In C++, the `append` function of the `std::string` class allows you to concatenate additional characters or another string to an existing string efficiently.
#include <iostream>
#include <string>
int main() {
std::string str = "Hello";
str.append(", World!"); // Appending to the string
std::cout << str << std::endl; // Output: Hello, World!
return 0;
}
Understanding the C++ std::string Class
The `std::string` class is a powerful and flexible way to handle text in C++. Unlike C-style strings, which are essentially arrays of characters terminated by a null character, `std::string` provides a more secure and convenient abstraction. Features such as dynamic resizing, built-in memory management, and a rich set of functions make it the preferred choice for managing strings in modern C++ programming.
Key features of `std::string` include:
- Automatic memory management: The `std::string` class takes care of memory allocation and deallocation, so developers don’t have to manage memory manually.
- Versatile methods: Includes numerous member functions for comparison, concatenation, and searching, making string manipulation easier.
- Dynamic sizing: It can grow and shrink as needed, allowing for ease of use without worrying about buffer overflows.
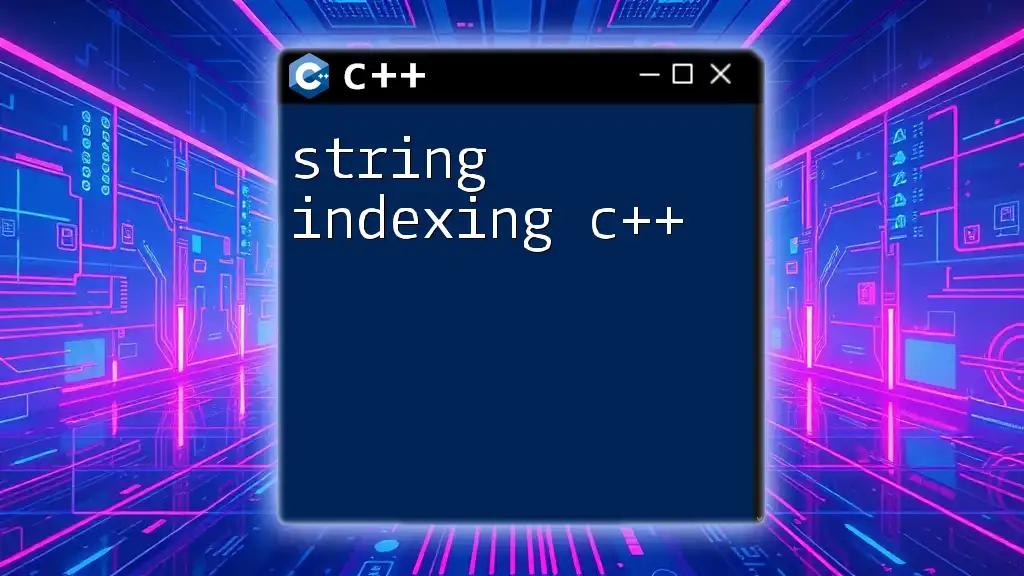
The Basics of Appending Strings in C++
Appending strings is an essential operation when building and modifying text data in your applications. In C++, appending is the act of adding one string to another, which can enhance the readability of your code compared to traditional concatenation methods. While concatenation usually implies creating a new string from two others, appending modifies the existing string.
Why Use Append Functions?
The motivation for using appending functions, such as `append`, is multi-faceted:
- Efficiency: Appending to an existing string can be more efficient than creating a new string every time you need to combine text.
- Convenience: The `append` method provides a clear and meaningful way to read and understand the code related to string construction.

Using the C++ `append` Function
What is the `append` Function in C++?
The `append` function in C++ is a member function of the `std::string` class that allows you to concatenate additional characters, strings, or even multiple strings to the end of an existing string. Its syntax is straightforward:
std::string& append(const std::string& str);
Types of Appends with the `append` Function
Appending a Standard String
The `append` function can easily add a standard `std::string` to another. Here’s an example to illustrate this:
std::string str1 = "Hello";
std::string str2 = " World!";
str1.append(str2);
std::cout << str1; // Output: Hello World!
In this code, `str2` is appended to `str1`, resulting in a seamless combination of both strings.
Appending C-Strings
You can also append C-style strings (null-terminated character arrays) effectively. Consider this example:
std::string str = "Hello";
str.append(" World!");
std::cout << str; // Output: Hello World!
Here, the C-string `" World!"` is appended directly to `str`, demonstrating the flexibility of the `append` function.
Appending Multiple Strings
Appending multiple strings in succession is also straightforward. You can chain calls to `append` to combine several strings efficiently:
std::string str1 = "I ";
std::string str2 = "love ";
std::string str3 = "C++!";
str1.append(str2).append(str3);
std::cout << str1; // Output: I love C++!
In this example, we see clear readability and understanding, making the code intuitive to follow.
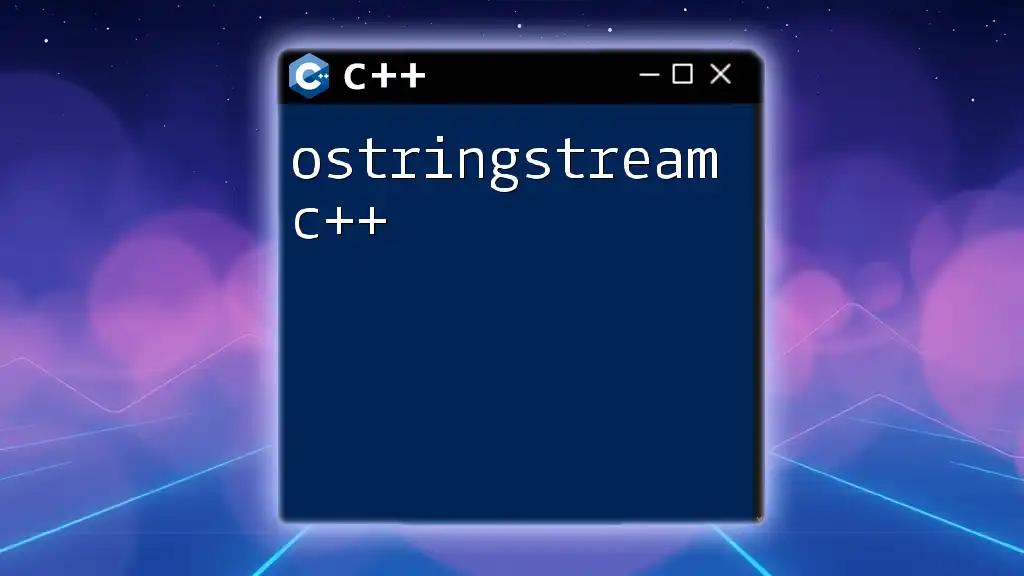
Additional Append Methods in C++
Using the `operator+=` for String Append
An alternative to the `append` function is the `+=` operator, which offers an even more concise way to append strings. Using `+=` has several advantages, particularly in terms of simplicity:
std::string str = "Goodbye";
str += " World!";
std::cout << str; // Output: Goodbye World!
This example shows that the `+=` operator can be an elegant solution for appending strings while making the intent clear to anyone reading the code.
Appending Characters
The `append` function isn't limited to strings; you can also append individual characters or repeated characters. Here’s how:
std::string str = "A";
str.append(3, 'B'); // Appending 'B' three times
std::cout << str; // Output: AB BB
This demonstrates `append`'s versatility, allowing you to build strings dynamically.

Performance Considerations When Appending Strings
When working with string appending, it’s essential to consider performance implications. Repeatedly appending strings can cause reallocations, which may impact efficiency. Here are some best practices:
- Reserve space: Use `str.reserve()` if you know the total length of your string in advance.
- Minimize copies: Techniques that minimize copying can improve performance; for instance, use references where appropriate.
Understanding memory management and resizing strategies allows you to optimize your string operations, ensuring your applications run smoothly.

Real-World Applications of String Append
In real-world applications, string appending is invaluable. Key use cases include:
- File handling: When constructing paths or file names dynamically, string appending becomes crucial.
- Creating dynamic messages: For generating logs or output messages tailored to user inputs, appending strings allows precise messaging.
- Building user interfaces: From generating HTML to formatting output for consoles, string manipulation plays an essential role.

Common Mistakes to Avoid When Appending Strings
When using string append methods, it's important to be aware of common pitfalls that can lead to bugs or inefficiencies:
- Handling null pointers: Failing to check for null strings can lead to crashes.
- Ignoring capacity: Improperly managing string capacity can lead to performance issues due to excessive reallocations.
- Overusing temporary strings: Creating unnecessary temporary strings can add overhead, so be mindful of memory consumption.

Conclusion
In conclusion, understanding how string append in C++ works is essential for writing efficient and readable code. The dynamic capabilities of the `std::string` class, along with the various append methods, enable developers to manipulate text data effectively. By mastering these concepts, you equip yourself with the necessary tools to streamline string operations in your applications.

Additional Resources
For those interested in diving deeper into C++, consider exploring books and online courses. Engaging with online communities and forums can also facilitate deeper understanding and place you in touch with others on the same learning journey.
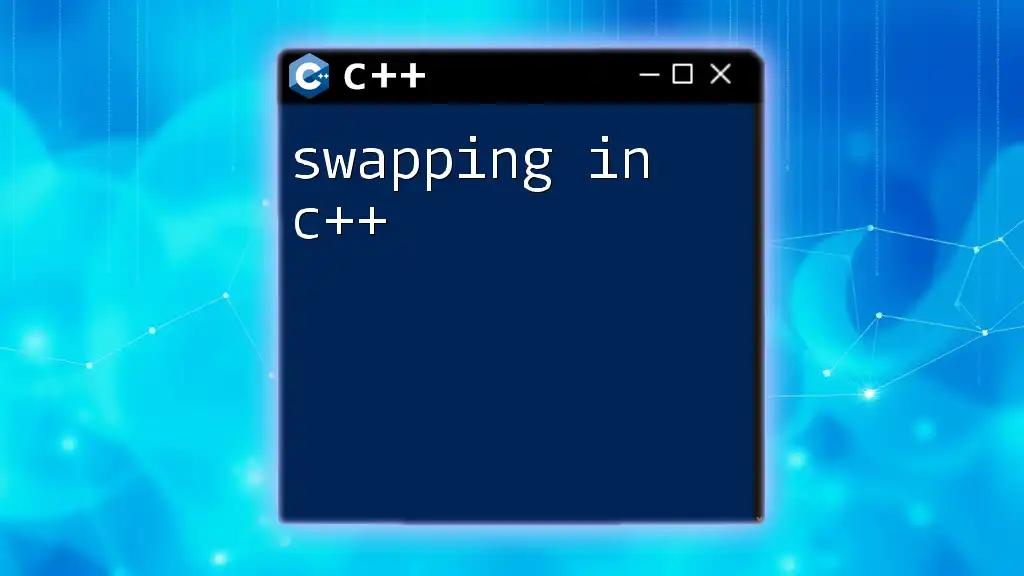
Call to Action
We'd love to hear about your experiences with string manipulation in C++. Share your stories or questions with us and consider signing up for our courses focused on mastering essential C++ commands!