The C++ clipper refers to a library used for geometric operations, such as clipping and offsetting polygons, which is essential for various computer graphics and CAD applications.
#include "clipper.hpp"
// Example of using Clipper to perform polygon clipping
ClipperLib::Clipper clipper;
ClipperLib::Path subject = {{10, 10}, {20, 10}, {20, 20}, {10, 20}};
ClipperLib::Path clip = {{15, 15}, {25, 15}, {25, 25}, {15, 25}};
ClipperLib::Paths solution;
clipper.AddSubject(subject);
clipper.AddClip(clip);
clipper.Execute(ClipperLib::ctIntersection, solution);
What is C++ Clipper?
C++ Clipper is a powerful library designed for performing geometric operations on polygons. It provides developers with the ability to execute complex operations efficiently, making it invaluable for applications requiring spatial computations, such as computer graphics, GIS (Geographic Information Systems), and game development.
The necessity of C++ Clipper arises from the complexity involved in handling polygonal shapes. Whether it’s merging two areas into a single space or determining their overlap, the C++ Clipper library allows developers to manage these tasks with ease and precision.
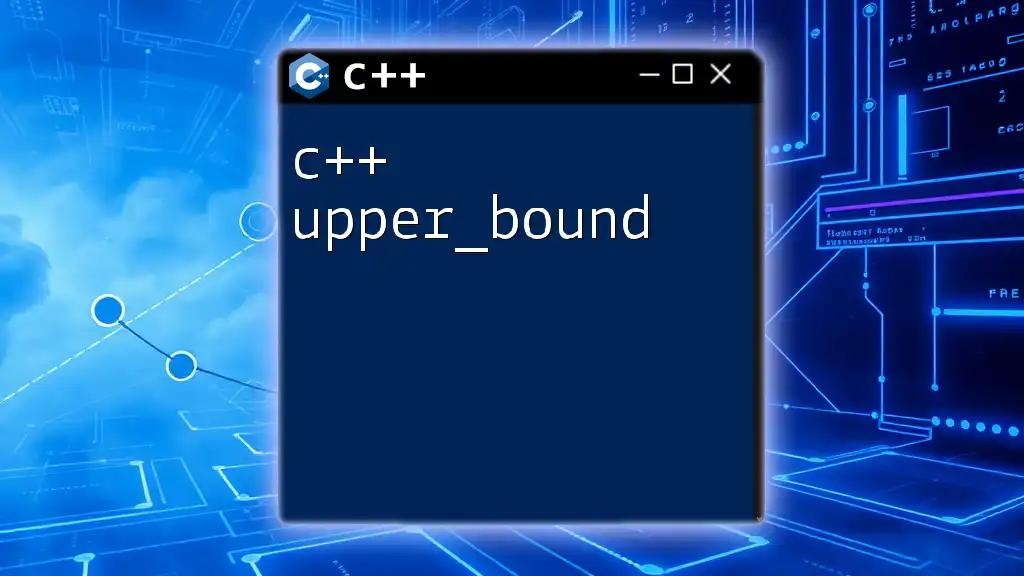
Key Features
C++ Clipper stands out due to several important features:
- Efficiency: Designed for speed, C++ Clipper performs operations quickly, making it suitable for real-time applications.
- Robustness: The library effectively handles complex cases such as self-intersecting shapes and overlapping polygons.
- Versatility: Support for various polygon operations – union, intersection, difference, and more.
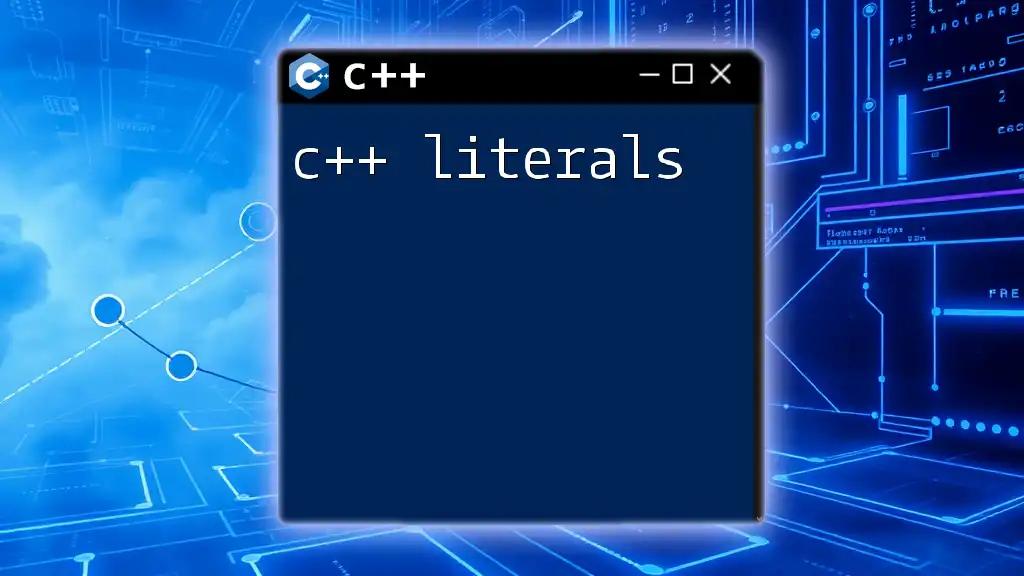
Understanding Polygon Operations
Defining Polygons in C++
In C++, polygons are often defined using a data structure consisting of vertices or points. Typically, these polygons are represented as lists of coordinates.
You can create a polygon by defining a sequence of points:
#include <clipper.hpp> // Include Clipper header
using namespace ClipperLib;
// Define a point type
using IntPoint = ClipperLib::IntPoint;
// Create a polygon
std::vector<IntPoint> polygon = { IntPoint(10, 10), IntPoint(20, 10), IntPoint(20, 20), IntPoint(10, 20) };
Polygon Operations Overview
C++ Clipper supports various polygon operations:
- Union: Combines two or more polygons into a single polygon.
- Intersection: Finds the area that two polygons share.
- Difference: Subtracts one polygon from another.
- XOR: Combines polygons while removing areas that overlap.

Setting Up C++ Clipper in Your Project
Installation of C++ Clipper
To use C++ Clipper, you can download it from its [GitHub repository](https://github.com/angusj/clipper2). Once downloaded, include the necessary files in your project.
Configuration Settings
Make sure your project configuration allows for the proper linking of the Clipper library. For most compilers, this includes setting the correct include paths and ensuring that the Clipper source files are compiled along with your project code.
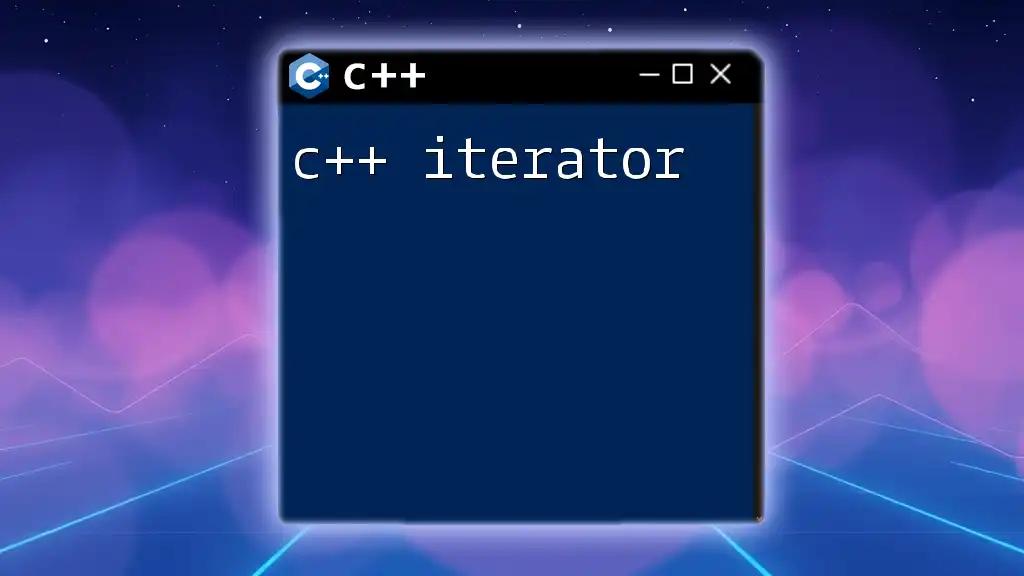
Basic Usage of C++ Clipper
Creating Simple Polygons
With your project set up, you can create and manipulate polygons. A simple square polygon can be defined as follows:
Polygon square;
square.push_back(IntPoint(0, 0));
square.push_back(IntPoint(0, 10));
square.push_back(IntPoint(10, 10));
square.push_back(IntPoint(10, 0));
Performing Basic Operations
Union Operation
The union operation merges two polygons. Here’s how to perform it with C++ Clipper:
Paths solution;
Clipper clipper;
clipper.AddPath(square, ptSubject, true);
clipper.AddPath(otherPolygon, ptClip, true);
clipper.Execute(ctUnion, solution);
The result will be stored in the `solution` variable, which contains the newly formed polygon.
Intersection Operation
To find the overlapping area between two polygons, use the intersection operation:
Paths intersectionSolution;
clipper.Execute(ctIntersection, intersectionSolution);
This will yield the areas where the two polygons coincide, allowing for the assessment of common territory.
Difference Operation
The difference operation takes one polygon away from another. Here's how it can be done:
Paths differenceSolution;
clipper.Execute(ctDifference, differenceSolution);
Use this when you want to see what remains after one polygon is removed from another.
XOR Operation
The XOR operation will return the non-overlapping parts of the polygons:
Paths xorSolution;
clipper.Execute(ctXor, xorSolution);
This is particularly useful for visualizing how two shapes interact without considering their overlapping portions.
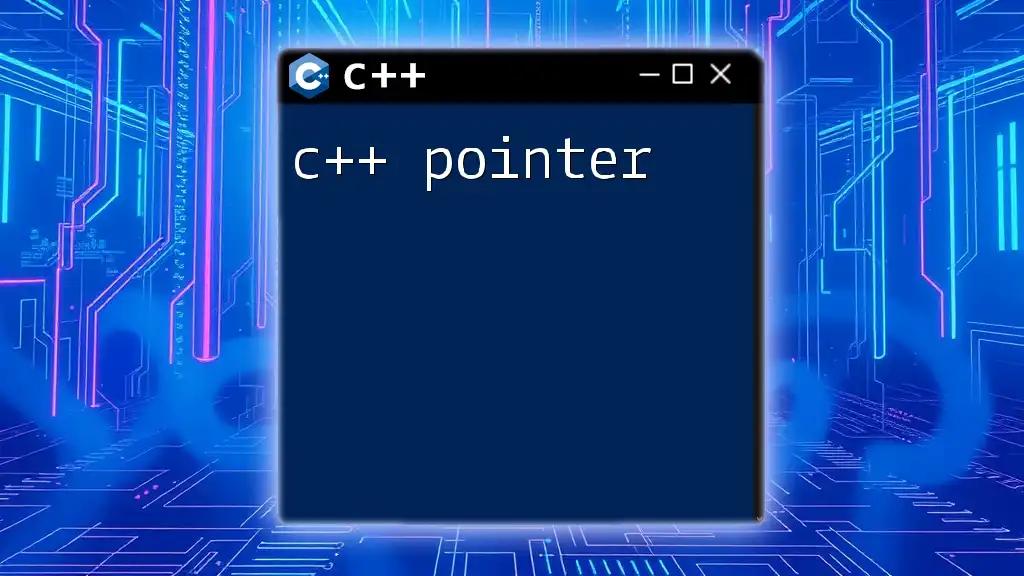
Advanced Features of C++ Clipper
Handling Complex Polygons
C++ Clipper is designed to handle complex and overlapping polygons, allowing developers to work with non-simple shapes effectively. By utilizing the library's robust algorithms, one can manage intricate geometric figures without worrying about the internal workings. For instance, if you have a polygon with self-intersecting edges, C++ Clipper can seamlessly process it:
clipper.AddPath(complexPolygon, ptSubject, true);
Offsetting Polygons
One of the advanced features of C++ Clipper is the ability to create offset polygons, which can be particularly advantageous in architectural layouts.
To offset a polygon, you can use the `Offset` method:
clipper.Offset(5.0);
This shifts the polygon outward or inward by 5 units, thus preserving its shape while changing its size.
Polygon Simplification
Polygon simplification is a useful feature to reduce the number of vertices while maintaining the overall shape of the polygon. This is essential in rendering and optimizing graphical applications.
You can achieve simplification with:
clipper.SimplifyPolygon(polygon);
This command retains the polygon's shape, ideally removing unnecessary vertices to streamline processing without sacrificing visual integrity.
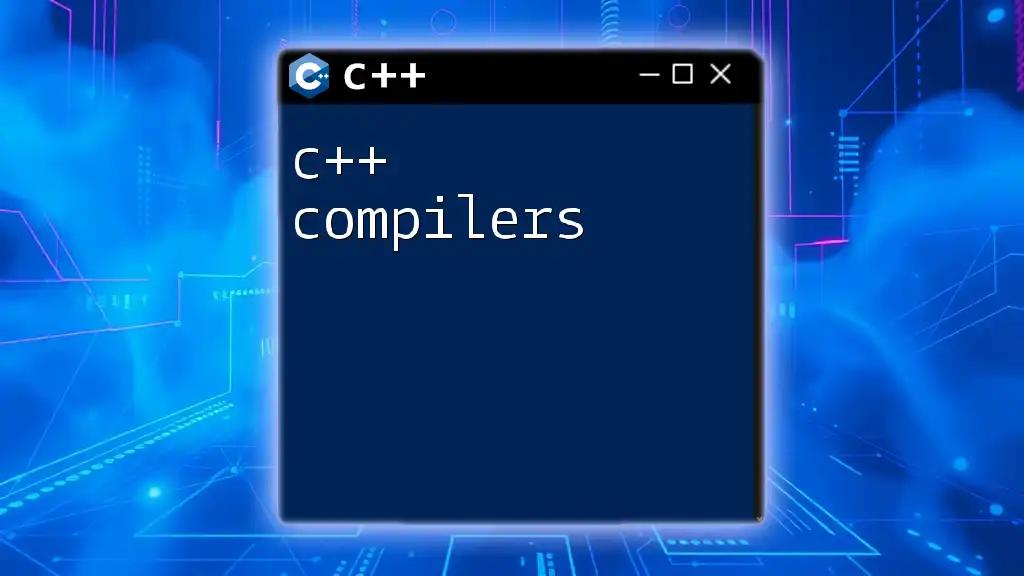
Performance Optimizations
Optimizing Code with C++ Clipper
For optimal performance, it's important to keep your code clean and efficient. Some best practices include:
- Reducing the number of vertices where possible.
- Grouping polygons by size or complexity for batch processing.
- Utilizing memory-efficient data structures.
Here’s an example of a best practice when adding polygons:
clipper.AddPaths(polygonList, ptSubject, true);
This approach minimizes overhead by processing multiple polygons in fewer calls.
Profiling Performance
Performance profiling tools, such as gprof or Visual Studio’s built-in profiler, can be utilized to understand where bottlenecks may occur in your usage of C++ Clipper. This allows for targeted optimizations based on actual usage patterns.
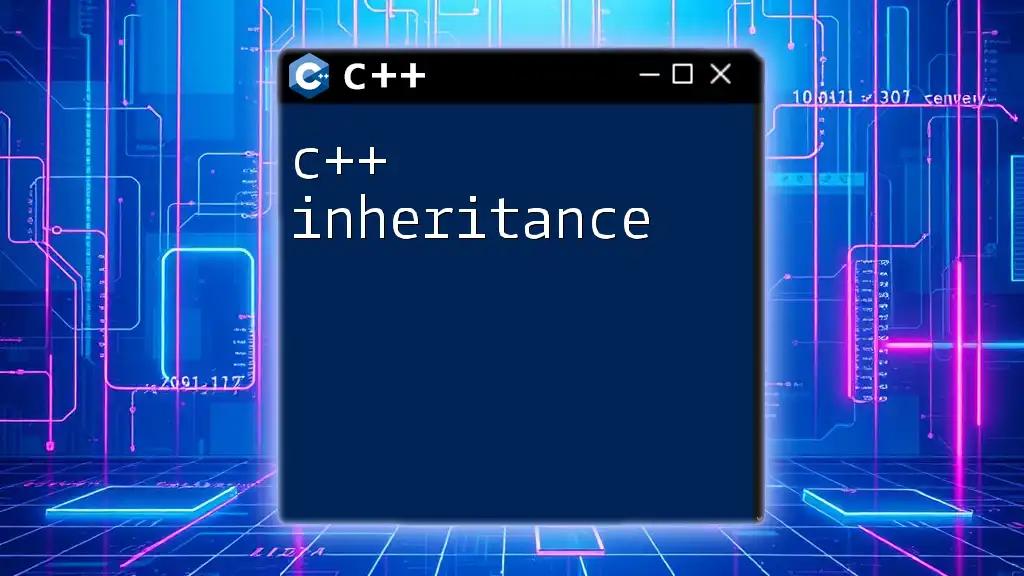
Common Issues and Troubleshooting
Debugging Common Errors
During development, you may encounter several common issues, such as:
- Self-Intersecting Polygons: Ensure polygons are defined correctly.
- Memory Leaks: Be cautious with pointers and dynamic allocations.
If you encounter an error, carefully review polygon definitions and ensure correct data types are used.
FAQs about C++ Clipper
- Is C++ Clipper free to use?
- Yes, C++ Clipper is open-source and can be used without any cost.
- Can Clipper handle 3D polygons?
- No, C++ Clipper is primarily designed for 2D polygon operations.

Conclusion
C++ Clipper is an essential library for any developer needing to perform geometric operations on polygons efficiently. The comprehensive capabilities allow seamless execution of diverse polygon operations, making it a must-have tool in the developer's toolkit. We encourage you to explore C++ Clipper in your projects, experiment with its features, and optimize your graphical applications with cutting-edge geometric processing.
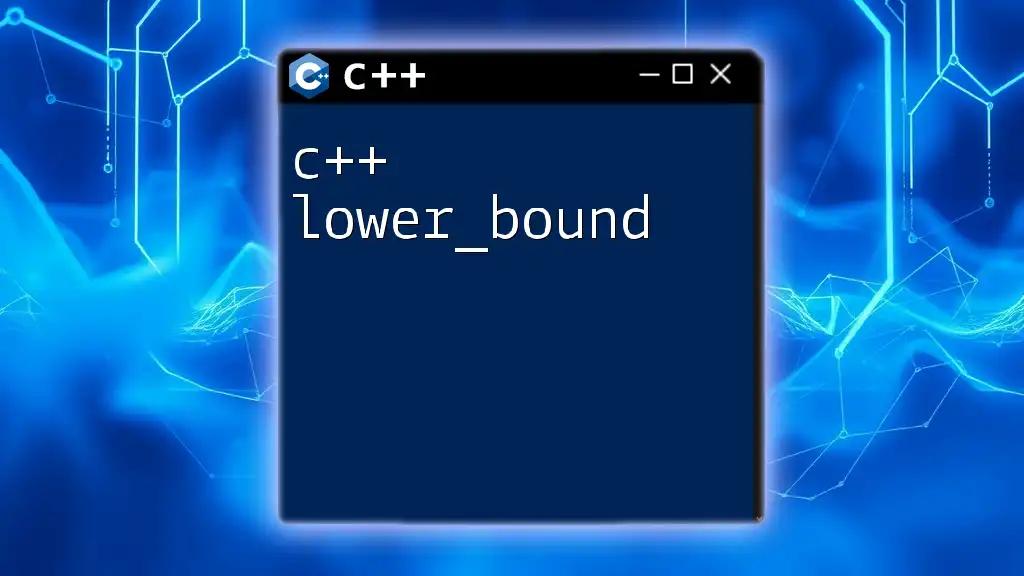
Further Resources
For further reading and learning, check out the official documentation of C++ Clipper for in-depth guidance and examples. Engage with the community through forums and support groups to share experiences and clarify doubts regarding usage.
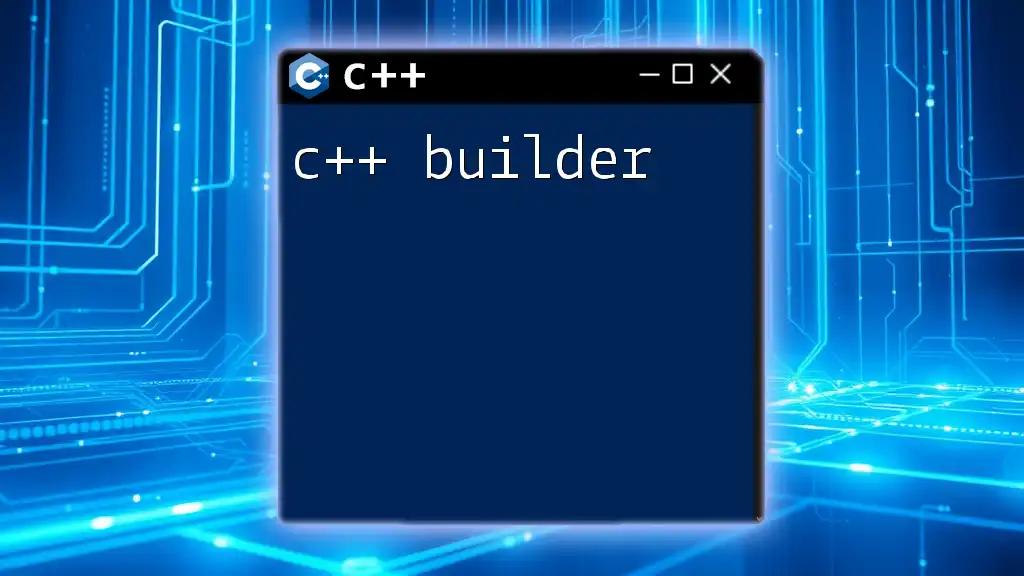
Acknowledgments
Special thanks to the authors and contributors of C++ Clipper, whose hard work has made these sophisticated polygon operations accessible and manageable for developers worldwide.