A ring buffer in C++ is a fixed-size data structure that uses a single, contiguous block of memory for efficient storage and retrieval of elements in a circular manner, allowing for constant time complexity on enqueue and dequeue operations.
Here's a simple implementation in C++:
#include <iostream>
class RingBuffer {
private:
int *buffer;
int head;
int tail;
int maxSize;
bool full;
public:
RingBuffer(int size) : head(0), tail(0), maxSize(size), full(false) {
buffer = new int[maxSize];
}
~RingBuffer() {
delete[] buffer;
}
void enqueue(int value) {
buffer[head] = value;
if(full) {
tail = (tail + 1) % maxSize; // Overwrite the oldest data
}
head = (head + 1) % maxSize;
full = head == tail;
}
int dequeue() {
if(isEmpty()) {
throw std::out_of_range("Buffer is empty");
}
int value = buffer[tail];
full = false;
tail = (tail + 1) % maxSize;
return value;
}
bool isEmpty() const {
return (!full && (head == tail));
}
bool isFull() const {
return full;
}
};
int main() {
RingBuffer ring(5);
ring.enqueue(1);
ring.enqueue(2);
ring.enqueue(3);
std::cout << ring.dequeue() << std::endl; // Outputs: 1
ring.enqueue(4);
ring.enqueue(5);
ring.enqueue(6); // Overwrites the oldest data
while(!ring.isEmpty()) {
std::cout << ring.dequeue() << " "; // Outputs: 2 3 4 5 6
}
}
What is a Ring Buffer?
A ring buffer, often referred to as a circular buffer, is a data structure that uses a fixed-size buffer in a way that it wraps around when the end is reached. It allows for efficient use of memory and provides a way to manage a continuously changing stream of data as it fills and empties.
Unlike traditional linear data structures where the data is added at the end and removed from the front, a ring buffer maintains a head (write position) and a tail (read position). When the buffer reaches its maximum capacity, it simply starts overwriting old data, making it suitable for scenarios where the latest data is more relevant than the old.

Advantages of Using a Ring Buffer
Using a ring buffer offers several advantages:
Memory Management: Since the size of a ring buffer is fixed, it provides predictable memory usage. Unlike dynamic arrays, it eliminates the overhead of resizing and minimizes the risk of memory fragmentation.
Performance: Ring buffers allow for fast read and write operations, making them ideal for applications that require high throughput, such as real-time data processing or audio streaming.
Use Cases: They are particularly useful in real-time systems, where data is produced and consumed at a high rate, such as in audio processing, video streaming, and inter-thread communication between producers and consumers.
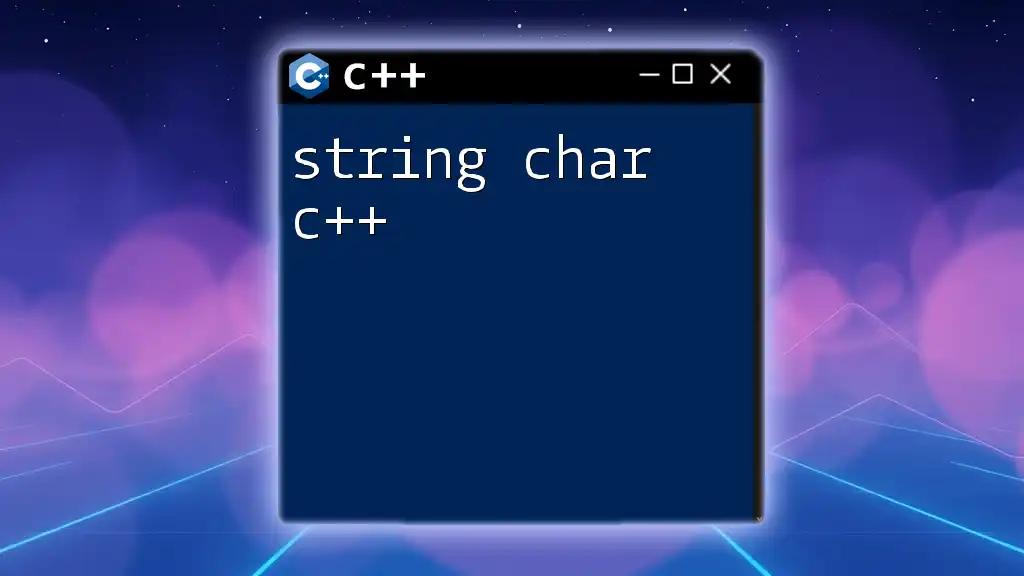
How a Ring Buffer Works
The structure of a ring buffer includes essential components:
- Size of the buffer: This indicates how many elements the buffer can hold.
- Head pointer: Points to the position where the next item will be written.
- Tail pointer: Points to the position where the next item will be read.
- Array to hold the buffer elements: This is the storage for the data.
When data is added, the head moves forward. When data is read, the tail moves forward. If the head moves past the end of the buffer array, it wraps around to the start, hence the name "circular."

Implementing a Ring Buffer in C++
Basic Structure
To create a ring buffer in C++, define a class that holds the necessary member variables.
template <typename T>
class RingBuffer {
public:
RingBuffer(size_t size);
~RingBuffer();
private:
T* buffer;
size_t head;
size_t tail;
size_t maxSize;
};
Constructor and Destructor
The constructor initializes the buffer by allocating memory and setting up the head and tail pointers.
template <typename T>
RingBuffer<T>::RingBuffer(size_t size) : maxSize(size), head(0), tail(0) {
buffer = new T[maxSize];
}
template <typename T>
RingBuffer<T>::~RingBuffer() {
delete[] buffer;
}
The destructor cleans up the allocated memory to prevent memory leaks.
Enqueue Operation
The enqueue function allows adding data to the ring buffer. If the buffer is full, an exception is thrown.
template <typename T>
void RingBuffer<T>::enqueue(const T& item) {
if ((head + 1) % maxSize == tail) {
throw std::runtime_error("Buffer is full");
}
buffer[head] = item;
head = (head + 1) % maxSize;
}
Dequeue Operation
The dequeue function removes and returns data from the buffer. If the buffer is empty, it throws an exception.
template <typename T>
T RingBuffer<T>::dequeue() {
if (head == tail) {
throw std::runtime_error("Buffer is empty");
}
T item = buffer[tail];
tail = (tail + 1) % maxSize;
return item;
}
Peek Operation
To preview the next item in the buffer without removing it, the peek function can be implemented.
template <typename T>
T RingBuffer<T>::peek() const {
if (head == tail) {
throw std::runtime_error("Buffer is empty");
}
return buffer[tail];
}

Best Practices for Using Ring Buffers in C++
When implementing a ring buffer, it’s essential to consider error handling. Always ensure adequate checks for underflow (when attempting to dequeue from an empty buffer) and overflow (when attempting to enqueue into a full buffer).
In a multi-threaded environment, ensure that operations on the buffer are synchronized, preventing race conditions that could lead to data corruption. Consider using mutexes or locks to manage access between threads.
For performance optimization, choose the buffer size wisely. A buffer that is too small can lead to frequent overwriting of data, while a buffer that is too large may waste memory. Profiling can help determine the optimal size for specific applications.
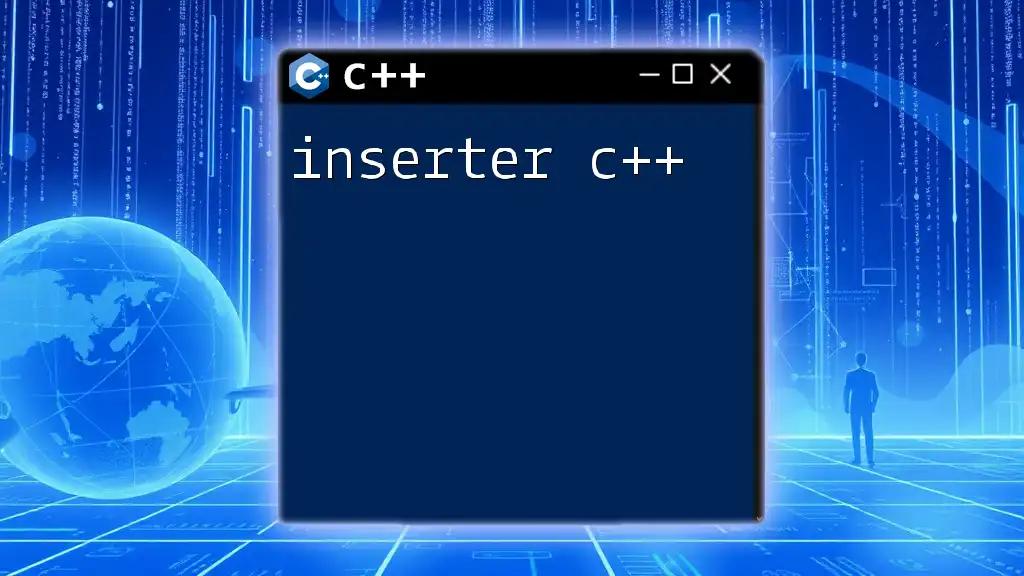
Real-world Applications of Ring Buffers
Ring buffers find extensive usage in audio processing, allowing smooth streaming of audio data while continuously buffering incoming data. They are also employed in network data packet buffering, where packets are queued and processed in a timely manner.
In real-time data processing systems, ring buffers efficiently manage streams of data collected from sensors, ensuring timely processing and minimal data loss.
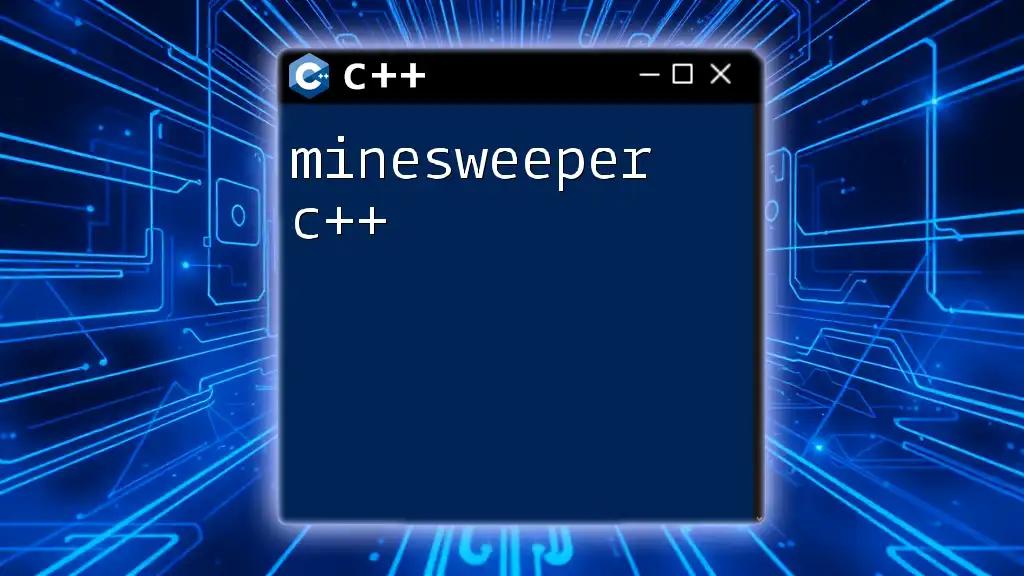
Conclusion
In conclusion, understanding and implementing a ring buffer in C++ is crucial for various applications that require efficient data handling. By utilizing a ring buffer's unique properties, programmers can enhance performance and memory management in their applications. Engage in practical coding of ring buffers to solidify your understanding and improve your C++ proficiency.
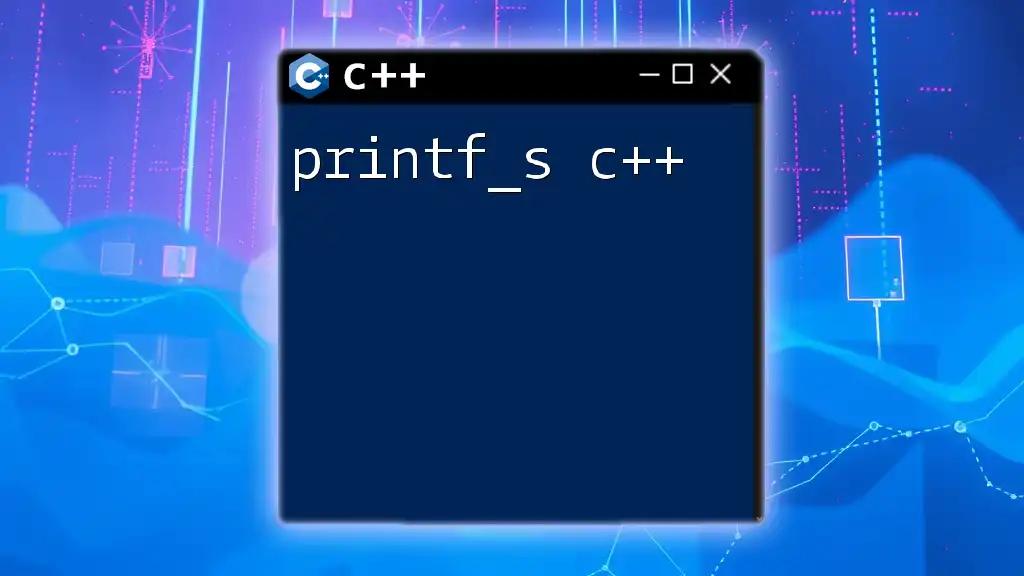
Additional Resources
For further exploration, consider consulting relevant documentation and resources that deepen your knowledge of C++ and data structures. Reading suggests books and articles can significantly enhance your understanding and skills in software development.