In C++, the array class allows you to create a fixed-size array with built-in functionalities such as accessing elements and getting the size of the array, making it easier to manage collections of data.
#include <array>
#include <iostream>
int main() {
std::array<int, 5> myArray = {1, 2, 3, 4, 5};
std::cout << "First element: " << myArray[0] << std::endl;
std::cout << "Size of array: " << myArray.size() << std::endl;
return 0;
}
Understanding Arrays in C++
What is an Array?
An array is a container that holds a fixed-size sequential collection of elements of the same type. This means that an array can store multiple items of the same data type under a single identifier, making it an efficient way to manage related data.
Arrays in C++ can be static (fixed-size) or dynamic (where the size can change during runtime). The static arrays are defined with a constant size at the time of declaration, while dynamic arrays can be allocated and deallocated from the heap, allowing for more flexible memory usage.
Example: Basic Syntax of Defining an Array in C++
int myArray[5]; // Declares an array of five integers
Types of Arrays
Single-Dimensional Arrays
Single-dimensional arrays, often referred to simply as arrays, are linear collections. Each element can be accessed using an index.
Code Snippet: Declare and Initialize a Single-Dimensional Array
int numbers[5] = {1, 2, 3, 4, 5}; // Initializes an array with 5 integers
Multi-Dimensional Arrays
Multi-dimensional arrays, such as two-dimensional arrays, can be thought of as arrays of arrays. They can be used to store data in tabular form (like matrices).
Code Snippet: Declare and Initialize a Two-Dimensional Array
int matrix[3][3] = { {1, 2, 3}, {4, 5, 6}, {7, 8, 9} }; // A 3x3 matrix
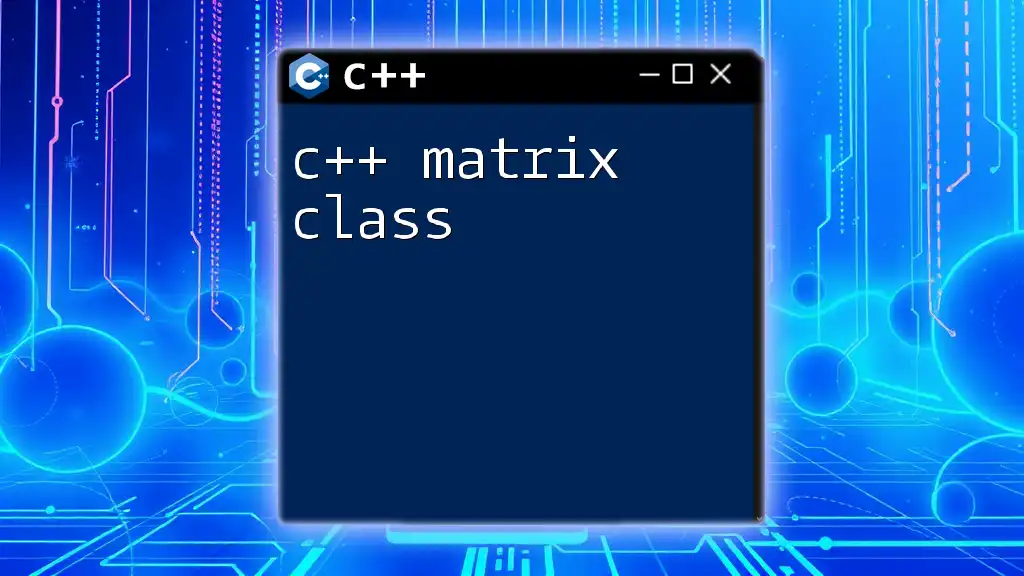
C++ Array Class
Overview of the C++ Standard Library Array
The C++ Standard Library provides a powerful container class called `std::array`, which is defined in the `<array>` header. This class offers an encapsulated array that comes with additional functionalities while maintaining the performance characteristics of a regular array.
Key Features of std::array
Fixed Size
`std::array` has a fixed size defined at compile time, meaning that the size cannot be modified during runtime. This characteristic ensures efficient memory allocation and access speed.
Advantages:
- Memory management is much simpler.
- Lower chances of memory-related bugs.
Ease of Use
The `std::array` class offers greater ease of use, providing member functions (such as `.begin()`, `.end()`, and `.size()`) and is compatible with STL algorithms. This integration simplifies tasks like sorting and searching.
Example: Using `std::array` in a Simple Program
#include <array>
#include <iostream>
int main() {
std::array<int, 5> arr = {10, 20, 30, 40, 50}; // Declare a std::array
std::cout << "First element: " << arr[0] << std::endl;
return 0;
}
Basic Operations with std::array
Initialization
You can initialize a `std::array` in several ways. The standard approach is as follows:
Code Snippet: Various Initialization Techniques
std::array<int, 3> arr1 = {1, 2, 3}; // Uniform initialization
std::array<int, 3> arr2 = std::array<int, 3>{4, 5, 6}; // Direct initialization
Accessing Elements
You can access elements in `std::array` using either the safe `.at()` method or the standard indexing operator `[]`. The `.at()` method throws an exception when accessing out-of-bounds elements, ensuring safety.
Example: Demonstrating Both Access Methods
std::array<int, 3> arr = {1, 2, 3};
std::cout << "Value using at(): " << arr.at(1) << std::endl; // Safe access
std::cout << "Value using []: " << arr[1] << std::endl; // Unsafe access without bounds checking
Iterating Over Elements
You can easily iterate over the elements of `std::array` using range-based for loops, simplifying the process of accessing each element.
Code Snippet: Range-based Loop Example
std::array<int, 3> arr = {1, 2, 3};
for (int value : arr) {
std::cout << value << " ";
}
Important Member Functions of std::array
size()
The `size()` member function returns the number of elements in the array, providing a simple way to retrieve its length.
std::array<int, 3> arr = {1, 2, 3};
std::cout << "Size of the array: " << arr.size() << std::endl; // Outputs 3
fill()
The `fill()` method allows you to fill all elements of the array with the same value. This is particularly useful for initializing an array quickly.
Code Snippet: Using `fill()`
std::array<int, 3> arr;
arr.fill(5); // Fill all elements with the value 5
swap()
The `swap()` method allows you to exchange the contents of two `std::array` objects, making it straightforward to manage temporary storage or caching.
Comparison: C-style Arrays vs std::array
Memory Management
C-style arrays require manual memory management, which can lead to memory leaks and undefined behavior if not handled correctly. In contrast, `std::array` manages its memory automatically, reducing the chance of errors.
Performance Considerations
While C-style arrays allow for some low-level operations that might be faster in specific contexts, they come with inherent risks. `std::array` strikes a balance between performance and safety, making it the preferred choice for most applications.
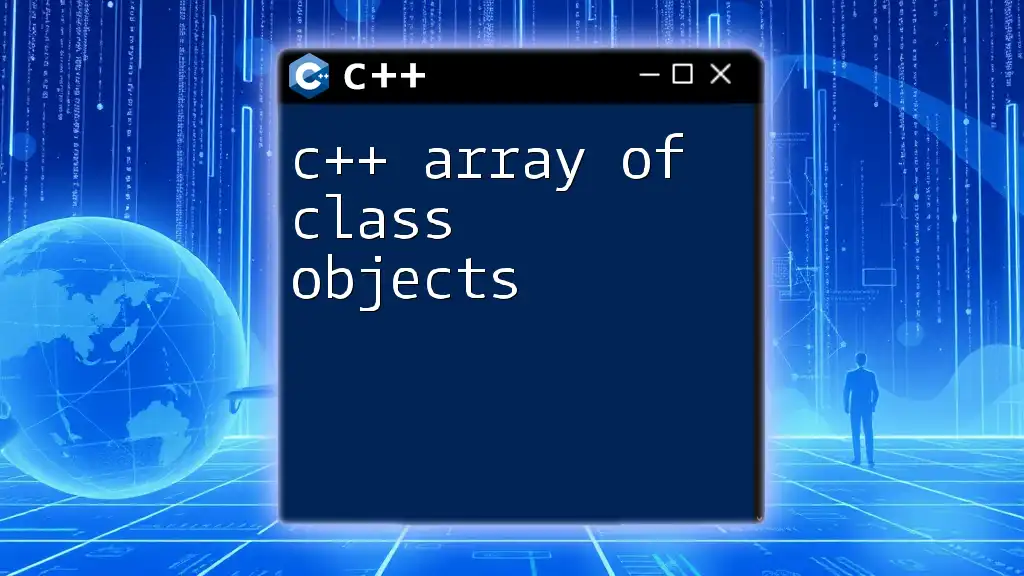
Advanced Features of C++ Arrays
Template Specialization for Custom Arrays
C++ allows for creating templates, which can be beneficial where you need to manage arrays of different types without duplicating code.
Example: Creating a Simple Template Array Class
template<typename T, std::size_t N>
class CustomArray {
private:
std::array<T, N> arr;
public:
// Access elements
T& operator[](std::size_t index) {
return arr.at(index);
}
// Get size
std::size_t size() const {
return arr.size();
}
};
Using Arrays with Functions
When passing arrays to functions, you can choose to pass them either by value or by reference. Passing by reference preserves memory and is usually more efficient than creating a copy.
Code Snippet: Example Function Demonstrating Array Passing
void printArray(std::array<int, 3>& arr) {
for (int value : arr) {
std::cout << value << " ";
}
}
Arrays and STL Algorithms
The STL integrates smoothly with `std::array`, allowing for utility functions like `std::sort` to operate directly on arrays, enhancing their functionality.
Example: Using `std::sort` with `std::array`
#include <algorithm>
std::array<int, 5> arr = {5, 1, 4, 2, 3};
std::sort(arr.begin(), arr.end()); // Sorts the array in ascending order
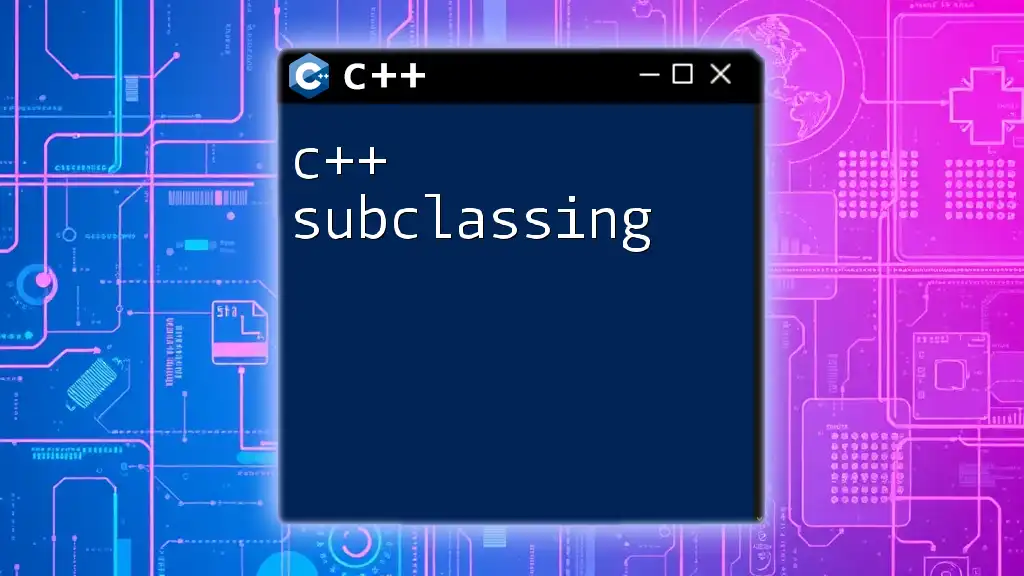
Common Mistakes to Avoid with C++ Arrays
When working with arrays, especially C-style arrays, developers often encounter pitfalls:
- Off-by-one errors in indexing: Due to the zero-based indexing of arrays, developers might accidentally exceed the bounds, leading to undefined behavior.
- Forgetting the size of the array: This can lead to attempts to access non-existent memory.
- Not considering scope and lifetime of arrays: Local arrays go out of scope after a function ends; if not managed properly, this could lead to stack corruption or access violations.
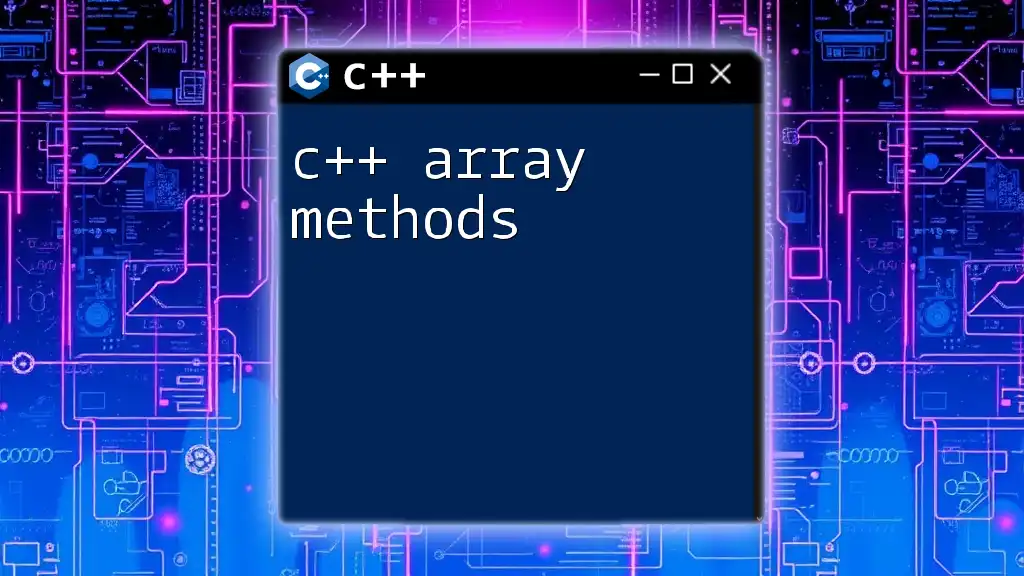
Practical Applications of C++ Arrays
Real-world Scenarios
Use Cases:
- Gaming: Arrays are fundamental in gaming for creating game boards or storing player data efficiently.
- Data Processing: Arrays are extensively used in statistical computations for handling modern big data.
In conclusion, understanding the C++ Array Class is crucial for effective programming in C++. Whether you use fixed-size arrays or modern `std::array`, mastering their use can significantly improve your code's efficiency and clarity. Make sure to explore and practice these concepts to enhance your proficiency in C++.