In C++, the include path is a set of directories that the compiler searches to find header files when you use the `#include` directive, allowing you to incorporate standard or user-defined libraries into your program.
Here's a code snippet demonstrating how to include a standard library header:
#include <iostream>
You can also include a custom header from your project directory like this:
#include "myHeaderFile.h"
Understanding the C++ Preprocessor
The C++ preprocessor plays a crucial role in the compilation process. It is responsible for transforming the code before it is compiled into machine language. One of its primary functions is handling include directives, allowing developers to manage dependencies in their programs efficiently. By including files, developers can modularize their code, making it reusable and easier to maintain.
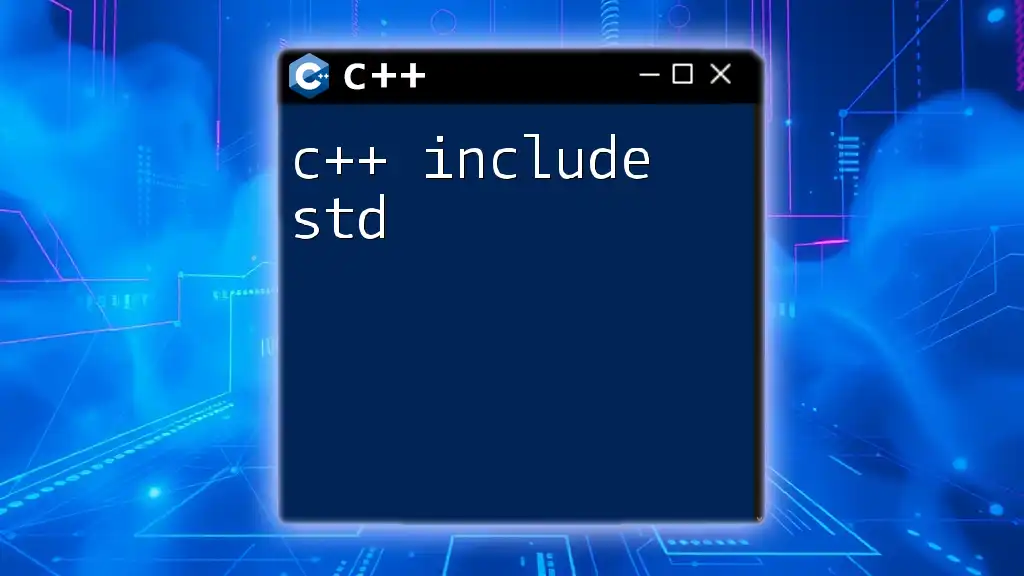
The Basics of Include Directives
What are Include Directives?
The `#include` directive is a preprocessor command used to include the contents of one file into another. This is essential in C++ for incorporating libraries, headers, and user-defined files required by the program. There are two main types of include directives:
-
Angle Bracket Syntax: Used to include system or standard library headers. Example:
#include <iostream>
-
Double Quote Syntax: Used for user-defined or custom header files. Example:
#include "myheader.h"
The choice between these two syntaxes depends on where the compiler should look for the files. Angle brackets indicate that the compiler should search the standard library paths, while double quotes instruct it to look in the current directory first.
Syntax of Include Directives
To include files in your C++ program, the syntax remains straightforward:
#include <library_name> // For standard libraries
#include "header_name.h" // For user-defined headers
It's vital to use the correct syntax to avoid compilation errors stemming from incorrect paths.
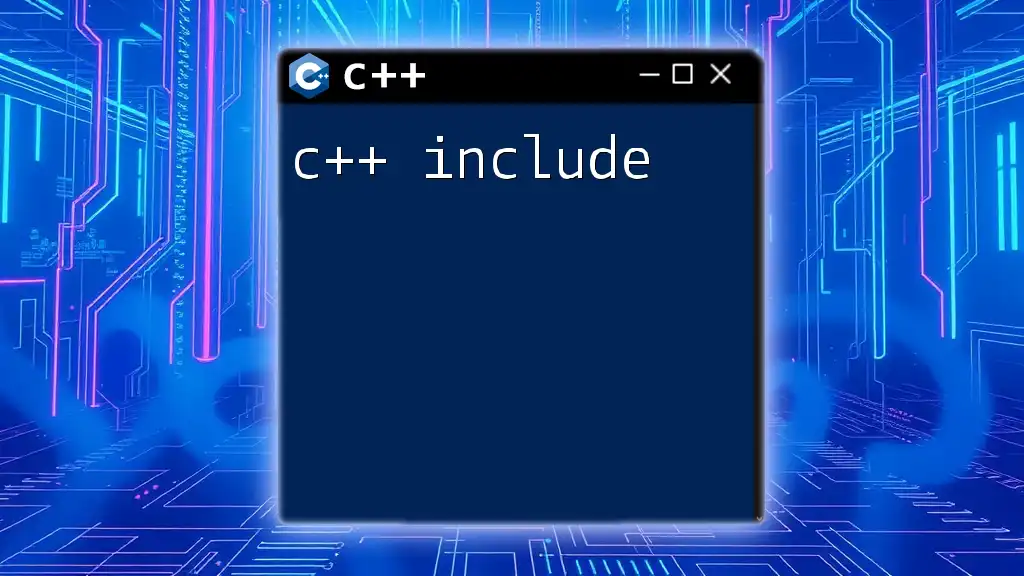
Default Include Paths in C++
Standard Include Paths
When you compile a C++ program, the compiler has default paths where it searches for standard headers. These paths depend on the installation of your C++ compiler. For example, on a typical installation of GCC, you might find standard headers in directories like `/usr/include` or `/usr/local/include`.
User-Defined Include Paths
In a project, you may often need to include files that are not in the standard directories. By adding user-defined include paths, you can instruct the compiler to search specific directories for headers needed in your project. This flexibility allows developers to structure their projects efficiently.
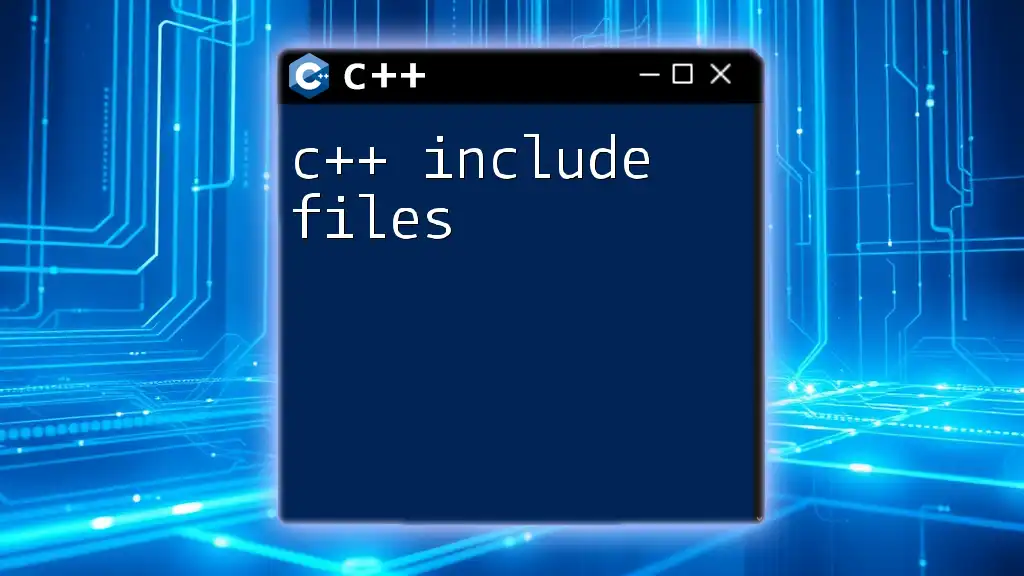
Configuring the Include Path
Using Compiler Options
Adding Include Paths with g++
For GCC-based compilers, you can add custom include paths using the `-I` option in your compile command. This option allows the compiler to search additional directories. Here’s how you can do it:
g++ -I/path/to/my/includes main.cpp
In this command, `/path/to/my/includes` is the directory where your custom headers are located. You can add multiple `-I` options to include various paths.
Adding Include Paths with Visual Studio
If you are using Visual Studio, the process to set include paths is user-friendly:
- Open Project Properties in your C++ project.
- Navigate to C/C++ -> General.
- Modify the Additional Include Directories field to add your custom paths.
This graphical interface allows you to quickly update your project settings without delving into command-line options.
Setting Up Environment Variables
Environment variables are another powerful method for managing include paths. By setting an environment variable, you can make paths accessible system-wide or to specific projects.
On Windows, you can set an environment variable as follows:
- Right-click on This PC or My Computer and select Properties.
- Click on Advanced system settings.
- Under the System Properties window, click Environment Variables.
- Add a new variable under User variables or System variables with the name `CPLUS_INCLUDE_PATH` and the value as the path to your include directory.
On UNIX-based systems, you can export the variable in your terminal session:
export CPLUS_INCLUDE_PATH=/path/to/your/includes
This method allows the compiler to automatically use the specified paths during compilation.
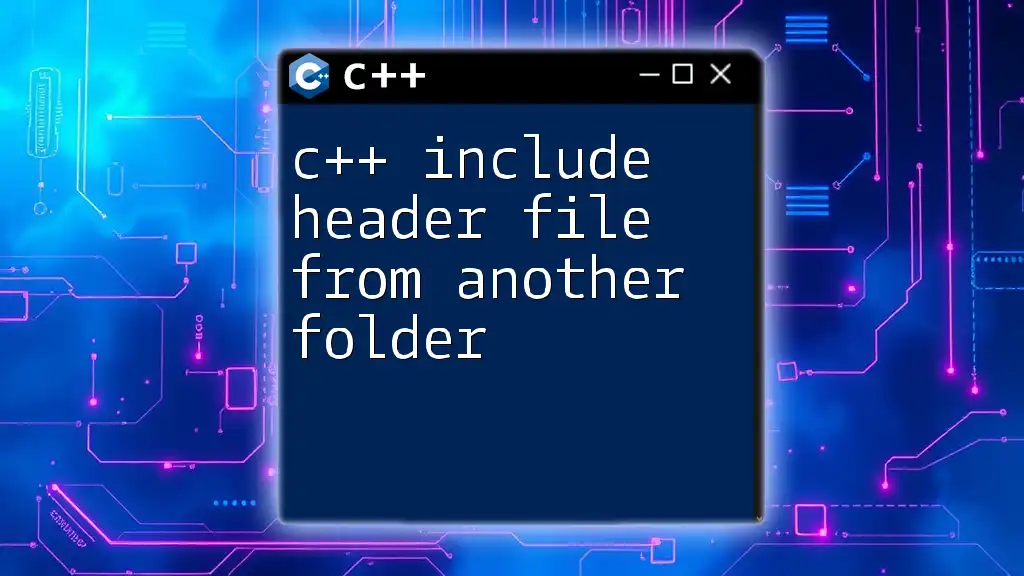
Managing Include Guards
What are Include Guards?
Include guards are a vital convention in C++ programming that prevents multiple inclusions of the same header file within a single translation unit. When a header file is included more than once, it can lead to errors and increased compile time. Include guards ensure that the declarations within a header file are processed only once.
Implementing Include Guards
To implement include guards, wrap the entire content of your header file with preprocessor directives:
#ifndef MYHEADER_H
#define MYHEADER_H
// Declarations, functions, and classes
#endif // MYHEADER_H
In this code, `#ifndef MYHEADER_H` checks if `MYHEADER_H` has not been defined. If it has not been defined, it processes the code between the `#define MYHEADER_H` and `#endif`. This simple technique significantly improves code organization and efficiency.
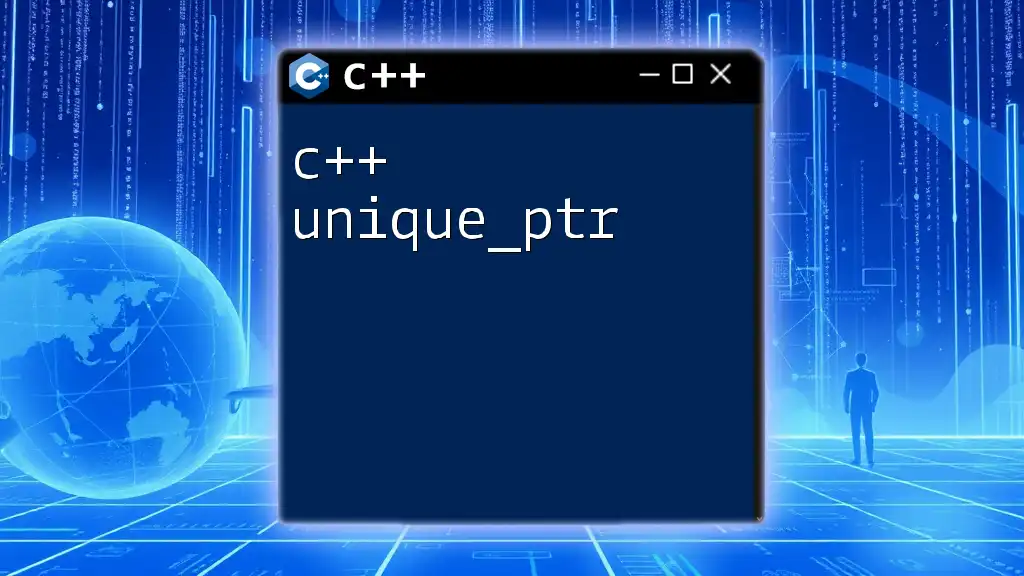
Common Issues with Include Paths
Troubleshooting Include Path Problems
When working with include paths, you may encounter problems, such as the compiler not finding the expected files. Common issues include:
-
File not found errors: These often arise from incorrect paths in the include statements or from forgetting to specify additional include directories.
-
Version conflicts: Sometimes different versions of libraries may exist on your filesystem. Ensure you're pointing to the correct version to avoid incompatibilities.
Best Practices for Organizing Include Files
To maintain a clean and manageable codebase, consider the following best practices:
-
Logical Directory Structure: Organize header files in logical directories based on functionality or module. This makes it easier to locate and manage files.
-
Keep User Headers Separate: Maintain a clear distinction between standard library headers and user-defined headers to avoid confusion.
-
Minimize Dependencies: Try not to include too many headers unnecessarily. Only include what you need to reduce compilation time and complexity.
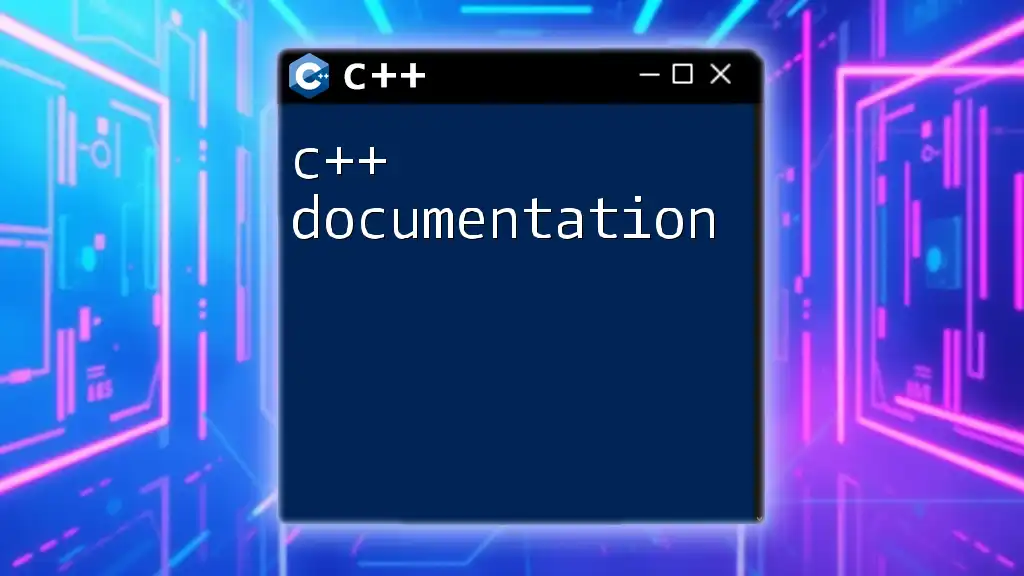
Conclusion
Understanding and configuring the C++ include path is essential for effective C++ programming. Mastering include directives, managing include paths, and implementing include guards will lead to more efficient and modular code. By following best practices and resolving common issues, you can streamline your development process, allowing you to focus on building robust applications.
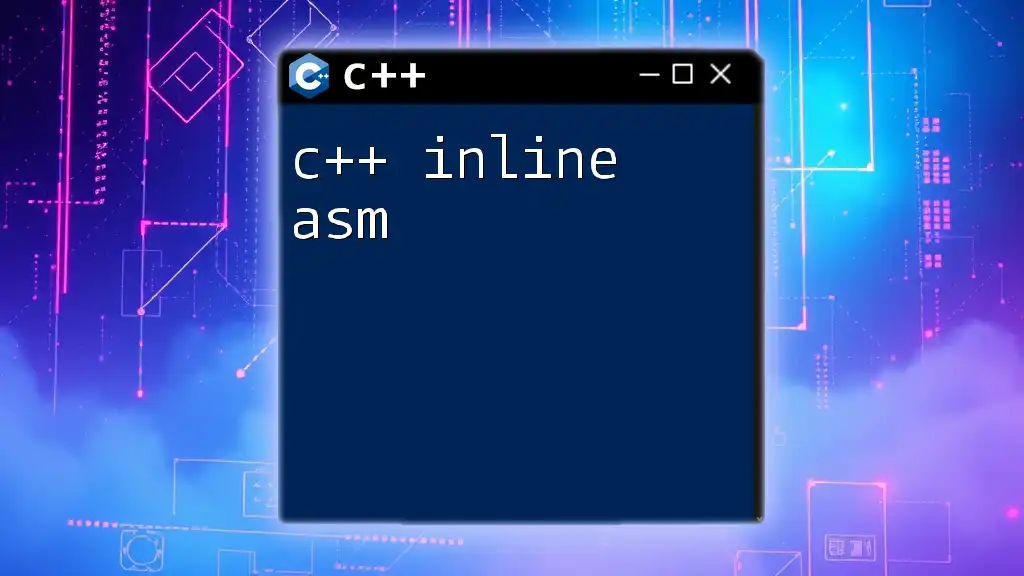
Additional Resources
To further enhance your skills, refer to official documentation, dedicated forums, and advanced C++ courses that dive deeper into the intricacies of C++. Learning is a journey, and mastery of the C++ include path can significantly elevate your programming proficiency.