C++ curl is a powerful library used to send HTTP requests and handle data transfers, making it easier to integrate web services into C++ applications.
Here's a simple example of using C++ curl to perform a GET request:
#include <curl/curl.h>
#include <iostream>
size_t WriteCallback(void* contents, size_t size, size_t nmemb, void* userp) {
((std::string*)userp)->append((char*)contents, size * nmemb);
return size * nmemb;
}
int main() {
CURL* curl;
CURLcode res;
std::string readBuffer;
curl = curl_easy_init();
if(curl) {
curl_easy_setopt(curl, CURLOPT_URL, "http://example.com");
curl_easy_setopt(curl, CURLOPT_WRITEFUNCTION, WriteCallback);
curl_easy_setopt(curl, CURLOPT_WRITEDATA, &readBuffer);
res = curl_easy_perform(curl);
curl_easy_cleanup(curl);
std::cout << readBuffer << std::endl;
}
return 0;
}
What is cURL?
cURL is a powerful command-line tool and library used for transferring data with URLs. It supports multiple protocols, including HTTP, HTTPS, FTP, and more, making it incredibly versatile for developers and system administrators alike. The significance of cURL grows as it allows seamless data transfer over networks, acting as a bridge between various applications and web services.
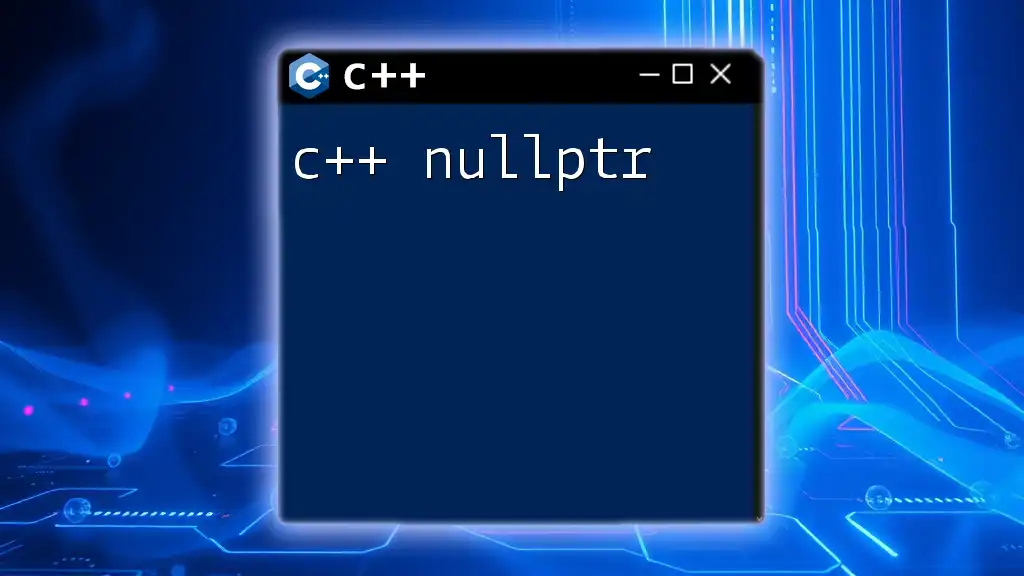
Brief Overview of libcurl and curl c++
libcurl is the underlying library that powers cURL. It provides developers with a robust API for integrating network communication into their applications. When we refer to curl c++, we emphasize the use of libcurl specifically within the C++ programming language. This combination allows C++ developers to efficiently handle network requests using an API that is both comprehensive and relatively easy to use. The benefits of using libcurl in C++ applications include:
- Access to a wide range of protocols.
- Robust error handling and debugging options.
- Support for modern features such as HTTPS and cookie handling.
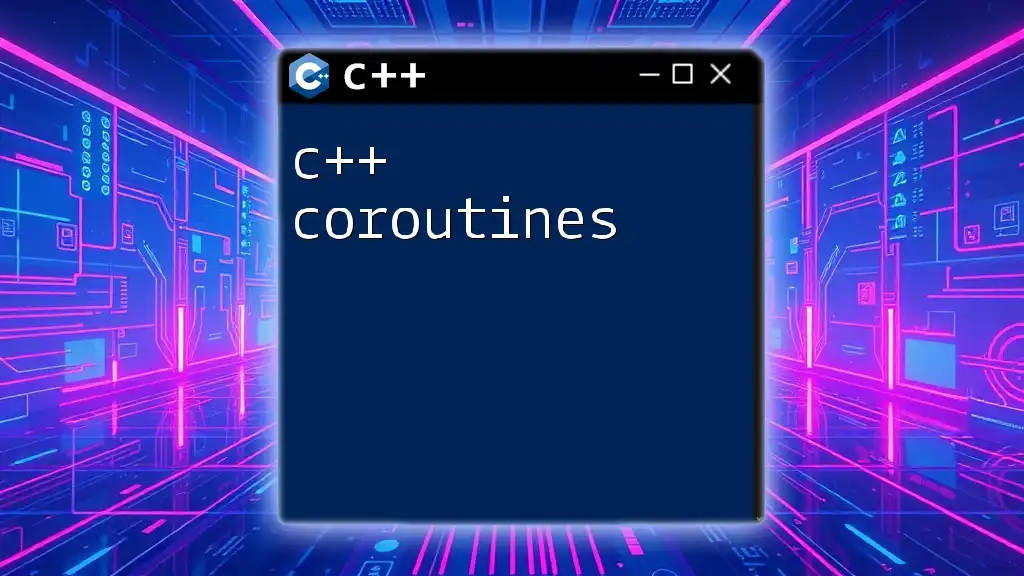
Setting Up cURL in C++
Installing libcurl
To effectively use c++ curl, you'll begin by installing libcurl. The installation procedure varies based on your operating system:
- Windows: You can download precompiled binaries from the official cURL website or install via package managers like vcpkg.
- macOS: Use Homebrew by running `brew install curl` in your terminal.
- Linux: Most distributions include libcurl. For Ubuntu, use `sudo apt-get install libcurl4-openssl-dev`.
Configuring Your C++ Environment
After installation, configuring your C++ environment is crucial. When starting a new project using libcurl:
- Set Up Your IDE: Ensure your IDE is configured to link against the libcurl library. For example, in Visual Studio, you can add the library in Project Properties under Linker > Input > Additional Dependencies.
- CMake Configuration: If you're using CMake, you can include libcurl as follows:
find_package(CURL REQUIRED)
target_link_libraries(your_project_name ${CURL_LIBRARIES})
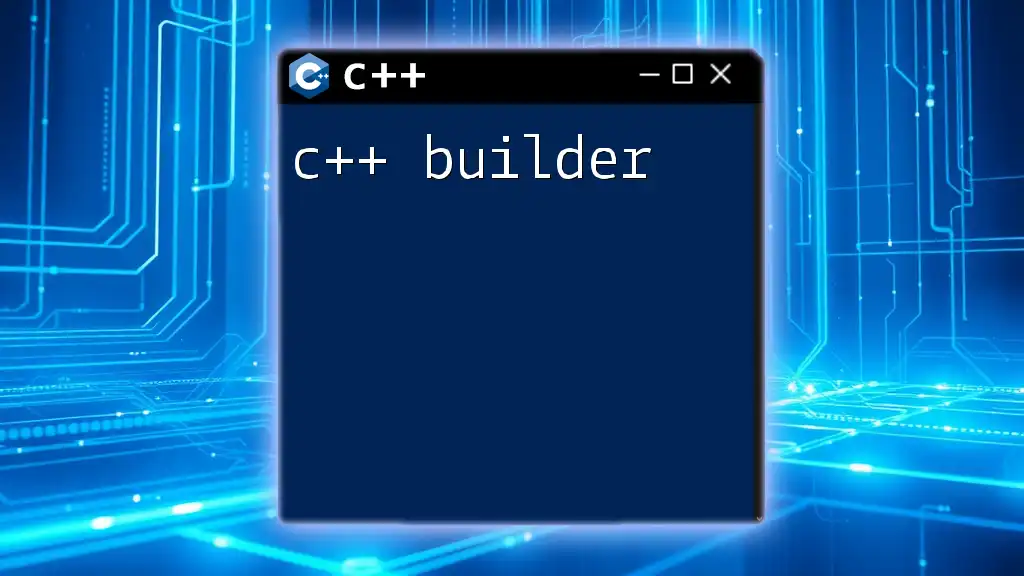
Basic Usage of cURL in C++
Initializing Curl
An essential step in using c++ curl is initializing the cURL environment. The initialization process involves using the `curl_easy_init()` function, which allocates a CURL handle. This handle serves as a reference for performing various operations:
#include <curl/curl.h>
CURL *curl;
curl_global_init(CURL_GLOBAL_DEFAULT);
curl = curl_easy_init();
if(curl) {
// cURL operations here
}
Making a GET Request
GET requests are one of the most common network operations. They are used to retrieve data from a specified resource. To perform a GET request in c++ curl, you set the URL option in the cURL handle:
curl_easy_setopt(curl, CURLOPT_URL, "http://example.com");
CURLcode res = curl_easy_perform(curl);
Handling Response Data
To effectively work with response data, you must implement a callback function. This function is called by cURL as data is received from the server. The following example shows how to write the response data to a string:
size_t WriteCallback(void* contents, size_t size, size_t nmemb, void* userp) {
((std::string*)userp)->append((char*)contents, size * nmemb);
return size * nmemb;
}
// Usage in GET request
std::string readBuffer;
curl_easy_setopt(curl, CURLOPT_WRITEFUNCTION, WriteCallback);
curl_easy_setopt(curl, CURLOPT_WRITEDATA, &readBuffer);
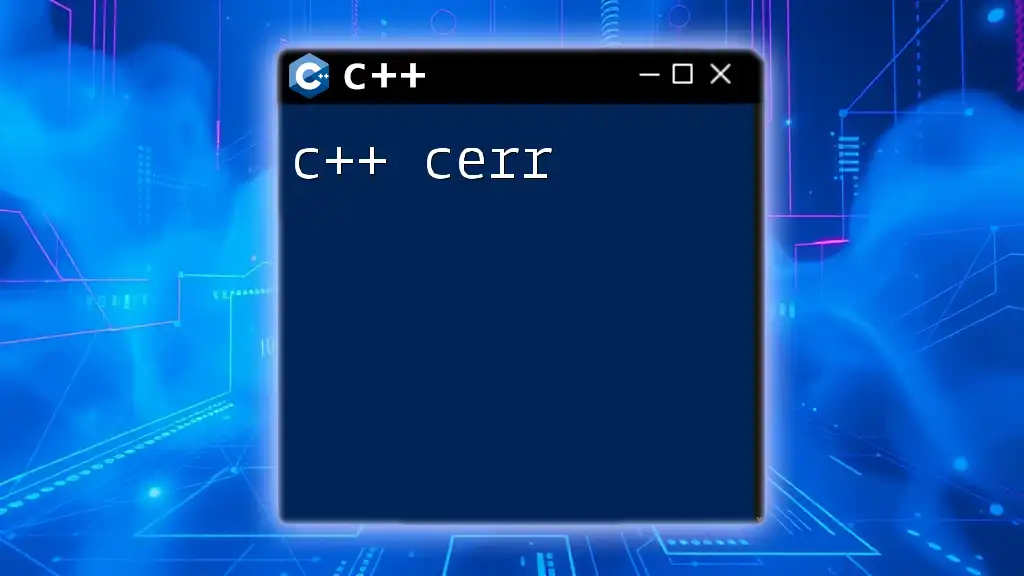
Advanced cURL Features
Making a POST Request
When you need to send data to a server, a POST request is the way to go. Unlike GET requests, POST requests encapsulate the data within the body of the request. Here’s how to perform a POST request in c++ curl:
curl_easy_setopt(curl, CURLOPT_URL, "http://example.com/post");
curl_easy_setopt(curl, CURLOPT_POSTFIELDS, "name=example&project=curl");
Uploading Files
libcurl also supports file uploads, which can be accomplished easily. You need to set the `CURLOPT_UPLOAD` option along with configuring the data to upload. Here’s an example of how to do this:
curl_easy_setopt(curl, CURLOPT_UPLOAD, 1L);
curl_easy_setopt(curl, CURLOPT_READDATA, your_file_pointer);
Setting Headers and User Agents
Custom HTTP headers and user agents can enhance your requests. You can set headers using `curl_slist`. Here’s how to add a JSON content type header:
struct curl_slist *headers = NULL;
headers = curl_slist_append(headers, "Content-Type: application/json");
curl_easy_setopt(curl, CURLOPT_HTTPHEADER, headers);
Handling Redirects
Handling redirects is crucial for many applications. By default, cURL does not follow HTTP redirects, but you can enable this behavior:
curl_easy_setopt(curl, CURLOPT_FOLLOWLOCATION, 1L);
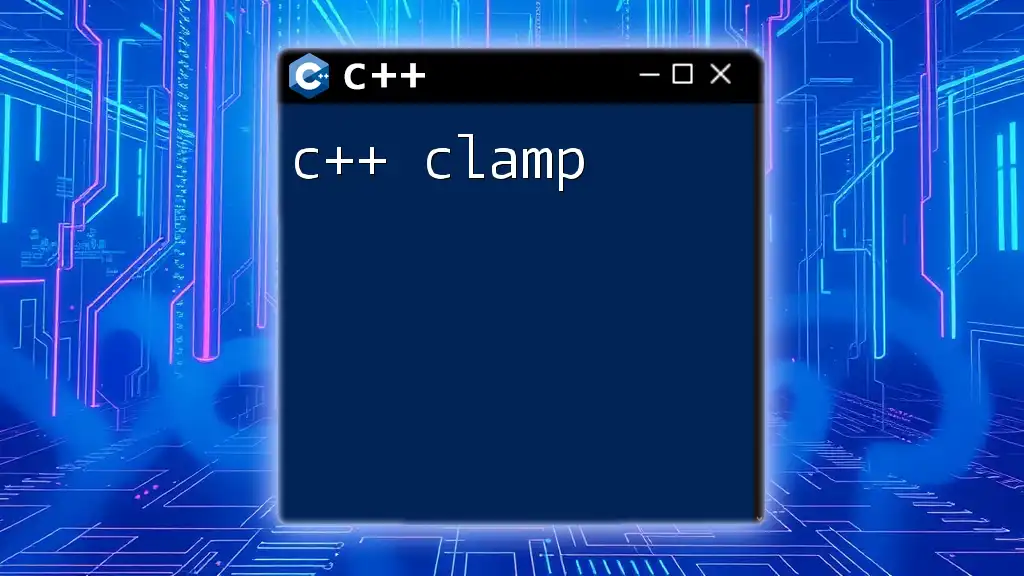
Error Handling in cURL
Common cURL Errors
As you work with cURL, you might encounter various error codes. Understanding these errors is vital for effective debugging. Common error codes include:
- CURLE_COULDNT_CONNECT: Connection could not be established.
- CURLE_HTTP_RETURNED_ERROR: The server returned an error code.
Implementing Error Handling
To effectively handle errors, check the return value of `curl_easy_perform()` and use `curl_easy_strerror()` to convert error codes into human-readable messages:
if (res != CURLE_OK) {
fprintf(stderr, "curl_easy_perform() failed: %s\n", curl_easy_strerror(res));
}
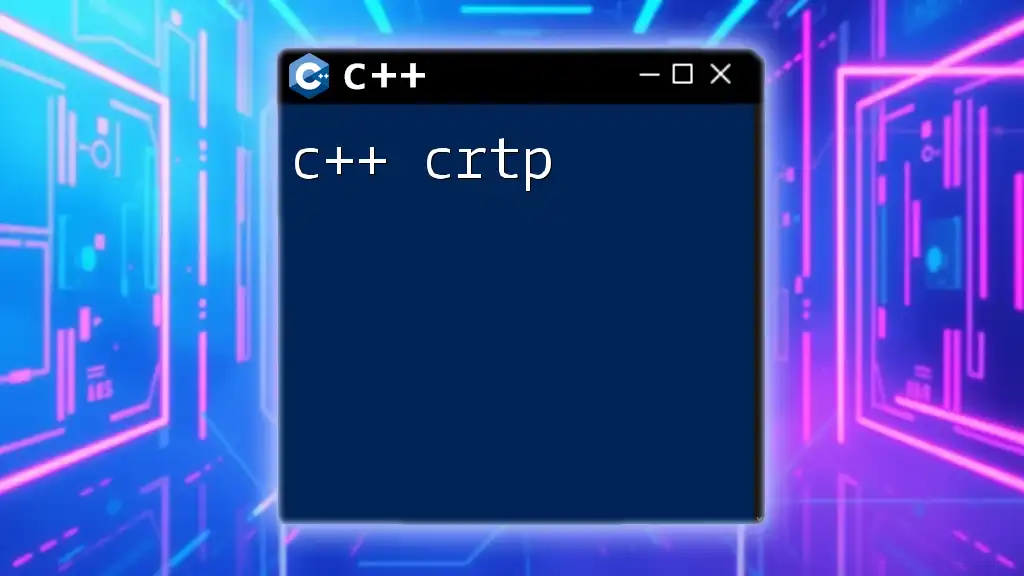
Conclusion
In this guide, we have explored the essentials of using c++ curl, from setting up the environment to making GET and POST requests, handling responses, and managing common errors. By integrating cURL into your C++ applications, you can empower your software with robust network capabilities.
For further exploration, consider diving into the official libcurl documentation and engaging with community forums. This continuous learning will enhance your skillset and improve your ability to use c++ curl effectively.