C++ scraping involves using C++ to programmatically extract data from websites by fetching HTML content and parsing it for specific information.
Here's a simple example of using the `libcurl` library to scrape a webpage:
#include <iostream>
#include <curl/curl.h>
size_t WriteCallback(void* contents, size_t size, size_t nmemb, void* userp) {
((std::string*)userp)->append((char*)contents, size * nmemb);
return size * nmemb;
}
int main() {
CURL* curl;
CURLcode res;
std::string readBuffer;
curl = curl_easy_init();
if(curl) {
curl_easy_setopt(curl, CURLOPT_URL, "http://example.com");
curl_easy_setopt(curl, CURLOPT_WRITEFUNCTION, WriteCallback);
curl_easy_setopt(curl, CURLOPT_WRITEDATA, &readBuffer);
res = curl_easy_perform(curl);
curl_easy_cleanup(curl);
}
std::cout << readBuffer << std::endl;
return 0;
}
Understanding the Basics of Web Scraping with C++
Web scraping is the process of automating the extraction of data from websites. It allows developers to gather useful information by programmatically navigating through web pages, identifying relevant data points, and capturing them for further analysis or use. With the growing need for data collection in various fields—from market research to academic studies—skillfully utilizing C++ scraping techniques can provide a performance edge due to the language's efficiency.
Choosing to use C++ for web scraping brings several advantages. Its performance characteristics and low-level memory management capabilities allow you to handle larger datasets and perform operations faster compared to higher-level languages. This can be particularly beneficial for extensive scraping tasks that involve multiple pages or large amounts of data.
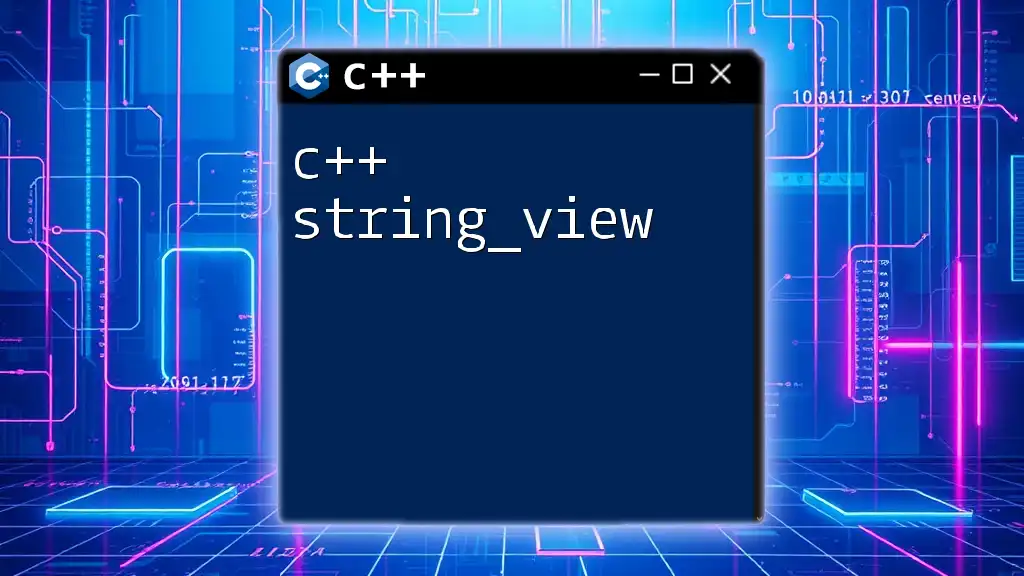
Setting Up Your C++ Environment for Web Scraping
Before diving into C++ scraping, it’s essential to set up your development environment properly.
Required Libraries
To get started, you will need to include libraries that allow HTTP requests and HTML parsing. Here are a couple of popular libraries to consider:
- libcurl: A powerful tool for making HTTP requests, allowing you to fetch web pages.
- HTML Parser: Libraries like Gumbo or HTMLcpp are useful for parsing HTML content.
Make sure to install them according to your platform's guidelines, which may include using package managers or compiling from source.
Configuring Your C++ Development Environment
When it comes to an IDE, consider using tools like Visual Studio or Code::Blocks. Make sure your C++ compiler is updated, and you have linked the necessary libraries in your project settings to avoid issues during compilation.
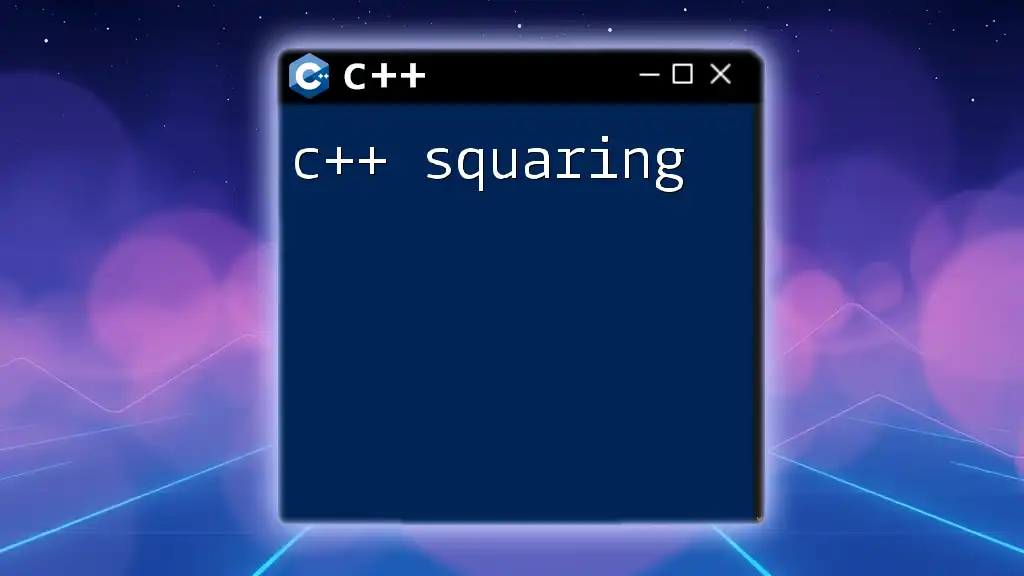
Making HTTP Requests in C++
With your environment set up, you can begin making HTTP requests to gather web content.
Using libcurl for HTTP Requests
libcurl provides a versatile API for handling various types of requests. Here’s a basic example of making a GET request in C++:
#include <curl/curl.h>
#include <iostream>
void getWebContent() {
CURL *curl;
CURLcode res;
curl = curl_easy_init();
if (curl) {
// Set the URL to fetch
curl_easy_setopt(curl, CURLOPT_URL, "http://example.com");
// Perform the request
res = curl_easy_perform(curl);
// Check for errors
if (res != CURLE_OK) {
std::cerr << "curl_easy_perform() failed: " << curl_easy_strerror(res) << std::endl;
}
// Clean up
curl_easy_cleanup(curl);
}
}
In this snippet, you initialize the `curl` library, set the target URL, and perform the request. Always check for errors to ensure successful operations.
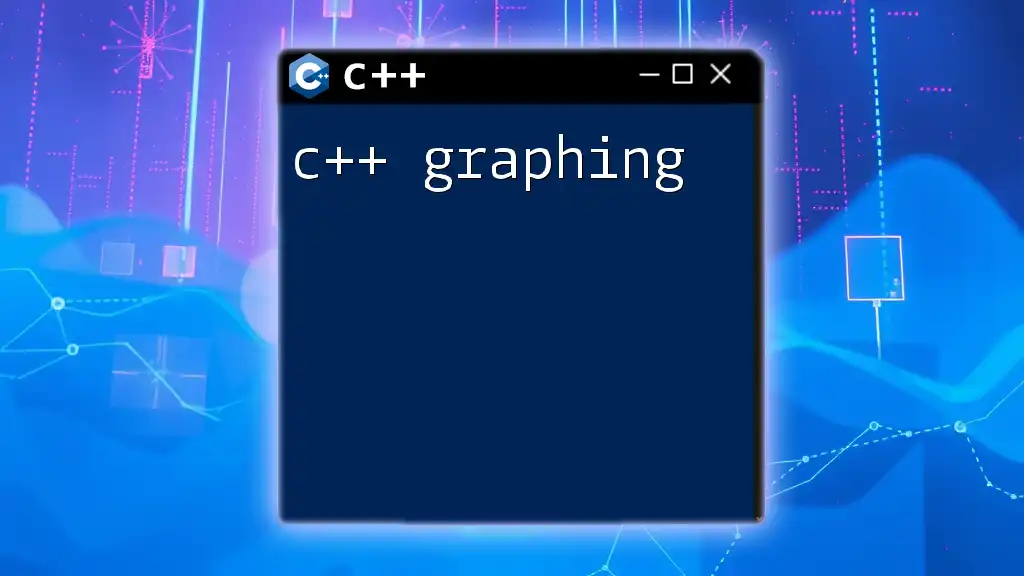
Parsing HTML Content
To extract useful information from web pages, parsing HTML is a key skill in C++ scraping.
Choosing the Right Parsing Library
Selecting a parsing library is critical for efficiently navigating the Document Object Model (DOM) of HTML. Libraries such as Gumbo provide a C API for parsing HTML, whereas HTMLcpp offers a more straightforward C++ interface.
Extracting Data
Once you have fetched the HTML content, you can parse it to retrieve the data you need. Here’s a basic approach:
// Pseudocode for parsing HTML content
std::string htmlContent = getHTMLContent(); // Assume this function retrieves the HTML.
// Use your chosen library to extract specific elements.
You would replace the pseudocode with actual parsing commands specific to the library you're utilizing. This may involve targeting elements by their tag names, classes, or IDs.
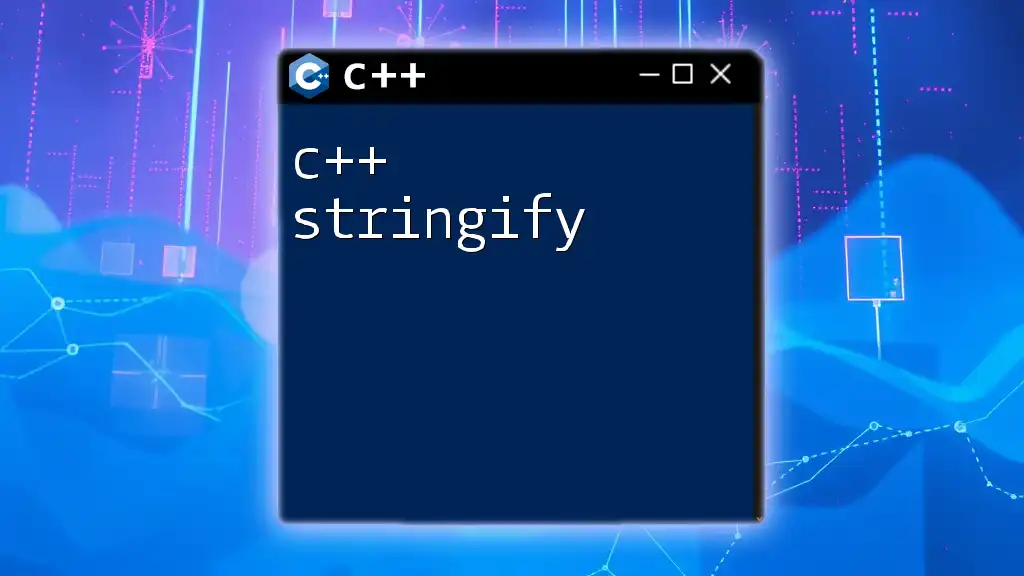
Handling Dynamic Content
Many modern websites rely on JavaScript to render content dynamically. This presents challenges for scrapers that typically handle static HTML.
Understanding JavaScript-Rendered Content
When scraping websites that employ JavaScript, the data you seek may not be present in the initial HTML returned by a GET request. Instead, the content may load after additional scripts run in the client’s browser.
Using C++ with Headless Browsers
A headless browser allows you to programmatically control a web browser without a graphical user interface. Using tools like Selenium with C++ bindings enables you to handle pages that change based on user interactions. This technique can open up many scraping opportunities.
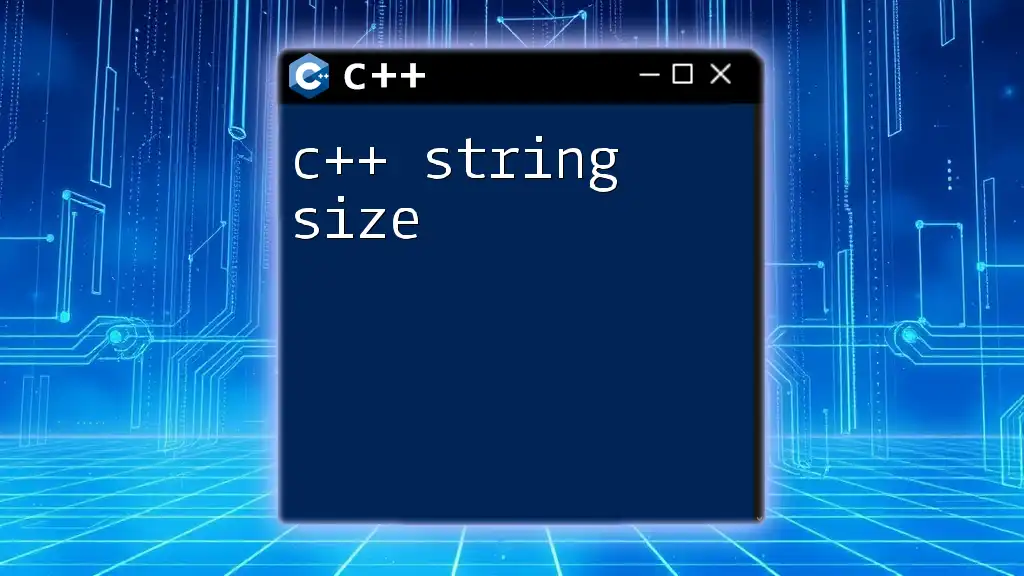
Best Practices for C++ Web Scraping
Respecting Robots.txt and Legal Considerations
Always check the robots.txt file of the website before scraping to understand what is allowed. This step helps you avoid unethical scraping practices and respect the rules established by website owners.
Efficient Data Storage Strategies
When managing your scraped data, choose appropriate file formats for storing it efficiently. Common options include JSON, CSV, or databases like SQLite. Here’s an example of how to save scraped data to a CSV file:
std::ofstream outputFile("data.csv");
outputFile << "Column1, Column2\n"; // Add headers
outputFile << "Data1, Data2\n"; // Add scraped data in rows
outputFile.close();
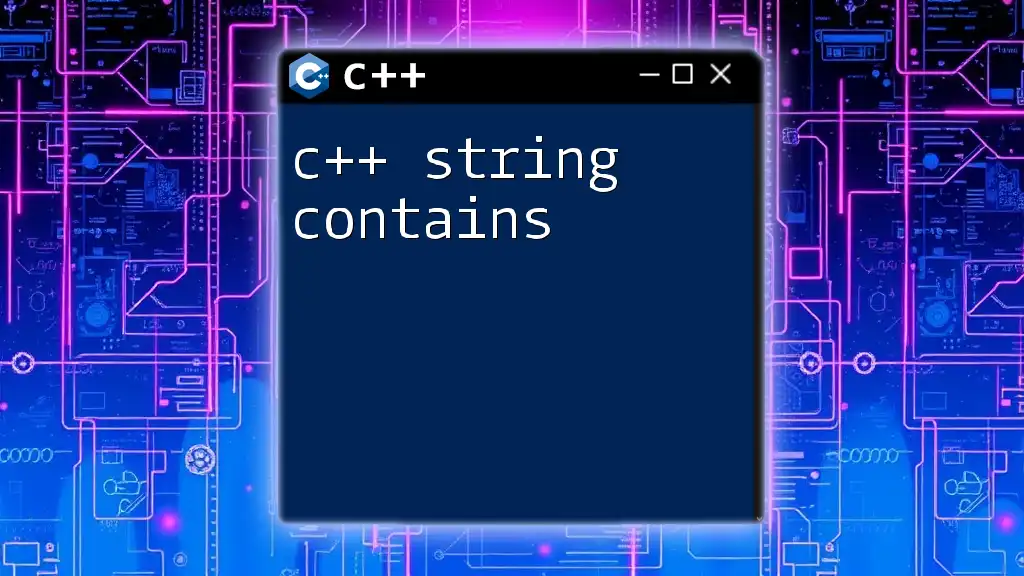
Common Challenges in Web Scraping with C++
Handling Rate Limiting
Many websites implement mechanisms to limit the frequency of requests. To avoid being blocked, consider implementing pauses between requests and limiting the number of concurrent threads doing the scraping.
Dealing with Captchas and Security Measures
Some sites employ Captchas or other security features to prevent automated access. While it’s essential to handle these ethically, note that bypassing them can lead to legal ramifications and potential bans from the website.
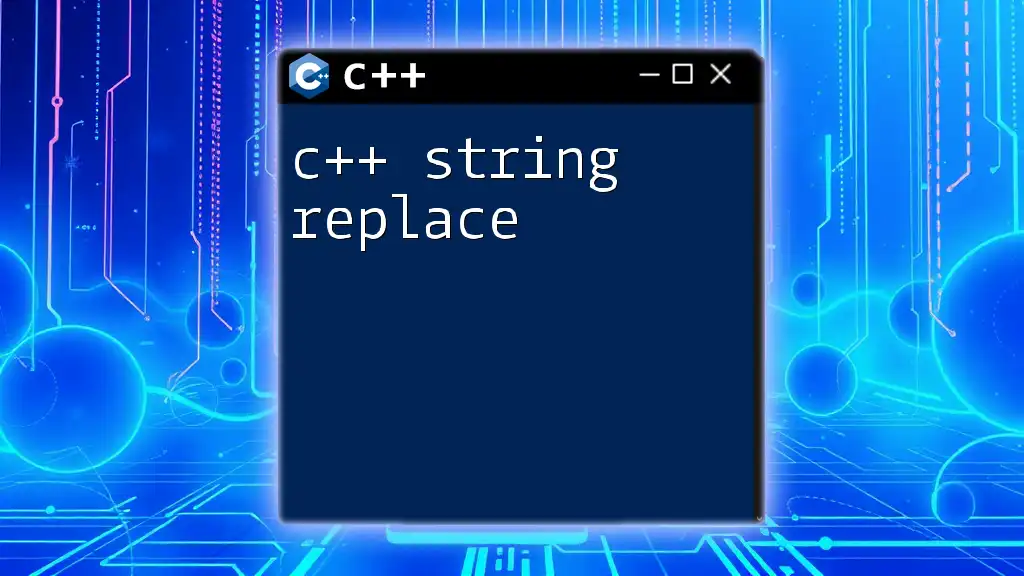
Real-world Examples of C++ Web Scraping
Case Study: Scraping Product Prices
Let’s consider a small project where you scrape product prices from an online store. The process involves identifying the product links, fetching their HTML pages, and extracting price data successfully using the techniques covered earlier.
Visualization of Scraped Data
Once you have the data collected, consider visualizing it using libraries that support graphical representations. Visualization can enhance the understanding of your data, offering insights at a glance.
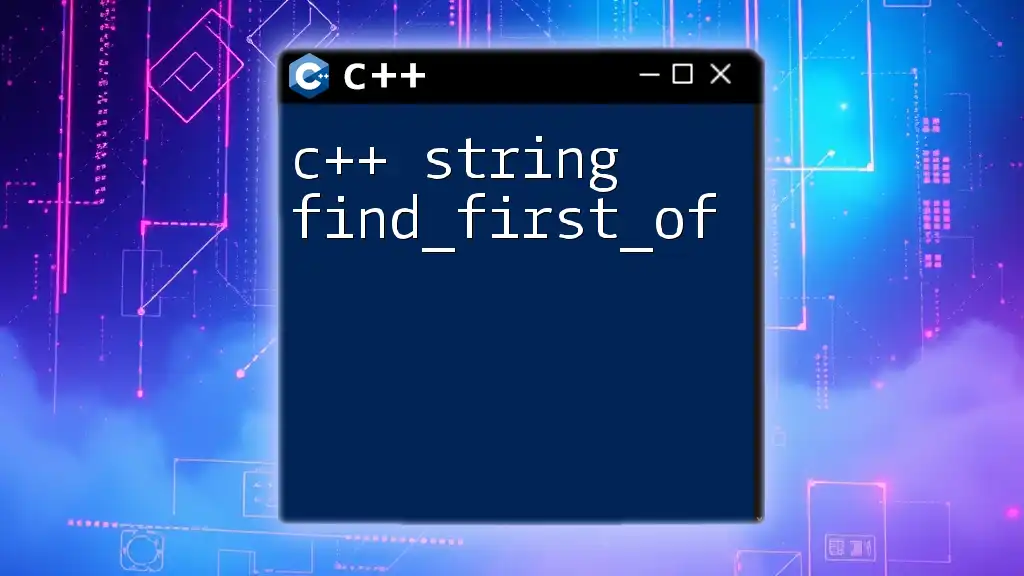
Conclusion
In this article, we have explored the fundamentals of C++ scraping. From setting up your environment and making HTTP requests to parsing data and handling challenges like dynamic content, we have covered essential techniques for mobile and desktop scraping projects. The skills you'll develop through C++ web scraping will not only enhance your ability to gather data but also contribute to your overall programming expertise.
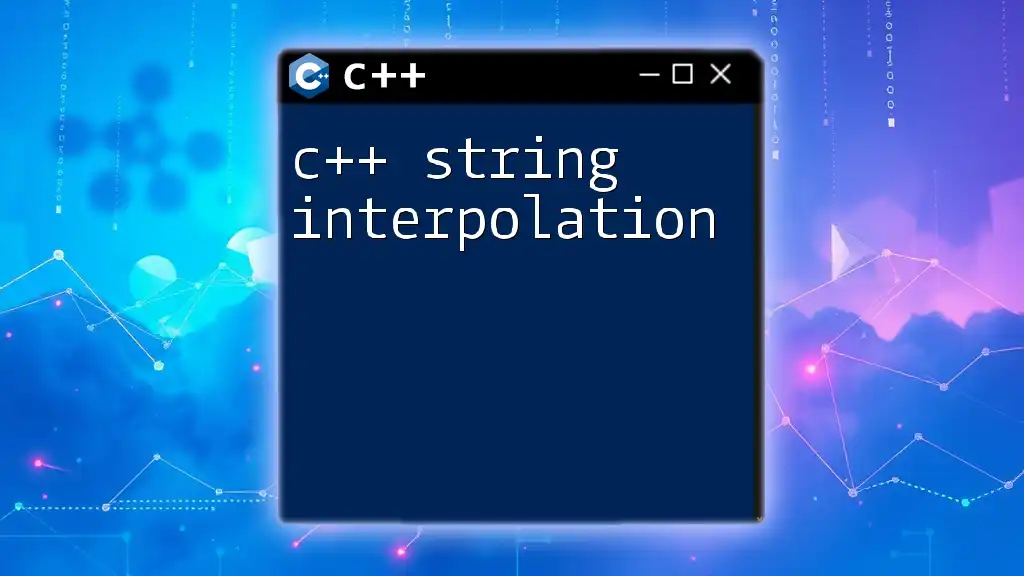
Additional Resources
To further enhance your understanding and skills in C++ web scraping, consider exploring books dedicated to C++, online courses on web scraping methodologies, and community forums where you can connect with fellow C++ developers. These resources will provide you with additional insights and support to continue your journey in web scraping.