In C++, string indexing allows you to access individual characters of a string using zero-based indexing. Here's a quick example:
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, World!";
char firstCharacter = str[0]; // Accessing the first character
std::cout << firstCharacter << std::endl; // Outputs: H
return 0;
}
Understanding Strings in C++
In C++, strings are primarily handled using the `std::string` class from the Standard Library. This class is versatile and provides numerous built-in methods to manipulate strings easily. What makes `std::string` powerful is that it abstracts away many complexities associated with C-style strings, such as manual memory management.
Characteristics of `std::string`
- Dynamic Length: Unlike C-style strings, `std::string` can grow or shrink in size dynamically, giving you the freedom to manipulate text without worrying about buffer overflow.
- Rich Functionality: `std::string` provides a wealth of methods for string manipulation, such as searching, substring extraction, and modification.
Code Example:
#include <iostream>
#include <string>
int main() {
std::string example = "Hello, World!";
std::cout << example << std::endl;
return 0;
}
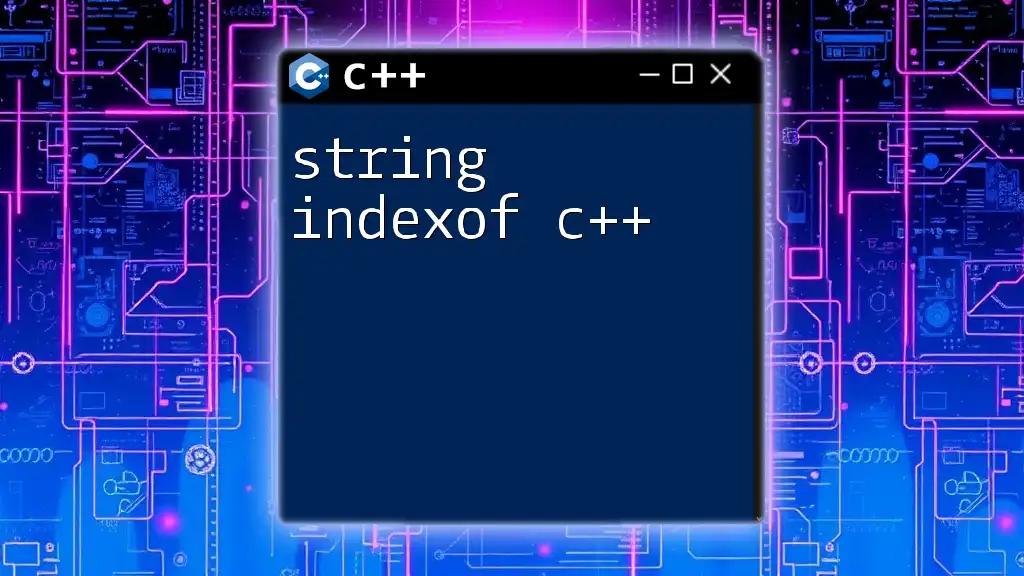
The Basics of String Indexing
String indexing refers to the method of accessing individual characters in a string through their respective positions or indices. In C++, string indexing starts at 0; that means the first character of the string is accessed using the index 0, the second character with index 1, and so forth.
Key Concepts:
- Zero-based Indexing: The first character is indexed at 0, the second at 1, and so forth.
- Accessing Characters: Each character can be accessed directly using its index.

Accessing Characters Using Indices
To access a character at a specific index, you can simply use the square brackets `[]` notation. The character at that index can be directly retrieved and utilized in your program.
Code Example:
std::string text = "Hello";
char firstChar = text[0]; // Accessing first character
std::cout << "First character: " << firstChar << std::endl; // Outputs: H
Handling Out-of-Bounds Indices
One important aspect to consider is what happens if you use an index that exceeds the bounds of the string. Accessing an invalid index results in undefined behavior, which can lead to crashes or data corruption. To prevent this, you can use the `.at()` method, which throws an exception if the index is out of range.
Best Practices:
- Use `.at()` for safer character access, as it checks the validity of the index.
Code Example:
try {
char outOfBoundsChar = text.at(10); // This will throw an exception
} catch (const std::out_of_range& e) {
std::cout << "Error: " << e.what() << std::endl; // Handle the error gracefully
}
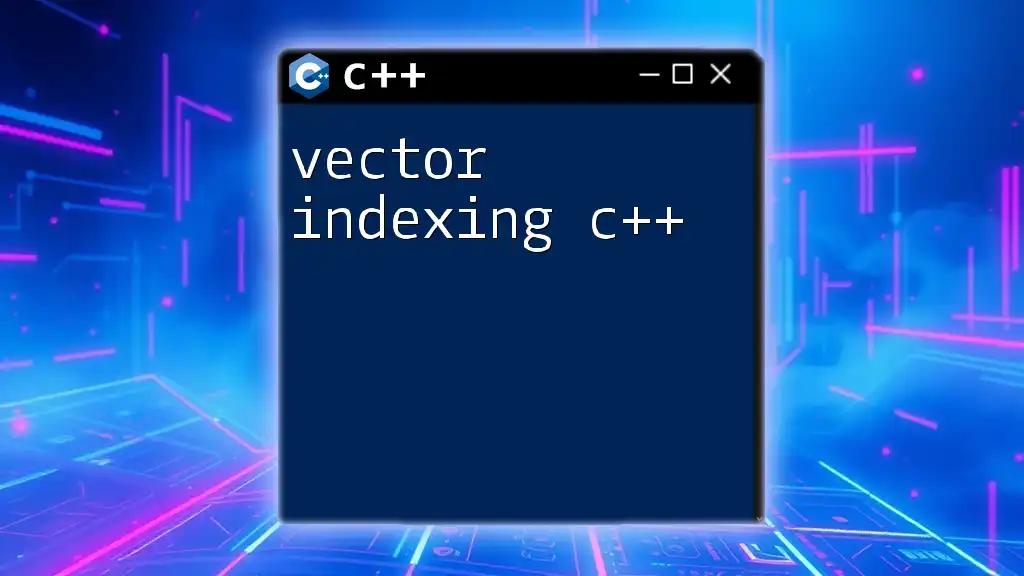
Modifying Characters in a String
C++ allows you to modify characters in a string by accessing them using their indices. You can simply assign a new character to a specific index, which will overwrite the existing character.
Code Example:
std::string sentence = "Hello";
sentence[0] = 'Y'; // Changing 'H' to 'Y'
std::cout << "Modified string: " << sentence << std::endl; // Outputs: Yello
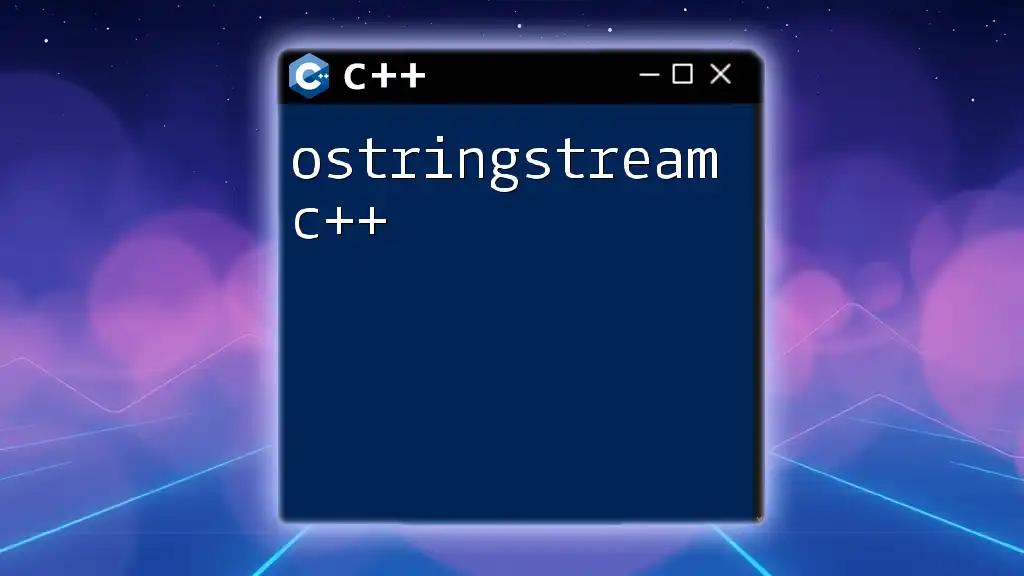
C++ String Indexing Methods
C++ strings come with several built-in methods that simplify working with string indexing. Understanding these methods unlocks more complex and efficient string manipulations.
Using Methods from the `std::string` Library
Some useful methods related to string indexing include:
- `.find()`: Searches for a substring or character and returns the index of its first occurrence.
- `.substr()`: Extracts a substring from the string based on starting index and length.
- `.replace()`: Replaces a part of the string starting at a given index.
Code Examples:
std::string example = "Hello World";
size_t index = example.find('W'); // Finding 'W'
std::cout << "Index of 'W': " << index << std::endl; // Outputs: 6
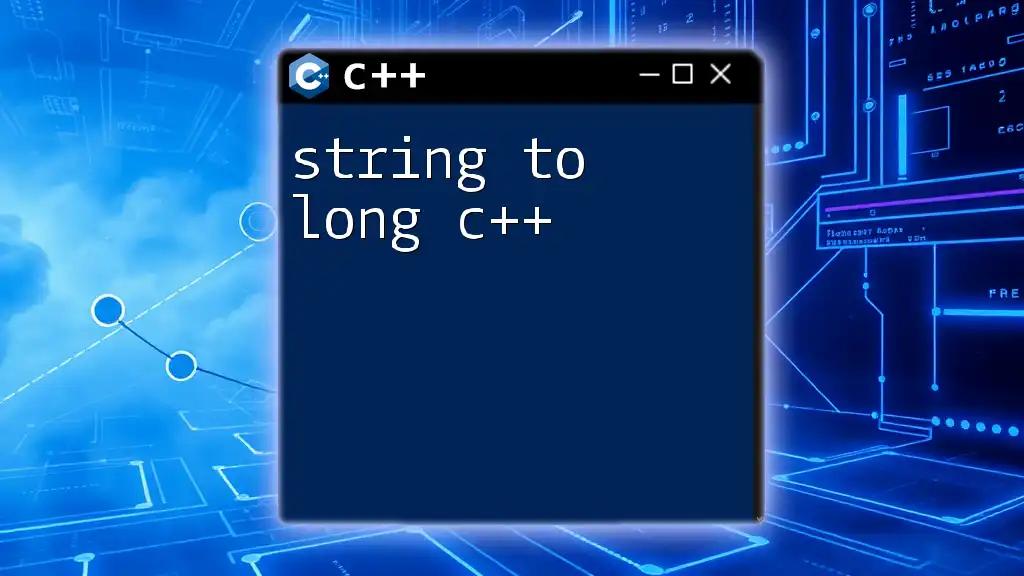
Advanced String Indexing Techniques
Iterating Over String Indices
You can easily loop through the characters of a string using a for loop. This allows you to access each character using its index.
Code Example:
std::string str = "Hello";
for (size_t i = 0; i < str.size(); ++i) {
std::cout << "Character at index " << i << ": " << str[i] << std::endl;
}
Combining String Indexing with Other STL Concepts
While string indexing is powerful on its own, combining it with other Standard Template Library (STL) features can create robust solutions. For instance, you might store strings in a vector and manipulate them using indices for various applications like text analysis or formatting.

Common Mistakes in String Indexing
As straightforward as string indexing may seem, there are common pitfalls programmers often encounter:
- Forgetting Zero-Based Index: A common mistake is to assume strings are one-based, leading to incorrect indexing and potential crashes.
- Off-By-One Errors: Failing to account for the `size()` method when looping through strings can lead to attempts to access invalid indices.
Tips to Avoid Mistakes
- Always check the length of the string with the `.size()` method before accessing an index.
- Prefer using the `.at()` method for safer coding practices, especially in production code where robustness is essential.

Conclusion
String indexing in C++ is an essential concept for anyone looking to master the language. By understanding how to access and manipulate characters within a string, along with associated best practices, you can significantly enhance your coding skills. Practice is crucial, so engage with various coding exercises that challenge your understanding of string indexing. Happy coding!
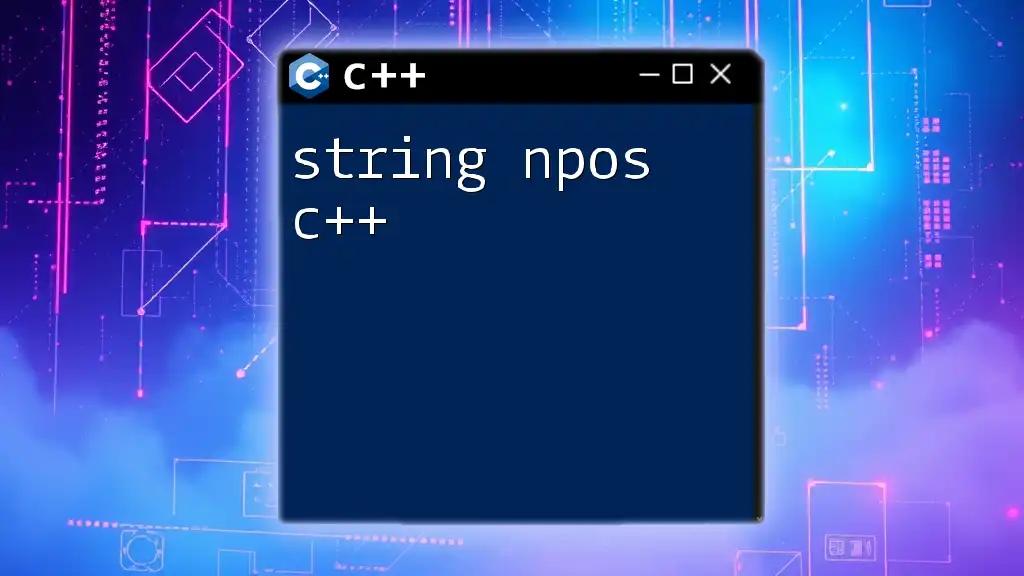
Additional Resources
For further learning, consult the official C++ documentation for strings and related STL components. Numerous online tutorials and programming books can also provide deeper insights into handling strings more effectively in C++.