To convert a string to a long integer in C++, you can use the `std::stol` function, which parses the string and returns its value as a long.
#include <string>
#include <iostream>
int main() {
std::string str = "12345";
long num = std::stol(str);
std::cout << "The long integer value is: " << num << std::endl;
return 0;
}
Understanding the Data Types
What is a String in C++?
In C++, a `std::string` is a class designed to house a sequence of characters. Unlike character arrays in C, `std::string` provides a rich set of methods and functionalities that facilitate easier manipulation of textual data. Strings are dynamic, meaning their size can change during runtime, allowing for more flexible programming.
The key characteristics of `std::string` include:
- Automatic Memory Management: Strings automatically allocate and deallocate memory as needed.
- Concatenation Capabilities: You can easily concatenate strings using the `+` operator or the `append()` method.
- Rich Member Functions: Strings come equipped with a variety of functions, such as `length()`, `find()`, and `substr()`, that make text processing simpler.
What is a Long Integer in C++?
In C++, the `long` and `long long` data types are used to represent large integer values. The choice between `long` and `long long` often depends on the size of the number you intend to manipulate.
- `long`: Typically provides a range from `-2,147,483,648` to `2,147,483,647`, or higher based on system architecture.
- `long long`: Used to cover even larger values, capable of representing numbers well beyond the range of `long`.
Knowing the difference between these data types is crucial, especially when you’re converting between them, as using an inappropriate type can lead to data loss or overflow errors.
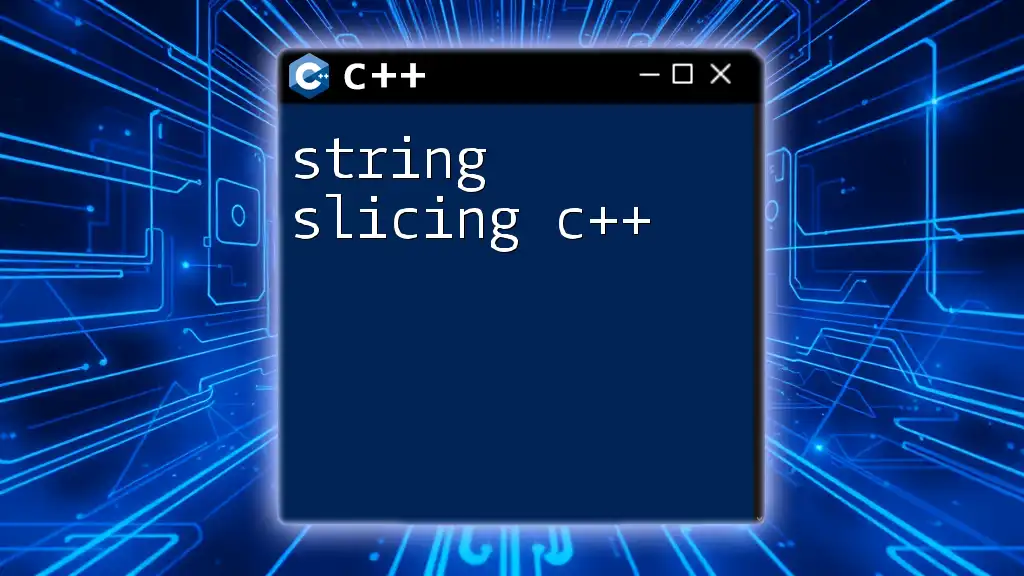
Converting String to Long
Why Convert String to Long?
Converting a `string` to `long` is a common operation in C++ programming, especially when dealing with user input or data read from files. For instance, if you receive a numeric value as a string from user input, you will need to convert it to a numeric type to perform calculations or comparisons.
Method 1: Using `std::stol` Function
Overview of `std::stol`
The `std::stol` function, included in the `<string>` library, allows for straightforward conversion of strings to `long`. Its syntax is as follows:
long std::stol(const std::string& str, std::size_t* pos = 0, int base = 10);
- Parameters:
- `str`: The source string to convert.
- `pos`: An optional parameter that will point to the first character after the number once the conversion is complete.
- `base`: An optional parameter allowing the specification of the number's base.
Example of Using `std::stol`
#include <iostream>
#include <string>
int main() {
std::string strNumber = "123456";
long number = std::stol(strNumber);
std::cout << "Converted Number: " << number << std::endl;
return 0;
}
In this example, the string `"123456"` is converted to the long integer `123456`. The output will confirm the successful conversion. One of the advantages of `std::stol` is its ability to handle leading spaces and various number formats.
Method 2: Using `std::stringstream`
Overview of `std::stringstream`
`std::stringstream`, part of the `<sstream>` library, is another effective way to convert strings to numeric types. This method enables formatted input and output, giving programmers more flexibility in handling data.
Example of Using `std::stringstream`
#include <iostream>
#include <sstream>
int main() {
std::string strNumber = "789012";
std::stringstream ss(strNumber);
long number;
ss >> number;
std::cout << "Converted Number: " << number << std::endl;
return 0;
}
This code snippet illustrates creating a `std::stringstream` object initialized with the string `"789012"`. The `>>` operator reads the content and converts it into the `long` variable `number`. This method is particularly useful when you need to parse multiple numbers from a string or if the input format is complex.
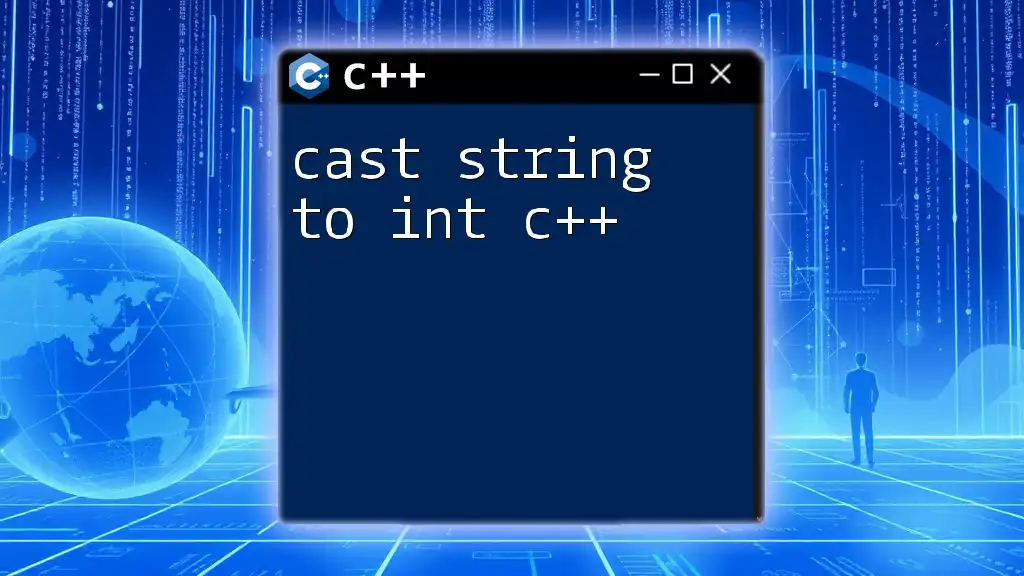
Handling Conversion Errors
Common Errors During Conversion
When converting from `string` to `long`, it's essential to be aware of potential errors such as unrecognized characters or overflow, which can cause exceptions. If the string cannot be completely converted into a long integer, it may result in either `std::invalid_argument` or `std::out_of_range` exceptions.
Example of Error Handling
#include <iostream>
#include <string>
int main() {
std::string strNumber = "abc123"; // Invalid string input
try {
long number = std::stol(strNumber); // This will throw invalid_argument
} catch (const std::invalid_argument& e) {
std::cerr << "Invalid input: " << e.what() << std::endl;
} catch (const std::out_of_range& e) {
std::cerr << "Input out of range: " << e.what() << std::endl;
}
return 0;
}
In this example, the string `"abc123"` triggers an `invalid_argument` exception when passed to `std::stol`. The error handling gracefully captures the exception, allowing the program to continue running and providing user-friendly feedback.
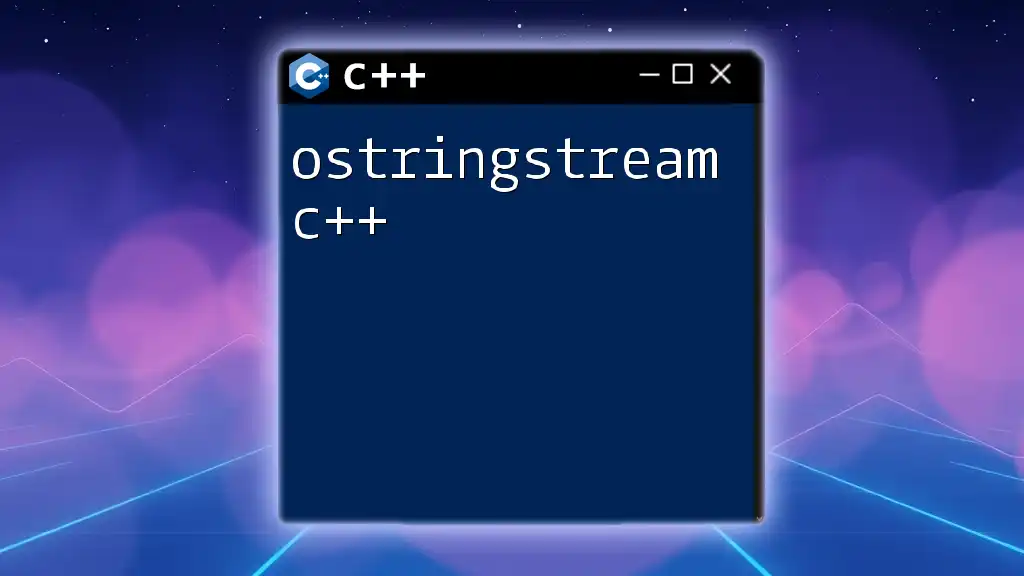
Best Practices for String to Long Conversion
When performing the conversion from `string` to `long`, consider the following best practices:
-
Validate Input: Always validate the input string before attempting conversion. This can include checking for non-numeric characters or ensuring that the input is not empty.
-
Use Exception Handling: Employ try-catch blocks around conversion functions. This will help manage errors seamlessly and avoid unexpected crashes.
-
Be Mindful of Limits: Understand the limits of the long integer type you are using. If there’s a possibility of overflow, consider using `long long` to accommodate larger values.
By following these practices, you can minimize risks and ensure your conversions are reliable and robust.
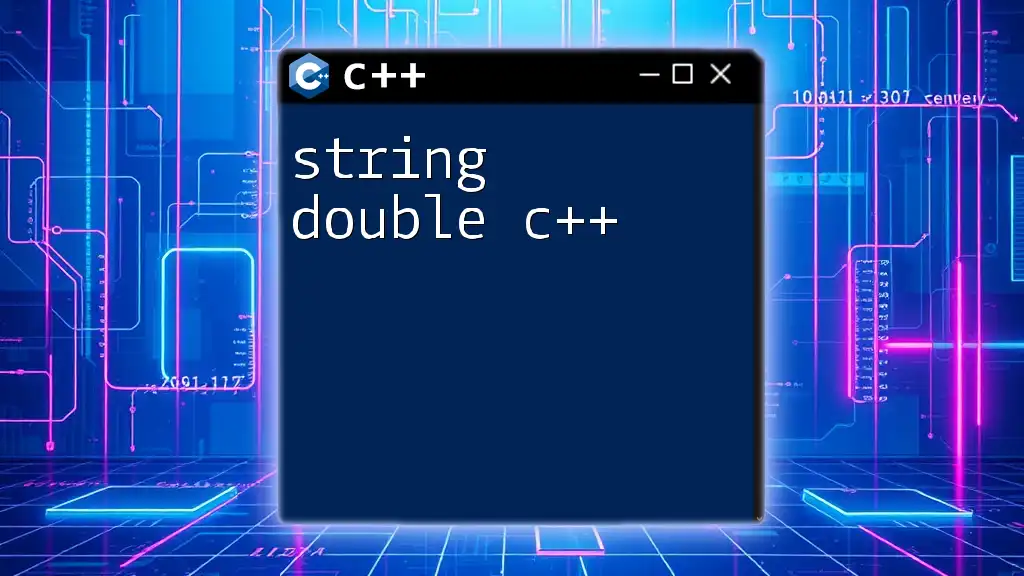
Conclusion
In summary, converting a string to a long integer in C++ is a fundamental skill that enhances data handling capabilities. This guide covered the two primary methods for conversion: `std::stol` and `std::stringstream`, alongside error handling techniques and best practices. As you gain more experience with C++, applying these techniques will become an integral part of your programming toolkit, allowing you to manage and manipulate data effectively.
Explore further into C++ documentation and tutorials to deepen your understanding, and practice with various examples to solidify your skills in converting between data types.