In C++, inheritance allows a class (derived class) to inherit the properties and behaviors (methods) of another class (base class), promoting code reusability and the creation of hierarchical relationships.
Here's a simple example:
#include <iostream>
using namespace std;
// Base class
class Animal {
public:
void speak() {
cout << "Animal speaks" << endl;
}
};
// Derived class
class Dog : public Animal {
public:
void bark() {
cout << "Dog barks" << endl;
}
};
int main() {
Dog myDog;
myDog.speak(); // Inherited method
myDog.bark(); // Dog's own method
return 0;
}
Understanding C++ Class Inheritance
What is Class Inheritance?
Class inheritance in C++ is a fundamental feature of object-oriented programming (OOP) that allows one class (derived class) to inherit the attributes and behaviors (methods) of another class (base class). This mechanism promotes code reusability, modularity, and a logical structure to your code. By establishing relationships between classes, you can create a hierarchy of functionality, reducing redundancy and simplifying maintenance.
In C++, there are two main classes involved in inheritance: the base class and the derived class. The base class serves as a template, while the derived class inherits certain characteristics and may introduce additional features or modifications.
Types of Inheritance in C++
C++ supports various types of inheritance, each serving a different purpose depending on how you want to structure your class hierarchy:
-
Single Inheritance: This is the simplest form of inheritance, where a derived class inherits from one base class. For example, a `Dog` class might inherit from an `Animal` class.
-
Multiple Inheritance: In this form, a derived class can inherit from multiple base classes. This allows the derived class to incorporate attributes and behaviors from more than one source, like a `FlyingFish` class inheriting from both `Fish` and `Bird`.
-
Multilevel Inheritance: This form occurs when a class is derived from another derived class. For example, if `Animal` is the base class and `Dog` is a derived class, a `Bulldog` class can inherit from `Dog`.
-
Hierarchical Inheritance: In this scenario, multiple derived classes inherit from a single base class. For instance, both `Dog` and `Cat` can inherit from the `Animal` class.
-
Hybrid Inheritance: This is a combination of two or more types of inheritance, demonstrating flexibility in design. Understanding hybrid inheritance requires a grasp of the other types mentioned.
Visualizing these inheritance types can aid in comprehension, as they illustrate how classes interact within your program.
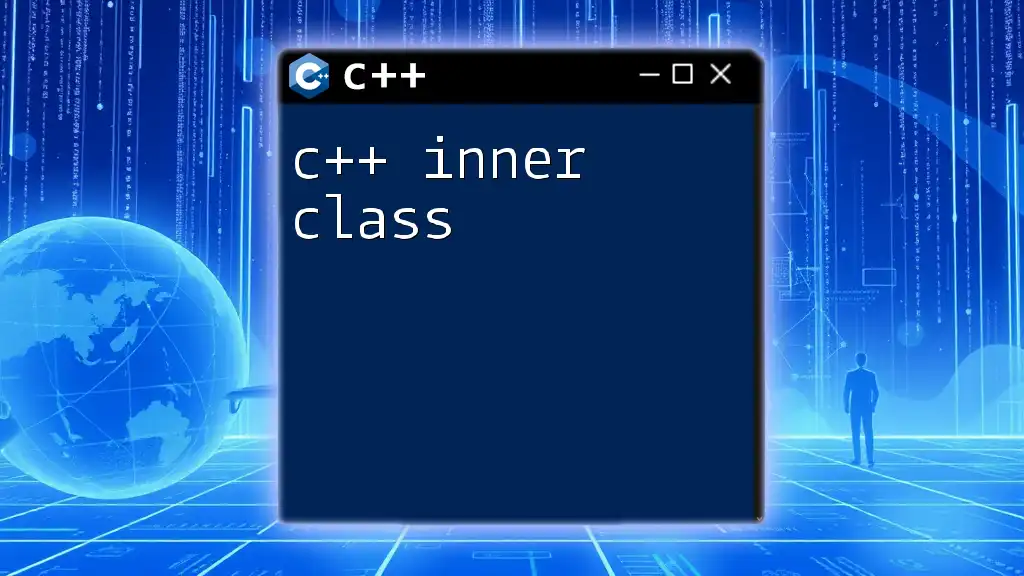
Syntax of Inheriting Classes in C++
Basic Syntax for Single Inheritance
The relationship between a base class and a derived class can be established using the following syntax:
class Base {
// Base class members
};
class Derived : public Base {
// Derived class members
};
Here, `Derived` inherits all public and protected members from `Base`. The `public` keyword signifies that the inheritance is public, allowing access to public members of the base class from the derived class.
Advanced Syntax for Multiple Inheritance
In cases where a derived class needs features from multiple base classes, you can define multiple inheritance as follows:
class Base1 {
// Base1 members
};
class Base2 {
// Base2 members
};
class Derived : public Base1, public Base2 {
// Derived class members
};
In this instance, the `Derived` class inherits from both `Base1` and `Base2`, allowing it to access the members and functions of both classes.
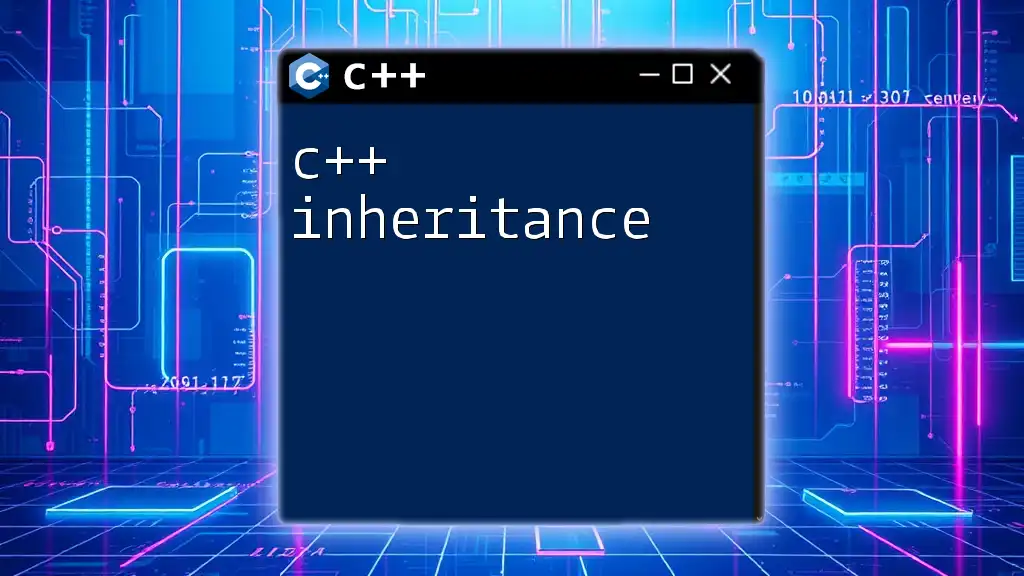
Implementation Examples
Simple Example: Animal and Dog Classes
Let’s examine a practical example demonstrating single inheritance. Consider an `Animal` class and a derived `Dog` class:
class Animal {
public:
void sound() {
std::cout << "Some sound";
}
};
class Dog : public Animal {
public:
void sound() {
std::cout << "Bark";
}
};
In this example, the `Dog` class inherits the `sound` method from `Animal`. However, it overrides this method to provide its custom implementation. This is an example of method overriding, a powerful concept that plays into polymorphism—the ability to call derived class functions using base class references or pointers.
Practical Example: Shape and Polygon Classes
Now, let’s explore multilevel inheritance through a simple shape hierarchy:
class Shape {
public:
void draw() {
std::cout << "Drawing a shape";
}
};
class Polygon : public Shape {
public:
void draw() {
std::cout << "Drawing a polygon";
}
};
class Rectangle : public Polygon {
public:
void draw() {
std::cout << "Drawing a rectangle";
}
};
In this case, the `Rectangle` class is derived from `Polygon`, which in turn derives from `Shape`. Each class showcases its `draw` method, demonstrating method overriding at different levels of the hierarchy.
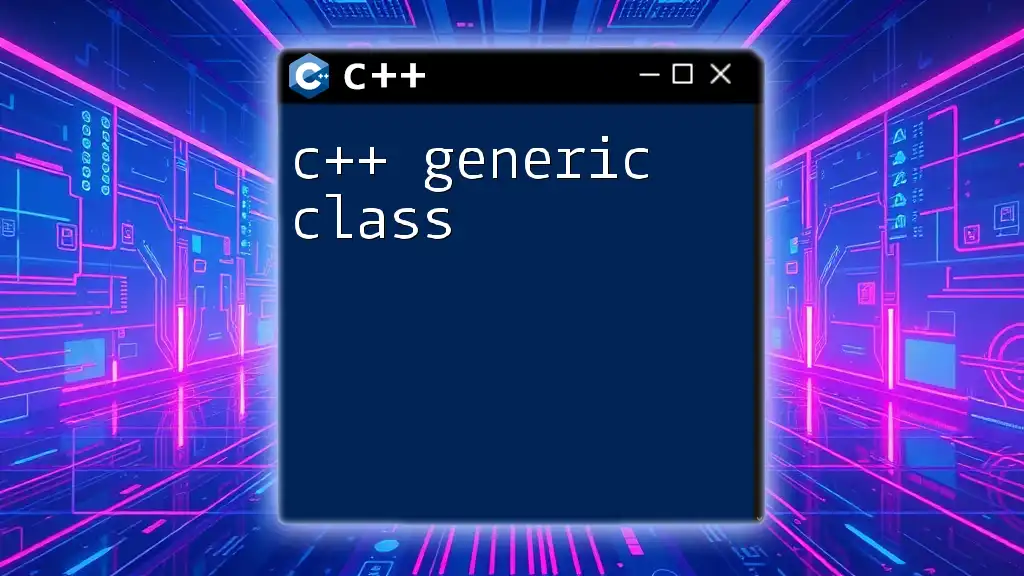
Access Modifiers and Their Effects on Inheritance
Access modifiers play a significant role in how members of the base class are accessed by derived classes.
Public, Protected, and Private Inheritance
-
Public Inheritance: Members of the base class are accessible in derived classes and can also be accessed by other classes and functions. This is the most common type of inheritance and reflects an "is-a" relationship.
-
Protected Inheritance: Members of the base class become protected in the derived class, meaning they are only accessible within the derived class and its subclasses but not outside these classes. This restricts access and is suitable in certain design situations.
-
Private Inheritance: Members of the base class become private in the derived class. This means they can only be accessed by member functions of the derived class and are hidden from other classes, which can be useful in specific architectural scenarios.
Understanding how to effectively utilize these access levels is crucial for the security and integrity of your code.
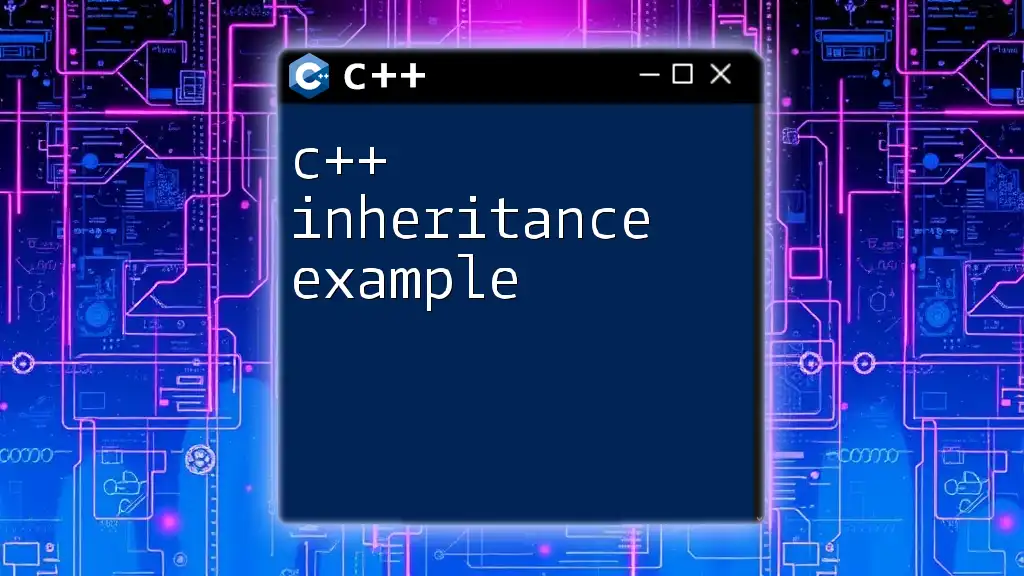
Common Mistakes and How to Avoid Them
Mistake 1: Not Understanding Access Modifiers
One common error among developers is misunderstanding access modifier implications. For instance, using `private` inheritance when `public` is more appropriate can lead to unnecessary complexity. Always assess your design to ensure that access levels align with your intent and the desired visibility of class members.
Mistake 2: Diamond Problem in Multiple Inheritance
When working with multiple inheritance, issues can arise, such as the diamond problem. This occurs when two base classes inherit from the same grandparent class. Here’s an example:
class A { /* ... */ };
class B : public A { /* ... */ };
class C : public A { /* ... */ };
class D : public B, public C { /* ... */ }; // Potential issues here
In this scenario, the compiler is uncertain which version of class `A` to use, leading to ambiguity.
To resolve the diamond problem, you can use virtual inheritance:
class A { /* ... */ };
class B : virtual public A { /* ... */ };
class C : virtual public A { /* ... */ };
class D : public B, public C { /* ... */ }; // Resolved diamond problem
By declaring `B` and `C` to inherit `A` virtually, you ensure that there is only one base class `A` in the `D` class, thereby eliminating ambiguity.
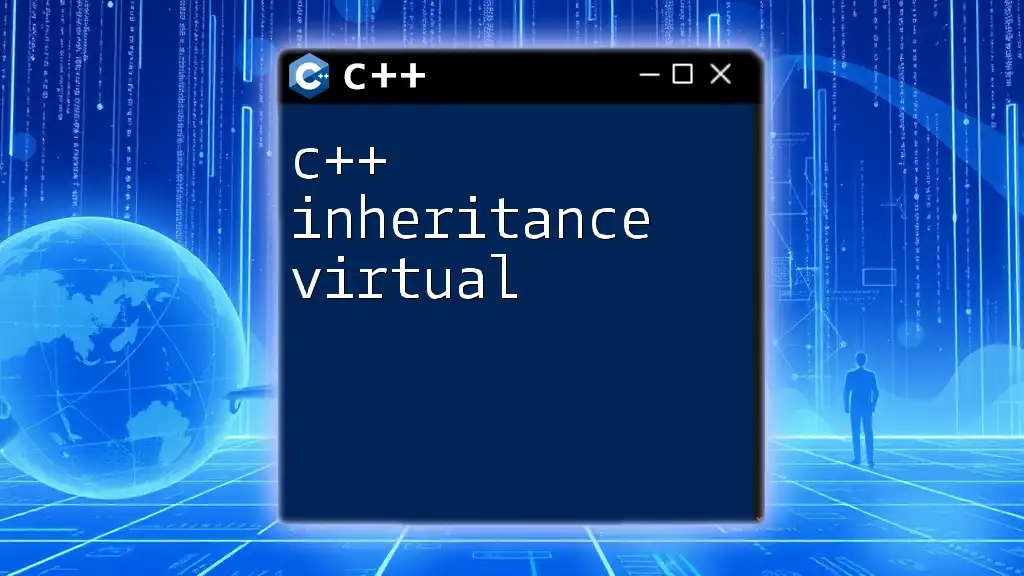
Conclusion
In this exploration of C++ class inheritance, we’ve unpacked essential concepts such as different types of inheritance, syntax basics, implications of access modifiers, and common pitfalls. Mastery of class inheritance is crucial for leveraging the full power of C++ in object-oriented programming. By practicing inheritance in your coding projects, you can design more sophisticated and efficient software solutions.