A C++ `lock_guard` is a simple RAII-style mechanism that provides exclusive ownership of a mutex, ensuring that the mutex is locked during the lifetime of the `lock_guard` object and automatically released when it goes out of scope.
#include <iostream>
#include <mutex>
#include <thread>
std::mutex mtx;
void printSafe(int id) {
std::lock_guard<std::mutex> lock(mtx); // Lock the mutex
std::cout << "Thread " << id << " is printing safely!" << std::endl;
}
int main() {
std::thread t1(printSafe, 1);
std::thread t2(printSafe, 2);
t1.join();
t2.join();
return 0;
}
Understanding Concurrency in C++
Concurrency in programming refers to the ability of a system to handle multiple tasks simultaneously, which can significantly improve the performance and responsiveness of applications. It’s often confused with parallelism, which signifies actual simultaneous execution. Understanding concurrency is crucial in C++, especially when developing multi-threaded applications, as improper handling can lead to issues such as race conditions, where multiple threads attempt to modify shared data at the same time, potentially leading to unpredictable behavior.
Challenges in Multi-Threading
In multi-threaded applications, synchronization is critical. Without effective management, you can encounter:
- Race Conditions: Occurs when the outcome of a computation depends on the sequence or timing of uncontrollable events, often resulting in incorrect data being processed.
- Deadlocks: A state where two or more threads are blocked forever, waiting on each other to release resources, leading to a standstill in program execution.
- Starvation: A condition where a thread is perpetually denied necessary resources for execution, hence it cannot proceed.
Given these challenges, effective synchronization mechanisms are imperative.
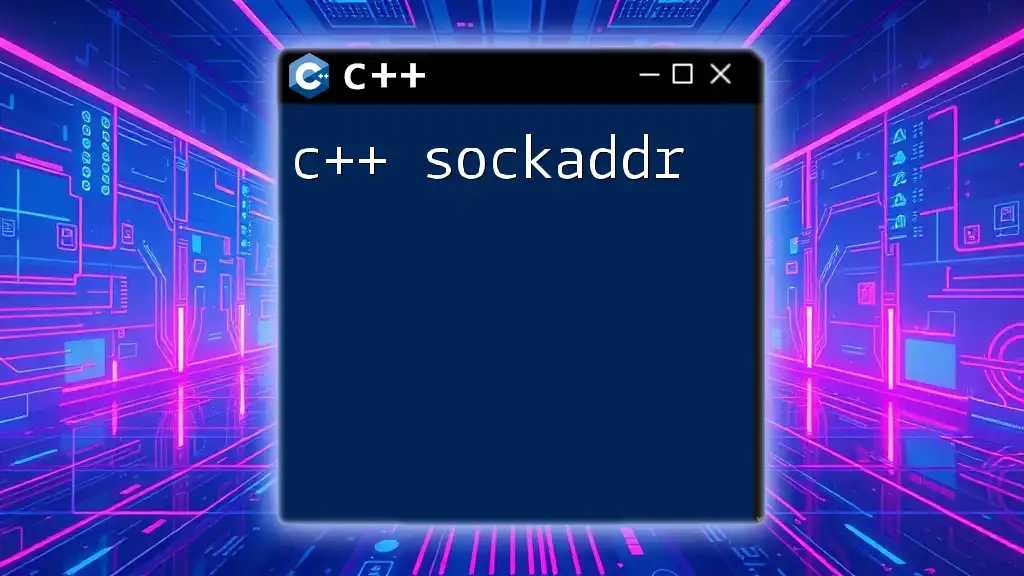
Introduction to `lock_guard`
The `lock_guard` is a simple yet powerful tool in C++ for managing mutex locks. It ensures that a mutex is locked when the guard is created and automatically unlocked when it goes out of scope, adhering to the RAII (Resource Acquisition Is Initialization) principle.
When to Use `lock_guard`
Using `lock_guard` is particularly useful:
- When you want simplicity: It provides a straightforward mechanism for locking without the boilerplate code associated with manual locking and unlocking.
- For scopes of execution: When the locks are only needed for a specific block of code, as it automatically releases the lock when exiting that scope.
Advantages of Automatic Resource Management
The primary advantage of using `lock_guard` is that it simplifies code by ensuring that locks are released even if an exception occurs within the locked scope. This reduces the chances of deadlock scenarios and promotes safer coding practices.
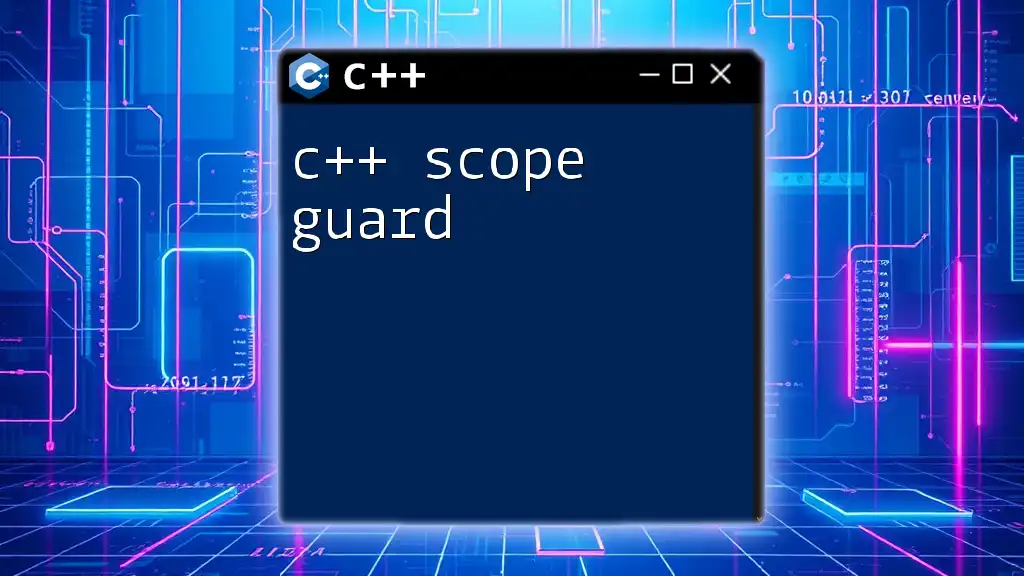
How `lock_guard` Works
Underlying Mechanism
`lock_guard` implements the RAII paradigm, ensuring that resources are acquired and released automatically. When a `lock_guard` object is created, it locks a given mutex. Upon destruction, which occurs when the `lock_guard` goes out of scope, the mutex is released. This automatic management avoids common pitfalls associated with manual mutex handling.
Syntax of `lock_guard`
Creating a `lock_guard` is straightforward and requires just a few lines of code. Here’s a simple example demonstrating the syntax:
#include <iostream>
#include <mutex>
std::mutex mtx;
void printTask() {
// Acquire lock_guard
std::lock_guard<std::mutex> guard(mtx);
std::cout << "Thread-safe message!" << std::endl;
}
In this example, `std::lock_guard<std::mutex> guard(mtx);` locks the mutex `mtx`. The lock is held for the duration of the function `printTask`.
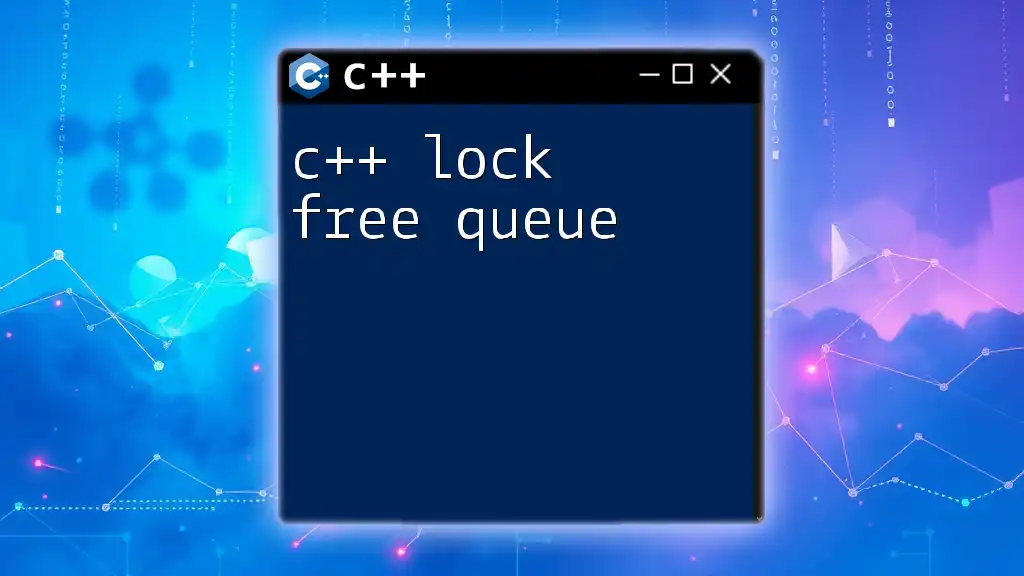
Practical Usage of `lock_guard`
Basic Example
Here’s a basic example of using `lock_guard` within a multi-threaded context:
#include <iostream>
#include <thread>
#include <mutex>
std::mutex mtx;
void printHello() {
std::lock_guard<std::mutex> guard(mtx);
std::cout << "Hello from thread!" << std::endl;
}
int main() {
std::thread t1(printHello);
std::thread t2(printHello);
t1.join();
t2.join();
return 0;
}
In this example, two threads are created, and each attempts to execute `printHello`, which is synchronized by the `lock_guard`. Consequently, no two threads can execute this function concurrently, preventing data corruption in shared resources.
Advanced Example
A more complex scenario can demonstrate `lock_guard` with shared resources:
#include <iostream>
#include <thread>
#include <mutex>
#include <vector>
std::mutex mtx;
int counter = 0;
void incrementCounter() {
std::lock_guard<std::mutex> guard(mtx);
++counter;
std::cout << "Counter: " << counter << std::endl;
}
int main() {
const int numThreads = 10;
std::vector<std::thread> threads;
for (int i = 0; i < numThreads; ++i) {
threads.emplace_back(incrementCounter);
}
for (auto& thread : threads) {
thread.join();
}
return 0;
}
This snippet creates ten threads, each incrementing a shared counter. Thanks to `lock_guard`, access to `counter` is synchronized, ensuring that each increment is thread-safe and outputs the correct counter value.
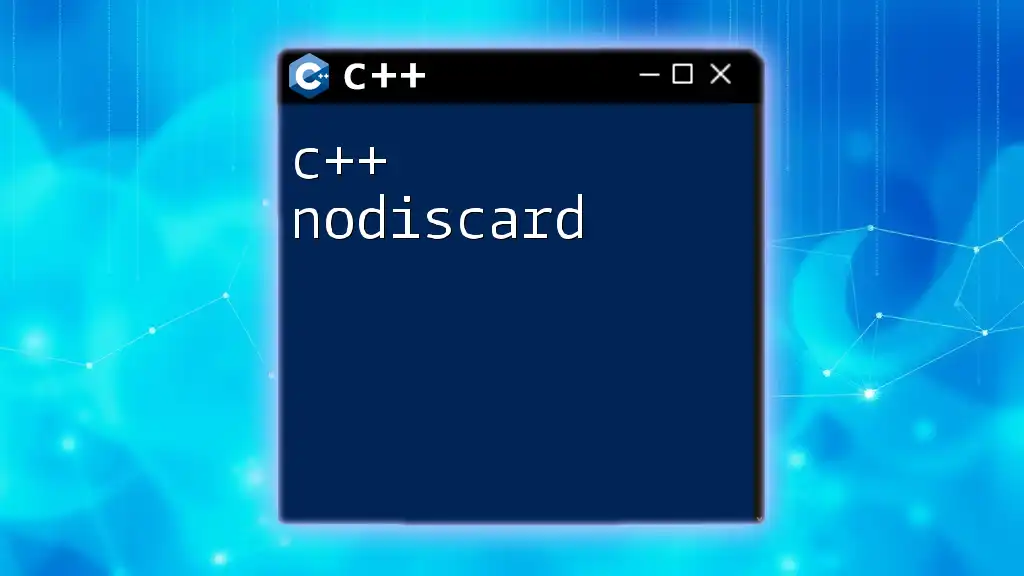
Benefits of Using `lock_guard`
Simple and Easy to Use
One of the most significant advantages of `lock_guard` is its simplicity. The boilerplate code to acquire and release locks is significantly reduced, allowing developers to focus on the core logic of their applications. This simplicity can lead to heightened productivity and fewer bugs during development.
Exception Safety
`lock_guard` inherently ensures that mutexes are released, even if an exception exits the current context unexpectedly. Consider the following example, illustrating exception safety:
#include <iostream>
#include <mutex>
#include <stdexcept>
std::mutex mtx;
void safeFunction() {
std::lock_guard<std::mutex> guard(mtx);
throw std::runtime_error("An error occurred!");
}
int main() {
try {
safeFunction();
} catch (const std::exception& e) {
std::cout << e.what() << std::endl;
}
// mutex is automatically released here
return 0;
}
In this case, throwing an exception from `safeFunction` does not lead to a deadlock. The `mutex` is released immediately once the `lock_guard` instance (`guard`) goes out of scope.
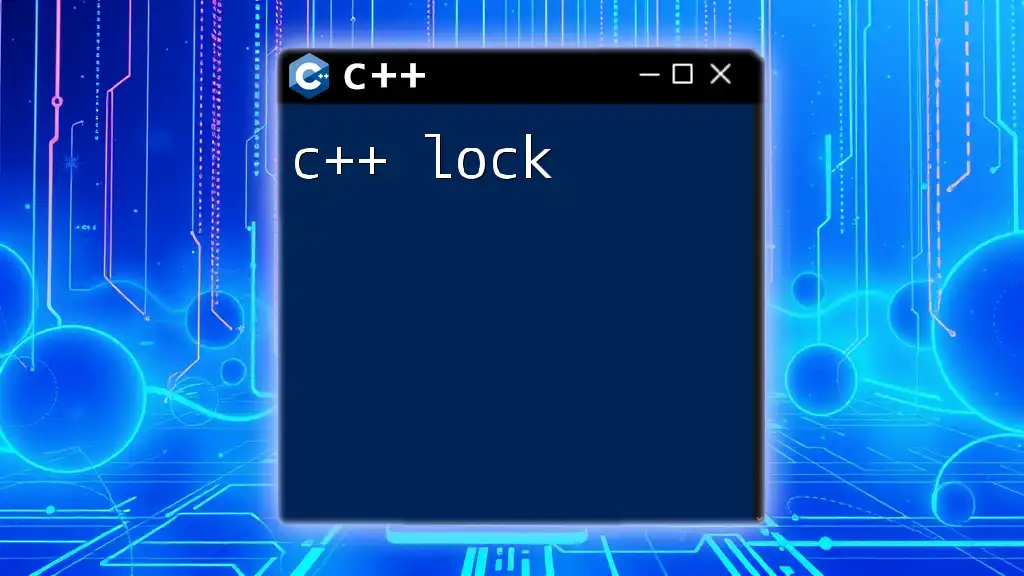
Limitations of `lock_guard`
No Manual Unlocking
While `lock_guard` offers straightforward automatic management, it lacks the flexibility for manual unlocking, which might be necessary in some cases. If you need to unlock a mutex before the scope ends, you will have to use `std::unique_lock`, which offers more control.
Scoped Locking
The locking scope of `lock_guard` can limit its usability in complex scenarios where locks might need to span multiple functions or require specific conditional unlocking. This limitation should be considered during architectural planning in concurrent applications.
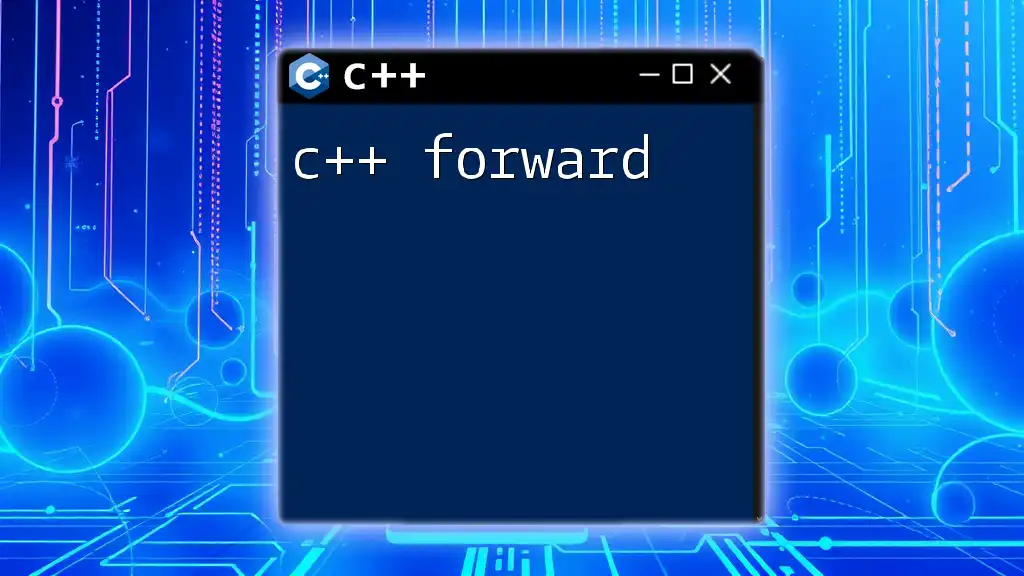
Summary
In summary, `c++ lock guard` is a powerful and simple tool for managing mutex locks in multi-threaded programming. It greatly simplifies the complexity associated with manual lock management, provides excellent exception safety, and adheres to the RAII principle. Understanding when and how to use `lock_guard` can significantly enhance the robustness and safety of concurrent applications.
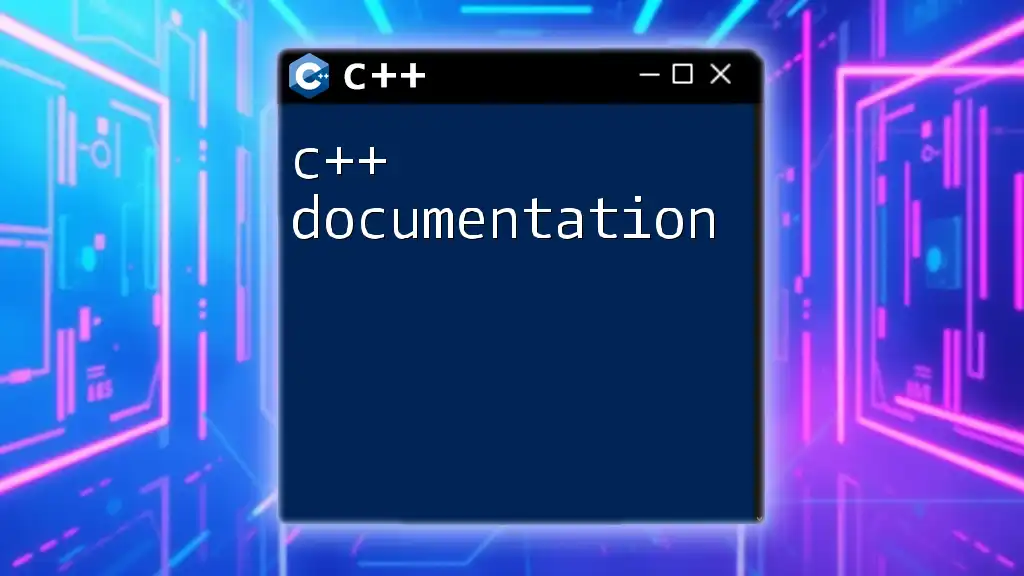
Conclusion
Utilizing `lock_guard` in your C++ applications fosters safer and more efficient concurrency management. We encourage you to practice using `lock_guard` and delve into additional concurrency topics to further enhance your programming toolkit.
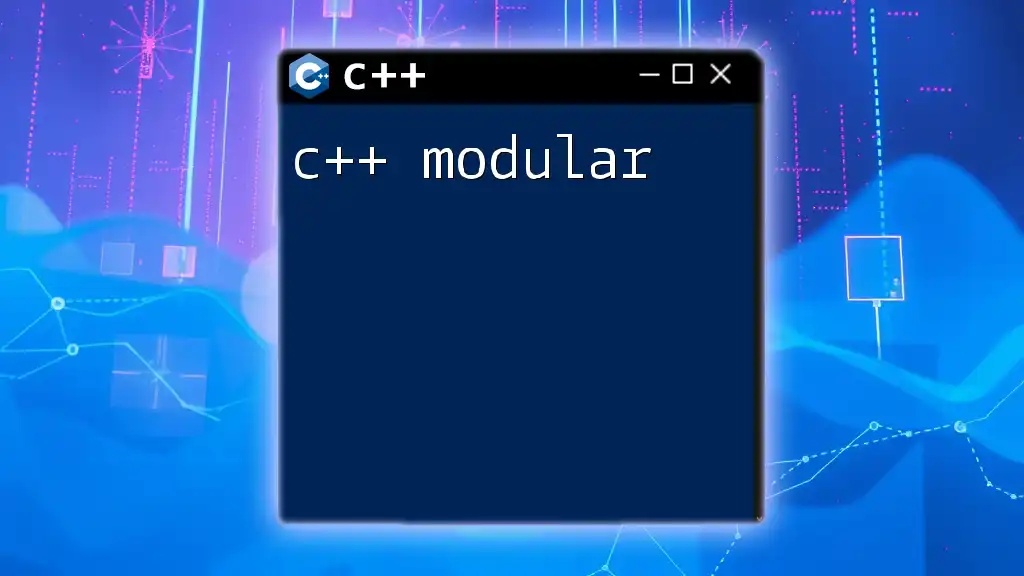
Additional Resources
For those seeking further knowledge, numerous materials are available. We recommend checking out C++ standard documentation, books focused on concurrent programming, and online platforms offering tutorials and discussions about multi-threaded development practices in C++.