C++ principles emphasize the importance of object-oriented programming, encapsulation, inheritance, and polymorphism to create robust and maintainable code.
Here's a simple example demonstrating encapsulation with a class in C++:
class Rectangle {
private:
double width;
double height;
public:
Rectangle(double w, double h) : width(w), height(h) {} // Constructor
double area() {
return width * height; // Method to calculate area
}
};
Fundamental Principles of C++
Encapsulation
Encapsulation is a core concept in object-oriented programming that involves bundling the data (variables) and methods (functions) that operate on the data into a single unit, often in the form of a class. By making variables private, encapsulation restricts direct access to some of an object's components, which is a crucial aspect of data hiding.
This principle provides numerous benefits, including data hiding and easier maintenance of code. It allows programmers to change the internal implementation of a class without affecting the classes that use it.
To illustrate encapsulation, consider the following example of a simple C++ class that implements a bank account:
class BankAccount {
private:
double balance; // encapsulated data
public:
void deposit(double amount) {
if(amount > 0) {
balance += amount;
}
}
double getBalance() const {
return balance;
}
};
In this code, the `balance` variable is private and can only be modified through the `deposit` method. This protects the integrity of the data.
Abstraction
Abstraction refers to the concept of hiding complex realities while exposing only the necessary parts. It helps to manage program complexity by allowing the programmer to focus on interactions at a higher level rather than concerning themselves with the finer details.
In C++, abstraction can be realized through abstract classes and interfaces. An abstract class is a class that cannot be instantiated and typically contains one or more pure virtual functions.
Here is an example that demonstrates abstraction in C++:
class Shape {
public:
virtual void draw() = 0; // pure virtual function
};
class Circle : public Shape {
public:
void draw() override {
// draw a circle
}
};
In this case, the `Shape` class defines a conceptual blueprint for all shapes. Specific shapes like `Circle` implement the actual drawing behavior.
Inheritance
Inheritance is a mechanism whereby a new class, known as a derived class, can inherit attributes and behaviors from an existing class, known as a base class. This promotes code reusability and establishes a natural hierarchy between classes.
There are several types of inheritance in C++, including single inheritance, where a class inherits from one parent class, and multiple inheritance, where a class can inherit from multiple base classes.
Consider the following example demonstrating inheritance:
class Animal {
public:
void eat() {
// implementation for eating behavior
}
};
class Dog : public Animal {
public:
void bark() {
// implementation for barking behavior
}
};
In this case, the `Dog` class inherits the `eat` method from the `Animal` class, allowing it to enact behaviors that are applicable to both animals and specific animals like dogs.
Polymorphism
Polymorphism, derived from the Greek words meaning "many shapes," allows methods to do different things based on the object that it is acting upon. In C++, polymorphism is primarily realized through function overloading and operator overloading, as well as through virtual functions.
There are two main types of polymorphism:
- Compile-time polymorphism: Handled by function overloading and operator overloading.
- Runtime polymorphism: Achieved through the use of virtual functions.
Here's an example demonstrating runtime polymorphism through the use of virtual functions:
class Base {
public:
virtual void show() {
std::cout << "Base class show function called." << std::endl;
}
};
class Derived : public Base {
public:
void show() override {
std::cout << "Derived class show function called." << std::endl;
}
};
In this instance, the `show` method can behave differently depending on whether it is called on a base class or derived class object, thus exemplifying polymorphism.

Additional Principles and Best Practices
DRY Principle (Don't Repeat Yourself)
The DRY principle emphasizes that every piece of knowledge should have a single, unambiguous representation within a system. This principle discourages redundancy, thereby making the code easier to maintain and modify.
For example, rather than duplicating code to calculate employee salaries in several places, you should create a single method that can be reused:
class Employee {
public:
double calculateSalary(double hoursWorked, double hourlyRate) {
return hoursWorked * hourlyRate;
}
};
By utilizing the DRY principle, any changes to the salary calculation need only be made in one place, enhancing maintainability.
KISS Principle (Keep It Simple, Stupid)
The KISS principle encourages simplicity in design and coding practices. By keeping solutions straightforward, programmers can reduce the risk of introducing bugs and enhance code readability.
Consider a simple function to calculate the area of a rectangle:
double calculateArea(double width, double height) {
return width * height;
}
Instead of complicating the calculation with unnecessary parameters or configuration options, this function remains effective and clear.
SOLID Principles
The SOLID principles are a set of five design principles that can improve software design and make it more understandable, flexible, and maintainable. Here's a brief overview of each:
-
Single Responsibility Principle: A class should have one, and only one, reason to change. This allows for better cohesion within classes.
-
Open/Closed Principle: Classes should be open for extension but closed for modification. This can often be achieved with abstract classes and interfaces.
-
Liskov Substitution Principle: Objects of a superclass should be replaceable with objects of a subclass without affecting the correctness of the program. This allows for flexibility in code.
-
Interface Segregation Principle: Clients should not be forced to depend on interfaces they do not use. Smaller, more specific interfaces are better than larger, general-purpose ones.
-
Dependency Inversion Principle: High-level modules should not depend on low-level modules. Both should depend on abstractions. This promotes loose coupling between components.
Each of these principles encourages a design that is more robust and easier to understand.
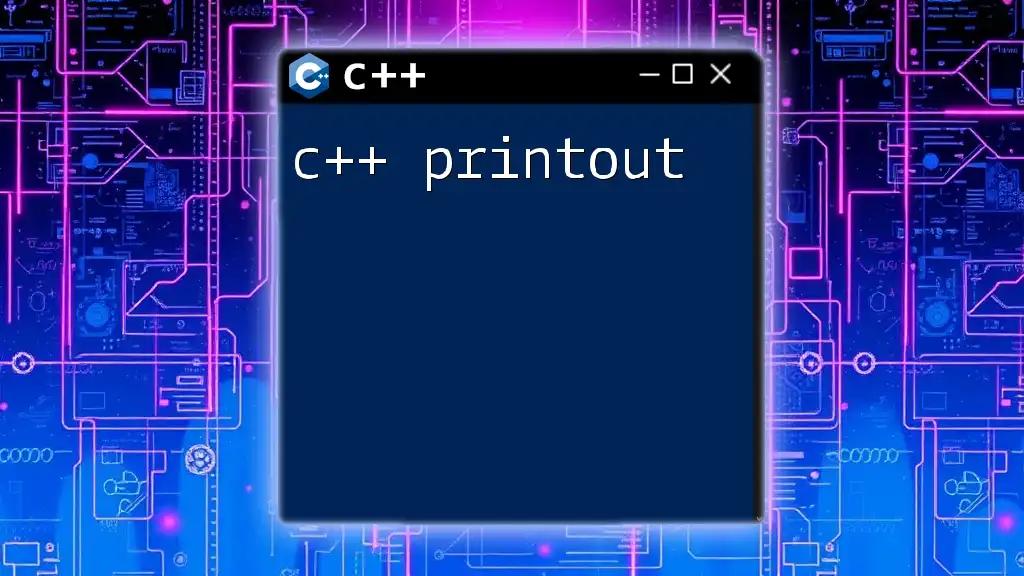
Conclusion
In summary, understanding C++ principles like encapsulation, abstraction, inheritance, and polymorphism, along with best practices such as DRY, KISS, and SOLID, forms the foundation of effective C++ programming. Mastering these principles can significantly enhance your coding skills and lead to better software design.
By grasping these core concepts, you can create more manageable, efficient, and scalable applications. As you continue your journey in learning C++, remember that consistent practice and exploration of these principles will lead to your growth as a proficient programmer.