A C++ timer class is a utility that enables you to measure the execution time of your code or perform actions after a specified duration, providing an easy way to manage time-related functionalities.
Here's a simple implementation of a timer class in C++:
#include <iostream>
#include <chrono>
class Timer {
public:
Timer() : start_time(std::chrono::high_resolution_clock::now()) {}
void reset() { start_time = std::chrono::high_resolution_clock::now(); }
double elapsed() {
return std::chrono::duration<double>(std::chrono::high_resolution_clock::now() - start_time).count();
}
private:
std::chrono::high_resolution_clock::time_point start_time;
};
int main() {
Timer timer;
// Simulate work
for (volatile int i = 0; i < 1e7; ++i);
std::cout << "Elapsed time: " << timer.elapsed() << " seconds" << std::endl;
return 0;
}
Understanding the Basics of Timing in C++
Timing is a fundamental concept in programming, particularly in performance measurement, animation, and timing events. In C++, there are different types of timers that can be employed, including:
- Real-time clocks: These are useful for tracking the system time.
- High-resolution timers: These are essential for measuring small time intervals accurately, supporting performance testing and time-sensitive applications.
With the introduction of the `<chrono>` library in C++11, timing tasks has become more straightforward and powerful, enabling developers to create high-performance applications that require precise timing capabilities.

Creating a Simple Timer Class
Designing the Timer Class
When designing a Timer Class in C++, a few crucial features should be taken into account:
- Start time: Record the moment the timer starts.
- End time or duration: Capture the moment when the timer stops or calculate the elapsed time on-demand.
- Accuracy: Leverage high-resolution clocks to ensure precision.
By encapsulating the timing functionality in a class, you can create a reusable and intuitive tool that can be adapted to various applications.
Writing the Timer Class Code
Below is a basic implementation of a Timer Class using the `<chrono>` library:
#include <chrono>
class Timer {
private:
std::chrono::time_point<std::chrono::high_resolution_clock> startTime;
public:
Timer() {
startTime = std::chrono::high_resolution_clock::now();
}
void reset() {
startTime = std::chrono::high_resolution_clock::now();
}
double elapsed() const {
auto endTime = std::chrono::high_resolution_clock::now();
return std::chrono::duration<double>(endTime - startTime).count();
}
};
Explanation:
- The class captures the current time using `std::chrono::high_resolution_clock::now()` at the moment of instantiation.
- The `reset()` method allows users to restart the timer at any point.
- The `elapsed()` method returns the total seconds passed since the timer started, making use of `std::chrono::duration<double>` for high accuracy.
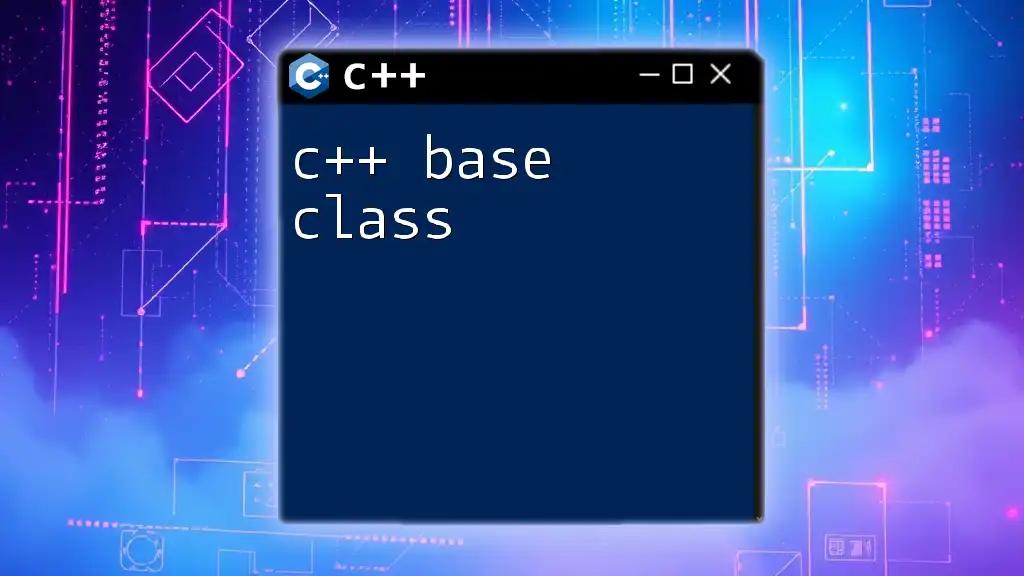
Using the Timer Class
Starting and Stopping the Timer
Here’s how you can utilize the Timer Class in a simple program:
#include <iostream>
#include <thread>
int main() {
Timer timer;
// Simulate a task
std::this_thread::sleep_for(std::chrono::seconds(2));
std::cout << "Elapsed time: " << timer.elapsed() << " seconds" << std::endl;
return 0;
}
Explanation:
In the code above, we create an instance of the Timer Class, simulate a delay of 2 seconds using `std::this_thread::sleep_for()`, and print the elapsed time. When you run this program, it will output approximately "Elapsed time: 2 seconds," showcasing the functionality of the Timer Class.
Multiple Timer Instances
Creating multiple instances of the Timer Class allows each timer to maintain its state independently. Here’s an example demonstrating this functionality:
#include <iostream>
#include <thread>
void task() {
Timer timer;
// Simulate work
std::this_thread::sleep_for(std::chrono::milliseconds(500));
std::cout << "Task took: " << timer.elapsed() << " seconds" << std::endl;
}
int main() {
task(); // Task runs, displaying elapsed time
task(); // Each task runs independently
}
Explanation:
In this case, each invocation of the `task()` function creates a new Timer instance, allowing independent timing for each execution. Each instance accurately tracks the time spent within the function, reiterating the usefulness of encapsulating timing logic within a class.

Advanced Timer Features
Adding Lap Functionality
Lap functionality allows you to mark multiple time intervals within a single timer session. This is particularly useful for applications like sports timings or performance tracking. Here’s how you can extend the Timer Class to support this feature:
#include <vector>
class AdvancedTimer : public Timer {
private:
std::vector<double> lapTimes;
public:
void markLap() {
lapTimes.push_back(elapsed());
}
const std::vector<double>& getLaps() const {
return lapTimes;
}
};
Explanation:
This `AdvancedTimer` class inherits from the original Timer class, adding functionality to store lap times in a vector. The `markLap()` method adds the current elapsed time to the `lapTimes` vector, enabling users to capture their progress at various stages.
Example of Using Advanced Timer
Here’s how you can implement the Advanced Timer in a program:
#include <iostream>
#include <thread>
int main() {
AdvancedTimer timer;
for (int i = 0; i < 3; ++i) {
std::this_thread::sleep_for(std::chrono::seconds(1));
timer.markLap();
}
std::cout << "Lap times: ";
for (const auto& lap : timer.getLaps()) {
std::cout << lap << " sec, ";
}
return 0;
}
Explanation:
The above program simulates three tasks separated by a 1-second delay. Each time `markLap()` is called, the current elapsed time is recorded. Finally, the program outputs the recorded lap times. This displays the practical use of laps in tracking progress, demonstrating how the extended functionality of the Timer Class can be employed efficiently.

Best Practices for Using Timers
When implementing a Timer Class, consider the following best practices:
- Error Handling: Ensure that your code gracefully handles any potential exceptions that might arise, such as invalid operations on the timer.
- Performance Considerations: Be aware of the overhead associated with high-resolution timers and avoid using them in performance-critical sections where frequent calls are made.
- Code Readability: Maintain clean and understandable code, commenting on sections that perform critical functions, to aid future developers or maintainers.
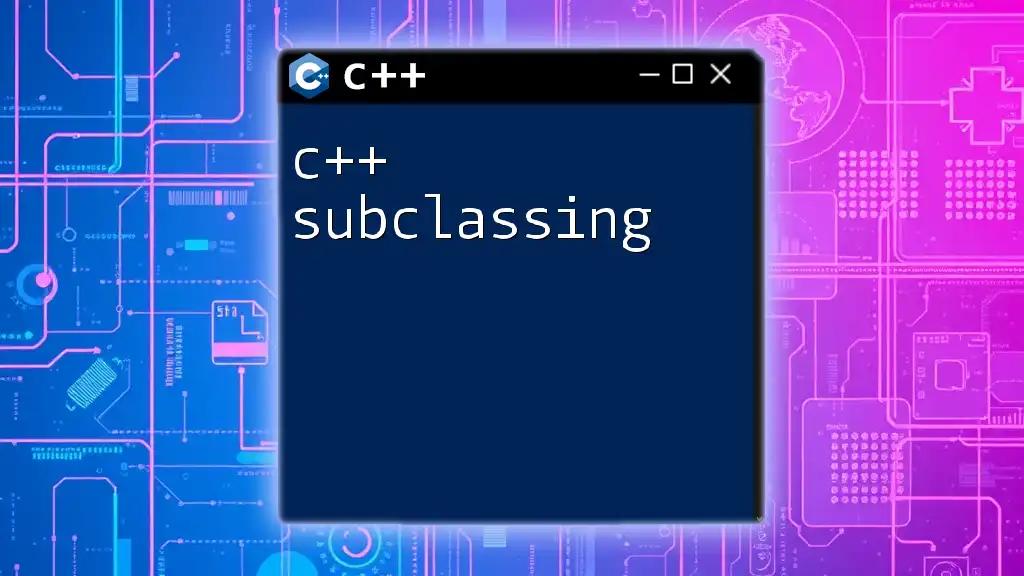
Conclusion
The C++ Timer Class is a powerful tool that enhances your programming toolkit, providing precise timing capabilities suitable for a variety of applications. Whether you’re tracking performance, handling animations, or simply measuring execution time, the Timer Class makes it simple and effective.
Experiment with the timer implementations presented in this article, and explore how you can adapt these concepts to your projects. By incorporating timing mechanisms into your workflows, you can build more efficient and responsive applications.
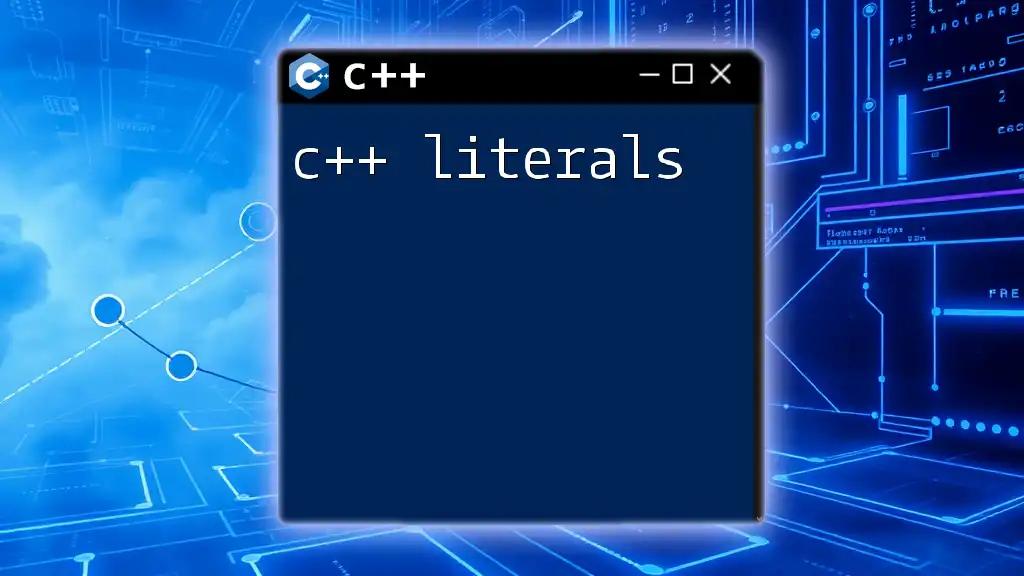
Additional Resources
- For more information, refer to the official C++ documentation on the `<chrono>` library.
- Consider exploring community forums for additional insights and discussions regarding timer implementations and optimizations.
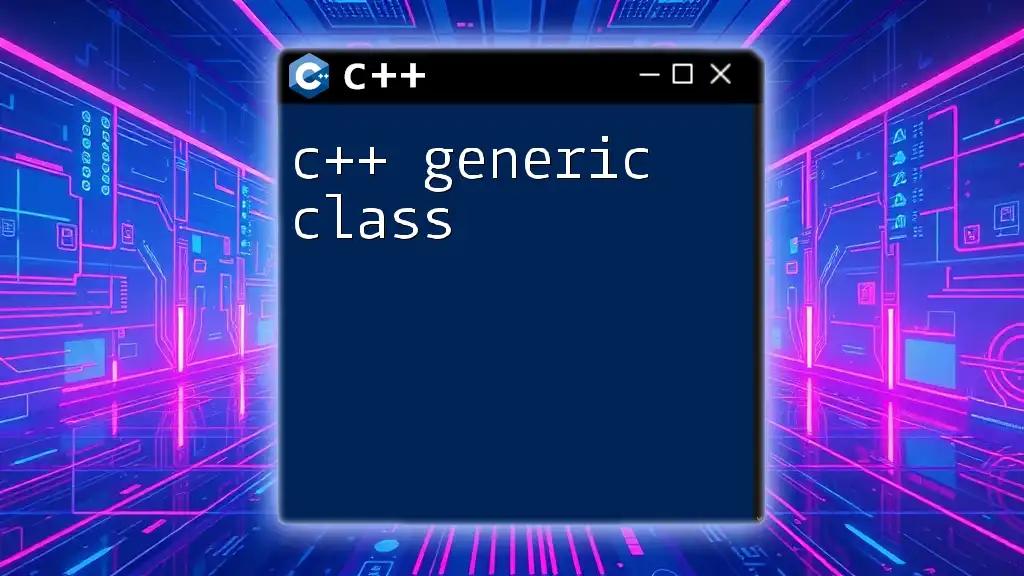
FAQs
-
What is `std::chrono` and why is it used? `std::chrono` is a part of the C++ standard library that provides features for manipulating time. It allows developers to measure time intervals accurately and perform timing operations conveniently.
-
Can timers be used in multithreaded applications? Yes, timers can be utilized in multithreaded applications; however, special care must be taken to manage concurrent access to shared resources.
-
How do I optimize my Timer Class for performance? To optimize your Timer Class, consider minimizing the overhead caused by high-resolution clocks, reduce unnecessary calculations, and ensure that you construct timers with appropriate granularities for your specific use case.