In C++, you can convert a string to a float using the `std::stof` function from the `<string>` library. Here's a simple code snippet demonstrating the conversion:
#include <iostream>
#include <string>
int main() {
std::string str = "3.14";
float num = std::stof(str);
std::cout << "The float value is: " << num << std::endl;
return 0;
}
Understanding C++ Data Types
In C++, data types play a crucial role in how we handle and manipulate information. The main categories of data types include:
- Fundamental Data Types: These are the basic building blocks, including `int`, `float`, `double`, and `string`. Each has its own characteristics that define how data is stored and accessed.
- User-Defined Data Types: These types are defined by the user, such as classes and structs, which can encapsulate more complex data structures.
Understanding the differences between these data types helps programmers work effectively with data, ensuring that values are processed correctly according to their nature.

The Need for String to Float Conversion
Converting strings to floats is a common requirement when dealing with user input, parsing data from files, or interacting with APIs that return numeric values as strings. For instance, when a user enters a numeric value in a console application, it is typically received as a string, necessitating conversion to a float for mathematical operations. In addition to user input, reading data from files often returns values formatted as strings, requiring conversion to process these values meaningfully.
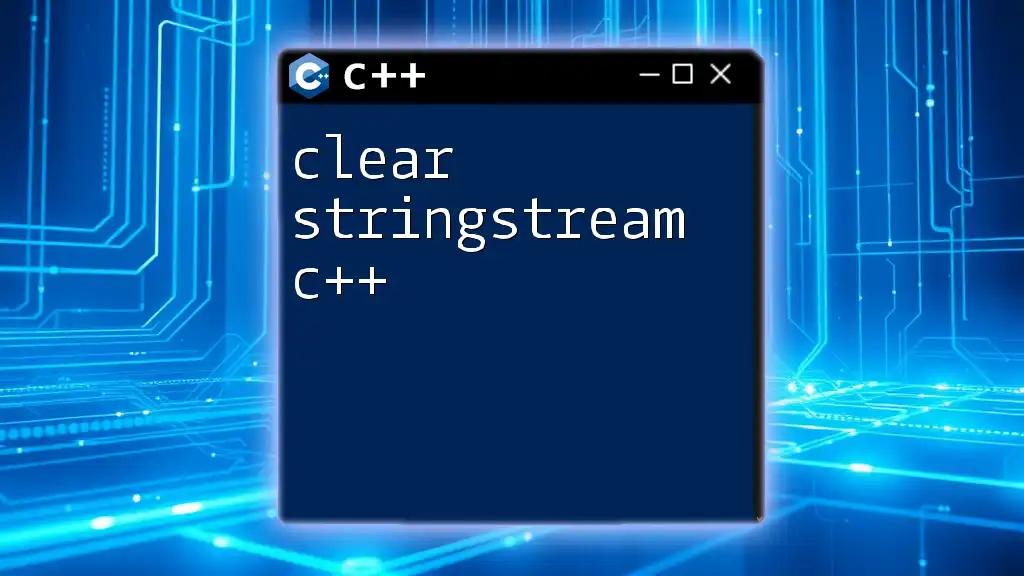
Methods to Convert String to Float in C++
There are several methods available in C++ to convert strings to floats, but two of the most commonly used approaches are `std::stof` and `std::istringstream`.
Using `std::stof`
`std::stof` is a straightforward method to convert a string to a float. It is included in the `<string>` header and enables seamless conversion.
Syntax
float std::stof(const std::string& str, std::size_t* idx = 0);
Basic Example of Using `std::stof`
#include <iostream>
#include <string>
int main() {
std::string str = "3.14";
float num = std::stof(str);
std::cout << "The float value is: " << num << std::endl;
return 0;
}
In this example, the string `"3.14"` is effectively converted to the float value `3.14`.
Explanation of the Example
In the code provided, we include the necessary headers, define a string containing a numeric value, and then call `std::stof` to convert this string to a float. The result is printed to the console.
Handling Exceptions
However, using `std::stof` comes with potential pitfalls that we must handle through exception management. The function can throw standard exceptions such as:
- `std::invalid_argument`: Thrown if the string does not contain a valid float.
- `std::out_of_range`: Thrown if the converted value is too large or too small for a float.
Code Example for Exception Handling
try {
std::string str = "abc"; // Example of invalid input
float num = std::stof(str);
} catch(const std::invalid_argument& e) {
std::cout << "Invalid input: " << e.what() << std::endl;
} catch(const std::out_of_range& e) {
std::cout << "Number out of range: " << e.what() << std::endl;
}
In this example, we showcase exception handling by wrapping our conversion code in a try-catch block. If the input is invalid, we catch the exception and provide feedback to the user, thereby preventing crashes.
Using `std::istringstream`
Another approach to convert strings to floats is using `std::istringstream`, which is part of the `<sstream>` header. This is particularly useful when dealing with larger streams of data or when you want to handle more complex parsing.
Example of Conversion Using `std::istringstream`
#include <iostream>
#include <sstream>
int main() {
std::string str = "2.71";
std::istringstream iss(str);
float num;
iss >> num;
std::cout << "The converted float is: " << num << std::endl;
return 0;
}
This code snippet demonstrates converting the string `"2.71"` into a float using an `istringstream`. The input string is passed to the stream (iss), and the stream extraction operator (`>>`) reads it into the float variable.
Explanation of the Example
In this snippet, we create an `istringstream` object initialized with our string. The extraction operator directly reads the float value into the `num` variable, which is then printed to the console.
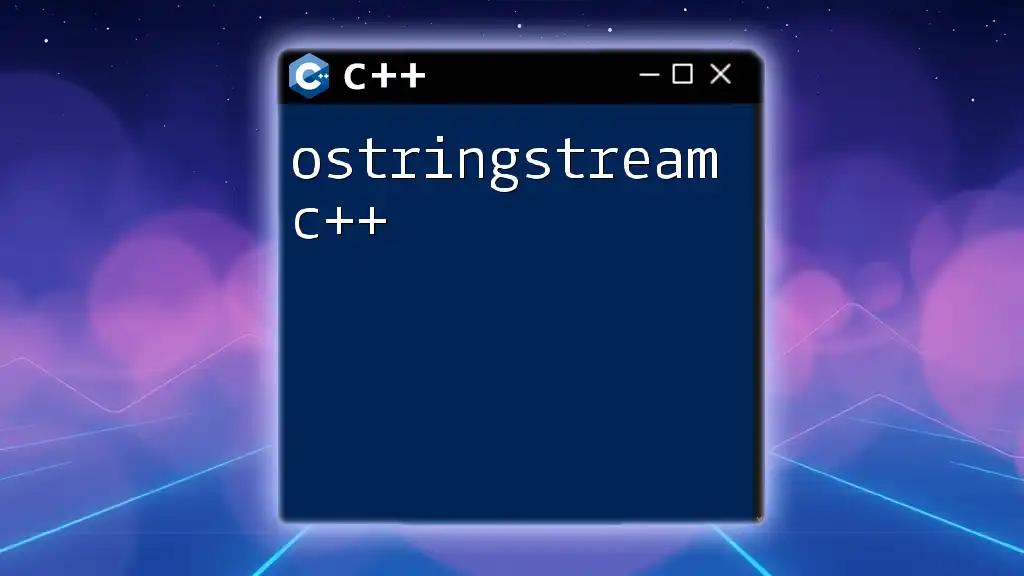
Comparing Methods: `std::stof` vs `std::istringstream`
When choosing between `std::stof` and `std::istringstream` for conversion, consider the following:
-
Pros of `std::stof`:
- Simplicity: The syntax is straightforward and easy to understand.
- Performance: Generally faster for single conversions.
-
Cons of `std::stof`:
- Limited error handling; exceptions must be specifically managed.
-
Pros of `std::istringstream`:
- Flexibility: Can handle multiple values from a single string and performs well with complex parsing.
- Stream-oriented: Good for applications requiring more robust input handling.
-
Cons of `std::istringstream`:
- Slightly more verbose and may introduce additional overhead for simple tasks.
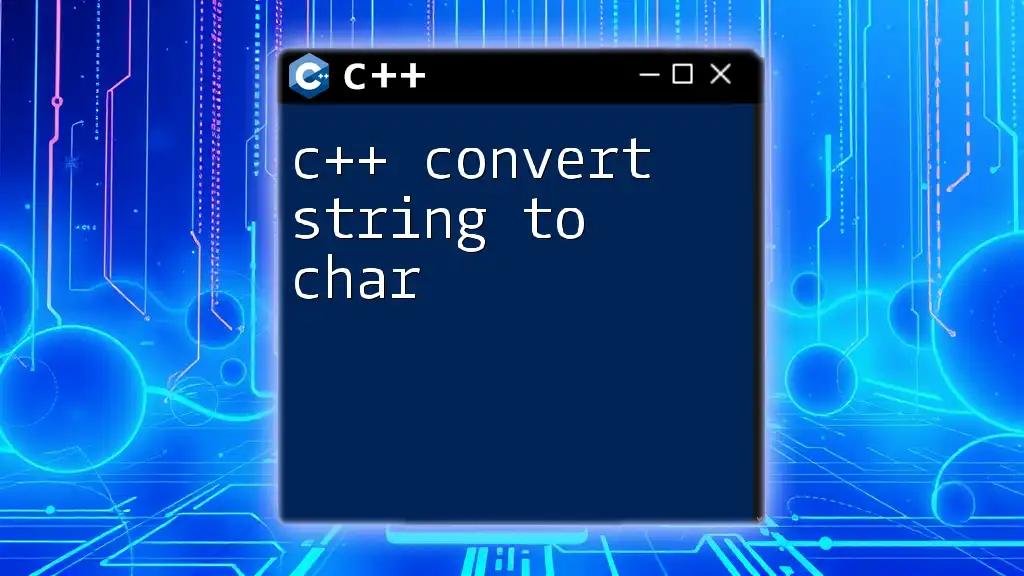
Common Pitfalls to Avoid
While converting a string to float in C++, several common issues can arise. One of the most significant pitfalls is dealing with incorrectly formatted strings. C++ will not automatically handle localizations, such as decimal separators differing across regions (e.g., using a comma instead of a period).
Handling Leading/Trailing Spaces
Ensure you manage strings with leading or trailing spaces, which can lead to `std::invalid_argument` exceptions. An approach to trim these spaces is:
std::string str = " 4.2 ";
str.erase(0, str.find_first_not_of(" \t")); // Trim left
str.erase(str.find_last_not_of(" \t") + 1); // Trim right
float num = std::stof(str);
By trimming spaces, we ensure that the string input is valid, reducing the risk of runtime exceptions.
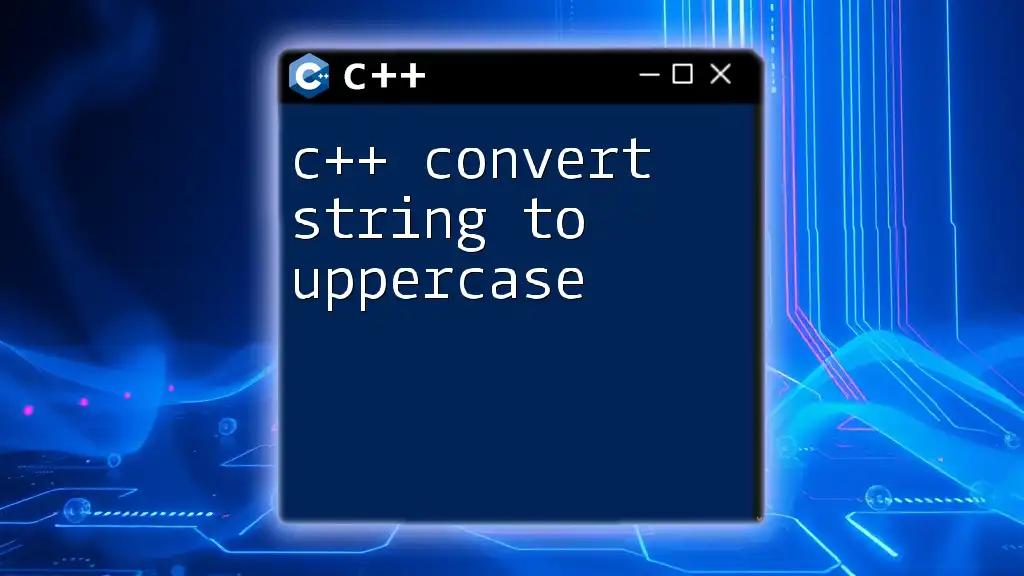
Conclusion
In summary, when you need to convert a string to float in C++, you have effective methods at your disposal in `std::stof` and `std::istringstream`. Each approach has its strengths and caters to different programming scenarios. By understanding the nuances of each method and remaining mindful of common pitfalls, you can perform this conversion reliably and efficiently in your C++ applications.
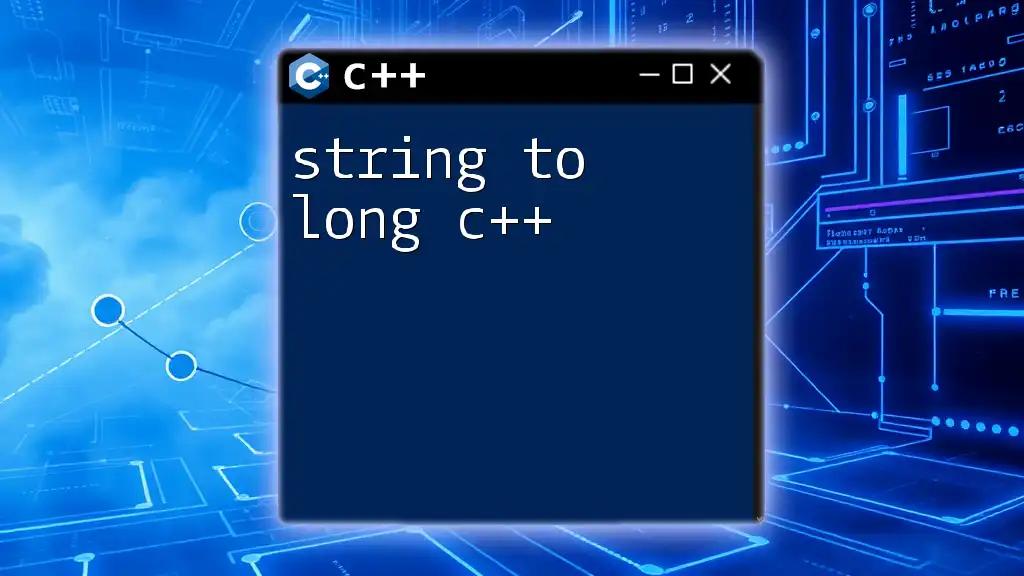
Call to Action
Experimenting with the provided code snippets is a great way to solidify your understanding. Try manipulating the examples, handling edge cases, and incorporating more complex input scenarios. Consider accessing further resources or enrolling in classes to deepen your mastery of C++ commands and data manipulation techniques.
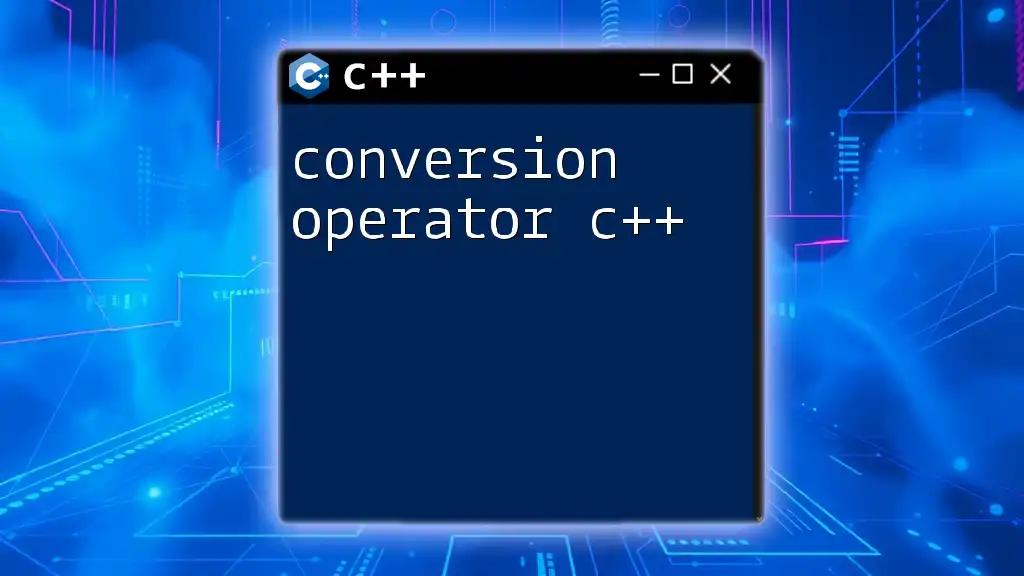
Additional Resources
For further learning, refer to:
- The [C++ reference for `std::stof`](https://en.cppreference.com/w/cpp/string/basic_string/stof).
- Documentation for [`std::istringstream`](https://en.cppreference.com/w/cpp/io/basic_istringstream).
- Coding platforms for practice problems related to string manipulation in C++.