C++ Minesweeper is a simple console-based game that challenges players to clear a grid of hidden mines by using numbers to indicate the count of adjacent mines, implemented with basic C++ commands for game logic and user interaction.
Here's a basic code snippet to illustrate the game's main functionality:
#include <iostream>
#include <vector>
#include <ctime>
#include <cstdlib>
class Minesweeper {
public:
Minesweeper(int width, int height, int mines);
void displayBoard();
void revealCell(int x, int y);
private:
std::vector<std::vector<int>> board;
int width, height, mines;
void placeMines();
};
Minesweeper::Minesweeper(int w, int h, int m) : width(w), height(h), mines(m) {
board.resize(height, std::vector<int>(width, 0));
placeMines();
}
void Minesweeper::placeMines() {
srand(time(0));
for (int i = 0; i < mines; ++i) {
int x = rand() % width;
int y = rand() % height;
board[y][x] = -1; // Place mine
}
}
void Minesweeper::displayBoard() {
for (const auto &row : board) {
for (int cell : row) {
std::cout << (cell == -1 ? '*' : '0') << " "; // Display the mine or 0
}
std::cout << std::endl;
}
}
This code snippet sets up a basic structure for a Minesweeper game in C++, allowing mine placement and board display.
Setting Up Your Development Environment
Choosing Your IDE
When embarking on your journey to create a C++ Minesweeper game, selecting the right Integrated Development Environment (IDE) is crucial for your productivity and comfort. Popular IDEs among C++ developers include Visual Studio, Code::Blocks, and CLion. Each of these environments offers useful features like syntax highlighting, debugging tools, and project management capabilities. Choose one that aligns with your preferences and development style.
Installing Necessary Libraries
To enhance the visual appeal and user experience of your game, you might want to leverage libraries like SFML (Simple and Fast Multimedia Library) or SDL (Simple DirectMedia Layer). These libraries simplify graphics rendering and input handling. Follow the documentation provided by these libraries to install and configure them correctly within your IDE.
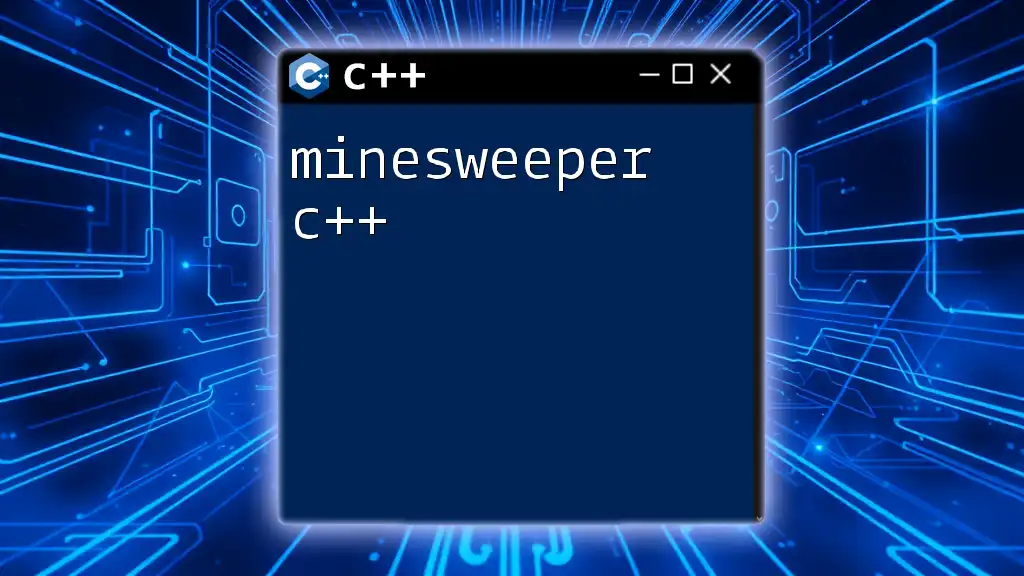
Game Fundamentals
Understanding the Game Mechanics
Before diving into the coding aspect, it is essential to grasp the core mechanics of Minesweeper. The objective of Minesweeper is straightforward: clear the board without detonating any hidden mines. The board consists of a grid of tiles that reveal either a number (indicating the count of mines in adjacent tiles) or a mine itself when clicked. The game ends when you click on a mine, resulting in a loss.
Defining Game Components
The game can be broken down into three fundamental components:
- Grid: The playing field represented as a 2D array, where each cell can be a mine or an empty space.
- Cells: Each individual square in the grid holds significant information, either revealing a mine or displaying a number indicating how many of the surrounding cells contain mines.
- Flags: Used to indicate a suspected mine. Players can flag cells, and these flags help in strategizing and navigating the game.
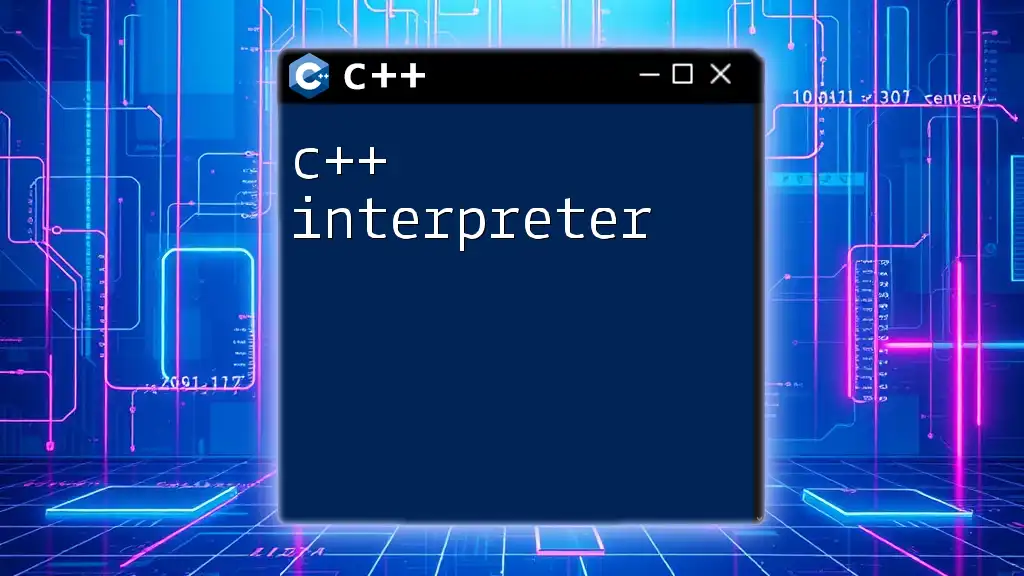
Designing the Game Structure
Creating a Class for the Game
Utilizing Object-Oriented Programming (OOP) principles will allow for a clean design and maintainable code. You can start with a simple `Minesweeper` class that encapsulates the game logic and state.
class Minesweeper {
private:
int width;
int height;
int mineCount;
std::vector<std::vector<Cell>> grid;
public:
Minesweeper(int w, int h, int mines);
void initialize();
void print();
// Additional methods...
};
In this basic structure, `width`, `height`, and `mineCount` define the dimensions of the game board and the total number of mines, respectively. The `grid` is a 2D vector that represents the current state of the game.
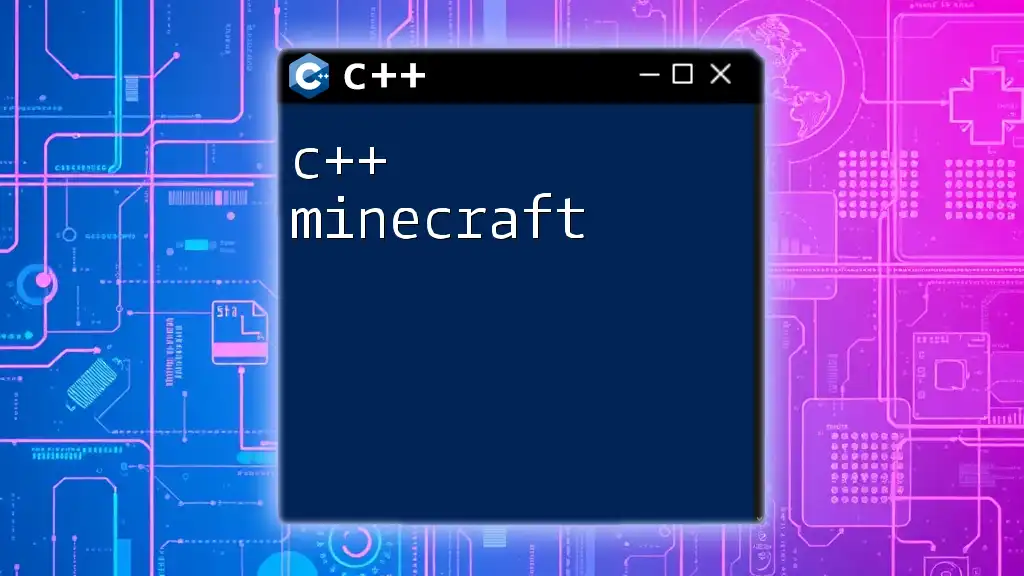
Implementing Key Features
Generating the Minefield
Creating a random minefield is a pivotal step. C++ provides the `<cstdlib>` library for random number generation. You can develop a function to initialize the game grid and randomly place mines.
void Minesweeper::initialize() {
std::srand(std::time(0)); // Seed for randomness
// Logic to place mines in the grid
}
The mine placement logic typically involves picking random indices within the grid dimensions and inserting mines until the desired count is achieved.
Calculating Cell Values
Once the mines are set, the next task is to compute how many mines are adjacent to each cell, preparing the grid for user interaction.
void Minesweeper::calculateCellValues() {
for (int x = 0; x < width; x++) {
for (int y = 0; y < height; y++) {
if (grid[x][y].isMine) {
continue;
}
grid[x][y].adjacentMines = countAdjacentMines(x, y);
}
}
}
int Minesweeper::countAdjacentMines(int x, int y) {
int count = 0;
// Logic to count mines adjacent to (x, y)
return count;
}
In this snippet, the method iteratively checks each cell and increments a counter based on neighboring mines.
Revealing Cells
A crucial part of gameplay is revealing cells. When a player clicks on a cell, the logic ensures they unveil either the number of adjacent mines or a mine itself. Implementing the reveal logic can look like this:
void Minesweeper::revealCell(int x, int y) {
if (grid[x][y].isRevealed || grid[x][y].isFlagged) {
return; // Ignore if already revealed or flagged
}
grid[x][y].isRevealed = true;
if (grid[x][y].isMine) {
// Player has hit a mine
gameOver = true;
} else if (grid[x][y].adjacentMines == 0) {
// Recursively reveal adjacent cells
revealAdjacentCells(x, y);
}
}
Here, the function checks the state of the cell being revealed and proceeds accordingly.
Flagging Cells
Implementing a flagging mechanism allows players to mark suspected mines strategically. The flagging action can be toggled based on user input.
void Minesweeper::toggleFlag(int x, int y) {
if (!grid[x][y].isRevealed) {
grid[x][y].isFlagged = !grid[x][y].isFlagged;
}
}
This method helps track potential mines without revealing adjacent cells.
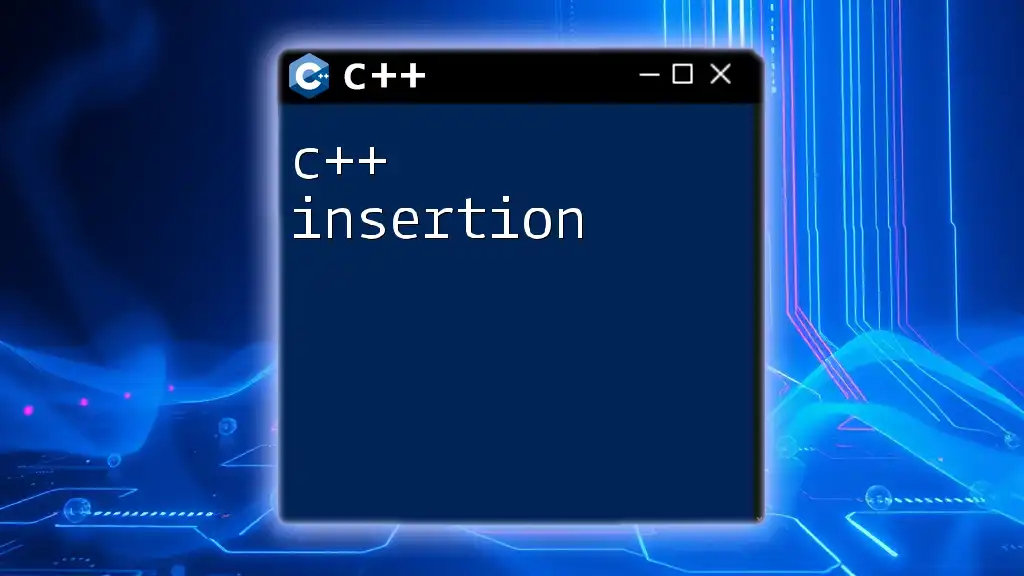
User Interface
Text-Based vs Graphical UI
For a C++ Minesweeper game, you can choose to create either a text-based interface or a graphical one. If you prefer simplicity and speed, a console application will suffice. However, for a more engaging experience, transitioning to a graphical UI using SFML or SDL could enhance gameplay.
Building the Console Interface
To build a text-based UI, you will need functions for basic input and output operations. You can implement a `print` function that displays the game grid to the console.
void Minesweeper::print() {
for (int y = 0; y < height; y++) {
for (int x = 0; x < width; x++) {
if (grid[x][y].isRevealed) {
std::cout << (grid[x][y].isMine ? '*' : grid[x][y].adjacentMines) << " ";
} else {
std::cout << (grid[x][y].isFlagged ? 'F' : '#') << " ";
}
}
std::cout << std::endl;
}
}
This method will display the grid's current state, showing revealed cells, flagged cells, and unrevealed tiles.
Creating a Graphical Interface
If you decide to create a graphical interface, you would first need to set up your chosen graphics library. Use the library's functions to create a window, set up event handling, and draw your grid based on the underlying game state.
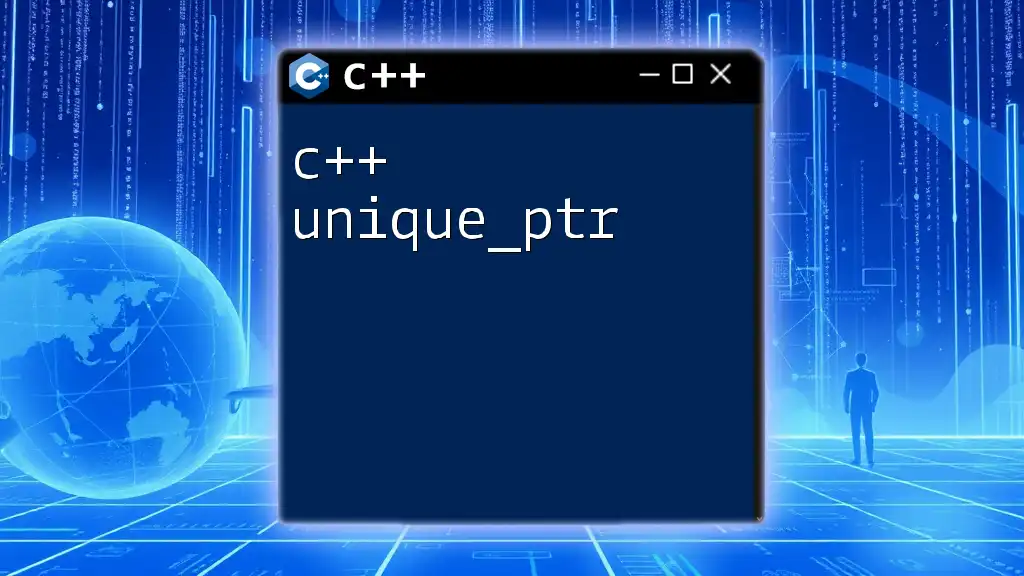
Game Logic and Control Flow
Game Loop Structure
The game's main control flow involves a loop that perpetually checks for user input and updates the game state. This loop manages the core gameplay experience, allowing players to reveal cells, flag mines, and end the game.
void runGame() {
Minesweeper game(10, 10, 15);
game.initialize();
while (!gameOver) {
game.print();
// Capture user input and call appropriate methods
}
}
In this structure, the `runGame` function initializes an instance of the `Minesweeper` class and continuously loops until the game ends.
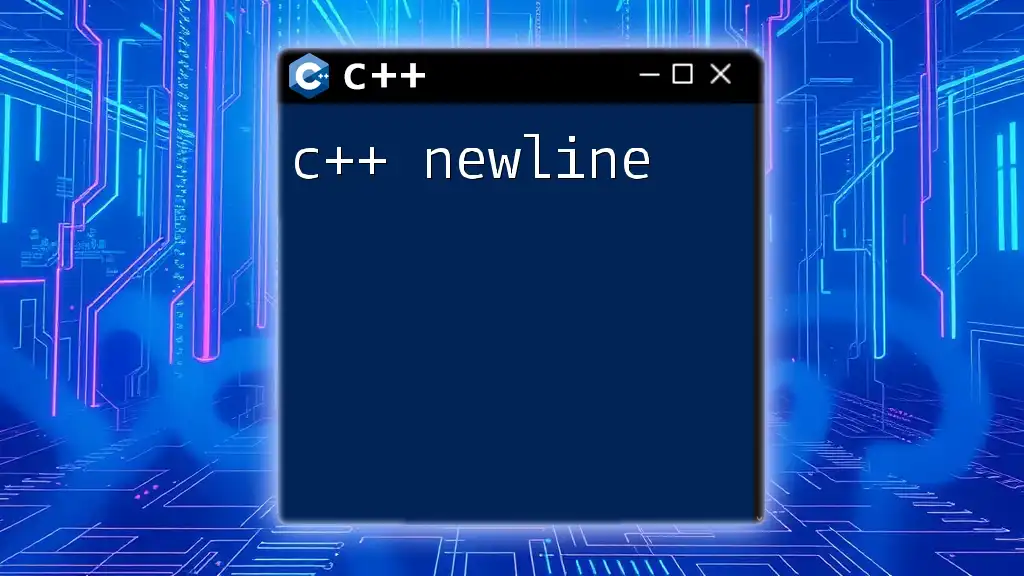
Testing and Debugging
Common Bugs in Minesweeper
While developing your C++ Minesweeper game, you may encounter several common issues, including:
- Incorrect mine placement or counting.
- Recursion errors during cell revealing.
- User input not being handled as expected.
Identifying these bugs early helps ensure a smoother user experience.
Strategies for Testing the Game
Implementing unit tests for your game logic is advisable. Use frameworks like Google Test to create automated tests for methods like mine placement and cell revealing to confirm they function as intended.
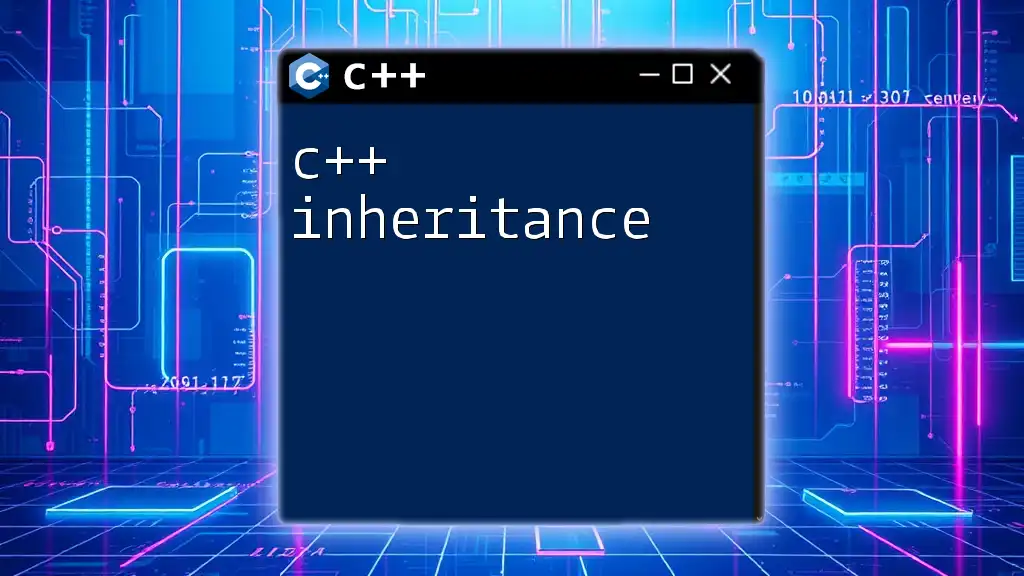
Conclusion
Completing a C++ Minesweeper game is a rewarding experience that consolidates critical programming concepts like OOP, algorithms, and data structures. You’ve learned how to create the game grid, implement logic for revealing cells, and manage user interaction effectively.
Next Steps
To enhance your Minesweeper game, consider adding features such as:
- Difficulty levels with varying grid sizes and mine counts.
- A timer to record the player's completion time.
- A high score system to track the best scores across various sessions.
Continuing to expand your project will deepen your understanding and mastery of C++. As you move forward, utilize available online resources, books, and community forums to grow your skills in game development.