Minesweeper in C++ is a classic game where players uncover squares on a grid while avoiding hidden mines, and here's a simple code snippet demonstrating how to set up the game board:
#include <iostream>
#include <vector>
#include <cstdlib>
#include <ctime>
void initializeBoard(std::vector<std::vector<int>>& board, int rows, int cols, int mines) {
// Place mines randomly on the board
for (int i = 0; i < mines; ++i) {
int row = rand() % rows;
int col = rand() % cols;
board[row][col] = -1; // -1 indicates a mine
}
}
int main() {
srand(time(0)); // Seed for random number generation
const int rows = 5, cols = 5, mines = 5;
std::vector<std::vector<int>> board(rows, std::vector<int>(cols, 0));
initializeBoard(board, rows, cols, mines);
// Print the board (for demonstration)
for (const auto& row : board) {
for (int cell : row) {
std::cout << (cell == -1 ? '*' : '.') << " "; // '*' for mines, '.' for empty
}
std::cout << std::endl;
}
return 0;
}
Setting Up Your C++ Environment
Before diving into coding Minesweeper in C++, you'll need to set up your development environment. Choosing a reliable C++ compiler is crucial, as it can significantly affect your coding experience.
Choosing a C++ Compiler
Popular Integrated Development Environments (IDEs) include Visual Studio, Code::Blocks, and CLion. Each of these IDEs offers unique features that cater to different preferences:
- Visual Studio: Rich in features and extensions, ideal for Windows users but available for Mac.
- Code::Blocks: Lightweight and portable, perfect for beginners seeking an easy start.
- CLion: A modern IDE with extensive support for C++ along with tools for refactoring and debugging.
Essential Libraries for Game Development
Using external libraries can enhance your Minesweeper game. Consider exploring libraries like SFML (Simple and Fast Multimedia Library) or SDL (Simple DirectMedia Layer). These libraries assist with graphics, sound, and user input—essential elements for any game development project. They provide a windowing environment and handle input and output efficiently, allowing you to focus more on game mechanics than on setup.
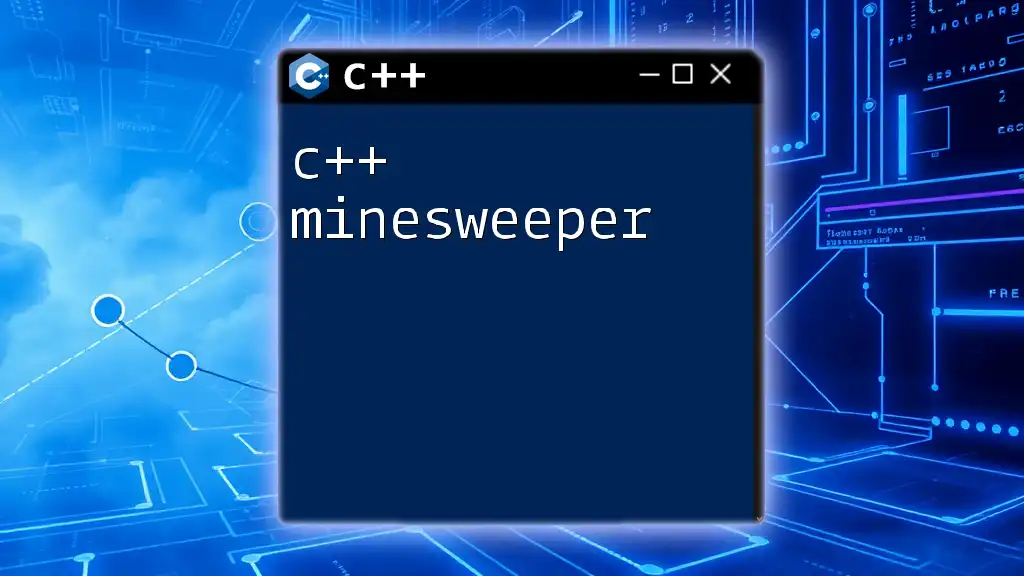
Game Logic Structure
Every game has its logic. Understanding the basics of how Minesweeper operates will help you implement it effectively in C++.
Understanding Game Mechanics
Grid Setup
Minesweeper is typically played on a grid of tiles. In C++, this can be represented using a 2D array or a vector. A standard grid might consist of predefined widths and heights, which you'll define at the start:
const int WIDTH = 10;
const int HEIGHT = 10;
int grid[WIDTH][HEIGHT];
This `grid` will hold various values: `0` for empty cells, `-1` for mines, and numbers indicating adjacent mines.
Placing Mines Randomly
One of the core functionalities is the random placement of mines across the grid. To achieve this, you can employ the random number generation capabilities of C++:
srand(time(0)); // Seed for random number generation
for (int i = 0; i < numberOfMines; i++) {
int x = rand() % WIDTH;
int y = rand() % HEIGHT;
grid[x][y] = -1; // Indicate a mine
}
This snippet uses the current time as a seed to ensure unique placements on each run.
Calculating Neighboring Mines
For every cell in the grid, you must determine how many mines are in adjacent cells. This step is crucial for providing players with hints about where the mines might be located:
void calculateNeighbors(int grid[WIDTH][HEIGHT]) {
for (int x = 0; x < WIDTH; x++) {
for (int y = 0; y < HEIGHT; y++) {
if (grid[x][y] != -1) { // If not a mine
// Logic to count adjacent mines
int count = 0;
for (int i = -1; i <= 1; i++) {
for (int j = -1; j <= 1; j++) {
if (x + i >= 0 && x + i < WIDTH && y + j >= 0 && y + j < HEIGHT && grid[x + i][y + j] == -1) {
count++;
}
}
}
grid[x][y] = count; // Store the mine count
}
}
}
}
This function iterates over the grid, counting mines surrounding each cell that isn’t a mine itself.
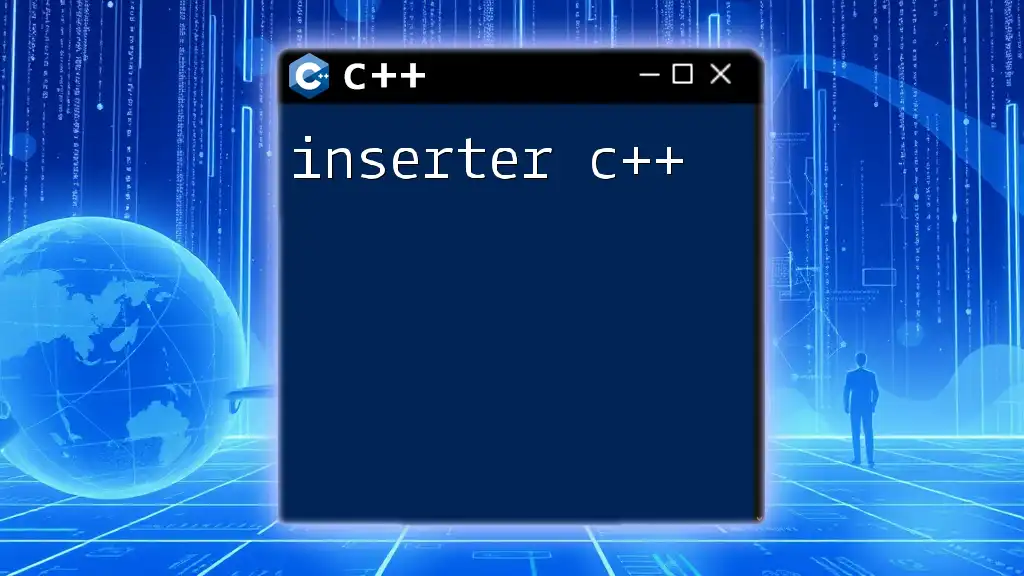
User Interface Design
A good user interface (UI) is imperative in ensuring an enjoyable gameplay experience. You have options for text-based or graphical interfaces.
Text-based vs. Graphical Interface
Text-based interfaces are simpler to implement, making them ideal for beginners. On the other hand, graphical interfaces enrich the experience substantially, though they require more advanced programming skills.
Creating a Simple Text-based Interface
For beginners, implementing a text-based interface allows for a rapid setup. You can render the grid directly in the console using simple print statements:
void printGrid(int grid[WIDTH][HEIGHT]) {
for (int y = 0; y < HEIGHT; y++) {
for (int x = 0; x < WIDTH; x++) {
// Logic to print based on cell value
if (grid[x][y] == -1) {
std::cout << "* "; // Mine representation
} else {
std::cout << grid[x][y] << " "; // Number of adjacent mines
}
}
std::cout << std::endl;
}
}
This function outputs the current state of the grid to the console, allowing the player to see where they have revealed tiles.
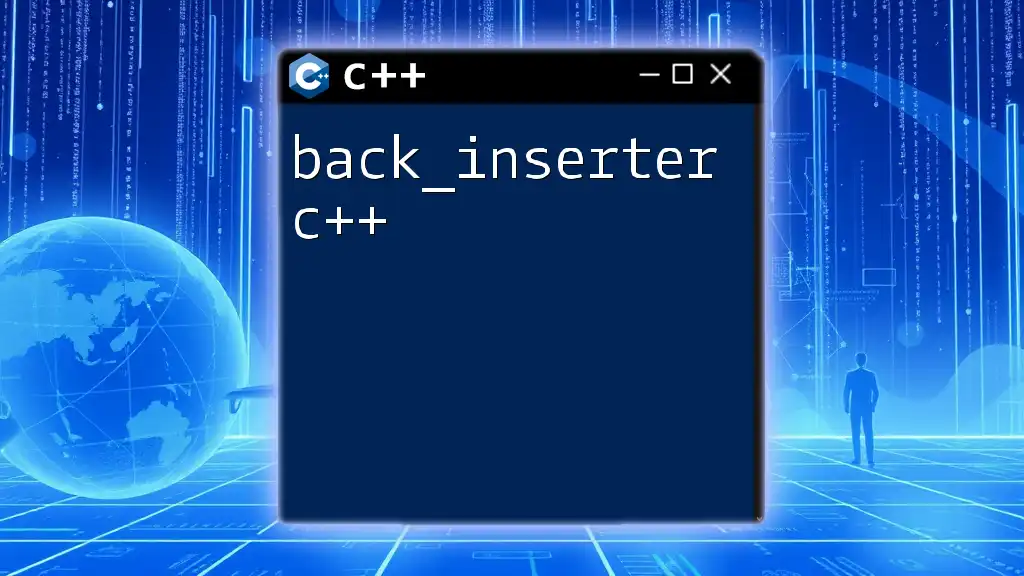
Game Interactions
Interactivity is a cornerstone of any game, especially in a strategic game like Minesweeper. Implementing user input and game mechanics requires thoughtful design.
Handling User Input
Capturing user input effectively allows players to interact with the game. In C++, you might use basic console input to manage player actions:
void getUserInput(int &x, int &y) {
std::cout << "Enter your move (x y): ";
std::cin >> x >> y;
}
By prompting the user for coordinates, you can determine which cell they wish to reveal.
Revealing Cells
After receiving input, you need to reveal the selected cell and potentially trigger a cascading reveal if the cell is empty. This creates additional gameplay depth:
void revealCell(int x, int y) {
if (grid[x][y] == -1) {
std::cout << "Game Over! You hit a mine." << std::endl;
} else {
std::cout << "You revealed a cell with " << grid[x][y] << " neighboring mines." << std::endl;
// Logic for empty cells could go here
}
}
This snippet checks whether a mine was revealed. If so, it outputs a losing message; otherwise, it reveals the adjacent mine count.
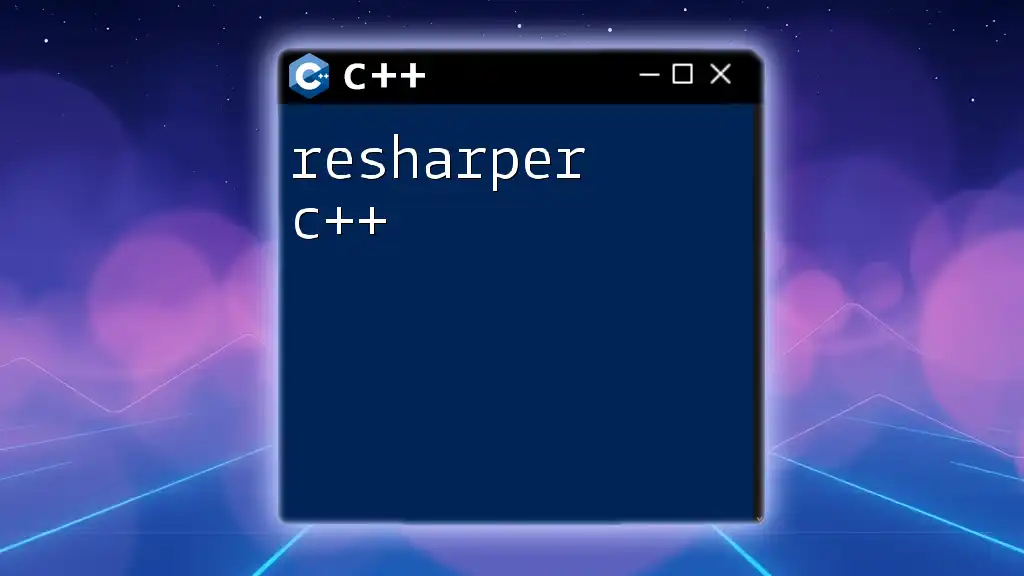
Enhancing the Game Experience
To improve player interaction, consider adding features which make the game more enjoyable.
Implementing Flags
Allow players to flag suspected mines. This feature adds a layer of strategy, as users can mark tiles they believe contain mines without revealing them:
void flagCell(int x, int y) {
// Logic to toggle flags
if (grid[x][y] != -1) {
// Update flag status in your grid representation
}
}
This function allows the player to mark a cell, which enhances strategic gameplay.
Game Status Indicators
Make it clear to users when they’ve won or lost. Clear notifications enhance user experience and closure regarding game outcomes:
if (userRevealedAllSafeCells()) {
std::cout << "You win!" << std::endl;
} else if (userHitMine()) {
std::cout << "Game Over!" << std::endl;
}
This conditional logic informs the player of the game's outcome based on their actions.
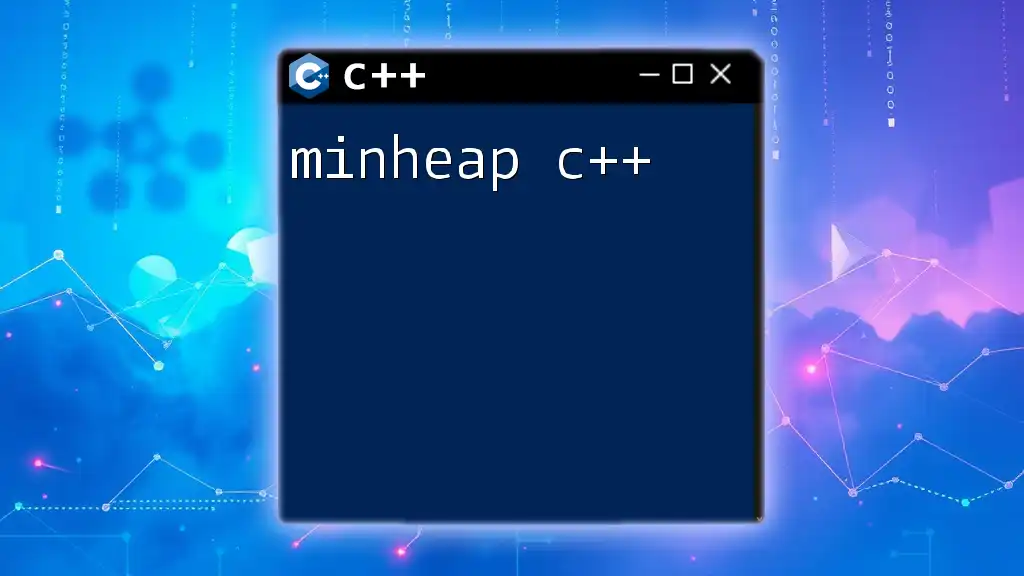
Conclusion
Implementing Minesweeper in C++ provides a fantastic opportunity to learn vital programming concepts, such as grid manipulation, user input handling, and game logic structuring. As you explore this project, you'll not only enhance your coding skills but also gain valuable insights into game development processes.
Encouraging Further Exploration
Once you've mastered this foundational version of Minesweeper, consider diving deeper into advanced topics. Implementing graphical interfaces or adding features like time tracking and scoreboards can broaden your project and refine your expertise.
Call-to-Action
Share your own implementations of Minesweeper or any challenges you've faced during development. Engage with your peers and let the community help as you embark on your C++ learning journey!
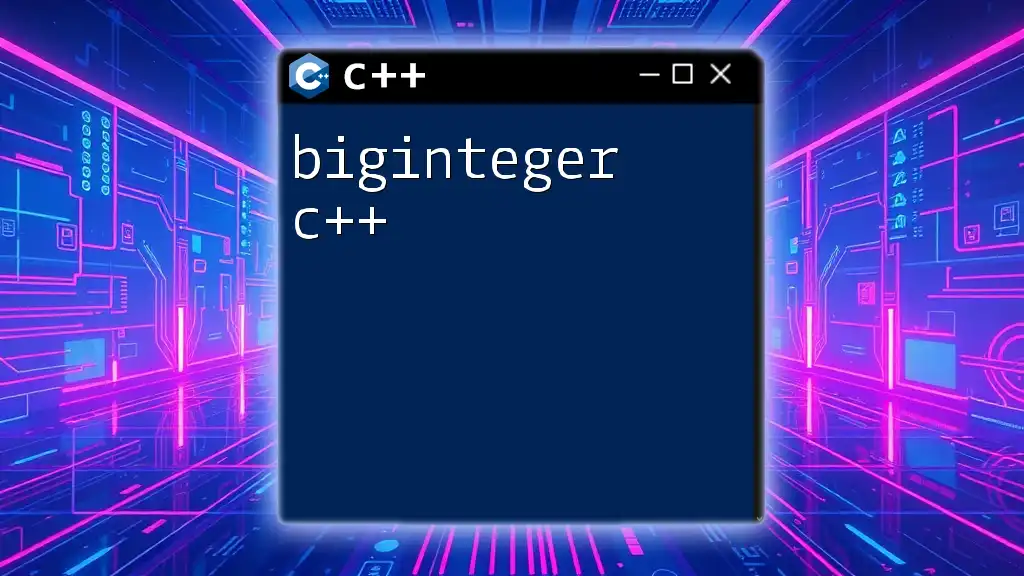
Additional Resources
For further study, check out tutorials and online forums that focus on game development in C++. Engaging with the community is a great way to deepen your understanding and troubleshoot challenges. Happy coding!