The Curiously Recurring Template Pattern (CRTP) is a design pattern in C++ where a derived class inherits from a base class template instantiation of itself, enabling static polymorphism.
Here's a simple example:
#include <iostream>
// Base class template
template <typename Derived>
class Base {
public:
void interface() {
// Call the derived class implementation
static_cast<Derived*>(this)->implementation();
}
};
// Derived class
class Derived : public Base<Derived> {
public:
void implementation() {
std::cout << "Derived implementation called!" << std::endl;
}
};
int main() {
Derived d;
d.interface(); // Calls Derived implementation
return 0;
}
What is CRTP?
C++ CRTP, known as the Curiously Recurring Template Pattern, is a powerful programming technique that capitalizes on C++'s template system to enable code reuse and achieve static polymorphism. In essence, CRTP uses inheritance, where a class inherits from a template base class, allowing developers to implement behaviors defined in the base class while leaving specific implementations to the derived class. This pattern is termed "curiously recurring" because the derived class involves itself as a template argument for its base class.
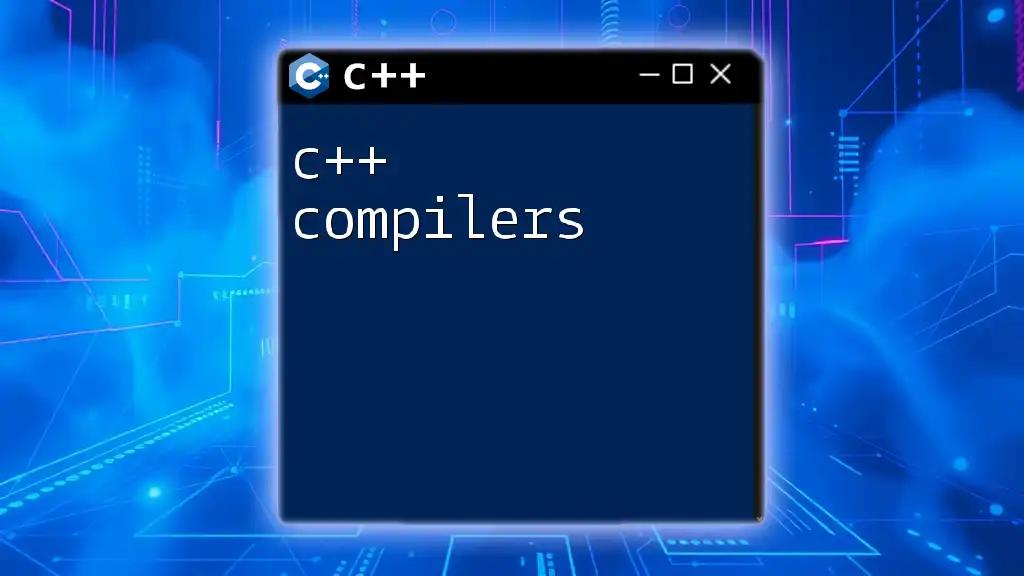
Why Use CRTP?
Choosing to implement CRTP provides several notable advantages:
-
Performance Benefits: With CRTP, all polymorphic behavior is resolved at compile-time, producing optimized code. Since most of the type resolution occurs before the program runs, it helps avoid the overhead associated with virtual function calls.
-
Code Reusability: CRTP promotes a more reusable code structure. Common logic can be encapsulated in the base class while allowing derived classes to provide specific functionality.
-
Static vs. Dynamic Polymorphism: CRTP utilizes static polymorphism, which means that the decision about which implementation to invoke is made at compile-time, leading to better-optimizing opportunities for the compiler.

Understanding the Basics of Templates in C++
What Are Templates?
Templates in C++ invite programmers to write flexible and reusable code by allowing functions and classes to operate with any data type. They act as blueprints or prototypes that enable the creation of a family of classes or functions.
Types of Templates
-
Function Templates: These allow the creation of functions that can accept arguments of different data types. For example, you can create a single sorting function that works on integers, doubles, or even user-defined types.
-
Class Templates: Similar in concept to function templates, class templates help create a class that can handle different data types. For instance, a class representing a box can store items of any type.
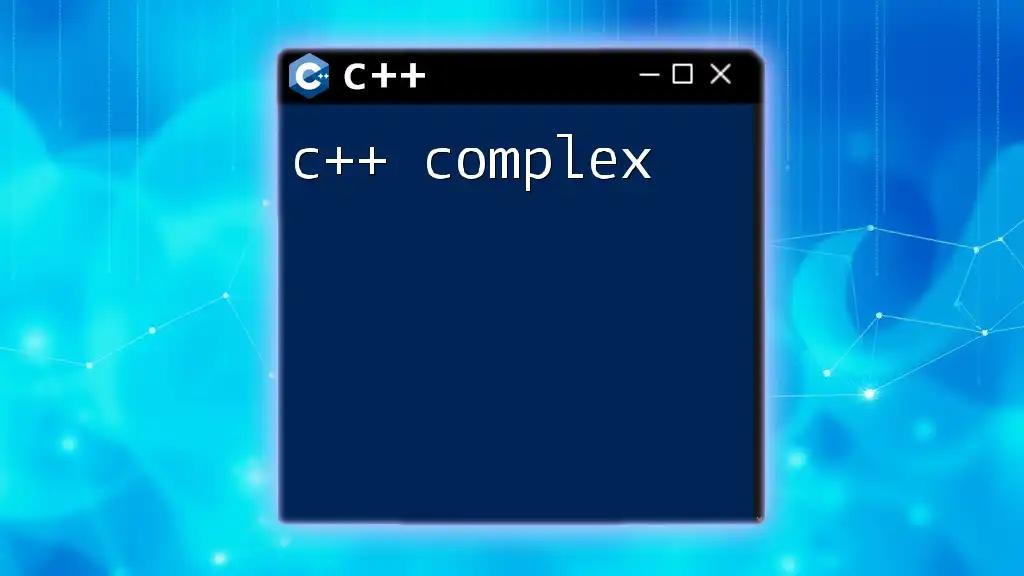
The Curiously Recurring Template Pattern (CRTP)
Understanding CRTP requires delving into the concept of inheritance within C++. When a class inherits from a template base class, it usually does this with its own type, forming a recursive pattern.
Advantages of Using CRTP
-
Type Safety: One of the most significant benefits of CRTP is resolving type issues at compile-time, significantly reducing runtime errors. The compiler can verify that the derived class conforms to expected behavior, increasing overall type safety.
-
Static Polymorphism: Unlike traditional polymorphism, where decisions are made at runtime through virtual functions (thus introducing a performance cost), static polymorphism enables the compiler to optimize calls and ensure that the correct member function is invoked without the overhead of pointers.
-
Increased Performance: Since CRTP resolves method calls at compile time, it often produces more optimized code than equivalent solutions using virtual methods, leading to faster execution times.
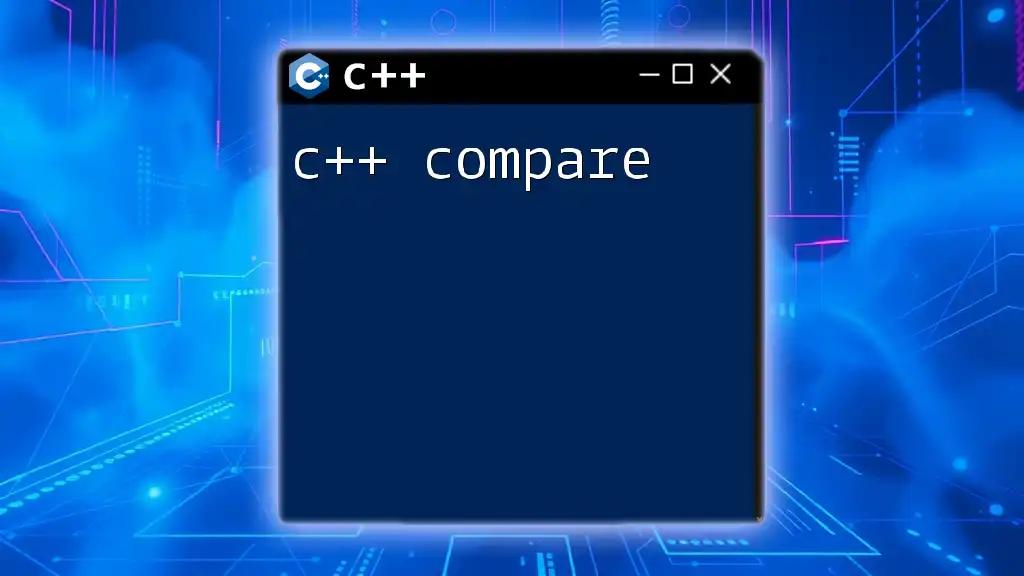
Implementing CRTP
Basic CRTP Implementation
Here’s a simple example demonstrating how CRTP works:
template <typename Derived>
class Base {
public:
void interface() {
static_cast<Derived*>(this)->implementation();
}
};
class Derived : public Base<Derived> {
public:
void implementation() {
// Derived implementation logic
}
};
In this example, `Base` is a template class that takes a type parameter `Derived`. The `interface` method calls the `implementation` method defined in the derived class, enabling polymorphic behavior.
Complex Example of CRTP
Let’s observe a more intricate implementation of CRTP through a shape class.
template <typename Derived>
class Shape {
public:
double area() {
return static_cast<Derived*>(this)->calculateArea();
}
};
class Circle : public Shape<Circle> {
double radius;
public:
Circle(double r) : radius(r) {}
double calculateArea() {
return 3.14159 * radius * radius; // Area calculation
}
};
In this example, `Shape` serves as a template base with a method that calls the derived class’s `calculateArea` function. The `Circle` class inherits from `Shape`, and when `area()` is invoked, it executes the `calculateArea()` method of `Circle`, allowing for specific area calculations.

Practical Applications of CRTP
Design Patterns Using CRTP
CRTP finds use in various design patterns, including the Visitor Pattern, where you may want to define operations across a number of different classes without modifying those classes. CRTP allows the implementation of this pattern without the need for runtime type checks.
Code Reusability
By defining common functionalities in the template base class, you achieve practical code reuse. This reflects the DRY (Don't Repeat Yourself) principle, making your code cleaner and easier to maintain.
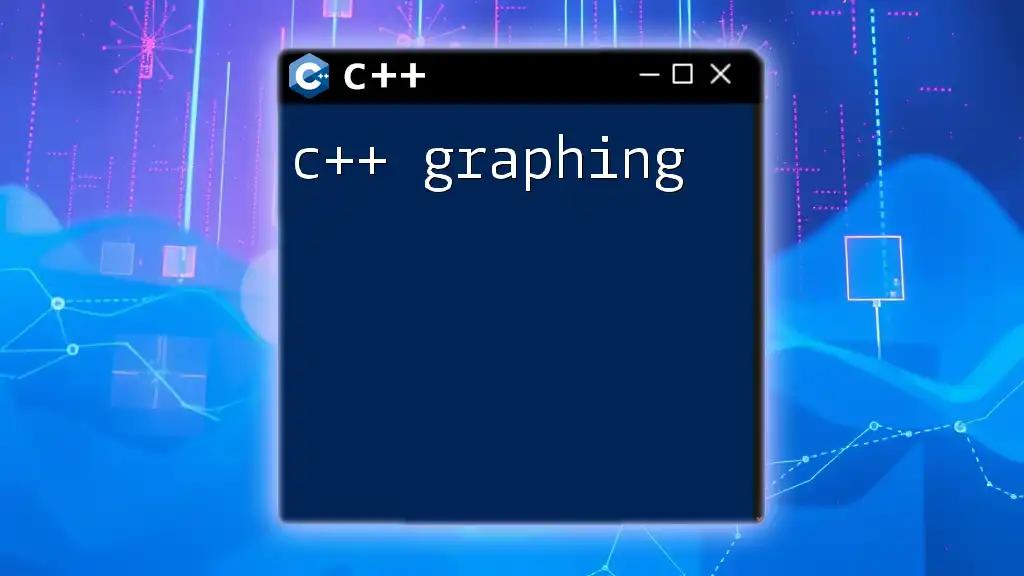
Common Pitfalls and Mistakes
Complexity Management
CRTP can introduce complexity when overused, as it introduces templates and multiple inheritance. Therefore, it's essential to balance the use of templates with code readability and maintainability.
Template Bloat
Another consideration is template bloat, which can lead to longer compile times and larger binaries. If a template is instantiated with many different types, it results in multiple copies of the code, which can be avoided with careful design.

Best Practices for Using CRTP
Clear Documentation
With the potential complexities CRTP introduces, documenting your code becomes vital. Ensure that the relationships between base and derived classes are well described, making your code easier to navigate for yourself and others.
Testing and Debugging
When working with templates, testing can become intricate faster than with regular classes. Utilize unit tests effectively, and consider developing smaller classes to test granulated functionalities before integrating them into a more extensive framework.
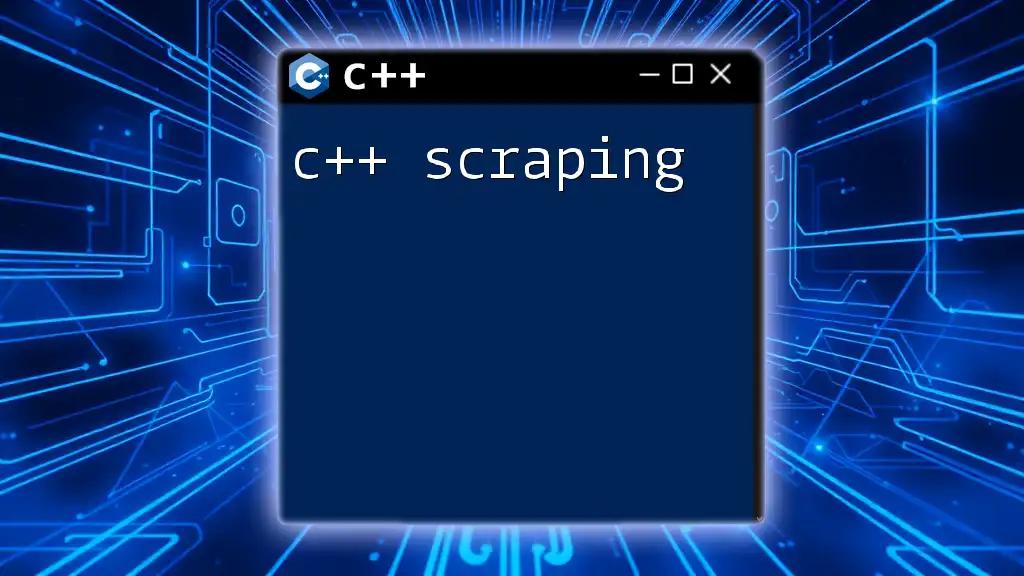
Conclusion
C++ CRTP is a robust and effective paradigm that offers high-performance capabilities through its unique approach to static polymorphism. By understanding the intricacies of CRTP, developers can write cleaner, more efficient, and reusable code structures. While there are challenges associated with its complexity and potential bloat, the benefits heavily outweigh the downsides when implemented with best practices. C++ programming enthusiasts should explore CRTP further and recognize its beneficial implications in modern software design.

Additional Resources
For those eager to deepen their understanding beyond this guide, consider referring to comprehensive texts on advanced C++ programming or engaging in online communities where members share experiences and insights related to templates and CRTP.
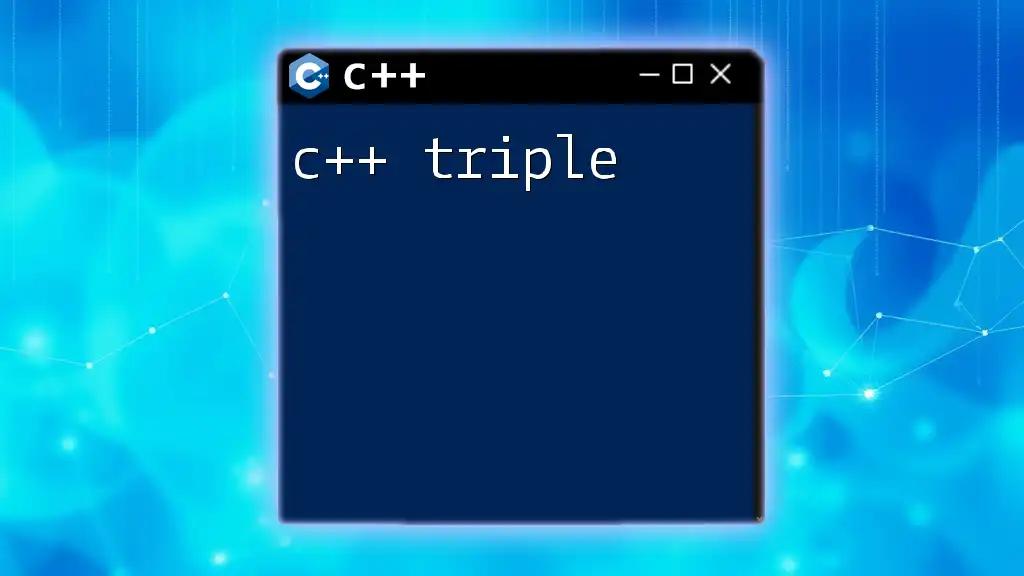
Call to Action
Join our community to stay updated on C++ programming concepts and best practices. We invite you to share your development experiences with CRTP or any other C++ paradigms, enriching everyone's knowledge in this evolving field!