables can help you identify where things are going wrong.
Integrated Version Control
Version control is essential in managing changes to your code. JetBrains IDEs support popular systems like Git, making it easy to track your project history and collaborate with others.
To integrate Git:
- Go to VCS → Enable Version Control Integration.
- Choose Git and hit OK.
- You can now commit changes, view your history, and manage branches directly from within the IDE.
Make sure to frequently commit your work with descriptive messages for clarity.
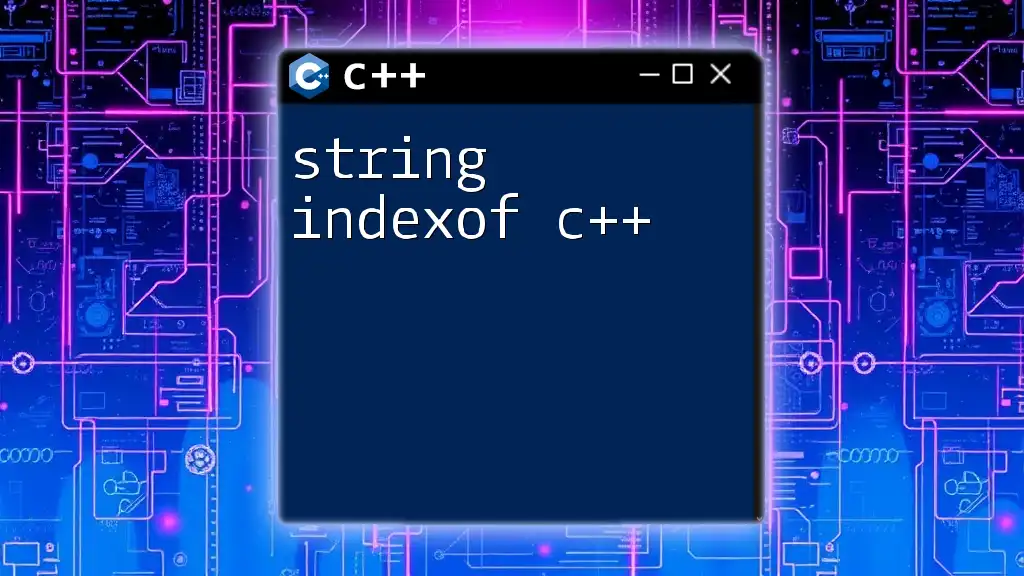
Customization and Extensions
Setting Up Your Environment
A productive coding environment is crucial. Personalize your CLion editor by adjusting themes, fonts, and layouts to suit your workflow. Go to File → Settings to explore customization options.
Using Plugins to Enhance Functionality
CLion supports plugins to extend its functionality. The JetBrains Plugin Repository offers a plethora of C++-specific, productivity-enhancing tools.
Consider installing:
- CMake Tools: For improved CMake file handling.
- Doxygen: For generating project documentation effortlessly.
These plugins can enhance your efficiency and code maintainability.
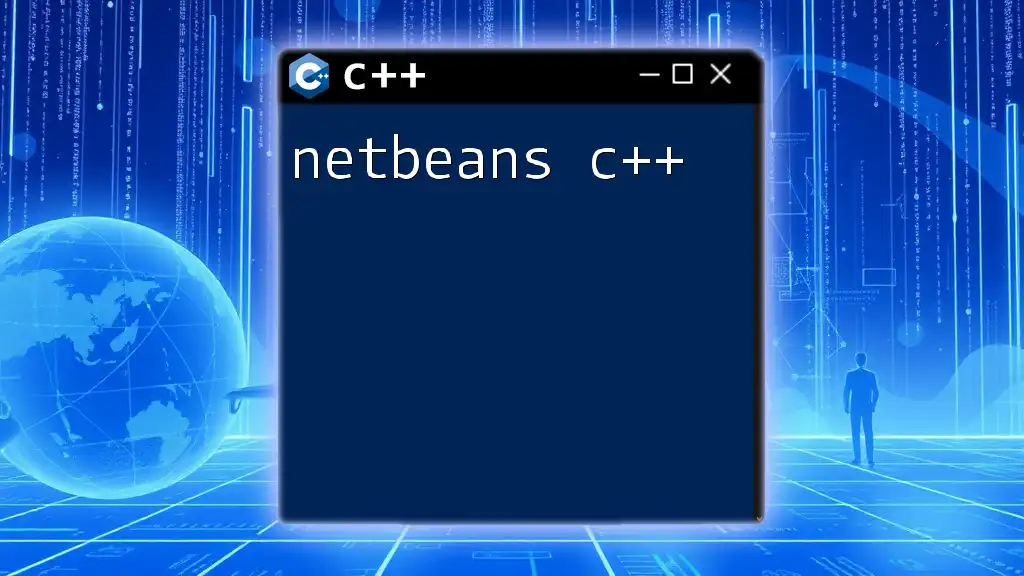
Best Practices for Using JetBrains IDE
Stay Updated
Ensure you keep your JetBrains IDE updated. Updates include vital performance improvements and new features. Automatic updates can simplify this process; enable them in the settings.
Regularly Utilize Inspections
CLion features built-in inspections that evaluate your code quality in real-time. Utilize these inspections to catch potential issues. You can customize inspections by navigating to File → Settings → Editor → Inspections.
Leveraging Built-in Documentation
JetBrains IDEs come with shortcuts to access documentation. Use `Ctrl + Q` (Windows/Linux) or `F1` (macOS) while hovering over a function or class for quick help without leaving your IDE. This feature can greatly enhance your comprehension of unfamiliar APIs.
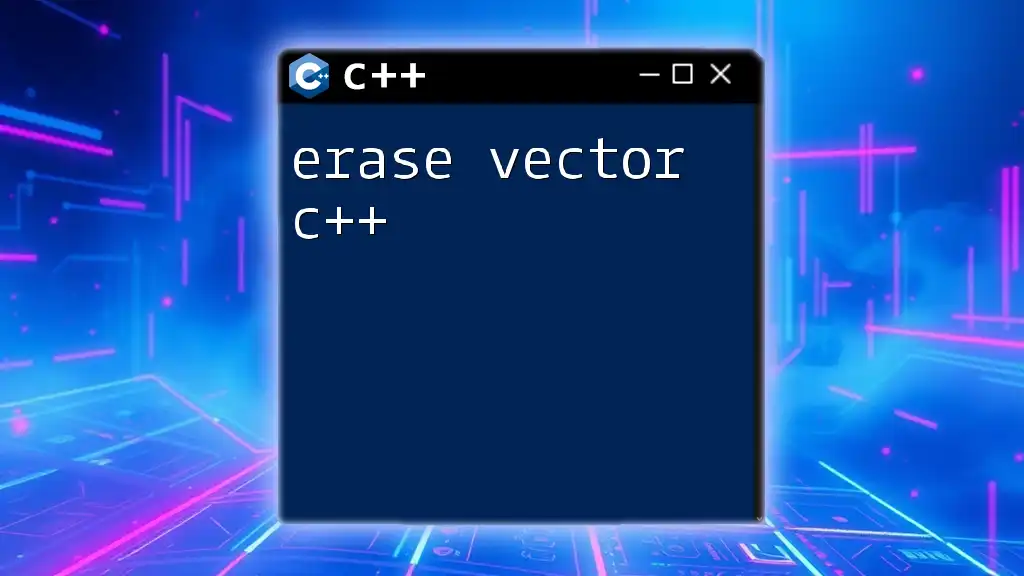
Troubleshooting Common Issues
Common Installation Problems
Installation issues can often be resolved by ensuring:
- Your system meets the minimum requirements.
- Any prior versions of JetBrains IDEs are fully uninstalled.
- You’re running the installer as an Administrator (for Windows users).
If you encounter installation errors, consulting the JetBrains support documentation can be helpful.
Debugging Problems in Projects
Difficulties in debugging may arise when configurations are incorrect. If a build fails or your debugger isn’t connecting:
- Ensure you've established the right build configurations.
- Double-check your CMake settings for compatibility.
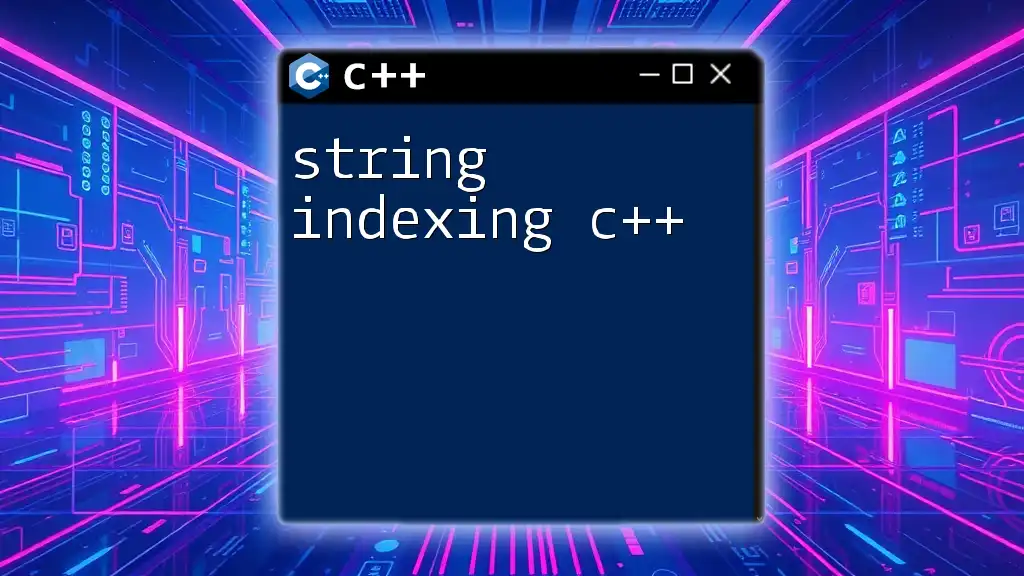
Conclusion
The JetBrains IDE for C++ (CLion) is a powerful, feature-rich tool that significantly eases the C++ development process. By leveraging its advanced capabilities—such as intelligent code completion, refactoring tools, a built-in debugger, and version control integration—you can improve your productivity while maintaining high code quality.
Exploring the various features and customization options will enhance your coding experience, allowing you to focus on what truly matters: writing clean, efficient, and working code.
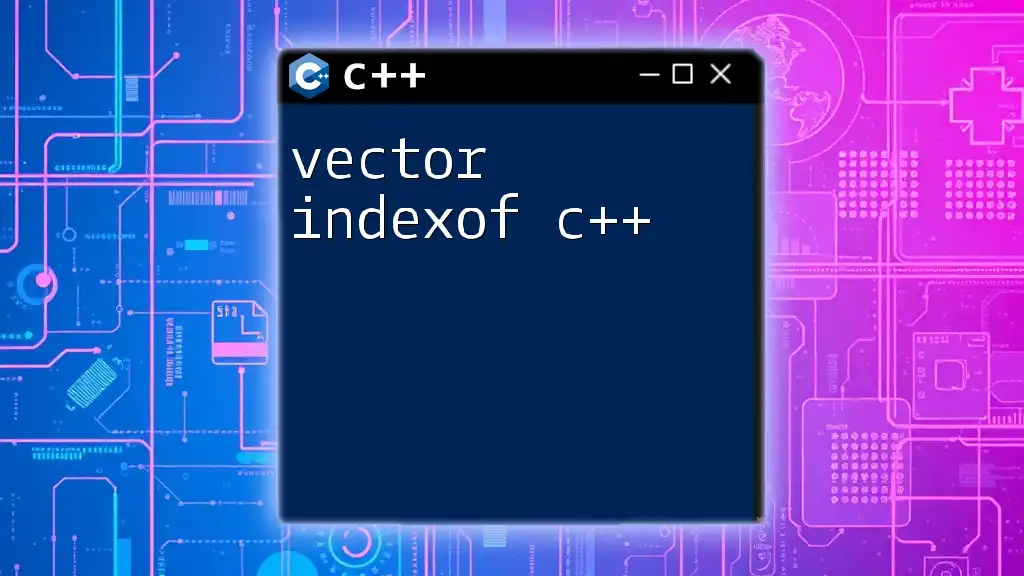
Additional Resources
For further study, consider and explore the following:
- Official JetBrains Documentation for C++: [JetBrains CLion Official Documentation](https://www.jetbrains.com/help/clion/)
- Community forums and GitHub repositories for troubleshooting and advanced topics within C++ development.
Take the leap and enjoy refining your C++ programming skills with JetBrains IDE today!