In C++, you can sort a list using the `std::sort` function from the `<algorithm>` header, which arranges the elements in ascending order.
Here's a code snippet demonstrating how to sort a list of integers:
#include <iostream>
#include <algorithm>
#include <list>
int main() {
std::list<int> myList = {5, 3, 8, 1, 4};
myList.sort(); // Using list's member function to sort elements
for (int n : myList) std::cout << n << ' '; // Output: 1 3 4 5 8
return 0;
}
Understanding Lists in C++
What is a List in C++?
In C++, a list is a collection of elements that allows for efficient insertions and deletions. The `std::list` is a doubly linked list provided by the C++ Standard Library, enabling traversal in both forward and backward directions. A unique characteristic of `std::list` is that elements can be added or removed from any position in the list without requiring reallocation or reorganization of other elements, making it ideal for applications where frequent modifications are expected.
Components of C++ Lists
The structure of a list node contains the data and pointers to both the previous and next nodes. This allows for bidirectional navigation and contributes to efficient insertion and deletion operations. Since lists don't allow random access like arrays or vectors, it's important to understand that accessing elements has linear time complexity, which might not be optimal for all use cases.
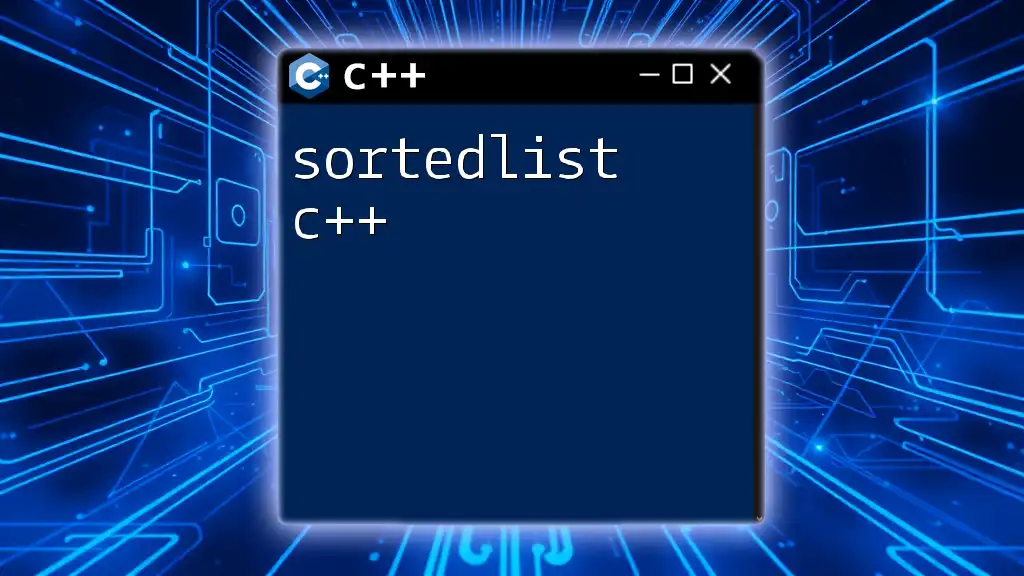
Basics of Sorting in C++
Why Sort?
Sorting is an essential operation in programming, providing order to data and enabling efficient searching and comparison processes. For example, when displaying data to users, sorted lists can significantly improve usability and readability. Additionally, sorting is vital in algorithms like binary search, which require sorted data to function correctly.
Sorting Algorithms Overview
There are several sorting algorithms, each with its unique approach and performance characteristics. Common sorting algorithms include:
- Quicksort: A divide-and-conquer algorithm that is efficient on average but has a worst-case complexity of O(n^2).
- Mergesort: A stable, divide-and-conquer algorithm with a predictable O(n log n) time complexity, often used for large datasets.
- Bubblesort: A straightforward algorithm that repeatedly steps through the list, comparing adjacent elements, but is inefficient for large datasets with a time complexity of O(n^2).
Understanding the time complexity and efficiency of these algorithms helps programmers select the appropriate sorting method for their applications.
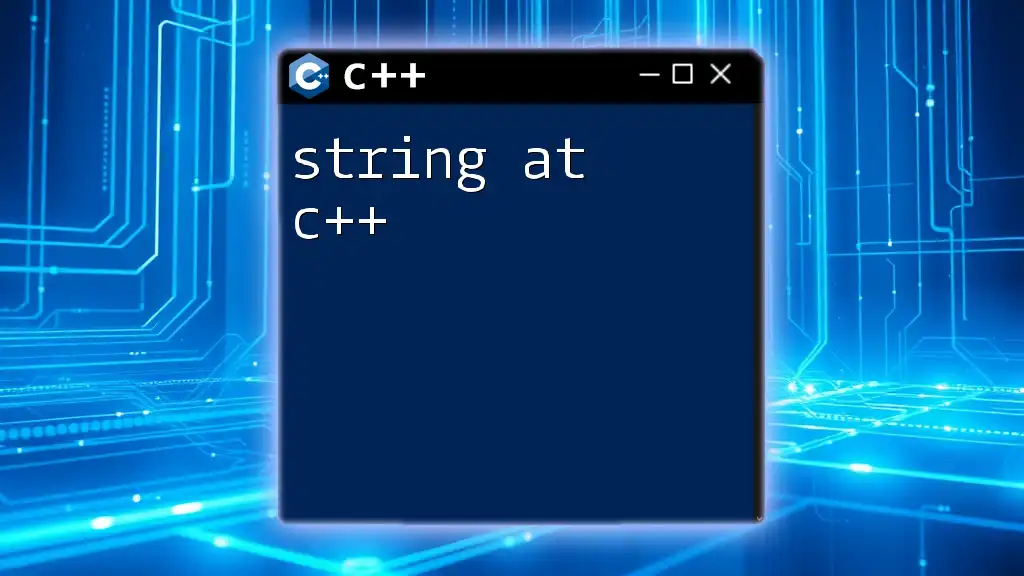
The C++ Standard Library Sorting Functions
The `std::sort` Function
The `std::sort` function is a general-purpose sorting algorithm in C++ that works with random-access iterators, such as those provided by vectors and arrays. It's important to note that `std::sort()` cannot be directly applied to `std::list` as it does not support random access iterators. Although `std::sort` is efficient, using it on lists would require converting the list to a vector, sorting it, and then copying it back. This inefficient process negates the advantages of using linked lists.
The `std::list::sort` Method
For sorting lists in C++, the `std::list` class provides a built-in sorting method, `sort()`, which is optimized for linked lists. This method sorts the elements within the existing list in ascending order using the merge sort algorithm, leveraging the efficient nature of linked lists for sorting operations.
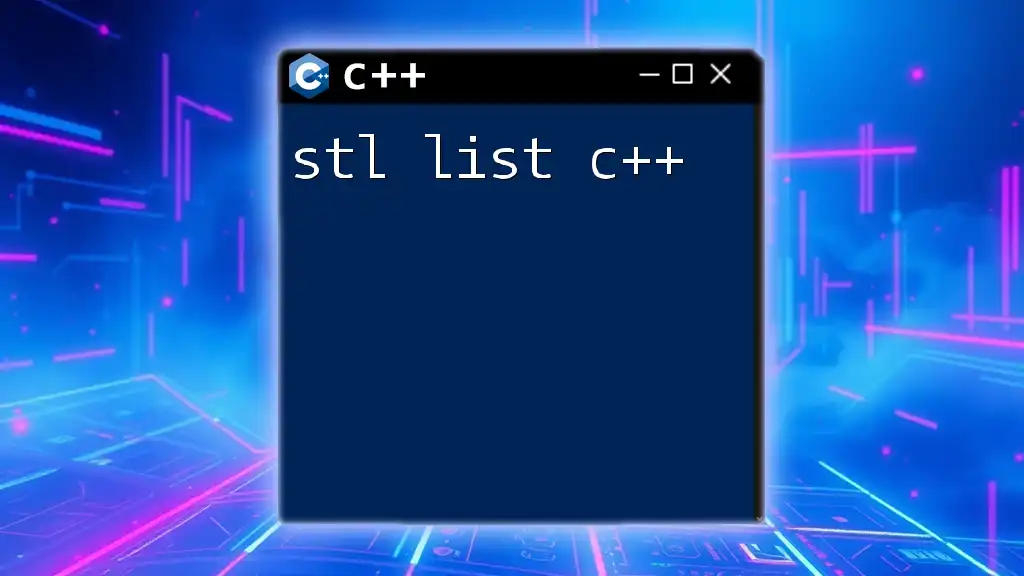
Implementing Sorting on C++ Lists
Using `std::sort` with Lists
While `std::sort` cannot be directly applied to lists, here's how you might use it if you convert your list to a vector first:
#include <iostream>
#include <list>
#include <algorithm>
#include <vector>
int main() {
std::list<int> myList = {5, 3, 8, 1, 2};
std::vector<int> vec(myList.begin(), myList.end()); // Convert list to vector
std::sort(vec.begin(), vec.end()); // Sorting the vector
std::cout << "Sorted list: ";
for (int n : vec) {
std::cout << n << " "; // Displaying sorted elements
}
return 0;
}
This approach, though possible, defeats the purpose of using lists for efficient sorting. Thus, it’s highly encouraged to utilize the built-in capabilities of `std::list`.
Using `std::list::sort()` Method
A more straightforward way to sort a list is by using its `sort()` method directly, which maintains the integrity of the `std::list`:
#include <iostream>
#include <list>
int main() {
std::list<int> myList = {5, 3, 8, 1, 2};
myList.sort(); // Utilizing std::list's built-in sort function
std::cout << "Sorted list: ";
for (int n : myList) {
std::cout << n << " "; // Displaying sorted elements
}
return 0;
}
This method is efficient and easy to use, sorting the list in-place without copious overhead.
Sorting a List in Descending Order
By default, `std::list::sort()` arranges elements in ascending order, but you may want to sort in descending order or apply a custom sort criteria. You can achieve this by providing a comparator function:
#include <iostream>
#include <list>
bool customComparator(int a, int b) {
return a > b; // For descending order
}
int main() {
std::list<int> myList = {5, 3, 8, 1, 2};
myList.sort(customComparator); // Sorting the list using a custom comparator
std::cout << "Sorted list (descending): ";
for (int n : myList) {
std::cout << n << " "; // Displaying sorted elements
}
return 0;
}
The ability to use custom comparison functions provides developers with the flexibility to dictate how data should be sorted based on specific requirements.
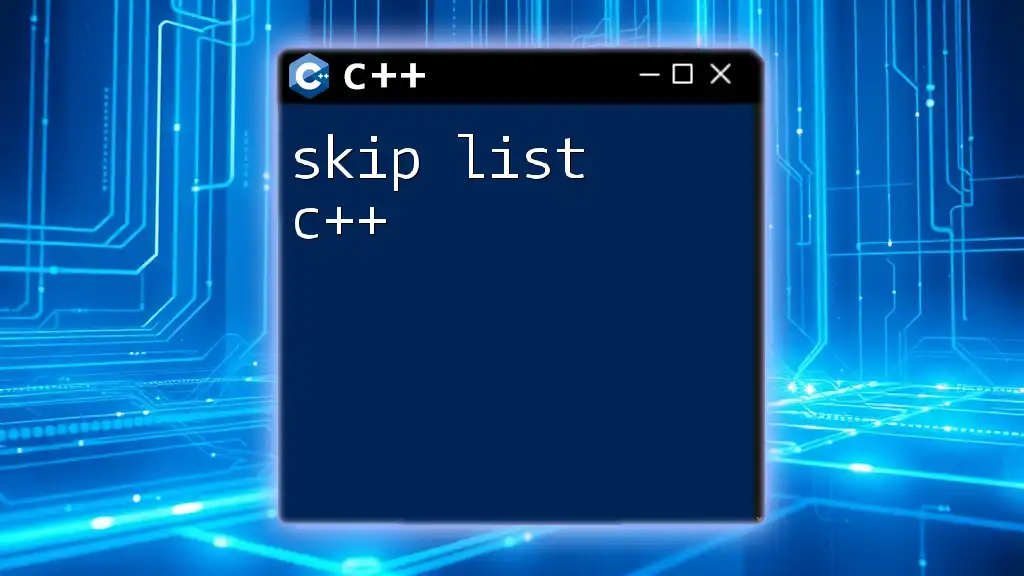
Error Handling and Edge Cases
Common Mistakes When Sorting Lists
One common mistake is attempting to sort an empty list. While `std::list::sort()` executes without errors, the result will be an unchanged empty list, potentially leading to confusion. Additionally, failing to validate the elements type or comparator function can introduce runtime errors.
Handling Edge Cases
Sorting linked lists containing circular references can lead to infinite loops. It's critical to ensure your list is properly established before executing sorting methods. Furthermore, take care to maintain the integrity of your data and prevent potential corruption during modifications.
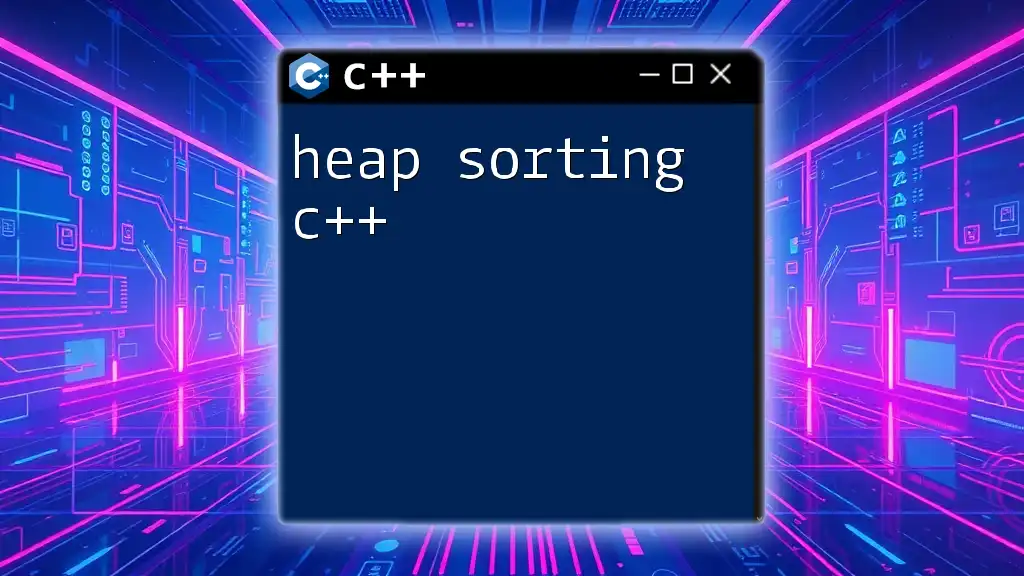
Conclusion
In summary, sorting a list in C++ is a fundamental skill that every programmer should master. Understanding when to use `std::sort` versus `std::list::sort()` is paramount, as is grasping the underlying mechanics of C++ lists. By employing these sorting techniques effectively, one can significantly enhance the performance and usability of applications.
For those looking to deepen their knowledge, consider experimenting with different data types and custom comparator functions to further develop your C++ skills. Happy coding!
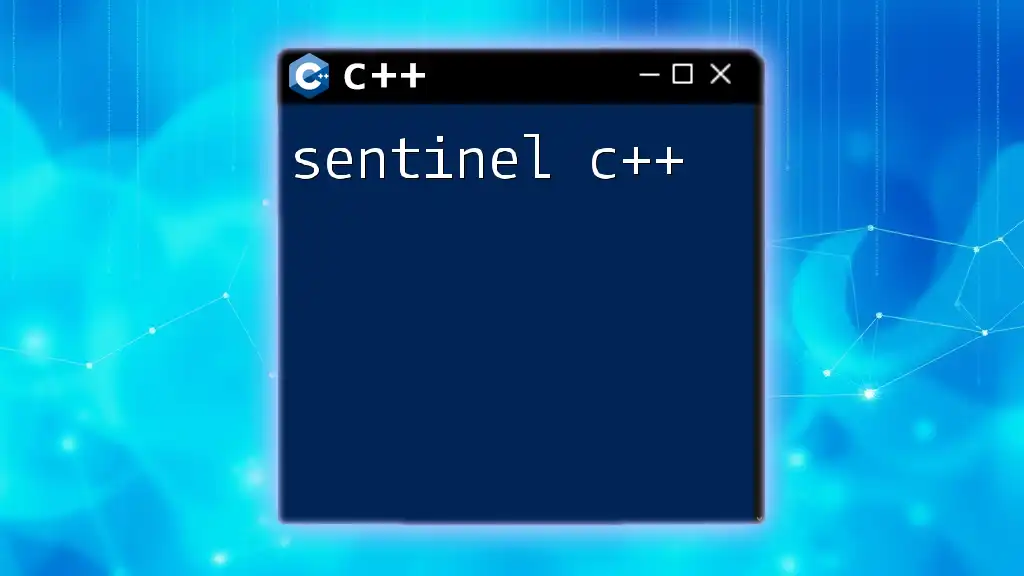
Additional Resources
For further exploration of sorting techniques and C++ lists, refer to the official C++ documentation on the Standard Library, and check resources dedicated to advanced sorting algorithms. Engaging in practical exercises can also greatly help refine your skills in sorting lists in C++.