A C++ spinlock is a simple locking mechanism that repeatedly checks and waits until a lock becomes available, making it efficient for short critical sections in multithreaded programming.
#include <atomic>
class Spinlock {
std::atomic_flag flag = ATOMIC_FLAG_INIT;
public:
void lock() {
while (flag.test_and_set(std::memory_order_acquire)) {
// busy-wait (spin)
}
}
void unlock() {
flag.clear(std::memory_order_release);
}
};
// Usage
Spinlock spinlock;
void thread_function() {
spinlock.lock();
// critical section
spinlock.unlock();
}
Understanding Spinlocks
What is a Spinlock?
A C++ spinlock is a type of synchronization primitive that operates by repeatedly checking (or "spinning") on a condition until it can successfully acquire a lock. This approach is particularly useful in low-contention scenarios where locking overhead needs to be minimized. Spinlocks allow a thread to keep checking for the lock's availability, making them suitable for cases where threads are expected to hold locks for only a brief period.
While spinlocks can provide a performance boost in certain conditions, they should be used judiciously. They are less effective in high-contention situations where the lock is heavily contested by multiple threads, as they can lead to excessive CPU usage.
Key Characteristics of Spinlocks
Spinlocks have several key characteristics that distinguish them from other locking mechanisms:
-
Busy-waiting mechanics: Unlike traditional locks that may put a thread to sleep when the lock can't be acquired, spinlocks cause the thread to remain in a "spinning" state, actively using CPU cycles.
-
Lock granularity: Spinlocks can be finer in granularity compared to mutex locks. This quality means they may be more efficient for shorter critical sections, reducing the overhead of context switching.
-
Performance implications: The performance of spinlocks is heavily influenced by contention levels. When contention is low, spinlocks can outperform other locking mechanisms. However, the performance quickly deteriorates as contention increases, leading to starvation and wasted CPU resources.
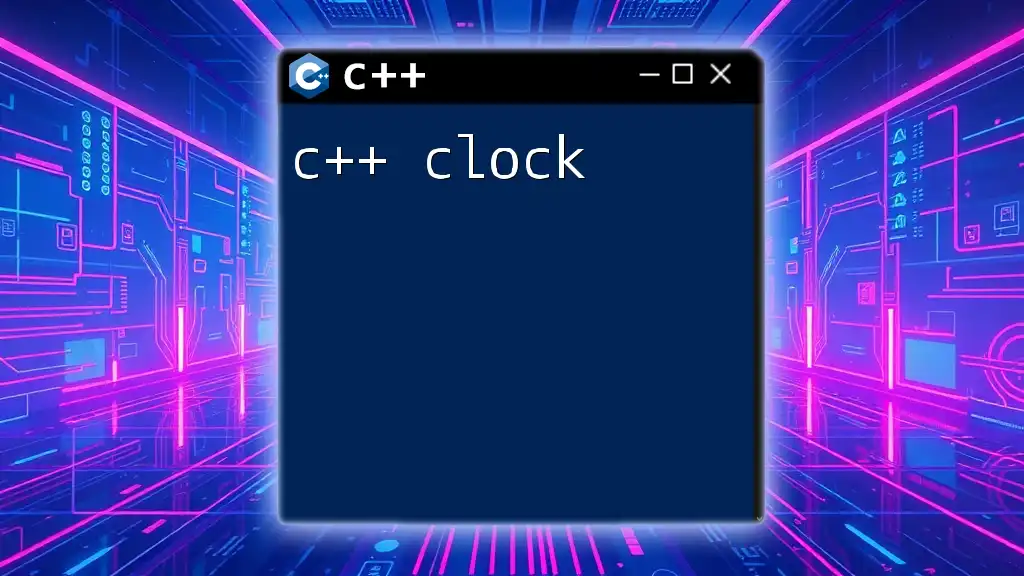
When to Use Spinlocks
Ideal Scenarios for Spinlocks
Spinlocks shine in specific scenarios:
-
Low contention situations: When few threads contend for the same lock, spinlocks can be more efficient than blocking locks due to the reduced overhead of context switching.
-
Short critical sections: If a lock is held for a very brief period, the active spinning can be faster than putting the thread to sleep and waking it up later.
-
Real-time systems considerations: In real-time systems where predictability is crucial, spinlocks may provide the needed performance without the unpredictability of context switches.
When Not to Use Spinlocks
Conversely, there are clear indications when spinlocks should be avoided:
-
High contention scenarios: If many threads compete for a lock simultaneously, the spinning will waste CPU cycles and degrade the overall performance of the application.
-
Long wait times: If the expected wait time to acquire a lock is significant, spinlocks can lead to significant CPU resource wastage, making traditional locking mechanisms a better choice.
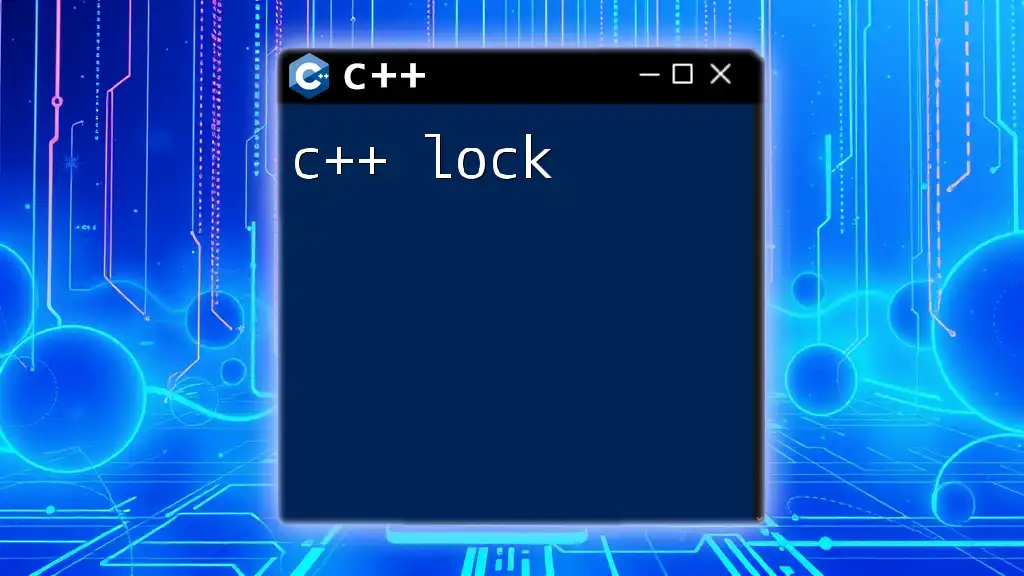
Implementing a Spinlock in C++
Basic Spinlock Implementation
Implementing a basic spinlock in C++ can be achieved with the help of atomics. Below is a simple spinlock implementation:
class Spinlock {
private:
std::atomic_flag flag = ATOMIC_FLAG_INIT;
public:
void lock() {
while (flag.test_and_set(std::memory_order_acquire)); // spin
}
void unlock() {
flag.clear(std::memory_order_release);
}
};
In this implementation, the `lock()` function uses `test_and_set`, which atomically sets the flag and returns its old value. If the old value is true (indicating the lock is already held), the thread continues to spin. The `unlock()` function clears the flag, effectively releasing the lock.
Enhancements to Basic Spinlock
While the basic implementation serves its purpose, performance can be improved with a backoff strategy to alleviate contention during high-load scenarios. Below is an enhanced implementation:
#include <thread>
#include <chrono>
class EnhancedSpinlock {
private:
std::atomic_flag flag = ATOMIC_FLAG_INIT;
public:
void lock() {
int attempts = 0;
while (flag.test_and_set(std::memory_order_acquire)) {
attempts++;
std::this_thread::sleep_for(std::chrono::microseconds(1 << attempts)); // exponential backoff
}
}
void unlock() {
flag.clear(std::memory_order_release);
}
};
In this enhanced version, the `lock()` method incorporates an exponential backoff strategy by increasing the wait time after each failed attempt to acquire the lock. This behavior reduces contention and increases the likelihood that other threads can acquire the lock successfully.
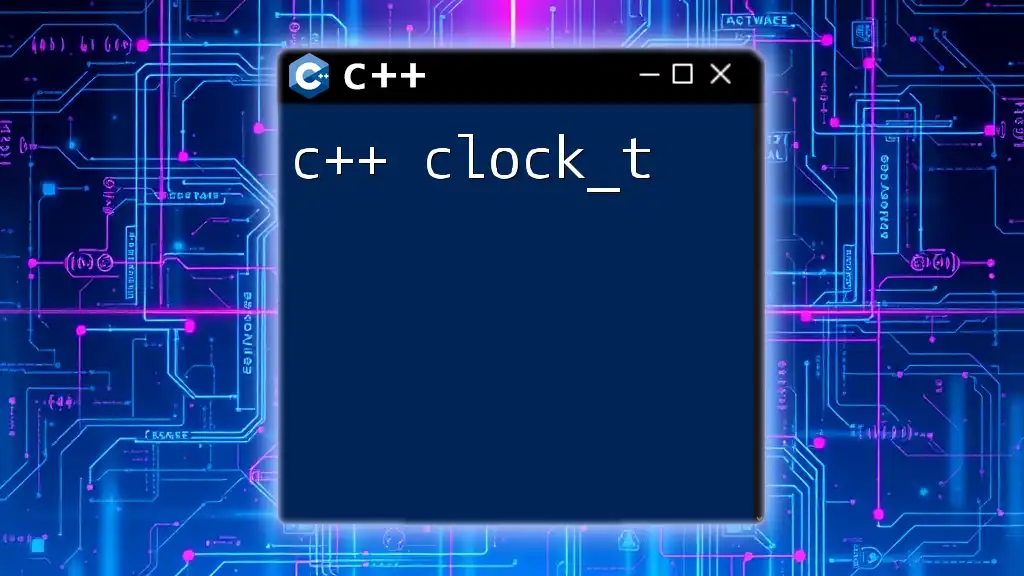
Spinlock in Multithreaded Environments
Using Spinlocks with Threads
Using spinlocks allows multiple threads to perform operations safely without stepping on each other's toes. Here’s an example involving multiple threads incrementing a counter:
#include <iostream>
#include <thread>
#include <vector>
Spinlock my_lock;
int counter = 0;
void increment() {
my_lock.lock();
++counter;
my_lock.unlock();
}
int main() {
std::vector<std::thread> threads;
for (int i = 0; i < 10; ++i) {
threads.push_back(std::thread(increment));
}
for (auto& th : threads) {
th.join();
}
std::cout << "Final count: " << counter << std::endl;
return 0;
}
In this example, ten threads increment a shared counter, demonstrating how spinlocks allow safe concurrent access. The `my_lock` object ensures that only one thread can access the counter at any given time.
Performance Analysis
Measuring the performance of spinlocks is essential, as it can vary significantly depending on system architecture and workload. To effectively analyze performance, consider:
-
Comparative benchmarks between spinlocks and mutexes: Create scenarios with varying thread counts and observe the time taken to complete tasks, comparing the results for both locking strategies.
-
Context switching overhead: Use profiling tools to measure CPU usage and context switching overhead to determine if spinlocks are saving time or creating bottlenecks.
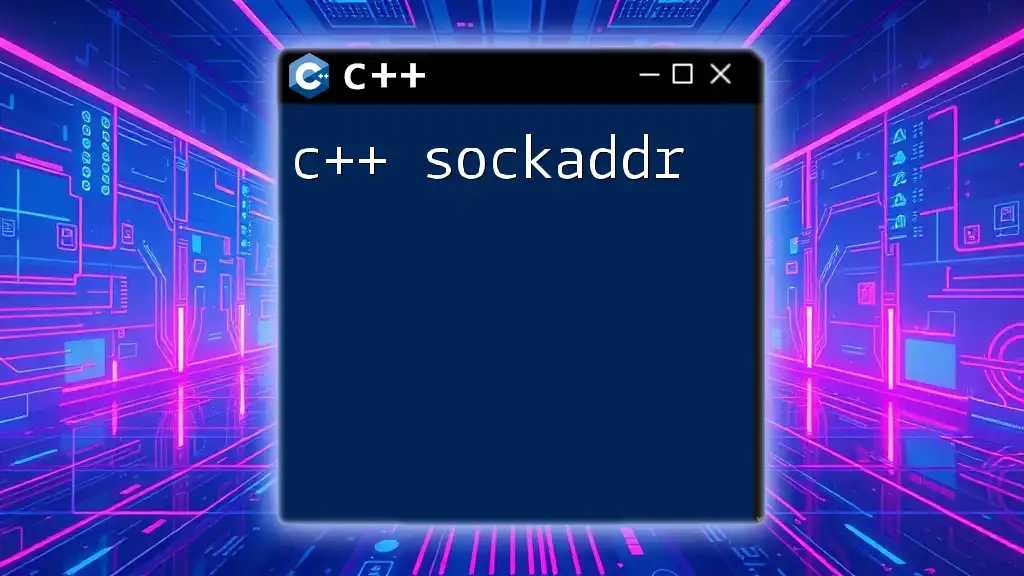
Best Practices for Using Spinlocks
Designing for Low Contention
To maximize the effectiveness of spinlocks, design your system to minimize contention:
-
Partition workloads: Distributing tasks across multiple locks can help reduce the chance of multiple threads contending for the same lock.
-
Keep critical sections short: Minimize the time spent within a locked section to lower the impact on overall performance.
Testing Spinlocks
Thorough testing of spinlocks is critical. Implement various concurrent testing techniques to simulate high-load scenarios, ensuring your spinlock implementation behaves as expected without introducing deadlocks or excessive CPU usage.
Common Pitfalls to Avoid
When working with spinlocks, common pitfalls to avoid include:
-
Overusing spinlocks: Recognize when a simple mutex or other synchronization primitive would be more effective; adaptability is key.
-
Neglecting performance measurement: Continuously measure performance in realistic environments to ensure that your locking strategy maintains its intended efficiency.
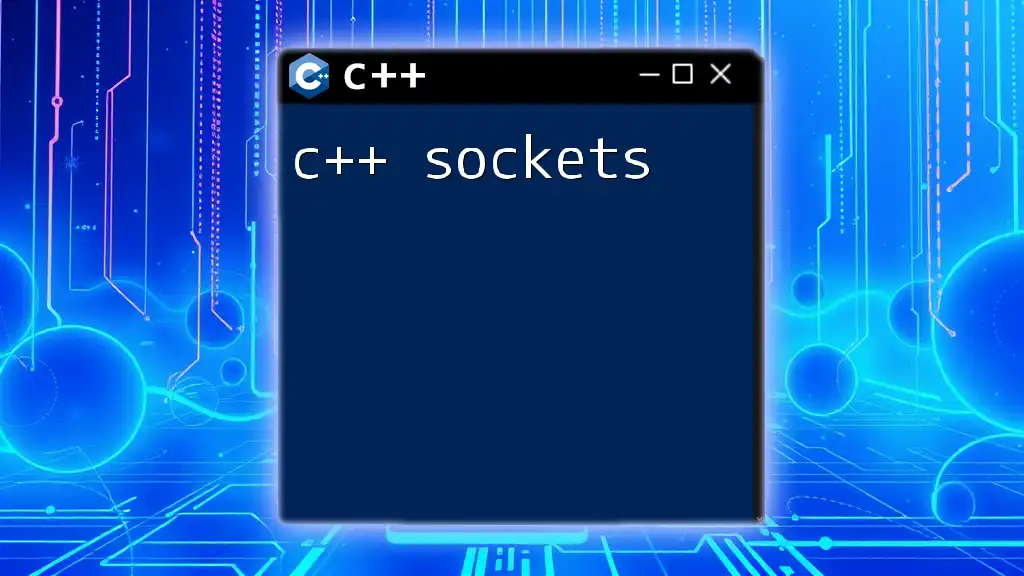
Conclusion
C++ spinlocks can be a powerful tool in your concurrency toolkit when used appropriately. By understanding their characteristics, ideal use cases, and performance implications, developers can make informed decisions about when to use them and how to optimize their implementations. Experimentation and hands-on experience will lead to a deeper understanding of the balance between simplicity and performance, ultimately improving your applications' efficiency.
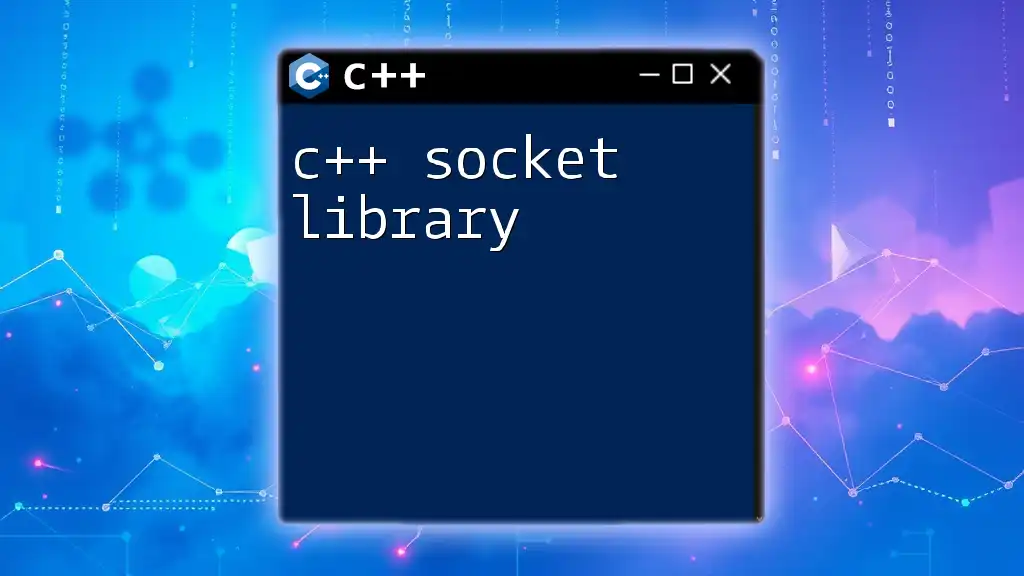
Additional Resources
Recommended Reading
To deepen your knowledge of C++ concurrency and synchronization, consider exploring:
-
Books: Authoritative texts covering multithreading in C++, focusing on practical applications and advanced locking techniques.
-
Online courses: Structured tutorials that provide clarity on concurrency concepts and hands-on exercises.
Community and Support
Engage with the broader C++ development community through forums and collaborative projects, sharing insights on spinlocks and other concurrency mechanisms. Participation in open-source initiatives can provide additional learning opportunities and enhance your understanding of practical implementations.