Mapping in C++ refers to the use of associative containers like `std::map` and `std::unordered_map` to store key-value pairs, enabling efficient data retrieval based on unique keys. Here's a simple example:
#include <iostream>
#include <map>
int main() {
std::map<std::string, int> ageMap;
ageMap["Alice"] = 30;
ageMap["Bob"] = 25;
for (const auto& pair : ageMap) {
std::cout << pair.first << " is " << pair.second << " years old." << std::endl;
}
return 0;
}
Understanding the Basics of Mapping
Definition of Mapping
In programming, mapping refers to the association between keys and values. A key acts as a unique identifier in this association, allowing for efficient retrieval of its corresponding value. When one thinks of mapping, think of a dictionary where each word (key) has a definition (value).
Use Cases for Mapping
Mappings are prevalent in many real-world applications such as:
- Storing relational data, where a unique identifier (like a user ID) maps to specific user information (like a name or email).
- Frequency counting, where items are counted based on their occurrence, allowing for efficient data analysis.
- Configuration and settings management, where different settings are retrieved via easily identifiable keys.
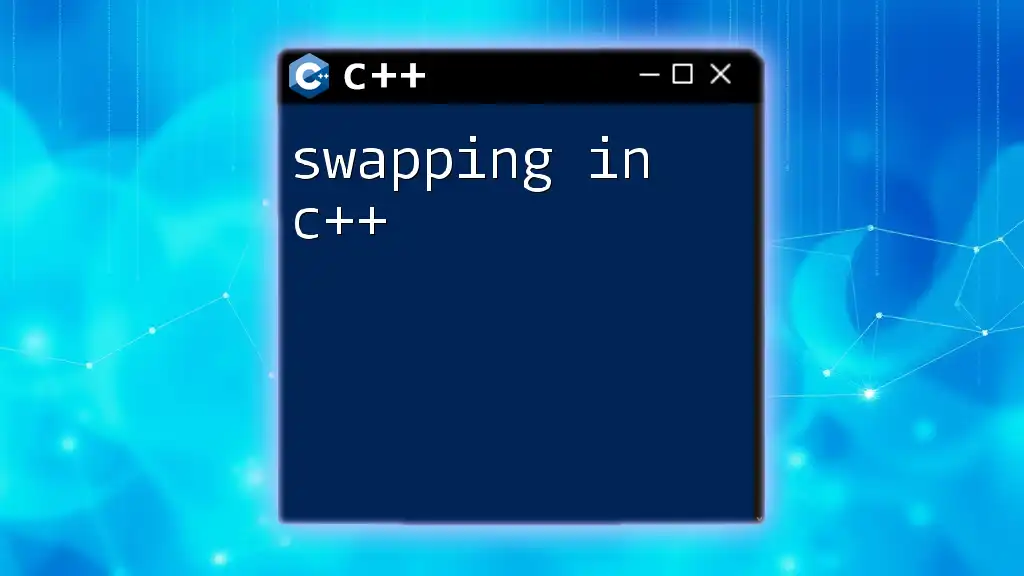
Containers in C++ for Mapping
C++ provides a robust Standard Template Library (STL) which includes several containers that facilitate mapping.
std::map
`std::map` is an ordered container that allows key-value pairs to be stored in a sorted manner. The keys are unique, and accessing values using keys is efficient, typically done in logarithmic time complexity. This makes `std::map` ideal for scenarios where order matters.
std::unordered_map
In contrast, `std::unordered_map` uses hash tables, offering average constant time complexity for key-based operations. This type of mapping is beneficial when the order of elements is not a priority, and performance is key.
std::multimap
`std::multimap` allows multiple associations for the same key. This is useful when you need to store several values that are linked to a singular key. For instance, if you want to store several phone numbers for a single person, `std::multimap` would facilitate that.
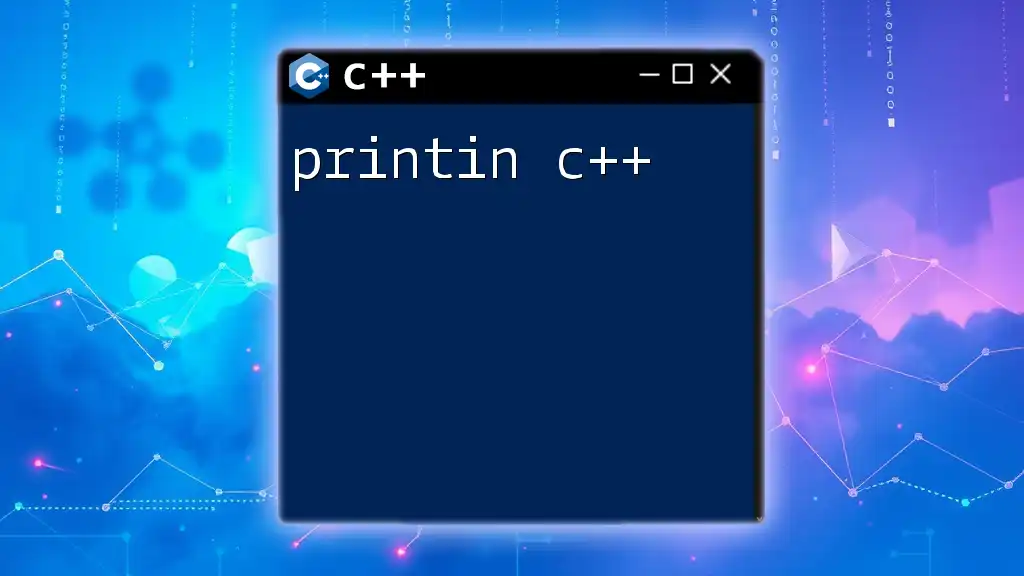
Key Features and Characteristics
Ordered vs Unordered Maps
The most significant difference between `std::map` and `std::unordered_map` lies in their ordering:
- `std::map` keeps elements sorted based on the key's value.
- `std::unordered_map` does not guarantee any specific order.
Complexity of Operations
Understanding the performance-related aspects of mapping is essential. The average case complexity for operations on `std::map` (insertions, deletions, lookups) is O(log n), while for `std::unordered_map`, it is O(1). However, in rare scenarios where hash collisions are high, the worst case for `std::unordered_map` can degrade to O(n).
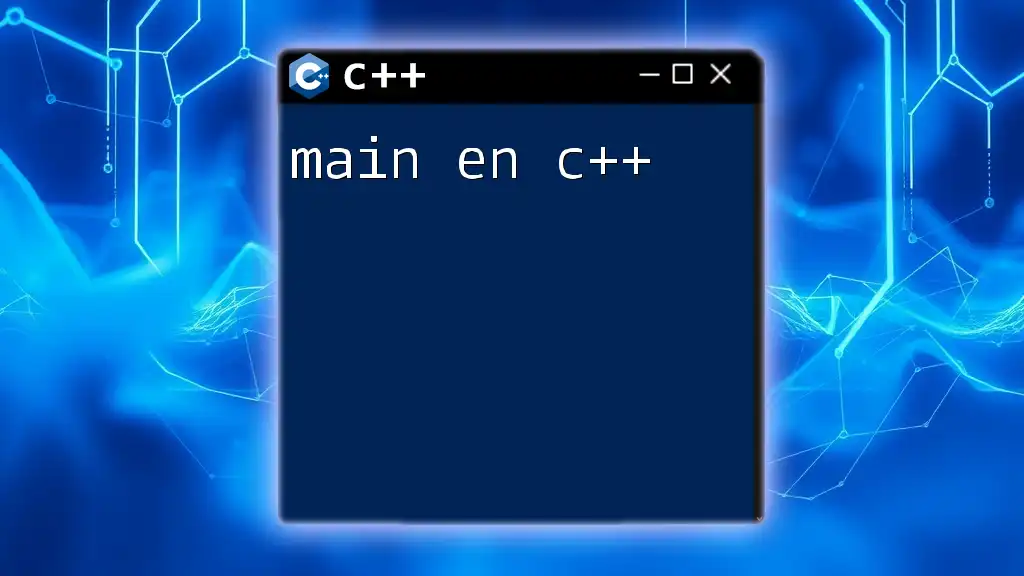
Basic Operations with Maps
Creating and Initializing Maps
Creating empty maps is straightforward in C++. Here’s how you can initialize both types:
std::map<int, std::string> myMap;
std::unordered_map<int, std::string> myUnorderedMap;
Inserting Elements
Elements can be added to maps using various methods. Here’s an example of inserting elements:
myMap.insert(std::make_pair(1, "One"));
myMap[2] = "Two"; // Another way to insert
Accessing Elements
Accessing the value of a key is also effortless. You can use the key to retrieve its associated value like this:
std::cout << myMap[1]; // Outputs: One
Iterating Through Maps
Iterating over the elements in a map can be achieved several ways.
- Using Iterators:
for(auto it = myMap.begin(); it != myMap.end(); ++it) {
std::cout << it->first << ": " << it->second << std::endl;
}
- Using a Range-Based for Loop:
for(const auto& pair : myMap) {
std::cout << pair.first << ": " << pair.second << std::endl;
}
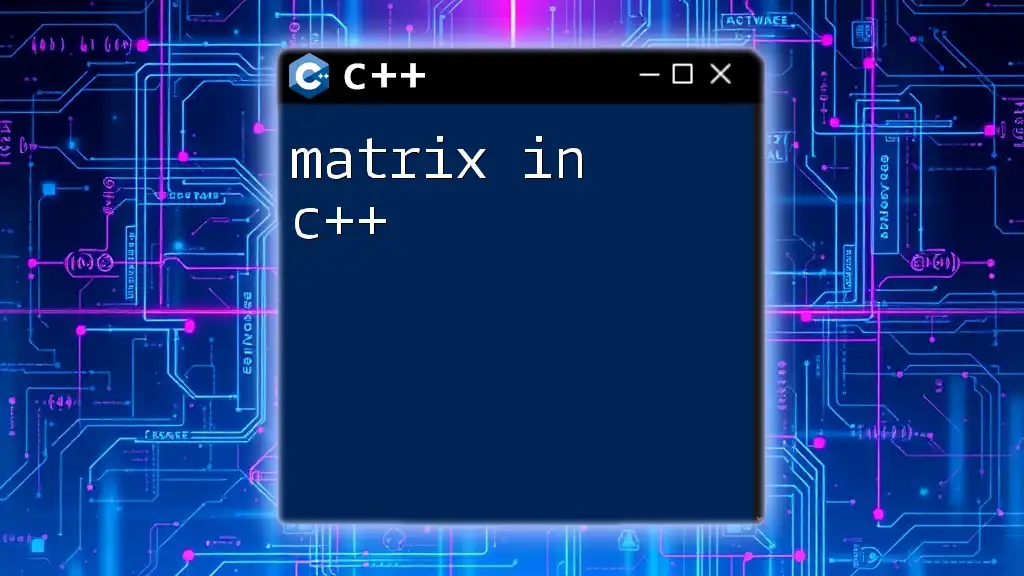
Advanced Features of Maps
Finding Elements
You can check for the existence of a key using the `find` method. Here’s how it works:
auto it = myMap.find(2);
if(it != myMap.end()) {
std::cout << "Found: " << it->second;
}
Removing Elements
To remove an element, you can use the `erase` function. For example:
myMap.erase(1); // Removes the element with key '1'
Clearing the Map
If you wish to remove all elements, simply call `clear`:
myMap.clear();
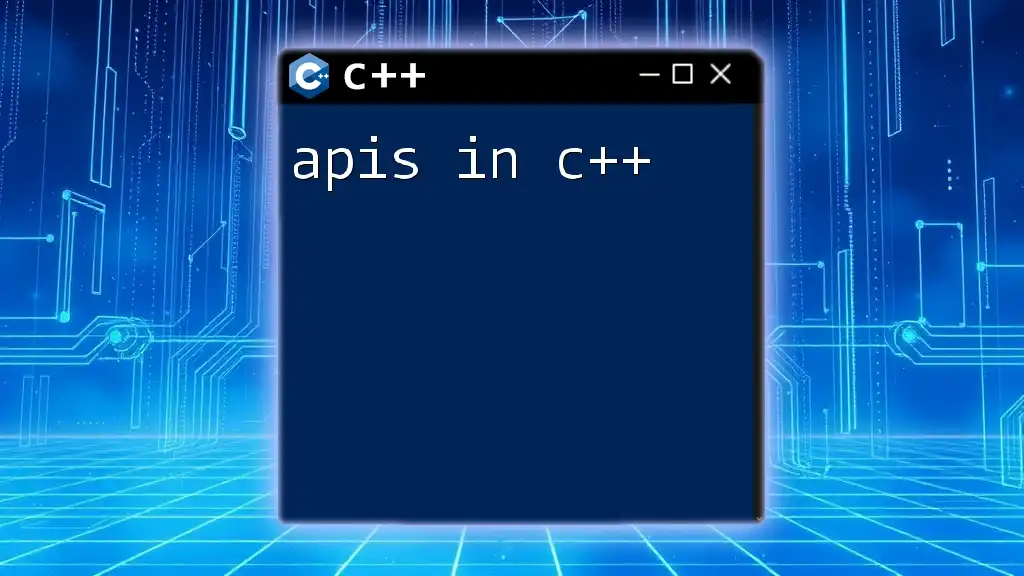
Real-World Applications of Mapping
Storing Settings or Configuration
Mappings can effectively store application configurations. For example, using `std::map` to hold various configuration parameters can facilitate easy access based on configuration names.
Counting Frequencies
A practical application of mappings is counting the occurrence of elements in a list. Here’s how that can be done efficiently:
std::unordered_map<std::string, int> frequencyMap;
std::string words[] = {"apple", "banana", "apple", "orange"};
for(const auto& word : words) {
frequencyMap[word]++;
}
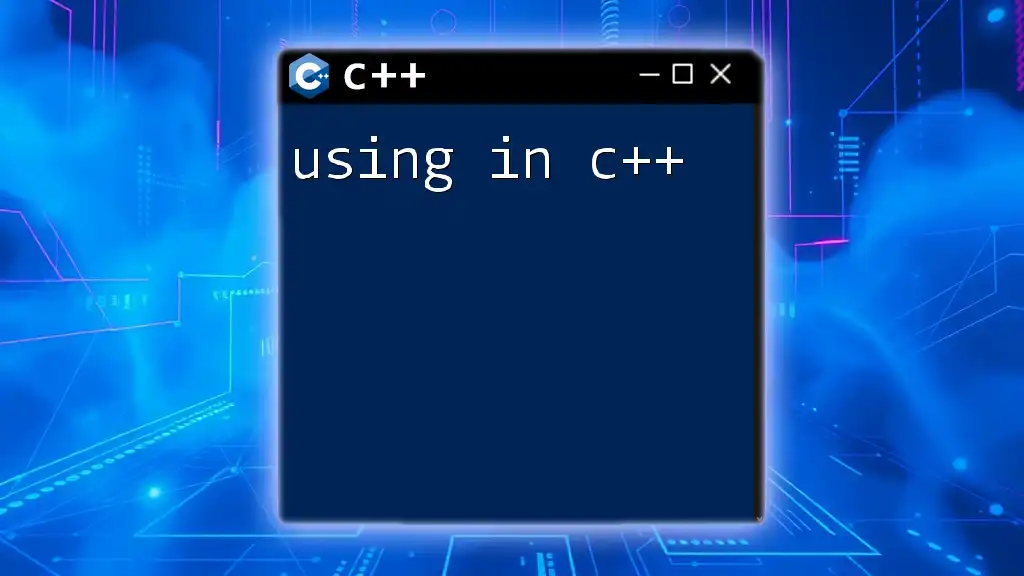
Performance Considerations
Memory Management
Using mappings consumes memory as they store pairs of keys and values. An understanding of the underlying storage mechanisms is crucial to effective memory management.
Optimizing Performance
Choosing the right type of mapping based on the size of data and type of access patterns will optimize performance. For smaller datasets, `std::map` may provide sufficient speed with the added advantage of order, while `std::unordered_map` could potentially yield faster results for larger datasets without concern for order.
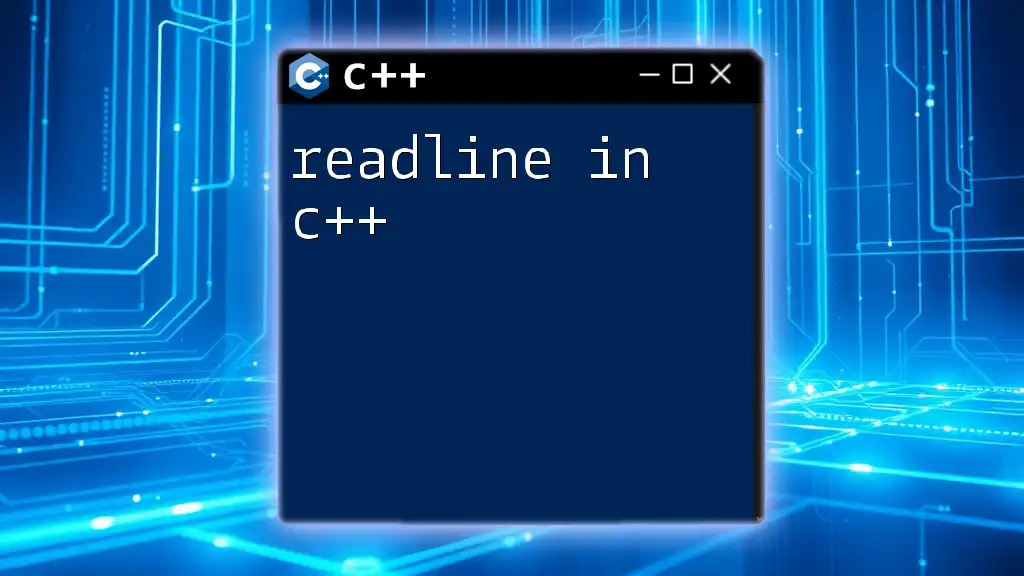
Conclusion
In summary, mapping in C++ is an invaluable tool for developers. Understanding how different containers operate, how to manipulate them, and their performance implications are essential steps to becoming proficient in using mappings. As you continue to learn, experimenting with these constructs will deepen your practical understanding, paving the way for more advanced programming techniques.
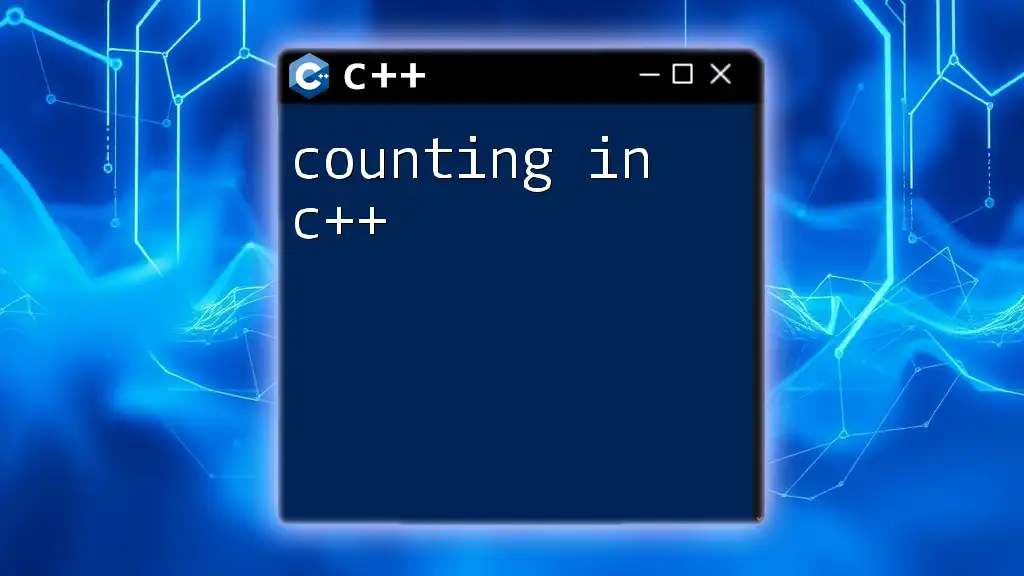
Additional Resources
To further your understanding, consider exploring:
- Recommended C++ books and online courses that delve into STL and advanced mapping techniques.
- Community forums and platforms like Stack Overflow that can provide real-time assistance and a network of fellow learners to share insights with.