C++ piping allows you to redirect the output of one command or stream to another, enabling efficient data processing and manipulation.
#include <iostream>
#include <sstream>
int main() {
std::ostringstream pipe;
pipe << "Hello, " << "World!" << std::endl;
std::cout << pipe.str(); // Output: Hello, World!
return 0;
}
What is Piping?
C++ piping refers to the mechanism of connecting different commands or outputs to utilize the results of one operation as the input for another. This streamlined data flow provides an efficient way to handle input and output, making C++ code cleaner and more intuitive. Piping is particularly useful in handling time-sensitive data, processing streams, or manipulating outputs seamlessly.
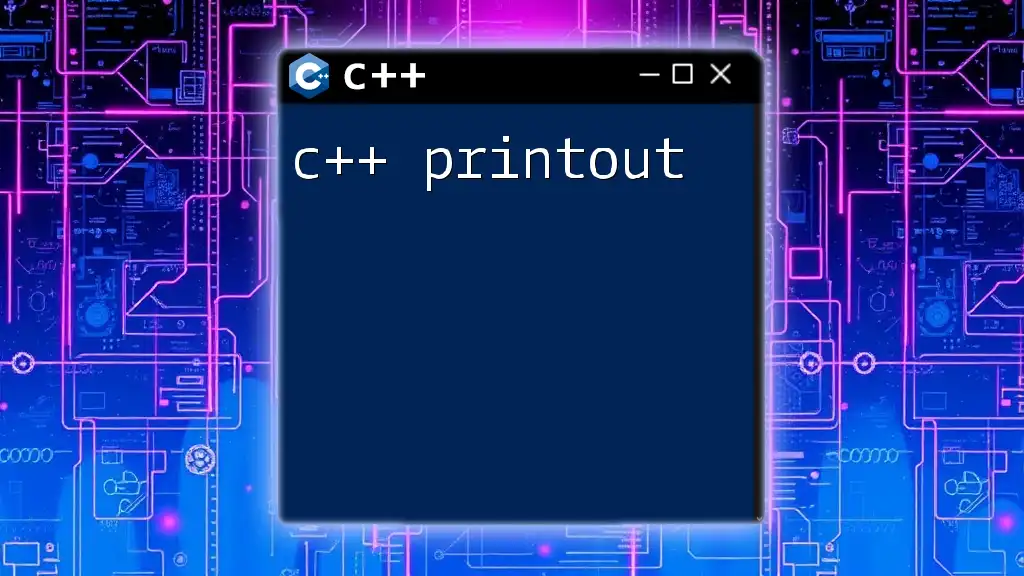
Understanding Output Streams
What are Output Streams?
Output streams represent channels through which data is sent out from a program. In C++, the most commonly used output stream is `std::cout`, which allows you to display output on the console. Stream operations make it simple to format and display data.
The Syntax of Output Streams
Using output streams in C++ is straightforward. The basic syntax for outputting data to the console involves the `<<` operator, which inserts the value into the output stream. For example:
std::cout << "Hello, World!";
This statement sends the phrase "Hello, World!" to the standard output.
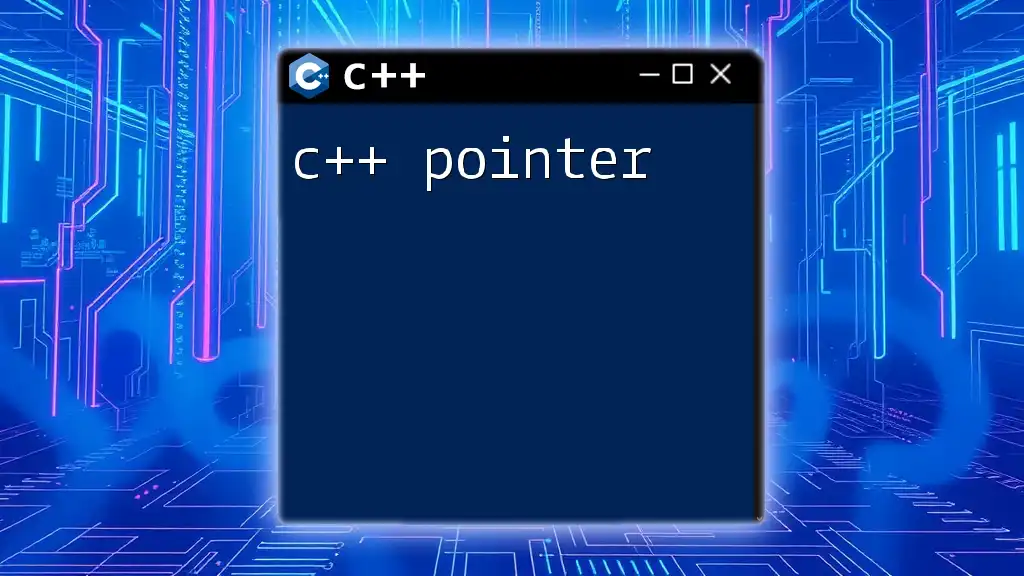
The Concept of Piping in C++
Defining Piping in C++
Piping in C++ can be viewed as a conduit for transferring output from one segment of code to another, enabling fluid communication between different operations. It is particularly prominent in contexts where values need to be processed or transformed before being displayed.
The Mechanism of Piping
Understanding the mechanics of piping involves recognizing how data flows through the program. Piping can be visualized as a series of connected nodes, where the output of one node (or command) directly feeds into the input of the next. This creates a smooth transition of data without the need for intermediate variables or complex function calls.
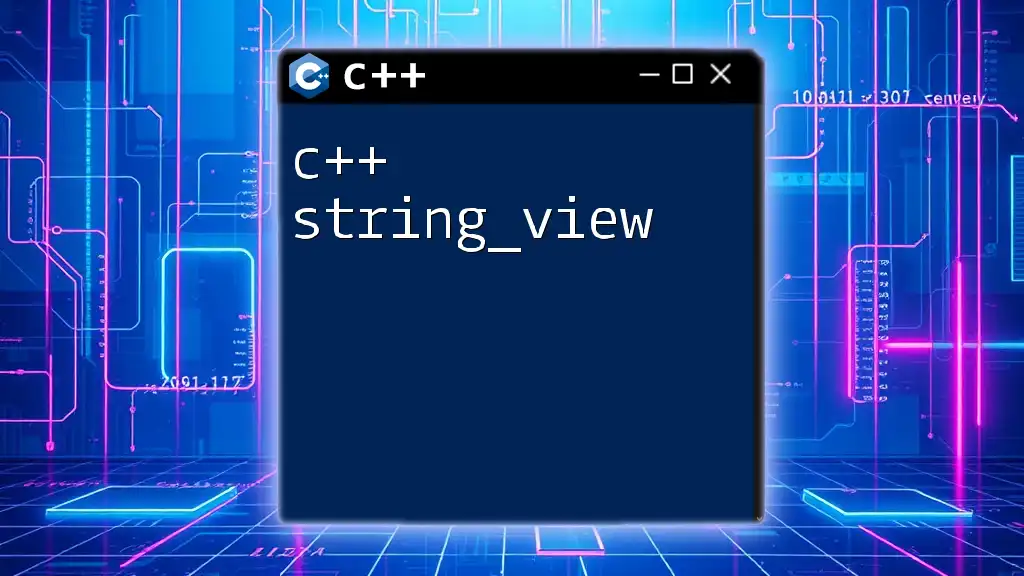
Implementing Piping with the C++ Standard Library
Using Standard Library Functions for Piping
Leveraging C++'s Standard Library is essential for effective pipelining. Include necessary headers such as:
#include <iostream>
#include <sstream>
Example of Simple Piping
A basic example of piping functionality can be demonstrated through the `std::ostringstream` class. This class allows us to construct strings with formatted output, which can later be processed or displayed:
std::ostringstream pipe;
pipe << "Piped data: " << 42;
std::cout << pipe.str() << std::endl;
In this example, the integer `42` is piped into a string stream and displayed as part of a formatted string.
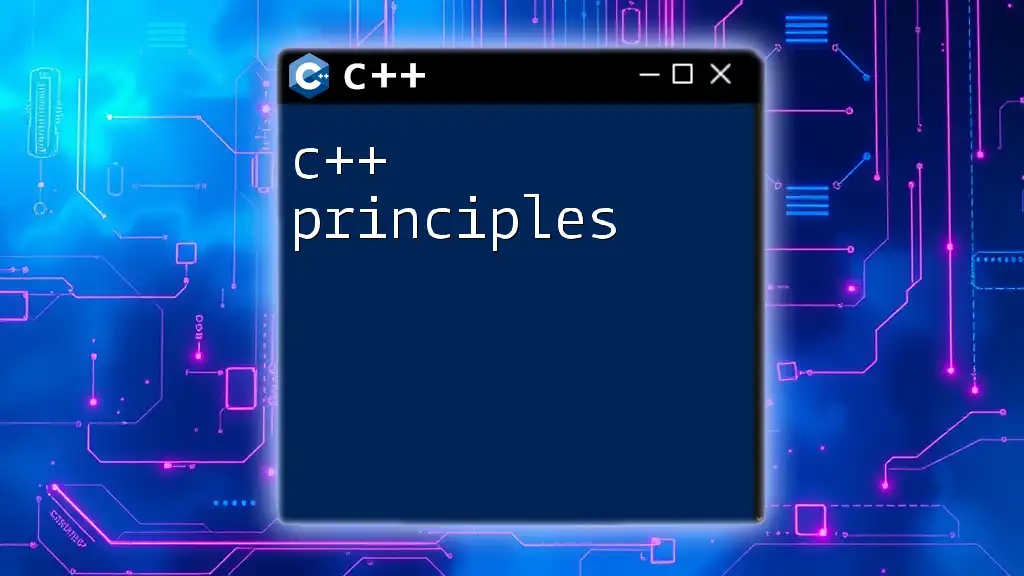
Advanced Piping Techniques
Chaining Commands with Piping
C++ makes it easy to chain commands using the piping mechanism. By sequentially linking output commands, we can improve readability and maintainability. For instance:
std::cout << "Initial Value" << std::endl << "Manipulated Value" << std::endl;
In this example, multiple outputs are handled in a single statement, showcasing the power of piping in reducing code complexity.
Error Handling in Pipe Operations
Proper error handling is crucial when working with pipes. Output operations can fail for various reasons, including stream issues or improper data types. Consider the following code snippet that checks for potential output stream errors:
if (!std::cout) {
std::cerr << "Error outputting to stream!" << std::endl;
}
This check ensures that the program does not silently fail, allowing for better debugging and stability.
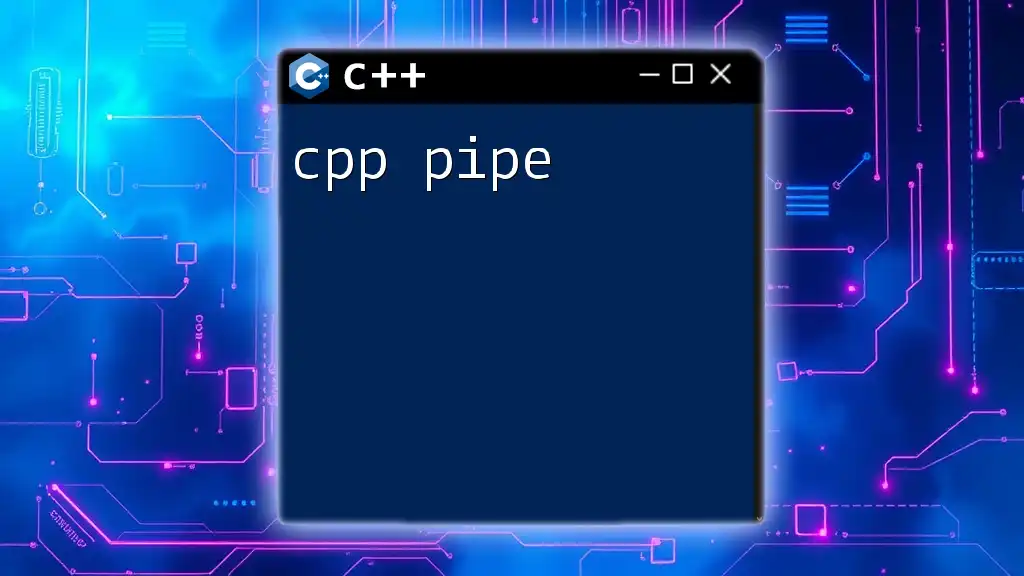
Piping with Custom Classes
Creating Custom Classes for Piping
One advanced application of C++ piping involves creating custom classes that leverage the piping mechanism. By controlling how our classes output data, we can tailor data representation that fits our needs.
Overloading the `<<` Operator
Overloading the `<<` operator is fundamental for enabling our custom classes to participate in piping seamlessly:
class MyClass {
public:
friend std::ostream& operator<<(std::ostream& os, const MyClass& obj) {
os << "Custom Object";
return os;
}
};
With this implementation, instances of `MyClass` can now be piped directly into output streams just like any built-in data type.
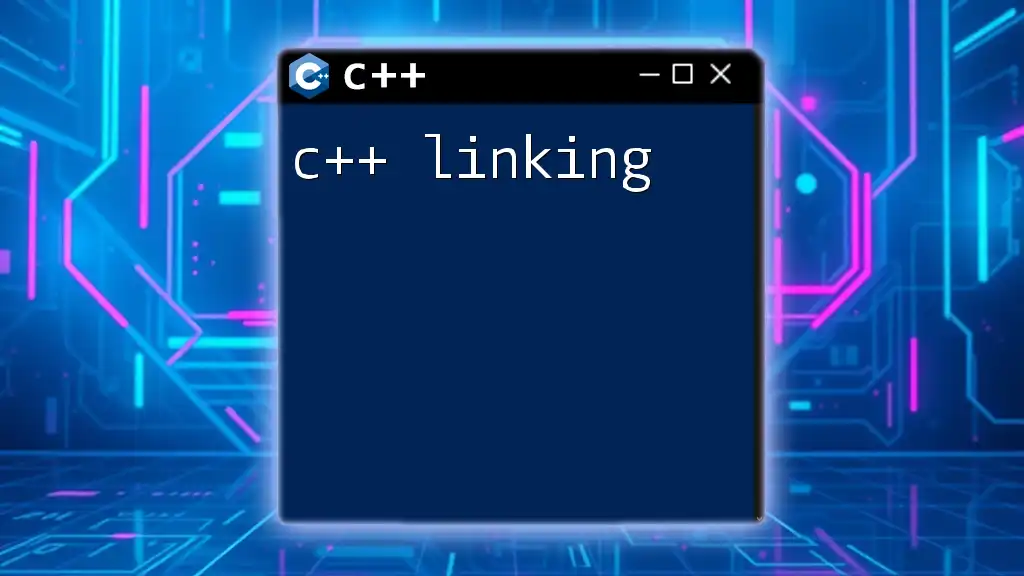
Real-world Applications of Piping
Using Piping in File I/O Operations
One practical application of piping is in file handling. Piping can be used to efficiently read from files while displaying or processing their contents. For instance, consider the following example, which demonstrates reading lines from a file and displaying them via piping:
std::ifstream file("example.txt");
if (file.is_open()) {
std::string line;
while (std::getline(file, line)) {
std::cout << line << std::endl;
}
file.close();
}
This code snippet showcases how easily we can pipe the content of a file directly to the console without creating unnecessary variables.
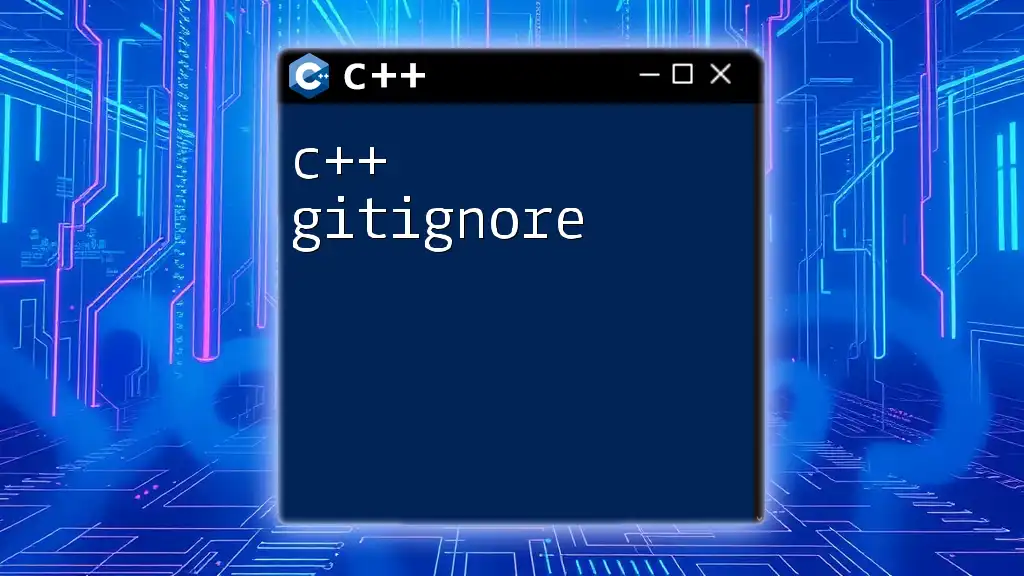
Common Pitfalls and Troubleshooting
Common Mistakes When Using Piping
While piping is powerful, it is essential to be aware of common pitfalls such as data type mismatches, forgetting to include necessary headers, or failing to check if the stream is operational. Being mindful of these mistakes can save significant debugging time.
Debugging Tips for Piped Operations
When encountering issues with piped operations, consider implementing logs or using debugger tools to identify where the data flow breaks down. Reading error messages carefully can also help pinpoint the source of the problem, ensuring a smoother coding experience.
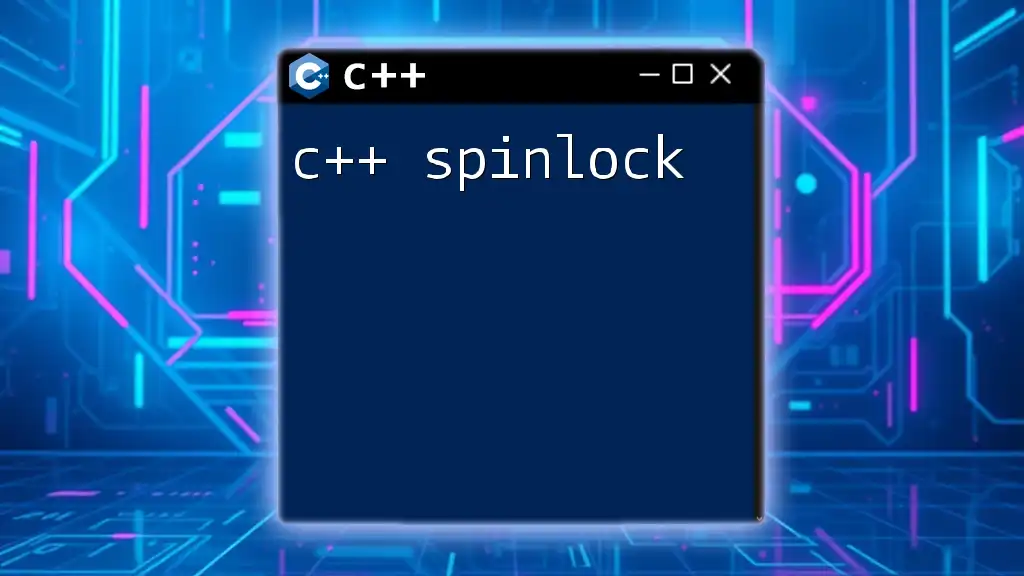
Conclusion
In summary, C++ piping is a valuable feature that enhances how we handle data flow within our applications. By understanding the mechanics behind piping, utilizing the Standard Library effectively, and implementing custom classes for enhanced output control, developers can create clean and efficient data-processing pipelines in their C++ programs. Encourage experimentation with these concepts to elevate your command over C++ and its capabilities.
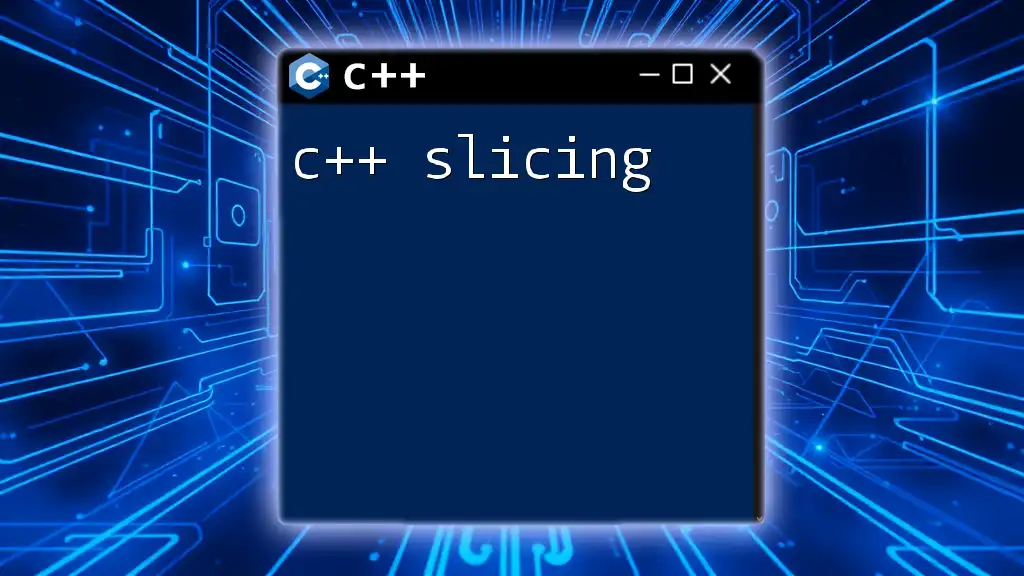
Further Reading and Resources
For a deeper dive into C++ piping and its related concepts, refer to official C++ documentation, tutorials, and books that expand upon these topics. Resources from established programming communities can also provide insights and best practices for effective coding in C++.