C++ can be utilized to create Minecraft mods or plugins that enhance gameplay or add new features, allowing developers to customize their gaming experience.
Here's a simple example of a C++ script that prints "Hello, Minecraft!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, Minecraft!" << std::endl;
return 0;
}
Introduction to C++ and Minecraft
C++ is a powerful programming language renowned for its performance and control over system resources. Minecraft, on the other hand, is a sandbox game that gives players incredible freedom to create and modify their environments. Pairing C++ to enhance Minecraft can result in optimized mods that yield impressive results, allowing developers to utilize low-level programming features to push their creativity.
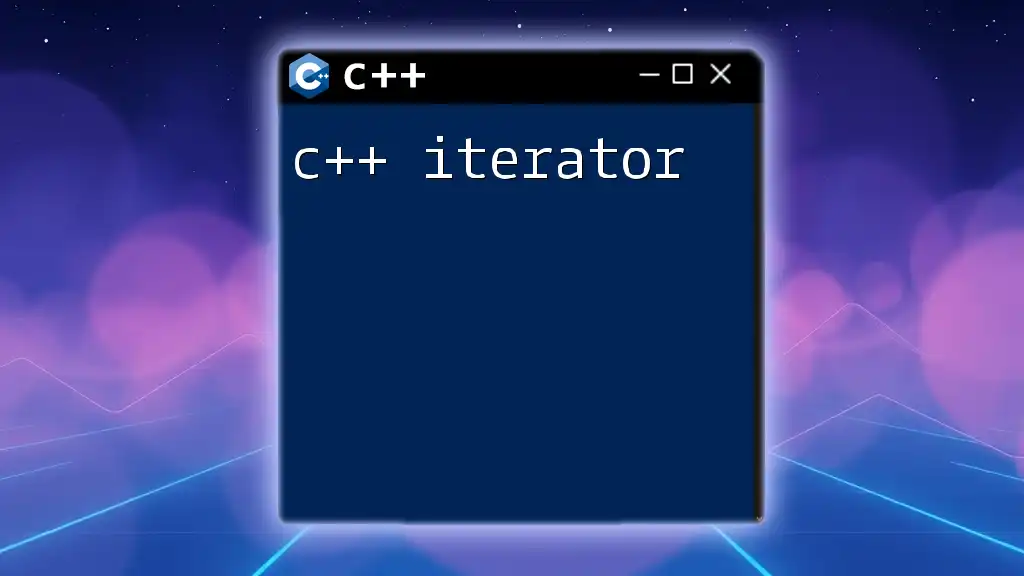
Understanding Minecraft's Modding Capabilities
What is Modding?
Modding refers to the process of altering or extending a game by using custom code. In Minecraft, modding has become a cornerstone of the community, allowing players to introduce new mechanics, visuals, and experiences, thereby enhancing gameplay significantly.
Popular Minecraft Modding Languages
While Java dominates the Minecraft modding landscape due to the game’s architecture, other languages, including C++, can be leveraged for specific needs or to improve performance. Many developers find C++ appealing for its efficiency, especially when they aim to create complex systems or heavy computations.
C++ vs Java in Minecraft Modding
C++ offers certain advantages over Java, especially in terms of performance and memory management. This can be particularly important when developing mods that require fast computations or that manipulate large datasets. However, working with C++ in Minecraft can also present challenges—such as additional complexity when interfacing with the game's Java-based architecture.
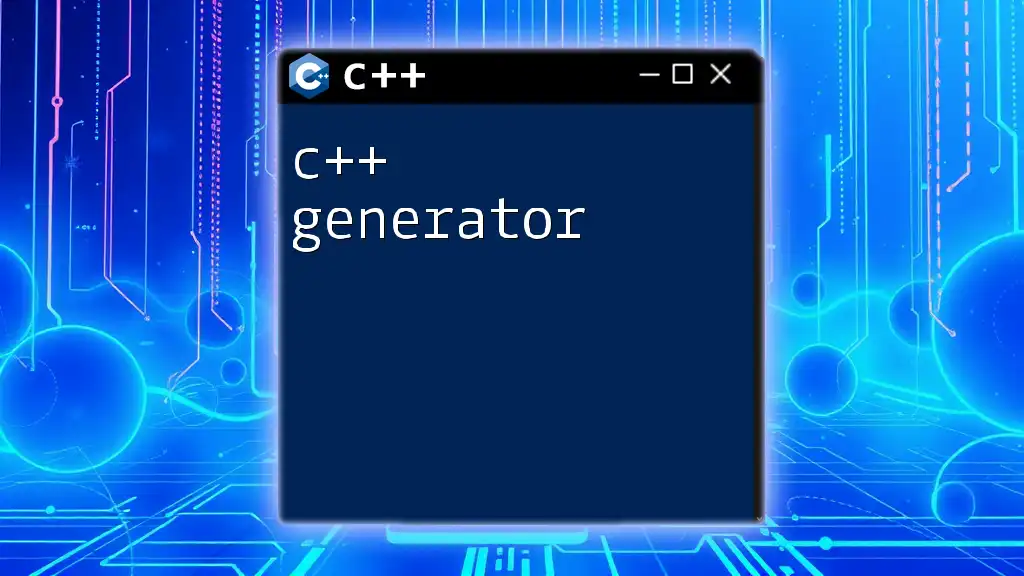
Setting Up the Development Environment
Requirements for C++ Development
To dive into C++ modding for Minecraft, you'll need:
- A C++ compiler (e.g., GCC or Clang)
- A suitable IDE (e.g., Visual Studio, Code::Blocks)
- Essential libraries (e.g., SDL, OpenGL) for rendering and graphics
Installing Minecraft C++ Modding Framework
There are several frameworks like Cocos2d-x or OGRE designed to facilitate C++ development in game settings. A simple guide for installation could include:
- Visiting the official website of the framework.
- Downloading the installer or cloning from GitHub.
- Following the documentation to integrate it with Minecraft.
Creating Your First C++ Minecraft Mod
Start with a simple "Hello World" mod to familiarize yourself with the modding process. Structure your mod's files correctly and ensure your IDE is set up to compile C++ code.
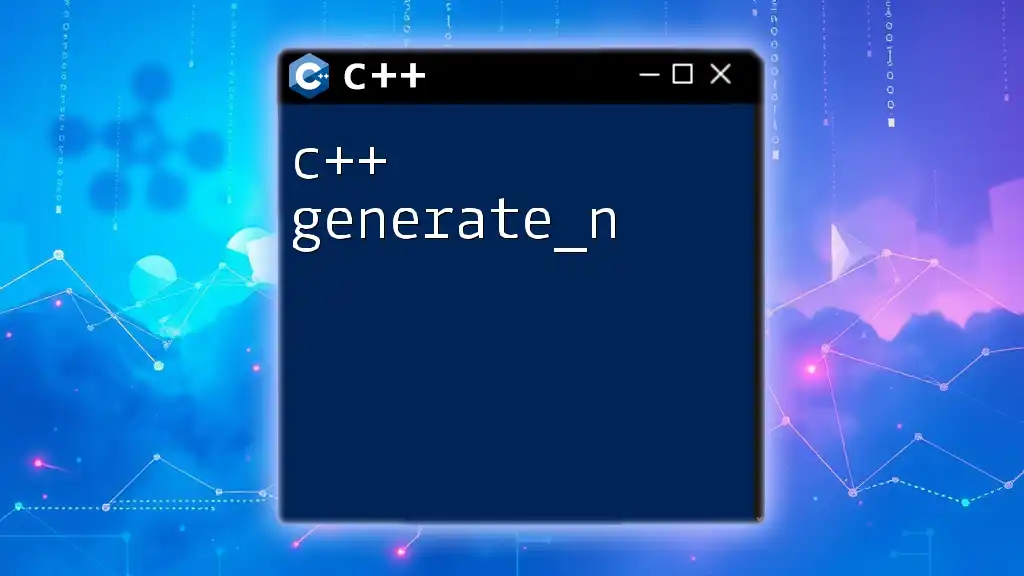
Core Concepts of C++ in Minecraft
Basic C++ Syntax for Minecraft Mod Development
Understanding C++'s syntax is crucial. Here’s a quick overview:
- Variables: Define a variable with a type, e.g., `int score = 0;`
- Control Structures: Use if-else statements, loops, and function definitions to control flow.
Understanding the Minecraft API
The Minecraft API provides functions and classes essential for interacting with the game world. Explore the documentation provided with the Minecraft modding tools to understand how to utilize its elements effectively.
Data Structures
Arrays & Vectors
Data structures are crucial for organizing and manipulating data. Arrays and vectors allow you to store collections of items:
#include <vector>
std::vector<int> playerScores;
void addScore(int score) {
playerScores.push_back(score);
}
This snippet demonstrates how to dynamically store player scores using a vector.
Classes and Objects
Utilizing classes in C++ fosters code reuse and organization. Define your own block, for instance:
class CustomBlock {
public:
CustomBlock() {
// Block attributes can be set here
}
void onBlockInteract() {
// Define interactions
}
};
By creating classes, you standardize your components and make it easier to manage interactions within Minecraft.
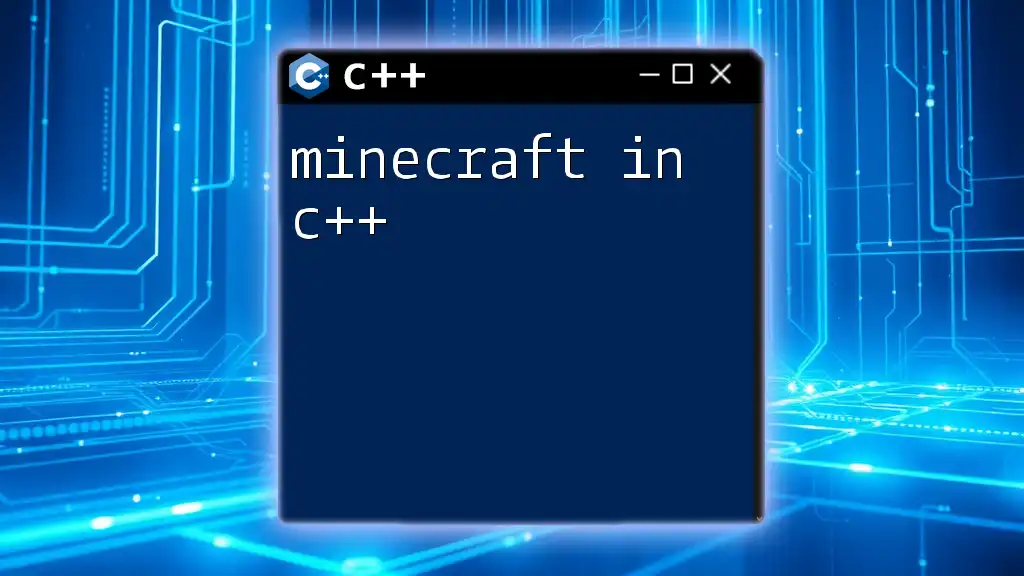
Coding Your First Minecraft Mod
Planning Your Mod
Before coding, plan your mod by sketching out ideas, functionalities, and what you want to achieve. This roadmap will guide your writing and help foresee potential issues.
Setting Up the Project Structure
A logical project structure is vital.
- Create folders for source files, assets, and configurations.
- Ensure your IDE can easily recognize these directories for compilation.
Writing Your Mod Code
Code Example: Basic Block Creation
Here’s how to define a simple custom block:
class CustomBlock : public Block {
public:
CustomBlock() : Block("CustomBlock", "textures/custom_block.png") {
// Constructor logic
}
void onBlockBreak() {
// Define behavior on block break
}
};
This code snippet demonstrates the creation of a custom block, allowing you to add unique textures and behaviors.
Compiling your Code
Once you’ve written your code, compiling it is essential:
- Make sure your project settings are correct in your IDE.
- Execute the compiler, and check for any errors or warnings.
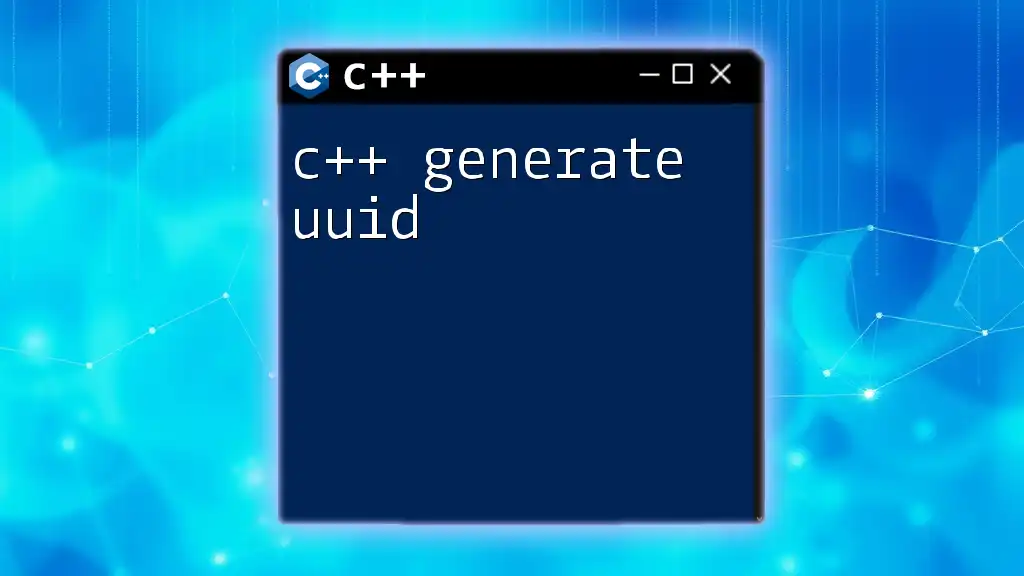
Testing and Debugging Your Mod
Setting Up a Testing Environment
Establish a separate instance of Minecraft specifically for testing your mods. This prevents errors in your primary installation while allowing you to see your mods in action.
Common Debugging Techniques
Debugging is an inevitable part of programming. Familiarize yourself with:
- Breakpoint Debugging: Stop execution at set points to inspect variable states.
- Error Message Analysis: Carefully read compiler error messages for clues about what went wrong.
Using Logging for Debugging
Implementing logging within your code can help track down issues or understand code flow:
#include <iostream>
std::cout << "Debug: Custom block initialized." << std::endl;
This allows you to see messages in the console while running your mod, helping identify where any problems may arise.
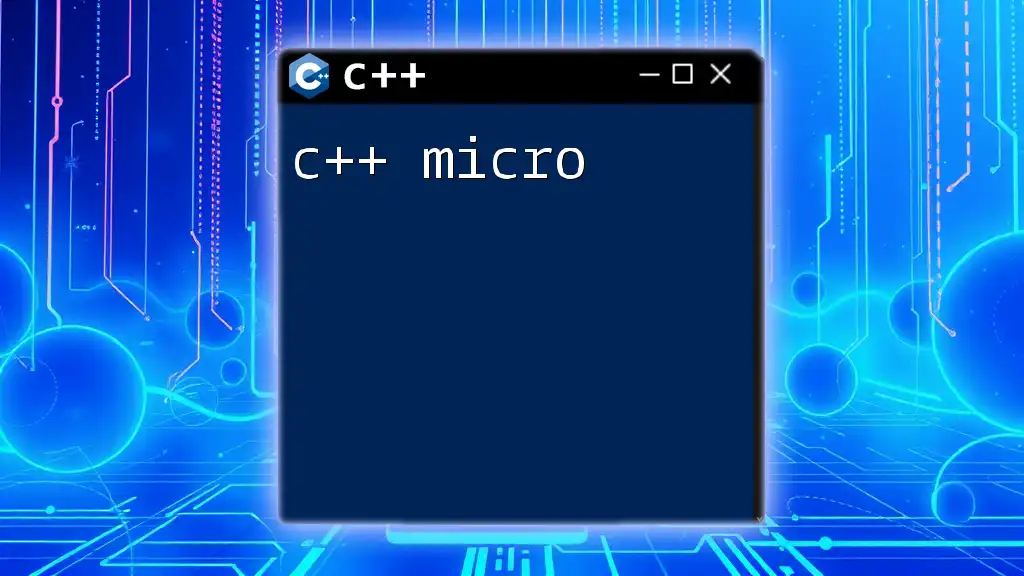
Advanced Modding Techniques
Integrating C++ with Other Languages
Often, it may be necessary to interface C++ with Java. Explore tools like JNI (Java Native Interface) which allow calling C++ functions from Java code, thus combining the performance of C++ with the flexibility of Java.
Creating Custom Entities and Behaviors
Delve into creating unique entities that behave differently from standard game mechanics. This involves registering your entity classes with the Minecraft entity system, allowing them to naturally coexist with game components.
Optimizing Performance
Performance is crucial when modding. Focus on efficient coding practices, such as minimizing expensive operations during the game loop or leveraging intelligent data structures that reduce memory overhead.
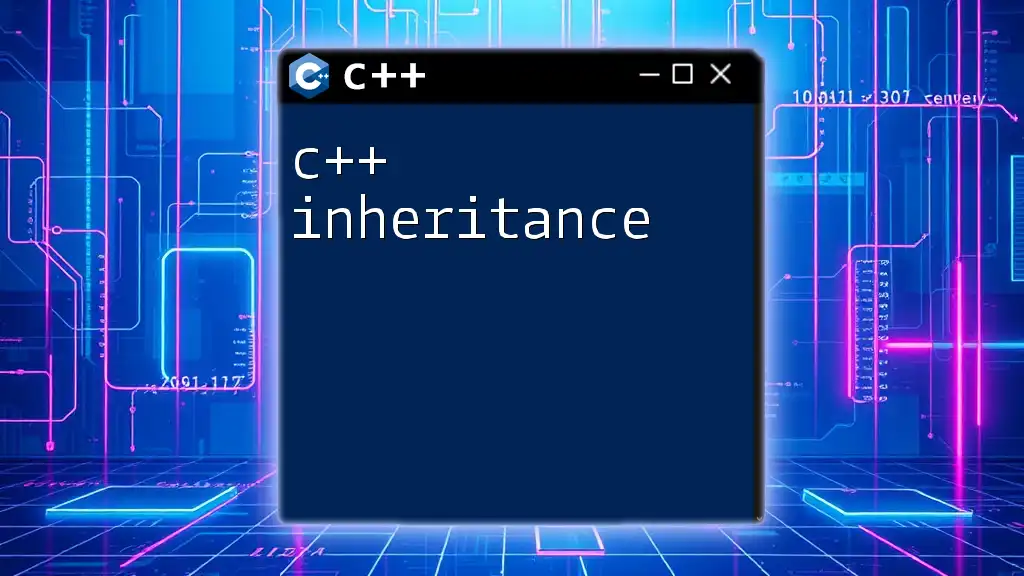
Distribution and Sharing Your Mod
Compiling Your Final Version
Once you’ve refined your mod, compile the final version ensuring it’s optimized and free from debug code.
Where to Share Your Mod
Publishing platforms such as CurseForge, ModDB, or even GitHub offer great spaces to share your creations. Engage with the community to promote your mod and showcase its features.
Gathering User Feedback
User feedback is invaluable. Establish channels through forums, social media, or gameplay videos to interact with users about their experiences, suggestions, and potential bugs to improve your mod continuously.
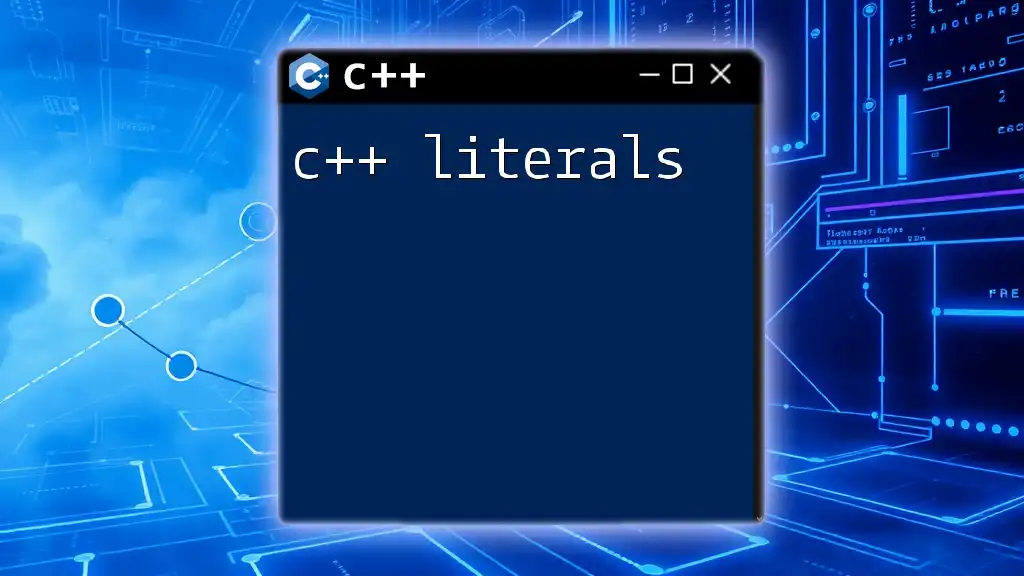
Conclusion
The world of C++ Minecraft modding is vast and promising. As you become more adept with both the C++ language and the nuances of Minecraft's ecosystem, your ability to create engaging, efficient mods will improve immensely. Consider exploring the various resources available and continue experimenting with your skills to unlock endless possibilities.