In C++, you can print the elements of an array using a simple loop that iterates through the array's indices. Here's how you can do it:
#include <iostream>
using namespace std;
int main() {
int arr[] = {1, 2, 3, 4, 5};
int length = sizeof(arr) / sizeof(arr[0]);
for (int i = 0; i < length; i++) {
cout << arr[i] << " ";
}
return 0;
}
Understanding C++ Arrays
What is an Array in C++?
An array in C++ is a collection of elements that are of the same type, organized in a contiguous block of memory. To define an array, you specify the type of its elements and the number of elements it will hold. For example, `int arr[5];` defines an array named `arr` that can hold five integers.
Arrays are indexed, meaning you can access each element via its index, starting from 0. This makes arrays efficient for both memory usage and access speed, but it is crucial to manage array bounds carefully to avoid accessing out-of-range elements.
Why Use Arrays?
Arrays play a vital role in C++ programming for several reasons:
- Memory Efficiency: Arrays allocate contiguous memory blocks, which can enhance performance.
- Easy Data Management: When working with a collection of similar items (like a list of scores), arrays simplify data manipulation.
- Convenience: Most algorithms operate on sequences of data and using arrays makes implementing these algorithms straightforward.
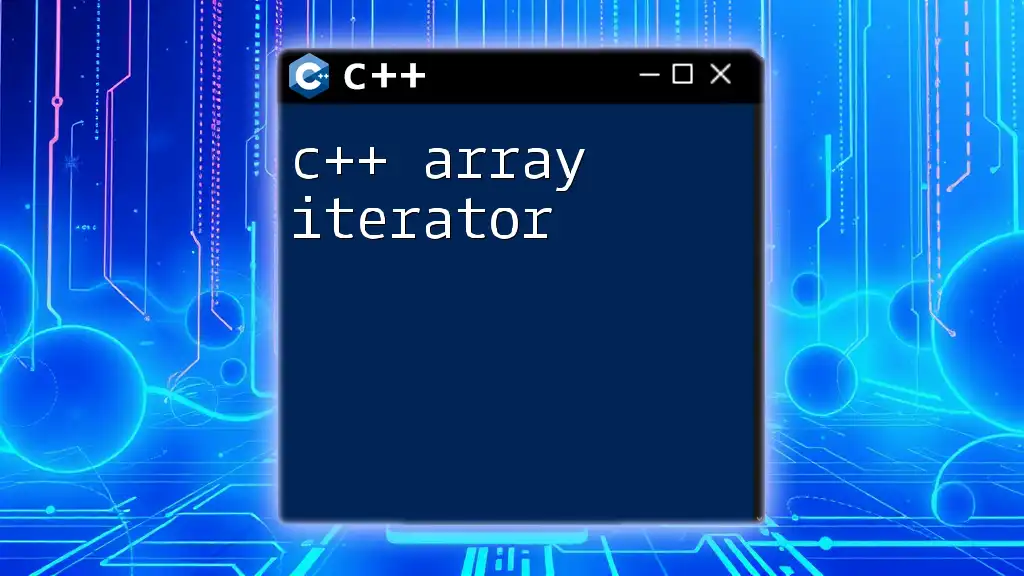
Basics of Printing C++ Arrays
How to Print an Array in C++
Printing an array in C++ requires understanding how to access its elements. Since arrays are continuous blocks of memory, you cannot simply use a print statement to display all elements. Instead, you will need to iterate over each index and print the respective value.
Using the `cout` Statement
`cout` is a predefined object in C++ used to output data to the standard output device, typically the console. To print an array with `cout`, you'll need to write a loop that accesses each element of the array.
However, it’s important to note that if you attempt to print the entire array directly:
#include <iostream>
using namespace std;
int main() {
int arr[] = {1, 2, 3, 4, 5};
cout << arr << endl; // Prints address, not elements
return 0;
}
This outputs the address of the first element rather than the contents of the array. Understanding this is key to effectively using C++ array print techniques.
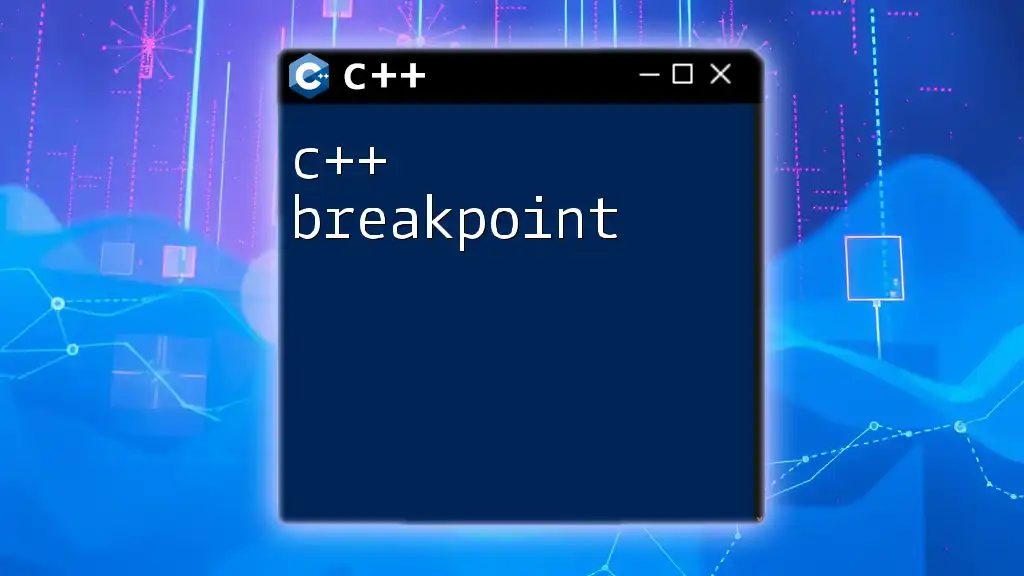
Printing Arrays in C++: Step-by-Step Guide
Looping Through the Array
To properly print array elements, you will typically use a for loop:
for (int i = 0; i < 5; i++) {
cout << arr[i] << " ";
}
In the above code snippet, the loop iterates from 0 to 4, accessing each element of the array `arr` by its index, which is what allows you to print the actual values rather than their memory addresses.
Using the Range-Based For Loop
Starting from C++11, you can utilize the range-based for loop, which significantly simplifies array iteration. This method iterates directly over the elements of the array without needing to manage indices explicitly.
Here’s how it works:
for (int value : arr) {
cout << value << " ";
}
In this example, the loop automatically retrieves each value from the array, making your code cleaner and easier to read.
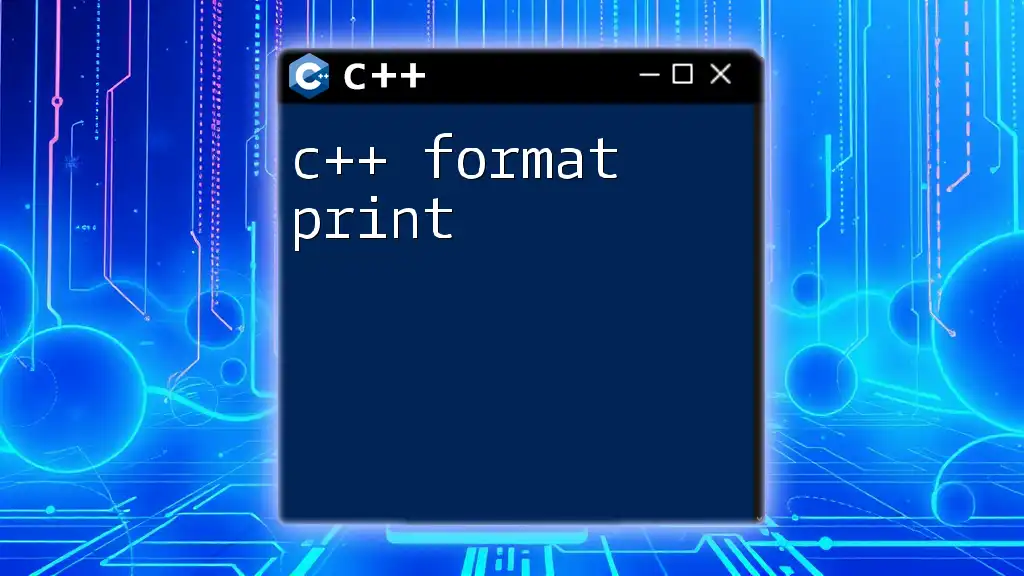
Printing Multidimensional Arrays
Understanding Multidimensional Arrays
A multidimensional array is an array of arrays. A common example is a 2D array, which you can think of as a grid or matrix. Here's how you might declare and initialize a 2D array:
int arr[3][3] = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
This creates a 3 by 3 grid, which can be visualized as three rows and three columns.
How to Print 2D Arrays
To print a 2D array, you must use nested loops. The outer loop iterates over the rows, while the inner loop iterates over the columns:
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
cout << arr[i][j] << " ";
}
cout << endl; // Print a newline after each row
}
This code will print each row of the 2D array on a new line, providing a structured format that enhances readability.
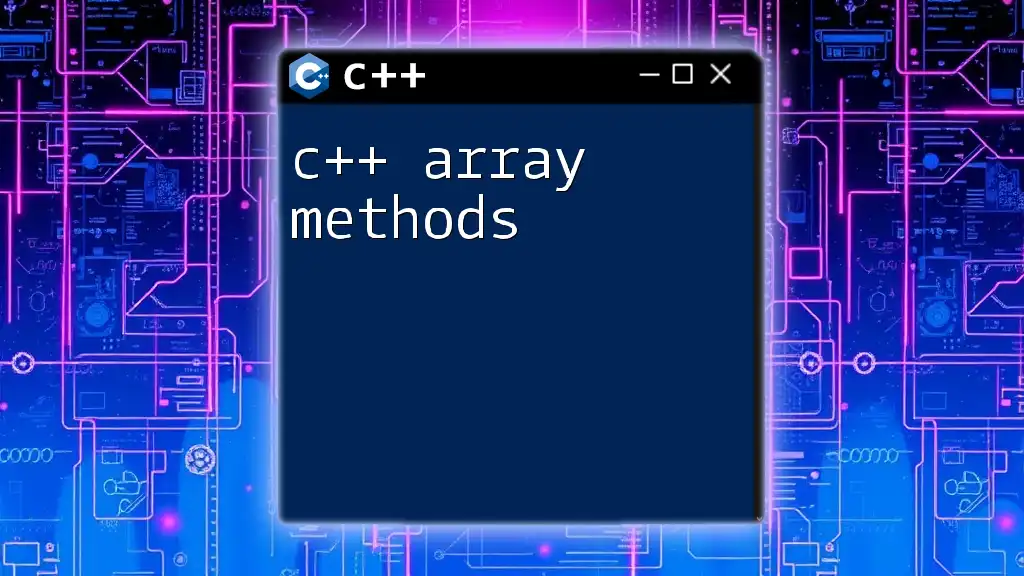
Advanced Techniques for Printing Arrays
Formatting Output
When printing arrays, particularly large ones or those compared to output from other sources, output formatting becomes crucial. Using manipulators from the `<iomanip>` library, such as `setw`, can help control the presentation of your data:
#include <iomanip>
cout << setw(5) << arr[i][j] << " ";
Here, `setw(5)` sets a field width of 5, ensuring that all printed values align neatly, enhancing the overall readability of your output.
Using Functions to Print Arrays
Creating a dedicated function to handle array printing is a good practice. It promotes code reuse and can simplify main logic significantly:
void printArray(int arr[], int size) {
for (int i = 0; i < size; i++) {
cout << arr[i] << " ";
}
cout << endl;
}
To call this function, simply pass an array along with its size to print it, which streamlines your code base and allows for easy modifications if your printing logic changes later.
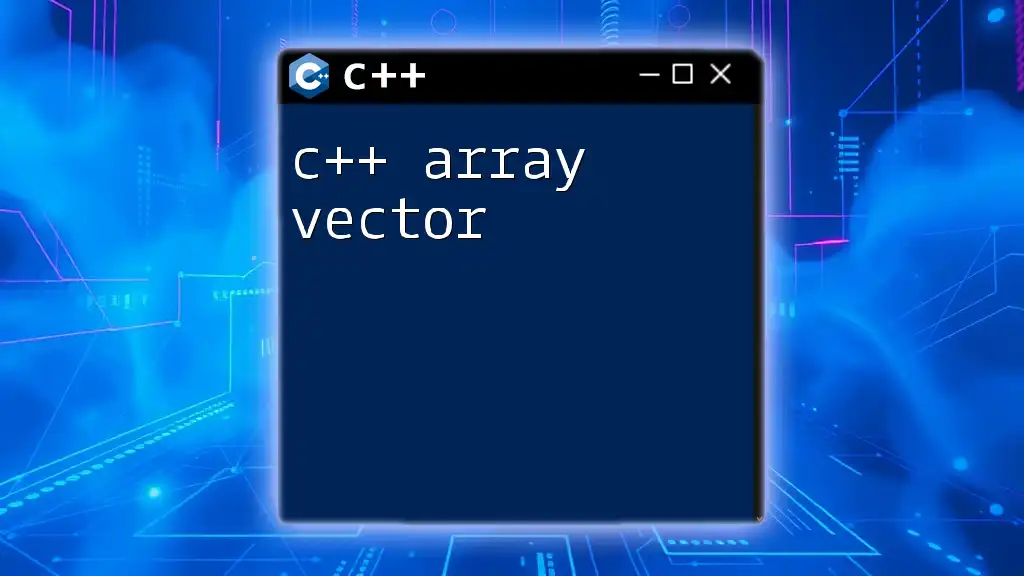
Common Mistakes and Troubleshooting
Common Issues when Printing Arrays
When printing arrays, it's vital to avoid common pitfalls, such as:
- Out-of-Bounds Access: Ensure that your loop boundaries match the actual size of the array; accessing an out-of-bounds index can lead to undefined behavior.
- Wrong Data Type: Make sure that the type of elements in your array matches what you are trying to print; for example, printing a float with an integer type format may lead to errors.
Debugging Tips
Debugging can be made simpler by following a few best practices. Use print statements to verify your array’s contents at various points in your code. Using a debugger lets you set breakpoints to inspect array values as the program executes, allowing you to easily identify where things go wrong.
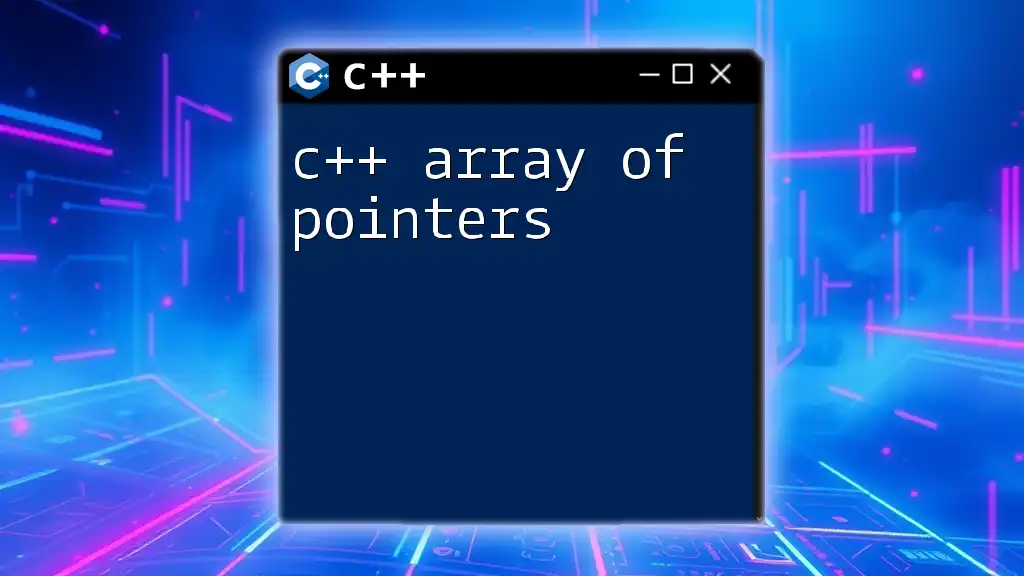
Conclusion
Mastering the concept of C++ array print is fundamental for any programmer working in C++. Efficiently printing arrays not only improves your understanding of how arrays function but also makes your code more readable and maintainable. By using loops, functions, formatting options, and being aware of common pitfalls, you can enhance your skills significantly. It’s encouraged to practice with various scenarios to solidify your knowledge and become proficient in C++ array manipulation and printing techniques.
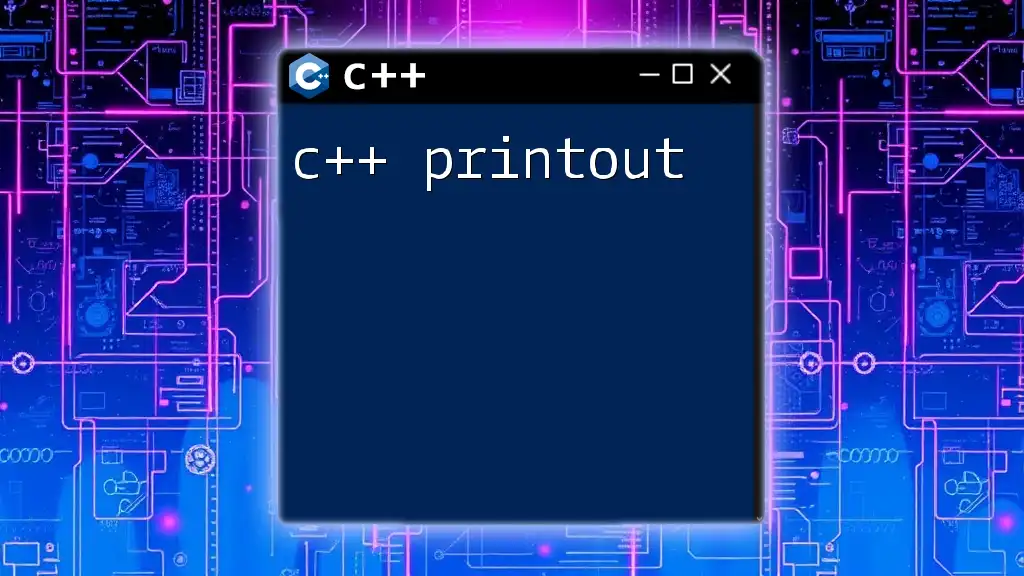
Additional Resources
To deepen your understanding, explore additional resources such as official C++ documentation, online tutorials, and community forums. Engaging with practical exercises will further solidify your confidence in handling arrays in C++.