In C++, a circle can be represented using a class that encapsulates radius and methods for calculating its area and circumference. Here’s a simple example:
#include <iostream>
#include <cmath>
class Circle {
public:
Circle(double r) : radius(r) {}
double area() {
return M_PI * radius * radius;
}
double circumference() {
return 2 * M_PI * radius;
}
private:
double radius;
};
int main() {
Circle c(5.0);
std::cout << "Area: " << c.area() << "\n";
std::cout << "Circumference: " << c.circumference() << "\n";
return 0;
}
What is C++?
C++ is a powerful, high-level programming language that is widely used for system/software development, game programming, and much more. It contains features from both high-level and low-level languages and provides a rich ground for object-oriented programming (OOP). Learning C++ equips you with a robust toolset for developing complex applications, enabling efficient memory management, and concrete object manipulation.
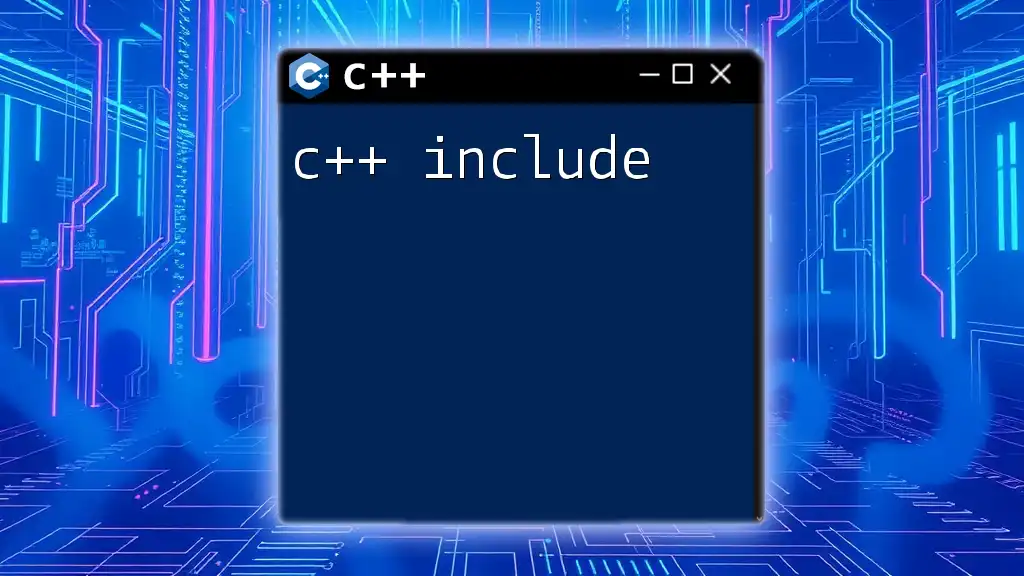
Importance of Geometry in Programming
Geometry serves a critical role in computer science. Real-world applications of geometry include graphics, simulations, and data visualization, where geometric forms like circles are essential. In C++, we often utilize geometric constructs to solve problems, illustrate concepts, and create software that has real-life relevance, such as computer-aided design (CAD) software or simulation frameworks in physics.
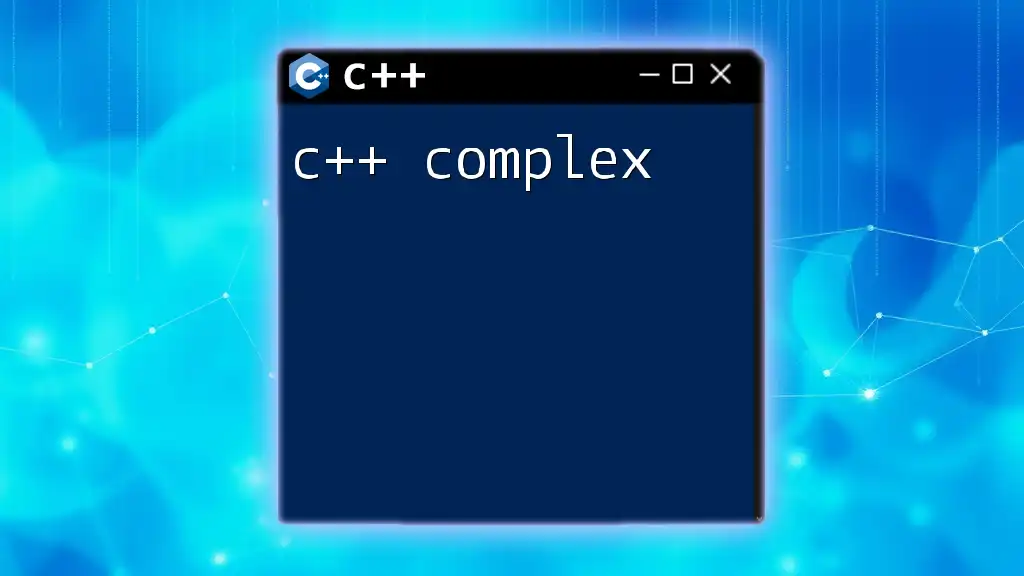
Understanding Circles
Definition of a Circle
A circle is defined mathematically as the set of all points in a plane that are at a given distance, known as the radius, from a fixed point called the center.
Key properties of circles include:
- Radius: The constant distance from the center to any point on the circle.
- Diameter: The distance across the circle through the center, equal to twice the radius.
- Circumference: The distance around the circle, calculated using the radius.
Circle Formulas
Understanding the formulas related to circles is crucial for implementing a circle class in C++. The two main formulas are:
-
Area of a Circle: \[ A = \pi r^2 \]
-
Circumference of a Circle: \[ C = 2\pi r \]
The relationship between radius, diameter, and circumference can be expressed as:
- Diameter = 2 × Radius
- Circumference = π × Diameter
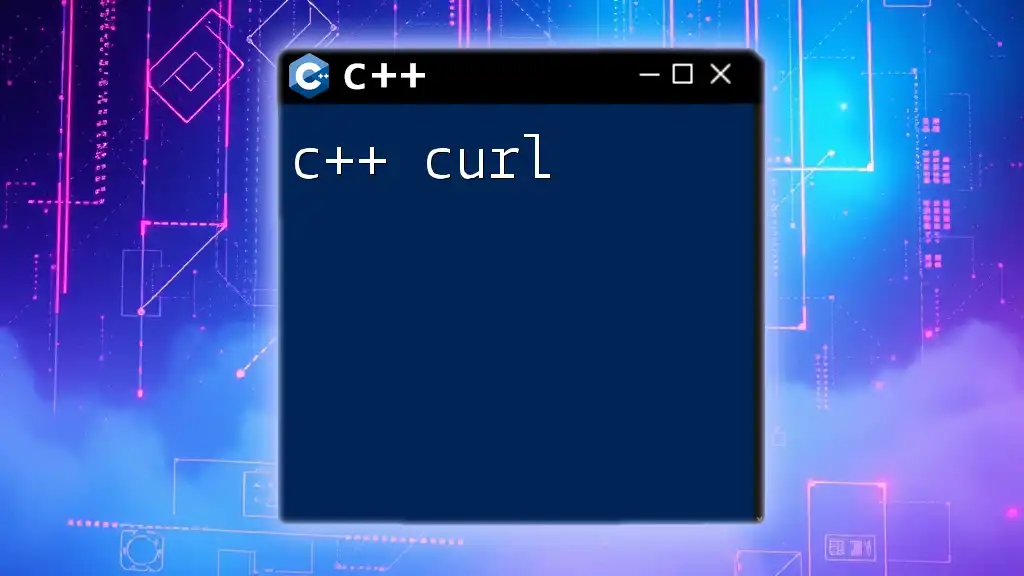
Setting Up Your C++ Environment
Required Tools
Before diving into coding, you need to have the right environment set up. Choosing an IDE (Integrated Development Environment) such as Visual Studio, Code::Blocks, or CLion is essential for writing and executing your C++ code effectively.
Installing a C++ compiler (like GCC for Linux or MinGW for Windows) is also crucial to translate your source code into executable programs.
Creating Your First C++ Project
Once you have the IDE and compiler set up, creating your first C++ project is straightforward:
- Open your IDE and create a new project.
- Select C++ as the programming language.
- Write your first C++ program, which might look something like this:
#include <iostream>
int main() {
std::cout << "Hello, C++ World!" << std::endl;
return 0;
}
- Compile and run the code to see the output in the console.
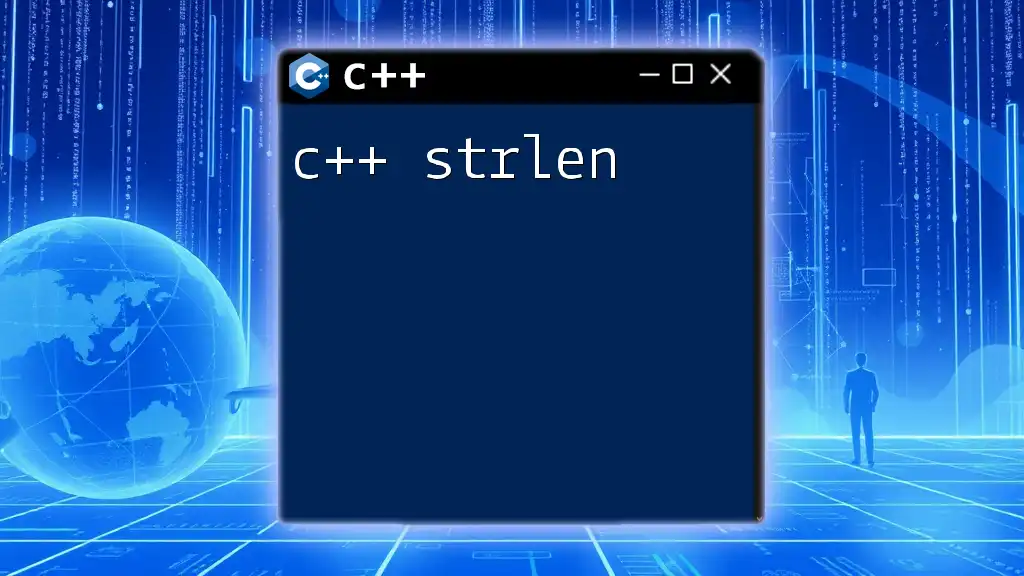
Implementing Circles in C++
Creating a Circle Class
To illustrate geometric concepts in our program, we start by creating a Circle class in C++. This class will encapsulate all properties and behaviors associated with circles.
Class Definition and Attributes
Attributes include:
- `float radius`: The radius of the circle.
The class definition might look as follows:
class Circle {
public:
float radius;
Circle(float r) : radius(r) {}
float area() {
return 3.14159 * radius * radius;
}
float circumference() {
return 2 * 3.14159 * radius;
}
};
Methods of the Circle Class
Calculating Area
The formula for calculating the area of a circle is embedded in the `area` method of the class. This method utilizes the property `radius` to compute the area, reinforcing the mathematical concepts learned earlier.
To implement the Area Method, use:
float Circle::area() {
return 3.14159 * radius * radius;
}
Calculating Circumference
Similar to area, we can calculate the circumference within the Circle class. Implementing the Circumference Method involves:
float Circle::circumference() {
return 2 * 3.14159 * radius;
}
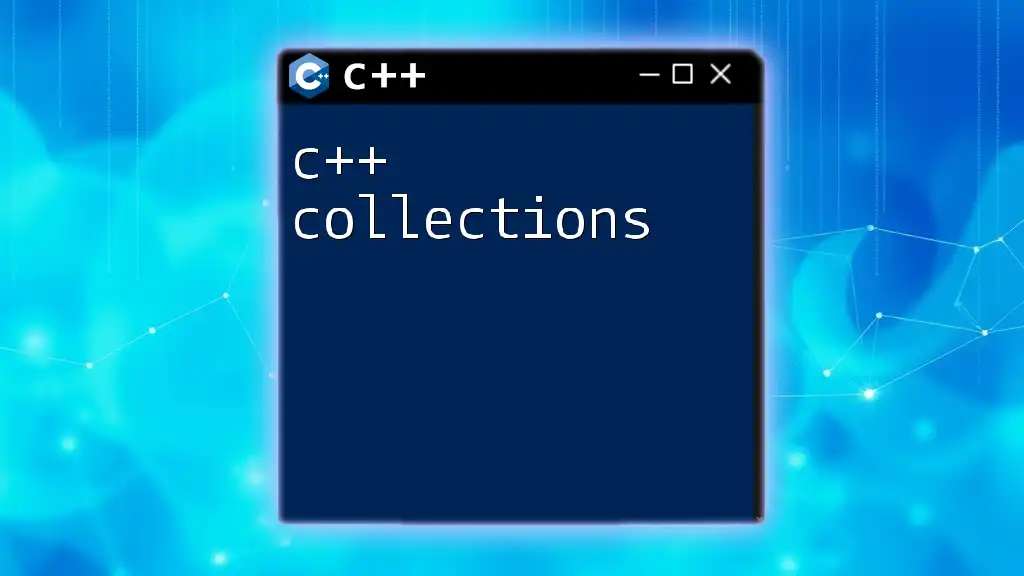
Utilizing the Circle Class
Creating and Using Circle Objects
With the Circle class defined, the next step is to create and utilize circle objects effectively. You can instantiate the Circle class and call its methods to perform calculations.
Here’s an example that demonstrates Object Creation and Method Calls:
int main() {
Circle circle1(5.0);
std::cout << "Area: " << circle1.area() << std::endl;
std::cout << "Circumference: " << circle1.circumference() << std::endl;
return 0;
}
Handling Edge Cases
To ensure your program operates efficiently and accurately, consider handling edge cases, such as negative radius inputs. We can use assertions to validate inputs in our constructor.
Here's how to implement it:
Circle(float r) {
assert(r >= 0); // Ensures radius is non-negative
radius = r;
}
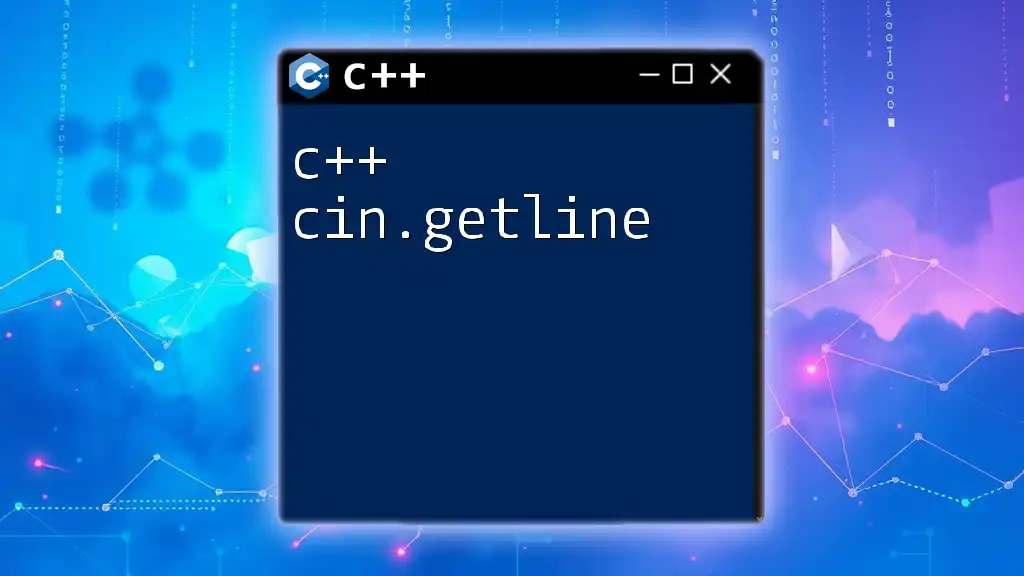
Advanced Circle Operations
Circle Intersection
In advanced geometry, understanding circle intersection can be essential. Implementing the mathematical background can be complex, but it is straightforward with the right approach. For example, written as a method, you can compute intersection points given the equations of two circles.
Circle Movement Animation in C++
For graphics-intensive applications, you might want to animate circles. Utilizing graphics libraries such as SFML or SDL is a great way to bring circles to life in a visual format. Here’s a simplified example of initiating a moving circle using SFML:
#include <SFML/Graphics.hpp>
int main() {
sf::RenderWindow window(sf::VideoMode(800, 600), "Circle Animation");
sf::CircleShape circle(50);
circle.setFillColor(sf::Color::Green);
while (window.isOpen()) {
sf::Event event;
while (window.pollEvent(event)) {
if (event.type == sf::Event::Closed)
window.close();
}
window.clear();
circle.move(1, 0); // Move the circle horizontally
window.draw(circle);
window.display();
}
return 0;
}
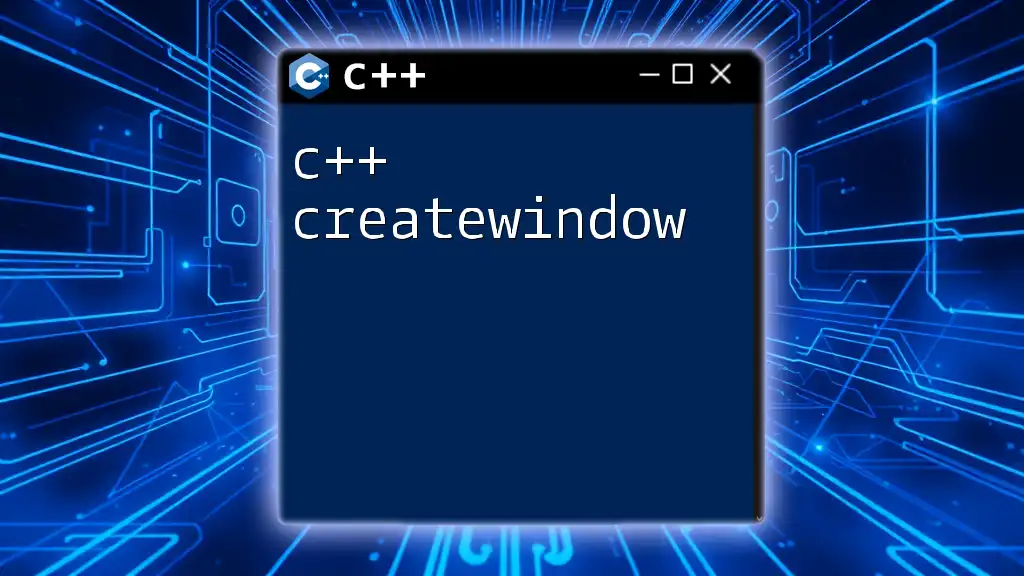
Conclusion
Recap of What We've Learned
In this guide, we delved deeply into the concept of a C++ circle, examining its mathematical properties, how to implement a Circle class, and how to utilize circle objects in C++. We also touched on advanced functionality such as circle intersection logic and animation.
Further Learning Resources
To enhance your understanding of C++, consider exploring additional books, online courses, and participating in C++ community forums. These resources can provide deeper insights and further your learning journey.
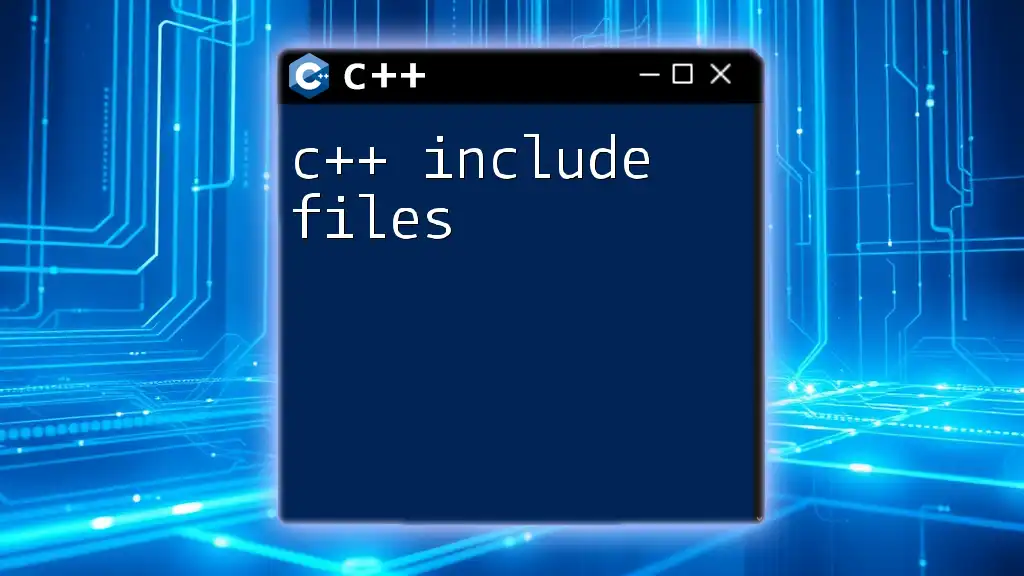
Frequently Asked Questions (FAQs)
What is the difference between radius and diameter?
- The radius is the distance from the center of the circle to any point on its boundary, while the diameter is twice the radius and spans the entire width through the center.
Why use classes in C++?
- Classes in C++ support encapsulation, facilitating better software design by grouping related functions and data together, enabling code reusability.
How can I handle multiple circle instances?
- Create multiple objects from the Circle class, as each object can maintain its own state and encapsulate its properties.
What graphics libraries can I use for C++?
- You can use libraries like SFML, SDL, or OpenGL for developing graphical applications in C++. Each has its strengths depending on your project requirements.