A C++ pipe, often associated with command-line interfaces, allows for the output of one process to be used as the input for another, facilitating streamlined data processing.
Here's a simple example of using pipe syntax in C++ with the `popen()` function:
#include <cstdio>
int main() {
FILE* pipe = popen("ls -l | grep '.cpp'", "r");
if (!pipe) return 1;
char buffer[128];
while (fgets(buffer, sizeof(buffer), pipe) != nullptr) {
printf("%s", buffer);
}
pclose(pipe);
return 0;
}
Understanding the Concept of Pipes
A pipe in C++ is a powerful concept originally borrowed from Unix/Linux operating systems, facilitating the inter-process communication (IPC) by allowing the output of one process to be used as input for another. This capability enhances the modularity of programming by enabling different applications or processes to work together seamlessly without needing to share memory explicitly.
In modern programming, especially in systems-level programming and concurrent applications, understanding how to employ pipes effectively is crucial. They not only streamline data handling but also encapsulate functionality in a manageable format, allowing developers to build more efficient and organized code.
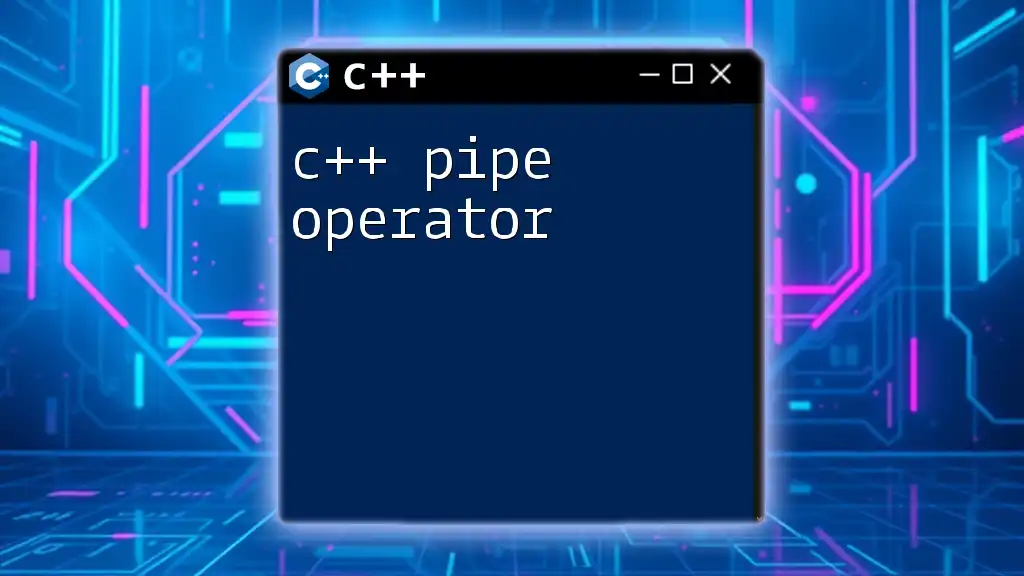
The Basics of Pipe Syntax in C++
Overview of Pipe Syntax
The syntax for using pipes in C++ primarily revolves around the pipe operator (`|`). This operator takes the output of one command and transforms it into the input for another, simplifying how data flows between processes.
Creating a Simple Pipe
To demonstrate the use of a C++ pipe, let's create a simple example that uses a pipe for basic communication between a parent process and a child process. The following code illustrates how to achieve this:
#include <iostream>
#include <unistd.h>
int main() {
int pipefd[2];
pid_t p1;
pipe(pipefd); // Create a pipe
p1 = fork(); // Create a child process
if (p1 == 0) { // Child process
close(pipefd[1]); // Close the write end
char buffer[10];
read(pipefd[0], buffer, sizeof(buffer)); // Read from pipe
std::cout << "Received: " << buffer << std::endl;
close(pipefd[0]); // Close the read end
} else { // Parent process
close(pipefd[0]); // Close the read end
const char* msg = "Hello";
write(pipefd[1], msg, sizeof(msg)); // Write to pipe
close(pipefd[1]); // Close the write end
}
return 0;
}
In this code:
- We create a pipe using the `pipe()` function, which returns two file descriptors: one for reading and one for writing.
- A child process is created with the `fork()` function. The child process reads from the pipe, while the parent process writes to it.
- Proper handling of the file descriptors is ensured by closing the unused ends of the pipe in each process.
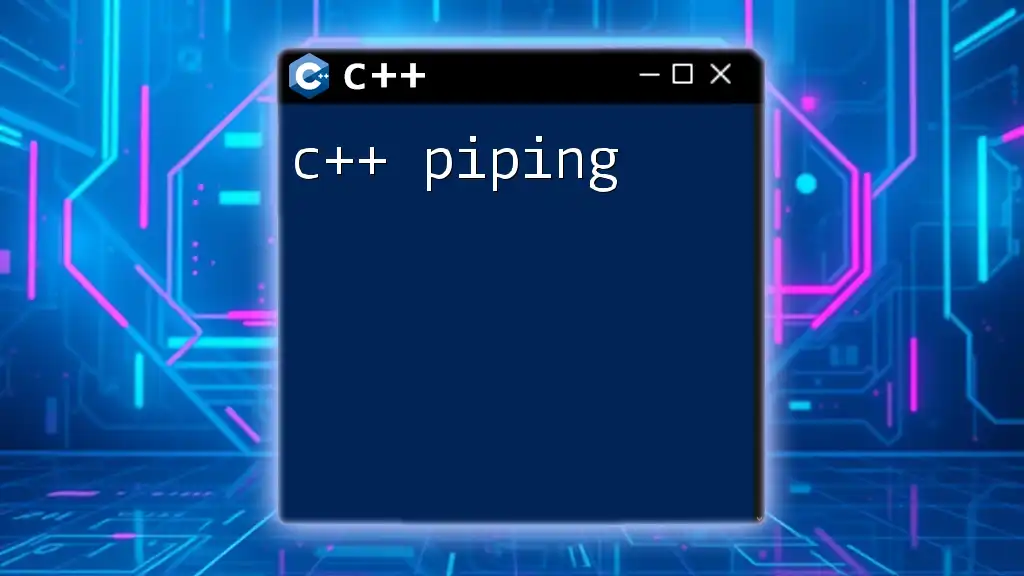
Advanced Pipe Concepts
Using Multiple Pipes
A common scenario in advanced programming involves the use of multiple pipes to handle more complex communication between processes. You can chain several processes together where the output from one process becomes the input for another, creating a sophisticated flow of data.
Pipe with More Complex Data Types
When working with more complex data types, such as structures or classes, you can serialize the object and send it through the pipe. Here’s an example demonstrating how to send a custom structure using pipes:
#include <iostream>
#include <cstring>
#include <unistd.h>
struct Data {
int num;
char message[20];
};
int main() {
int pipefd[2];
pipe(pipefd);
Data data = {42, "HelloPipe"};
write(pipefd[1], &data, sizeof(data)); // Write structured data to pipe
Data received;
read(pipefd[0], &received, sizeof(received)); // Read structured data from pipe
std::cout << "Received Number: " << received.num << ", Message: " << received.message << std::endl;
return 0;
}
In this example, we define a structure `Data` containing an integer and a character array. We write this structure to the pipe and read it in another process, demonstrating how to handle more complex data types with pipes.
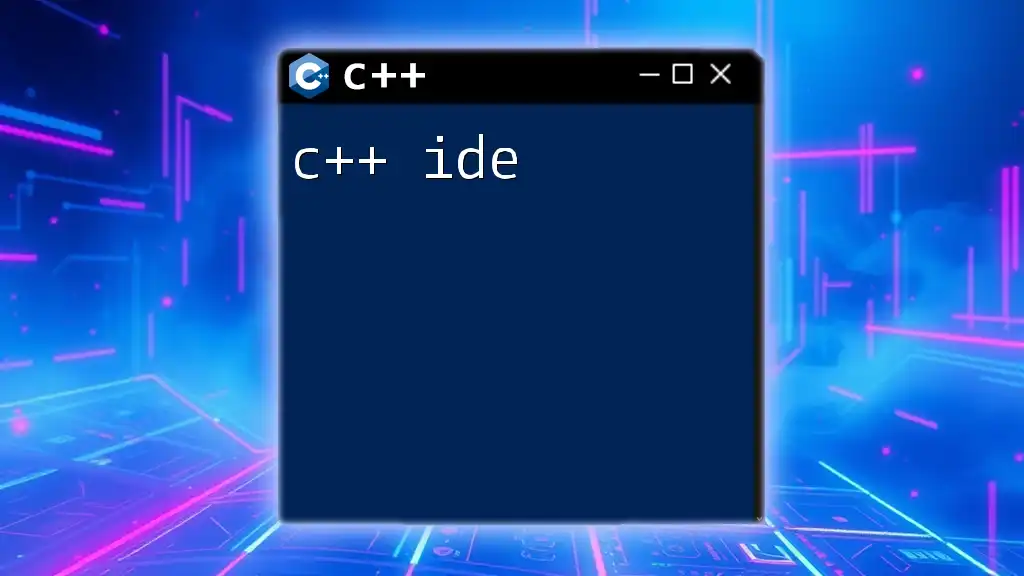
Error Handling in C++ Pipes
Common Errors and Solutions
When working with pipes, it's essential to be aware of potential errors that may arise, such as:
- Failed pipe creation: Check if the `pipe()` function returns -1.
- Read/write failures: Always validate the return values of `read()` and `write()` functions to handle any issues during data transmission.
Best Practices for Pipe Management
To ensure robust pipe management, follow these guidelines:
- Always check the return value of system calls like `pipe()`, `fork()`, `read()`, and `write()`.
- Close the file descriptors that are no longer needed to avoid resource leaks.
- Implement error handling by using `perror()` or checking `errno` to gain insights into potential failure reasons.
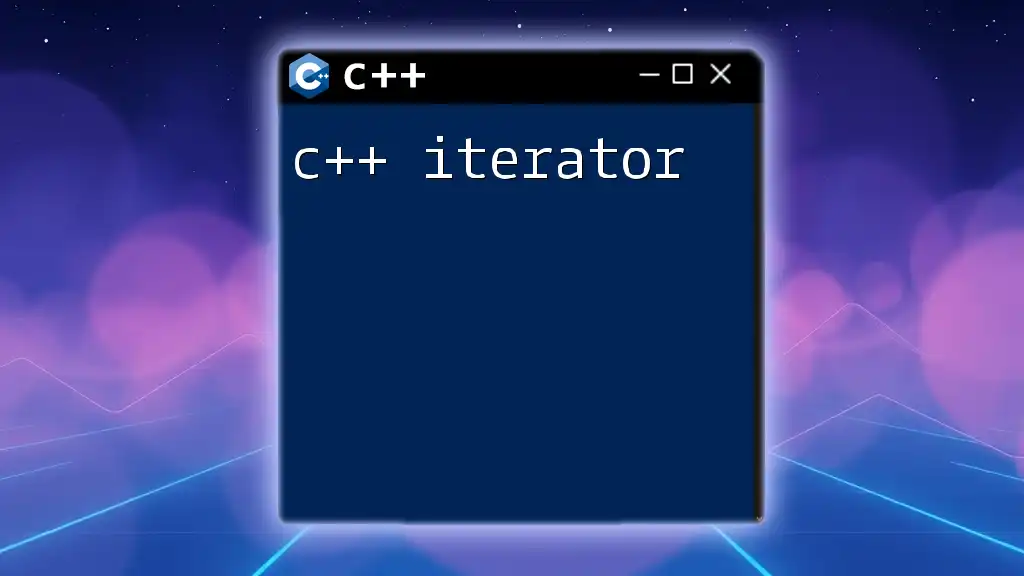
Real-World Applications of C++ Pipes
Interprocess Communication
The most prevalent use case for C++ pipes is inter-process communication, where data needs to be smoothly transmitted between multiple processes. For example, in a web server architecture, different processes may handle requests and responses using pipes, improving overall efficiency.
Data Processing Workflows
Pipes can be utilized to create complex data processing workflows. In scenarios such as data transformations, you can harness pipes to pass input data through a series of processing stages, each transforming the data until the final output is achieved. This approach not only simplifies the codebase but also enhances maintainability.
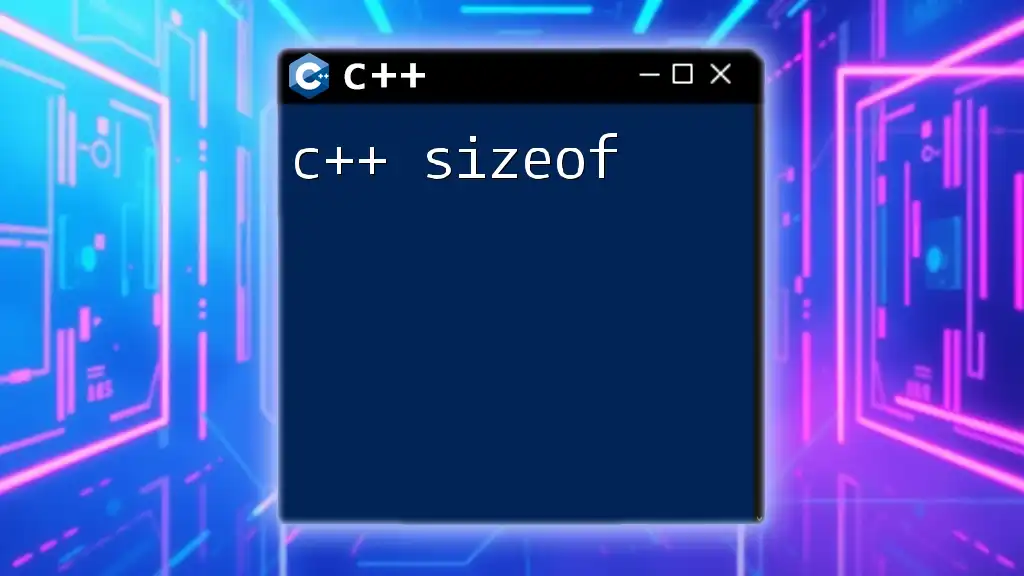
Conclusion
In summary, C++ pipes provide an effective method for inter-process communication and data handling. From building simple communication mechanisms to structuring complex data workflows, mastering pipe functionality will significantly enhance your programming skills. Continue diving deeper into this topic through various resources, tutorials, and community forums to harness the full potential of C++ pipes in your projects.