An array of pointers in C++ is a collection of pointer variables that can each point to different elements or data types, allowing for flexible memory management and data structure manipulation.
Here's a code snippet to illustrate an array of pointers:
#include <iostream>
int main() {
int values[] = {10, 20, 30};
int* pointers[3]; // Array of pointers
for (int i = 0; i < 3; i++) {
pointers[i] = &values[i]; // Assign addresses of values
}
// Accessing the values using pointers
for (int i = 0; i < 3; i++) {
std::cout << *pointers[i] << std::endl; // Dereference pointers to get values
}
return 0;
}
Understanding Pointers in C++
What is a Pointer?
A pointer in C++ is a variable that stores the memory address of another variable. Understanding pointers is crucial in C++ as they provide direct access to memory and enable sophisticated data manipulation.
To declare a pointer, you use the `*` operator. For example:
int* ptr;
In this snippet, `ptr` is declared as a pointer to an integer. Initializing pointers is essential, either by pointing them to an existing variable or by using the `new` keyword for dynamic allocation. Remember to always initialize pointers to avoid undefined behavior.
Why Use Pointers?
Pointers are powerful tools for several reasons:
- Memory Efficiency: Pointers allow you to efficiently manage memory by directly accessing memory locations instead of copying large data structures.
- Dynamic Memory Allocation: Using pointers lets you allocate memory at runtime, making your programs more flexible.
- Building Complex Data Structures: Many data structures, such as linked lists and trees, rely on pointers to connect nodes easily.
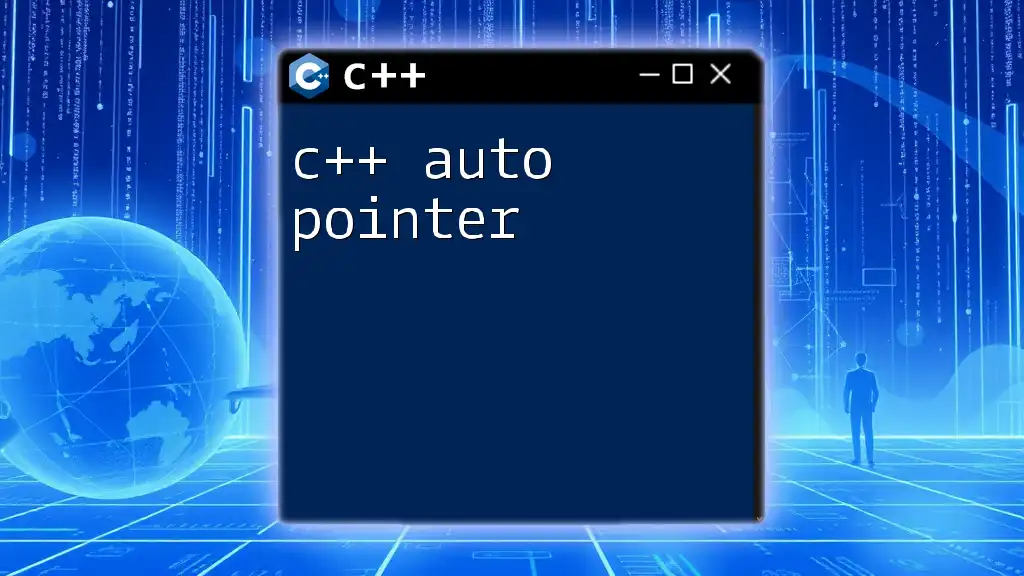
Introduction to Arrays
What is an Array?
An array is a contiguous block of memory that holds multiple variables of the same type. Arrays simplify storing and accessing large datasets. For instance, you can declare an array of integers as follows:
int myArray[5] = {10, 20, 30, 40, 50};
In this example, `myArray` can hold five integers, accessed via indexes starting from 0.
Using Arrays in C++
Accessing elements from an array is straightforward. You can retrieve values by their index:
int firstElement = myArray[0]; // firstElement is now 10
You can also modify array elements in a similar manner:
myArray[2] = 60; // This changes the third element from 30 to 60
Understanding how to manipulate arrays is fundamental before diving into arrays of pointers.
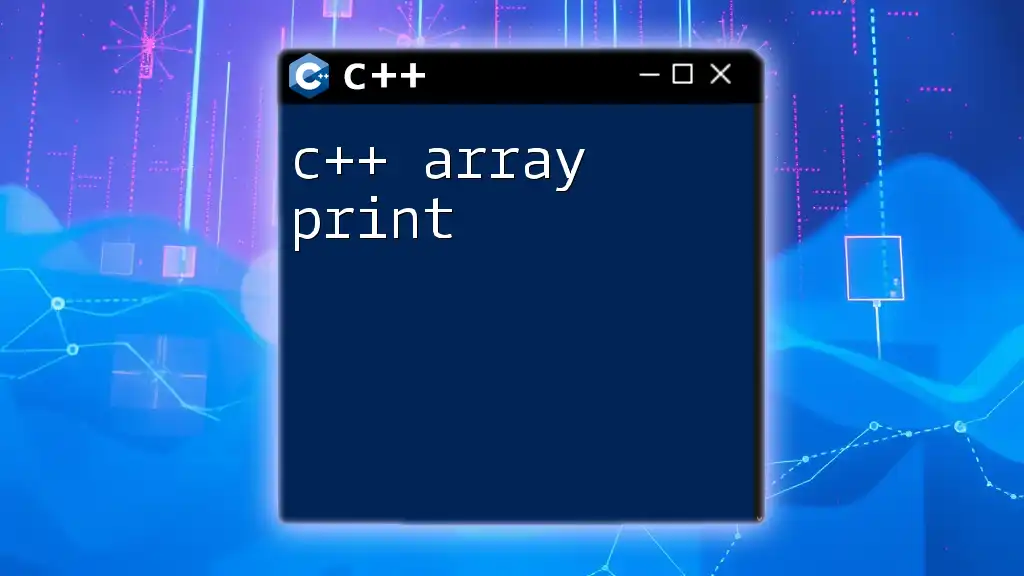
C++ Array of Pointers
What is an Array of Pointers?
An array of pointers is a collection where each element is a pointer, generally pointing to a variable or a data structure. This concept extends the idea of a regular array by allowing each element to reference a different memory location, making it especially useful for handling dynamic data.
For example, consider this declaration of an array of integer pointers:
int* ptrArray[3];
Here, `ptrArray` can hold three pointers, each capable of pointing to an integer.
Declaration and Initialization of an Array of Pointers
To initialize an array of pointers, you'll typically create individual pointer variables and assign them:
int a = 10, b = 20, c = 30;
int* ptrArray[3] = {&a, &b, &c};
In this code snippet, `ptrArray` holds the addresses of `a`, `b`, and `c`.
Accessing Elements in an Array of Pointers
To access elements through an array of pointers, dereference the pointer:
for (int i = 0; i < 3; ++i) {
std::cout << *ptrArray[i] << " "; // Output: 10 20 30
}
This loop prints the values stored at the memory addresses present in `ptrArray`.
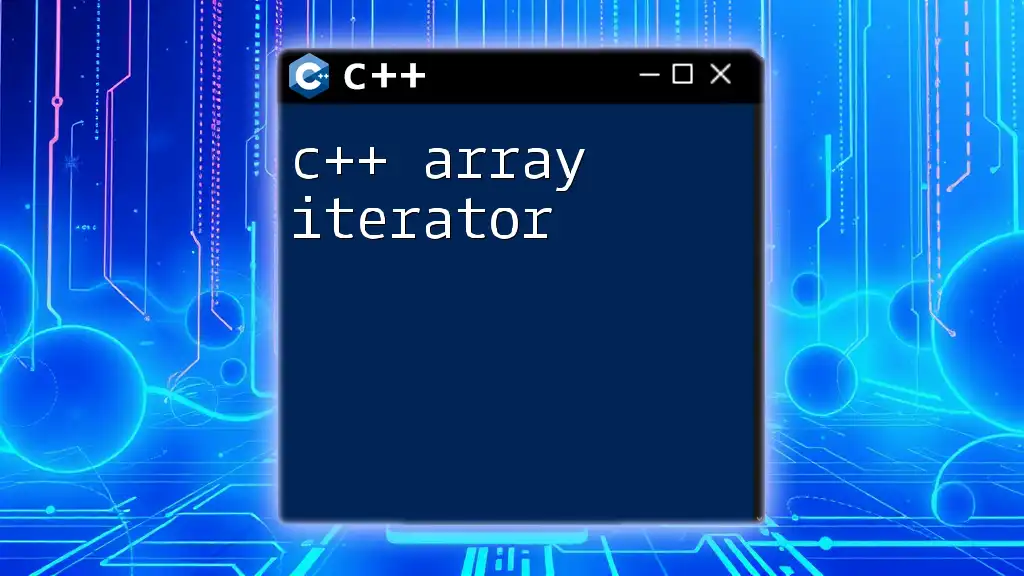
Advanced Topics
Array of Array Pointers in C++
An array of array pointers extends the concept of an array of pointers, allowing for multi-dimensional data structures. This is particularly useful for implementing 2D arrays dynamically.
Here's how to declare a 2D array using pointers:
int** array2D;
array2D = new int*[rows];
for (int i = 0; i < rows; ++i) {
array2D[i] = new int[cols];
}
In this example, `array2D` becomes an array of pointers, with each pointer pointing to a newly allocated array of integers (representing a row). Remember to free the memory allocated:
for (int i = 0; i < rows; ++i) {
delete[] array2D[i]; // Delete each row
}
delete[] array2D; // Delete the array of pointers
Dynamic Allocation with Array of Pointers
Dynamic memory allocation is a powerful feature in C++, allowing flexibility when handling arrays of pointers. This can be done using the `new` and `delete` keywords, as shown:
int* dynamicArray = new int[5]; // Allocate an array of 5 integers
Access and modify the values just like a regular array. However, always remember to free the dynamically allocated memory to prevent leaks:
delete[] dynamicArray; // Deallocate memory
Common Pitfalls and Best Practices
When working with arrays of pointers, there are common mistakes to watch out for:
- Avoiding Dangling Pointers: Ensure pointers do not reference deleted memory.
- Initialization: Always initialize pointers. Uninitialized pointers can lead to undefined behavior.
Following best practices such as RAII (Resource Acquisition Is Initialization) can help manage memory more efficiently and reduce errors.
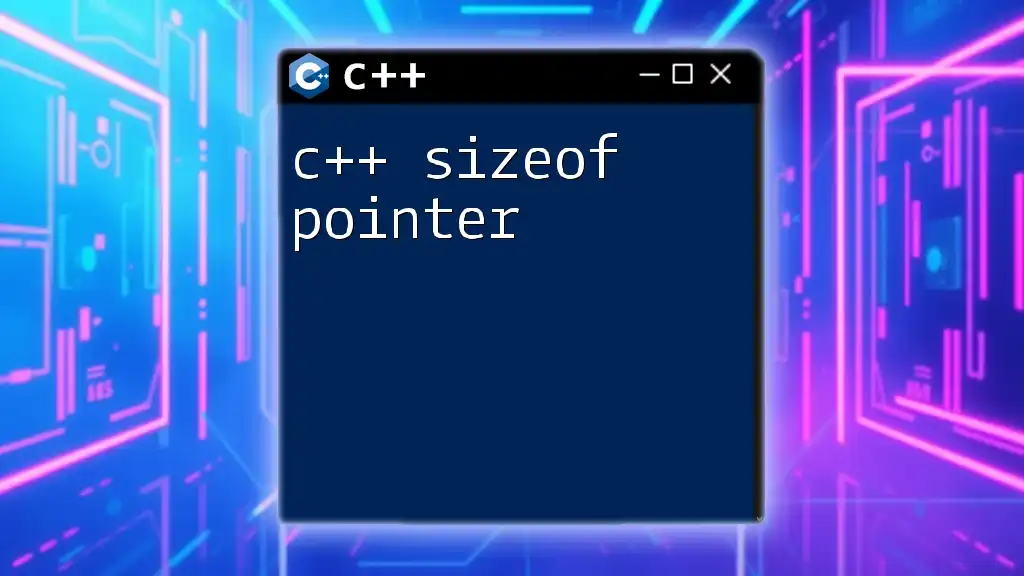
Practical Applications
Real-World Examples of Array of Pointers
A practical application of an array of pointers is in the construction of linked lists. Each node in a linked list can point to the next node, and an array can be used to keep track of these pointers.
For example, in a filtered list of names:
std::string* namesArray[3];
namesArray[0] = new std::string("Alice");
namesArray[1] = new std::string("Bob");
namesArray[2] = new std::string("Charlie");
This provides a flexible and efficient way to handle data.
Performance Considerations
When using an array of pointers, it's important to consider performance. Accessing elements in a standard array is usually faster because the data is stored contiguously in memory. In contrast, arrays of pointers may introduce overhead due to their non-contiguous nature. However, when dealing with large data structures where items have variable sizes, using an array of pointers often provides more flexibility and efficiency.
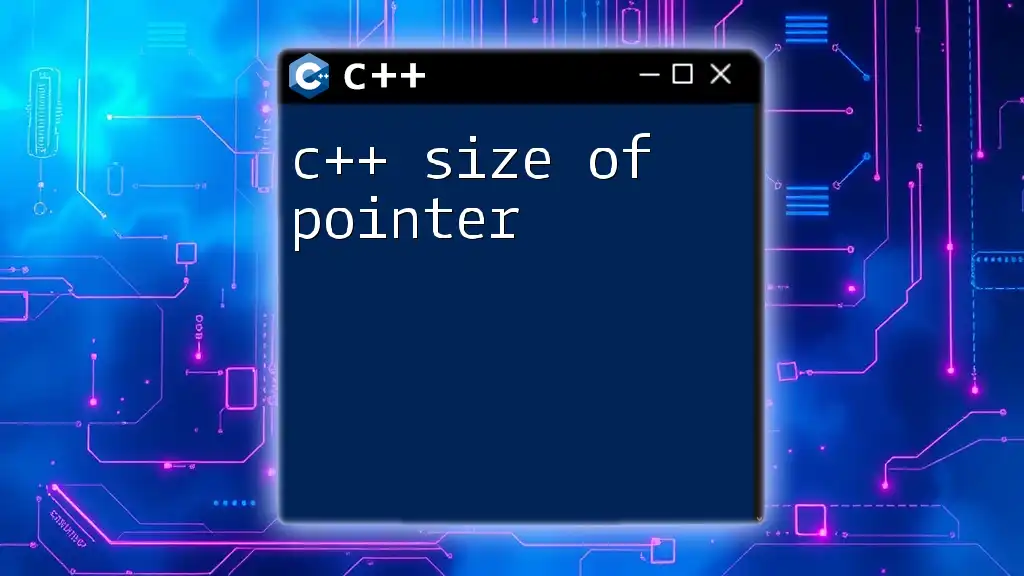
Conclusion
In this article, we explored the concept of a C++ array of pointers, understanding its structure, benefits, and practical applications. Mastering arrays and pointers is foundational for any aspiring C++ developer. With the right knowledge, you can efficiently manipulate data structures and optimize performance in your programs.
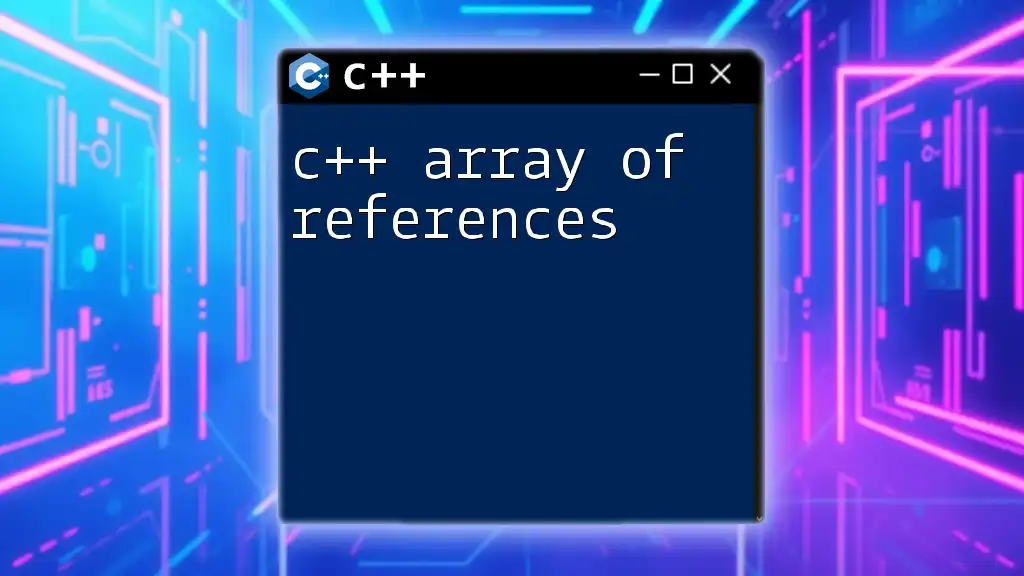
Further Resources
To deepen your understanding of C++ pointers and arrays, consider exploring the following resources:
- Comprehensive C++ programming books
- Online platforms offering interactive C++ tutorials
- Community forums for troubleshooting and collaboration on projects
Code Snippets Repository
For easy access and reference, we encourage readers to compile a collection of code snippets discussed throughout the article. This can serve as a valuable learning tool during implementation.