A string parser in C++ is a utility that processes and interprets strings based on specific criteria, allowing you to extract or manipulate data efficiently.
Here’s an example code snippet demonstrating a simple string parser that splits a string by spaces:
#include <iostream>
#include <sstream>
#include <vector>
int main() {
std::string input = "Hello World from C++";
std::istringstream ss(input);
std::string word;
std::vector<std::string> words;
while (ss >> word) {
words.push_back(word);
}
for (const auto& w : words) {
std::cout << w << std::endl;
}
return 0;
}
Understanding Strings in C++
What is a String in C++?
In C++, a string is essentially a sequence of characters used to represent text. C++ offers two primary ways to handle strings: C-style strings, which are arrays of characters ended by a null character (`'\0'`), and the C++ `std::string` class, which provides a more flexible and user-friendly approach to handle strings.
Unlike C-style strings, `std::string` simplifies many operations, such as concatenation, comparison, and substring extraction, making it ideal for modern C++ applications.
Why Parse Strings?
String parsing is the process of analyzing a string to extract meaningful data. This practice is fundamental in various applications, such as:
- Data processing: Extracting information from formatted text (CSV files, logs, etc.)
- User input handling: Processing and validating user inputs in applications
- Communication protocols: Parsing messages across network communications
However, challenges frequently arise, such as handling different delimiters, managing varying formats, and ensuring robust error handling.
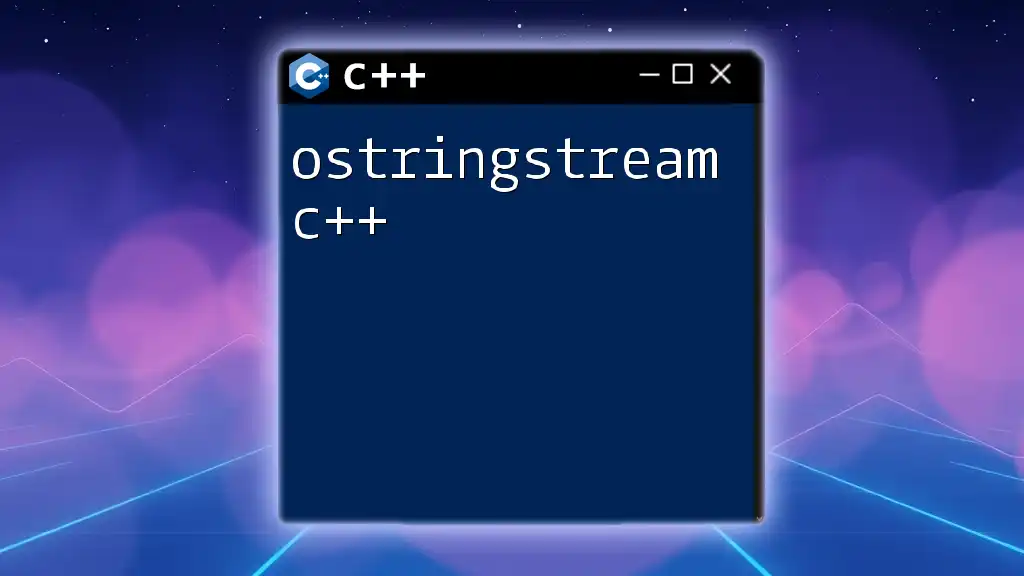
Fundamentals of String Parsing in C++
Using Standard Library Functions
The C++ Standard Library provides numerous functions that facilitate string manipulation, especially for parsing purposes. Key functions include:
- `std::getline`: Reads a line of text from an input stream into a string.
- `std::string::find`: Searches for a specific substring and returns its position.
- `std::string::substr`: Extracts a substring based on specified indices.
Example: Basic String Operations
Here’s a simple demonstration utilizing these functions:
#include <iostream>
#include <string>
int main() {
std::string sentence = "Hello, World!";
std::string word = sentence.substr(0, 5); // Extracting "Hello"
std::cout << word << std::endl; // Output: Hello
return 0;
}
The Role of Iterators in String Parsing
Iterators are essential when parsing strings as they allow traversal through the string effectively. Utilizing iterators, you can access each character sequentially without needing index manipulation.
Example: Using Iterators to Process Characters
The following example demonstrates traversing a string using iterators to display each character:
#include <iostream>
#include <string>
int main() {
std::string text = "C++ String Parsing";
for (auto it = text.begin(); it != text.end(); ++it) {
std::cout << *it << " "; // Output each character with a space
}
return 0;
}
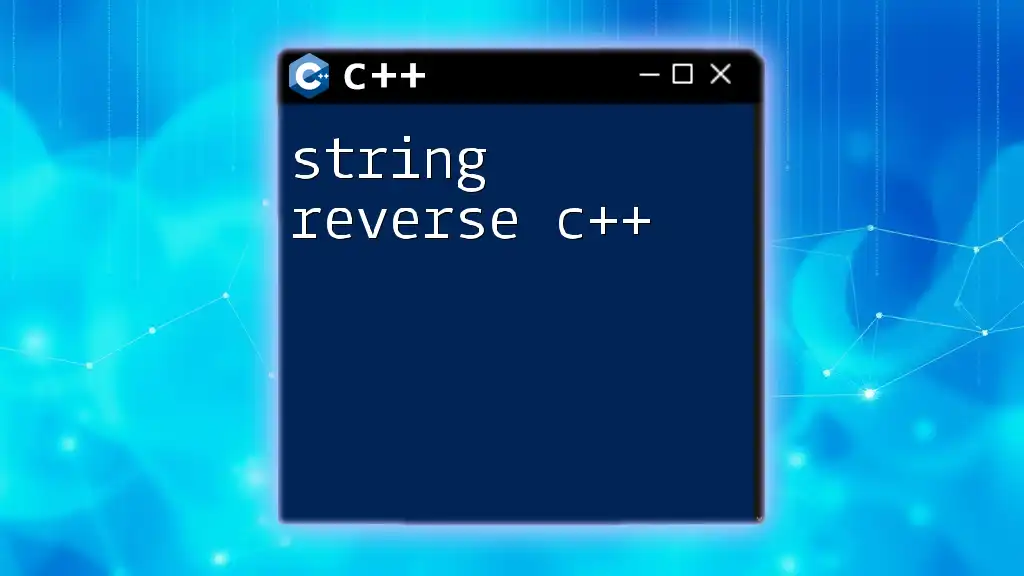
Advanced String Parsing Techniques
Tokenization
Tokenization divides a string into smaller pieces called tokens, which can be easier to process and analyze. The `std::istringstream` class is particularly useful for tokenization.
Example: Tokenizing a String
This example shows how to split a string based on commas:
#include <iostream>
#include <sstream>
#include <string>
int main() {
std::string data = "apple,banana,cherry";
std::istringstream stream(data);
std::string token;
while (std::getline(stream, token, ',')) { // Using comma as the delimiter
std::cout << token << std::endl; // Outputs each fruit on a new line
}
return 0;
}
Regular Expressions for String Parsing
Regular expressions (regex) provide a powerful way to search and match patterns within strings. The `<regex>` library simplifies tasks that would otherwise require more complex logic.
Example: Finding Matches with Regular Expressions
The following code snippet demonstrates how to use regex to find an email address pattern in a string:
#include <iostream>
#include <string>
#include <regex>
int main() {
std::string text = "Email me at example@test.com";
std::regex email_regex(R"((\w+)(\.\w+)*@(\w+)(\.\w+)+)"); // Regex for email
std::smatch matches;
if (std::regex_search(text, matches, email_regex)) {
std::cout << "Found email: " << matches[0] << std::endl; // Output: example@test.com
}
return 0;
}
Custom String Parsing Functions
Developing utility functions is invaluable for specific parsing needs. These functions encapsulate parsing logic, increasing modularity and readability in your code.
Example: Extracting Numeric Values from a String
Here's how to write a custom function that extracts numeric values from a string:
#include <iostream>
#include <string>
int extract_number(const std::string& str) {
std::string num_str;
for (char c : str) {
if (isdigit(c)) { // Check if the character is a digit
num_str += c; // Append digits to num_str
}
}
return num_str.empty() ? 0 : std::stoi(num_str); // Convert to integer or return 0
}
int main() {
std::string input = "The answer is 42.";
int number = extract_number(input);
std::cout << "Extracted Number: " << number << std::endl; // Output: 42
return 0;
}
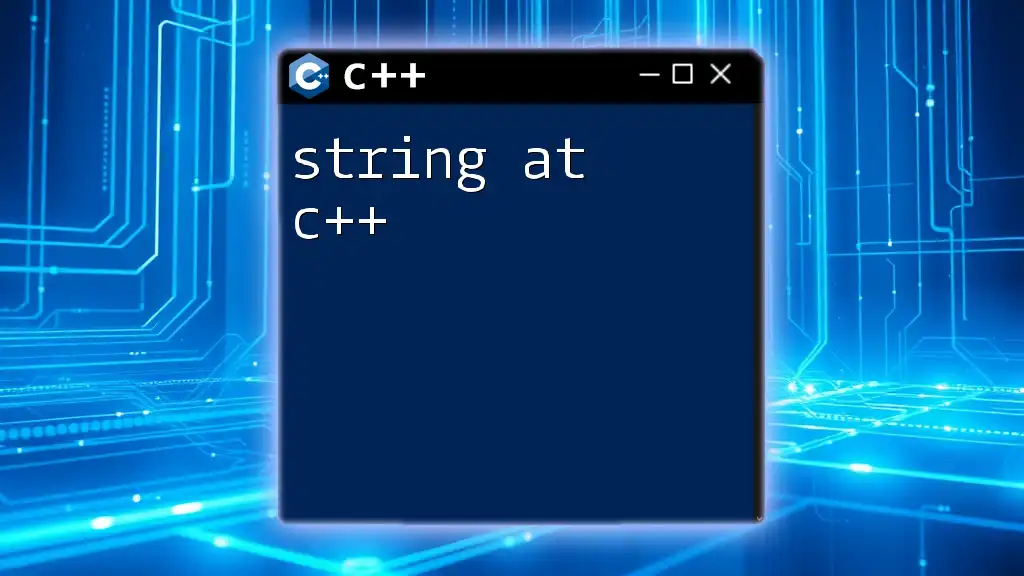
Best Practices for String Parsing in C++
Performance Considerations
When dealing with large strings, efficient handling is critical. Keep these practices in mind:
- Minimize allocations: Use `std::string` wisely to avoid unnecessary allocations and copies.
- Preallocate space: For large input data, consider reserving space in `std::string` using `std::string::reserve` to reduce overhead.
Error Handling During Parsing
String parsing can encounter multiple errors, such as unexpected formats or invalid input. Establish robust error handling strategies:
- Input validation: Always verify input formats before parsing.
- Use exceptions: Implement try-catch blocks to handle unforeseen parsing issues gracefully.
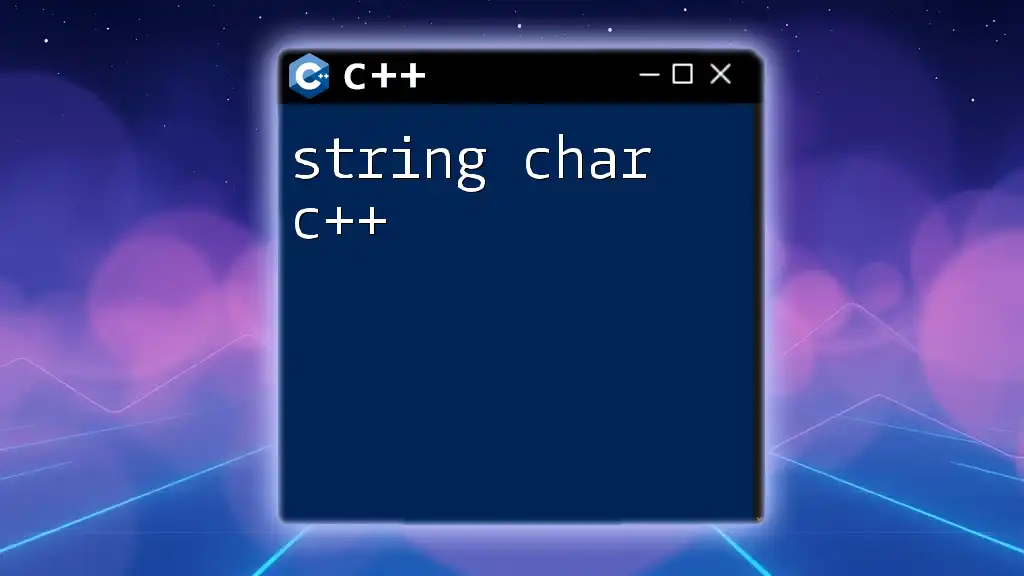
Conclusion
In summary, mastering the string parser c++ domain equips developers with the skills to handle text data efficiently. With an array of techniques—from utilizing standard library functions to implementing regex and custom functions—C++ string parsing can be powerful and flexible. As you engage with the rich capabilities of string parsing, practicing with real-world data will enhance your proficiency and confidence.
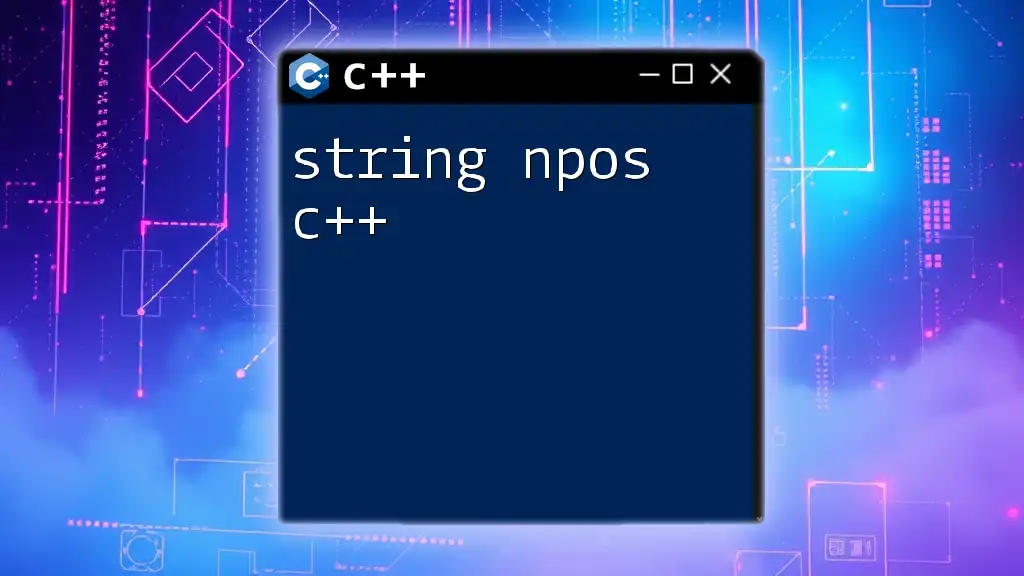
Additional Resources
To further enhance your understanding of string parsing in C++, consider exploring specialized books, online courses, and documentation. Engage in exercises that challenge your parsing skills and expand your expertise.