Counting in C++ can be accomplished using a simple loop to increment a counter variable and output its value, as illustrated in the following example:
#include <iostream>
using namespace std;
int main() {
for (int i = 1; i <= 10; ++i) {
cout << i << endl; // Output numbers from 1 to 10
}
return 0;
}
Understanding Counting in C++
What is Counting?
Counting in programming refers to the process of keeping track of numbers, indexes, or occurrences. In C++, counting is foundational for creating loops, developing algorithms, and manipulating data. Simply put, counting allows programmers to manage information efficiently and perform tasks iteratively.
Basic Counting Concepts in C++
In C++, counting primarily involves the use of variables and data types, especially integer types such as `int` and `unsigned int`. Understanding how to work with these types is crucial for developing robust counting mechanisms in your code.
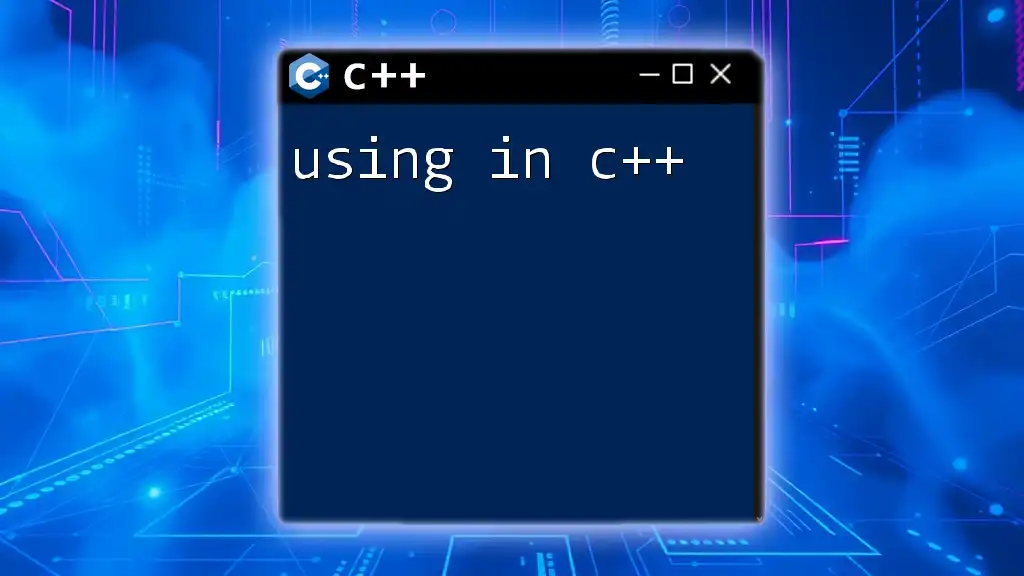
Counting Techniques in C++
Using Loops for Counting
For Loops
The `for` loop is one of the most common structures for counting in C++. It allows you to specify an initialization, a condition, and an incrementing mechanism in a single line, making it elegant and concise.
Example: Counting from 1 to 10 using a `for` loop:
for (int i = 1; i <= 10; i++) {
std::cout << i << " ";
}
In this code:
- The loop initializes the variable `i` to 1.
- It continues as long as `i` is less than or equal to 10.
- After each iteration, `i` is incremented by one. This will produce the output: `1 2 3 4 5 6 7 8 9 10`.
While Loops
The `while` loop is another option for counting, especially when the number of iterations is not predetermined.
Example: Counting down from 10 to 1 using a `while` loop:
int count = 10;
while (count > 0) {
std::cout << count << " ";
count--;
}
This code works as follows:
- It sets `count` to 10.
- The loop runs as long as `count` is greater than zero, printing the current value and then decrementing it. This results in the output: `10 9 8 7 6 5 4 3 2 1`.
Recursive Counting
Recursion is a programming technique where a function calls itself. Counting through recursion can be elegant but should be used carefully to avoid excessive memory consumption.
Example: A factorial function counts by multiplying numbers in a recursive manner:
int factorial(int n) {
if (n <= 1) return 1;
return n * factorial(n - 1);
}
Here, when `factorial(5)` is called, it recursively computes `5 * factorial(4)`, and so on, until it reaches `factorial(1)`. The final result is `120`, representing `5!`.
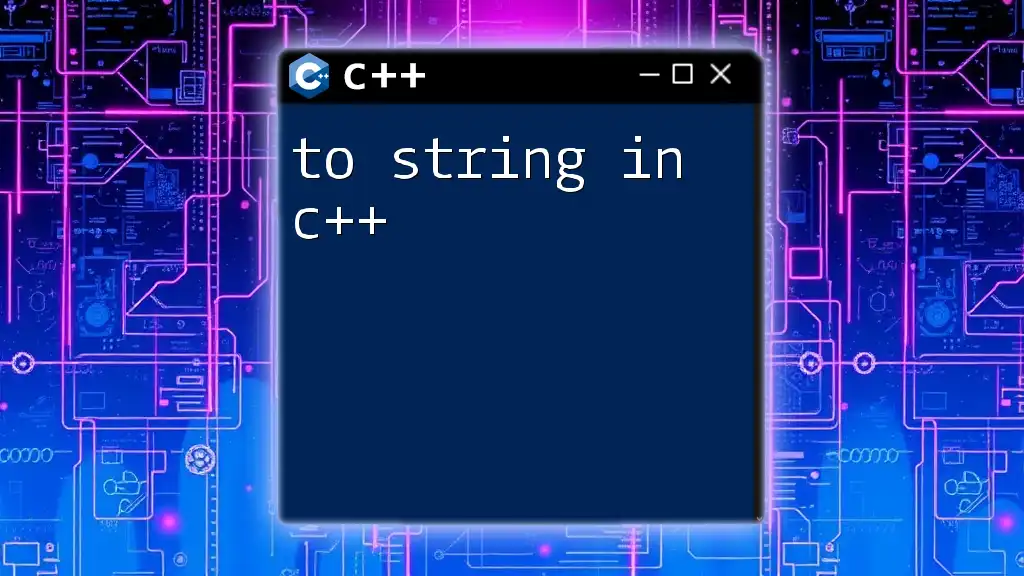
Advanced Counting Techniques
Counting with Arrays
Initializing and Using Arrays
Arrays in C++ provide a way to store a collection of elements, making them useful for counting occurrences of data.
Example: Counting occurrences of numbers in an array:
int counts[10] = {0}; // Initializes an array of size 10
for (int i = 0; i < 100; i++) {
int num = rand() % 10; // Random number between 0 and 9
counts[num]++;
}
In this code snippet:
- An array named `counts` is initialized to hold ten elements, all set to zero.
- The loop generates 100 random numbers between 0 and 9 and increments the corresponding index in the `counts` array. At the end, `counts` will reflect how many times each number appeared.
Counting Algorithms
Linear Search for Counting
The linear search algorithm can also be utilized for counting specific values within an array.
Example: Count the number of occurrences of a specific value in an array:
int countOccurrences(int arr[], int size, int value) {
int count = 0;
for (int i = 0; i < size; i++) {
if (arr[i] == value) count++;
}
return count;
}
This function iterates through the array `arr` and counts how many times `value` appears, returning the count. This approach is straightforward but can be less efficient for large datasets.
Efficient Counting with Maps
The `std::map` data structure provides an efficient way to count occurrences of elements, offering faster lookups and insertions.
Example: Using a `map` to count character occurrences in a string:
#include <map>
#include <string>
std::map<char, int> charCount;
std::string str = "example";
for (char c : str) {
charCount[c]++;
}
In this example:
- A `map` called `charCount` is utilized to keep track of how many times each character appears in the string `str`.
- This approach provides both efficiency and clarity, making it easier to handle counting in more complex datasets.
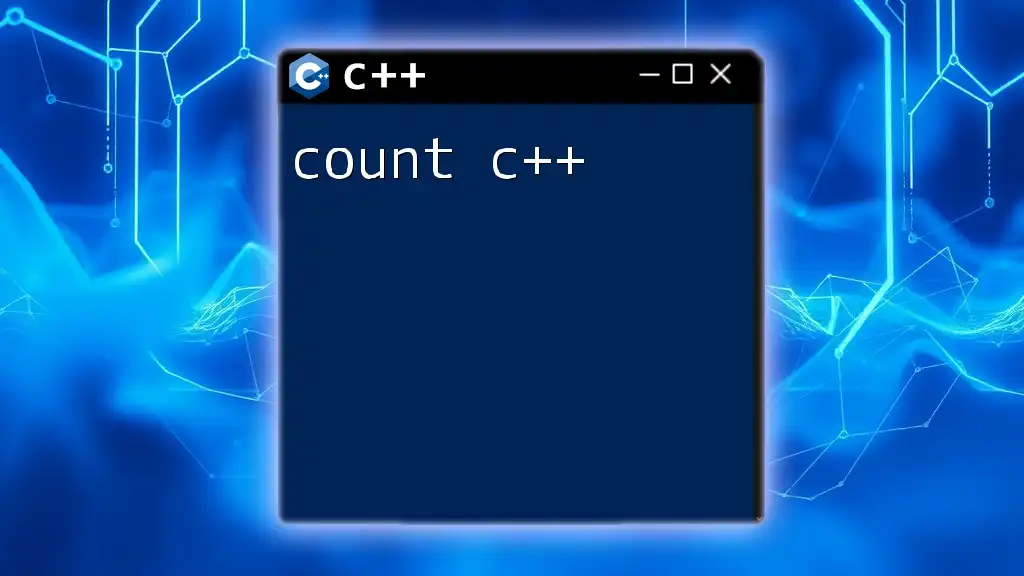
Common Mistakes in Counting
Off-by-One Errors
One of the most prevalent mistakes in counting is the off-by-one error, where loops and counts start or end at incorrect values. This can lead to missing or repeating values in your output.
Tip: Always double-check your loop bounds and conditions. Using descriptive variablenames can help clarify the intended range.
Initializing Counters
Proper initialization of counters is crucial. Forgetting to initialize a counter can lead to undefined behavior or misleading results. Always initialize your counting variables to a known value, typically zero.
Example: Instead of:
int count; // Undefined behavior if used before assignment
You should initialize as:
int count = 0; // Safe initialization
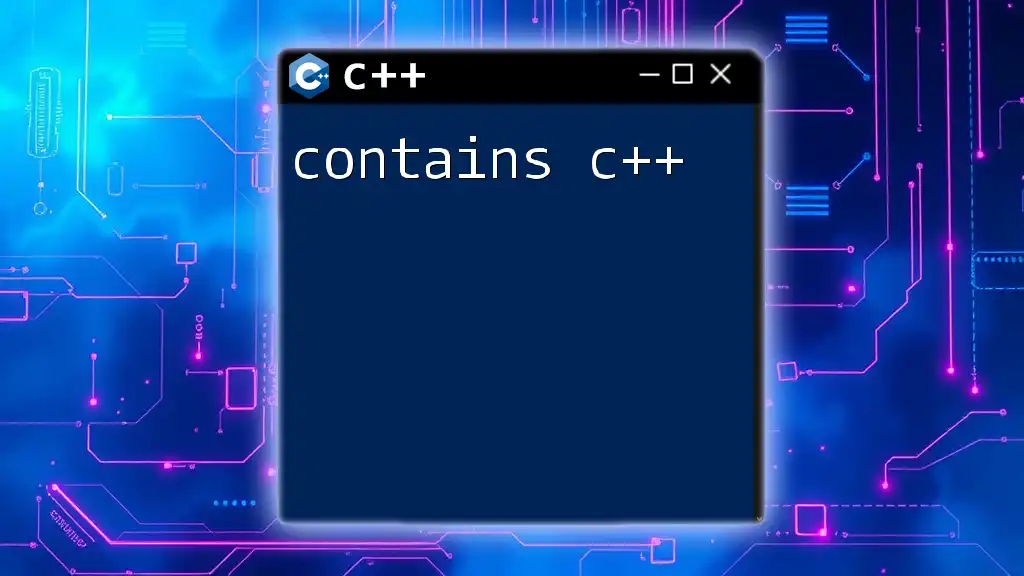
Conclusion
In this guide, we explored various concepts and techniques related to counting in C++. From basic loops and recursion to advanced counting using arrays and maps, counting serves as a foundation for effective programming. Understanding these concepts not only strengthens your ability to manage data but also enhances your skills in algorithm development.
As you practice counting in C++, remember to avoid common pitfalls like off-by-one errors and properly initialize your counters. Continue experimenting and applying these techniques to specific problems, and you will become proficient in managing counts effectively across different scenarios.
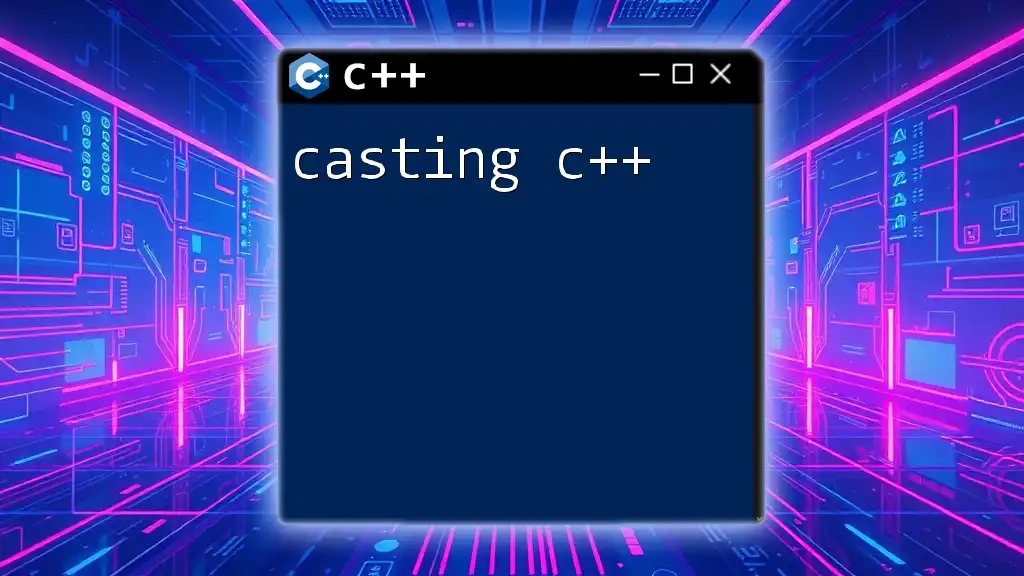
Additional Resources
- For comprehensive documentation on C++, refer to the official [C++ Documentation](https://en.cppreference.com).
- Consider resources like books or online courses focusing on intermediate and advanced C++ programming concepts to deepen your knowledge.
- Engage with coding platforms like LeetCode or HackerRank to practice counting algorithms and challenges related to the topic.