In C++, an index typically refers to the position of an element within an array or a similar data structure, allowing you to access specific elements efficiently.
Here’s a simple code snippet demonstrating how to access an element in an array using an index:
#include <iostream>
int main() {
int arr[] = {10, 20, 30, 40, 50};
int index = 2; // Accessing the third element
std::cout << "Element at index " << index << " is " << arr[index] << std::endl; // Output: 30
return 0;
}
What is Indexing in C++?
Indexing in C++ refers to the method of accessing elements within data structures using an integer index. It's a fundamental concept that enables programmers to retrieve, modify, and manipulate data efficiently. Understanding how to utilize indexing properly can significantly enhance the performance and functionality of any C++ application.
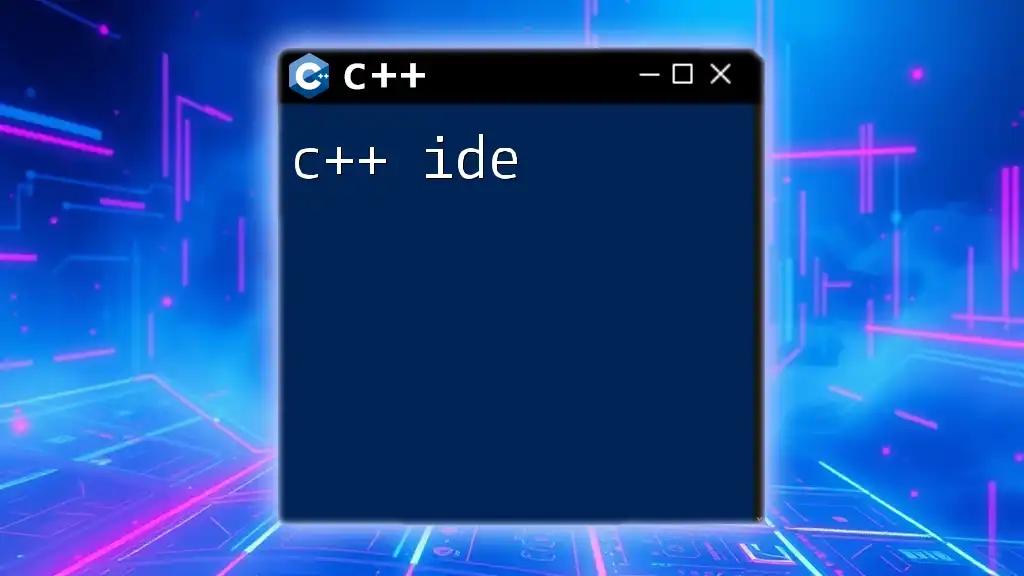
Why C++ Indexing Matters
The importance of indexing in C++ can't be overstated. It allows for rapid access to elements within data structures, making operations such as searching and sorting much more efficient. In real-world applications—from databases to game development—effective indexing can lead to optimized resource management and faster data retrieval.
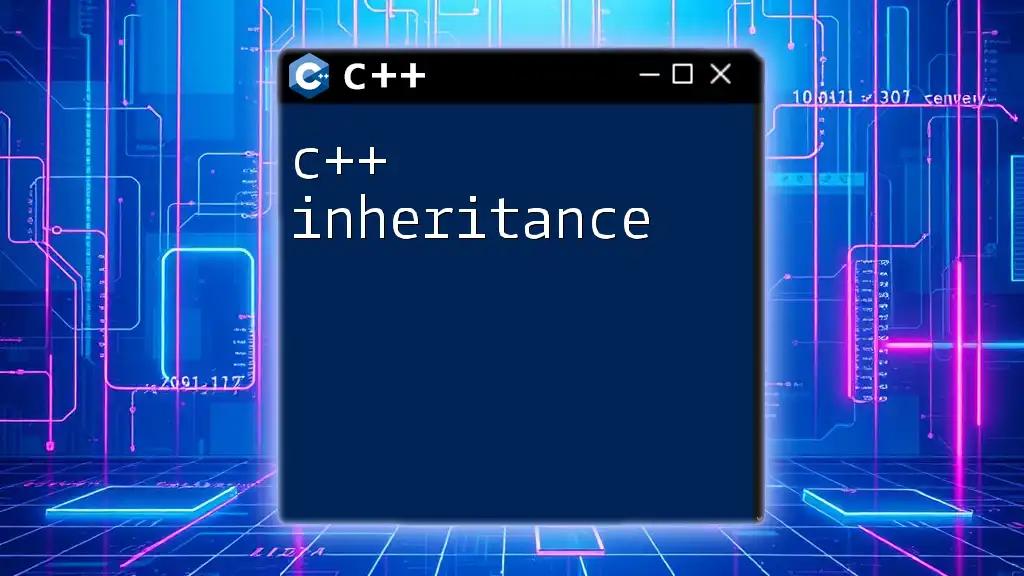
Understanding C++ Data Structures
Key Data Structures
In C++, several data structures benefit from indexing, each serving distinct purposes:
- Arrays offer a fixed size collection of elements.
- Strings handle sequences of characters efficiently.
- Vectors provide dynamic sizing for collections of items.
- Maps facilitate key-value pair storage, allowing for quick lookups.
Understanding these structures is crucial for making deliberate choices about how to index your data effectively.
Memory Management Considerations
Indexing also interacts closely with memory management in C++. Each data structure has its own way of allocating memory, which can directly impact how indexing works. For example, arrays are stored in contiguous memory locations, while vectors may dynamically allocate more space as they grow.
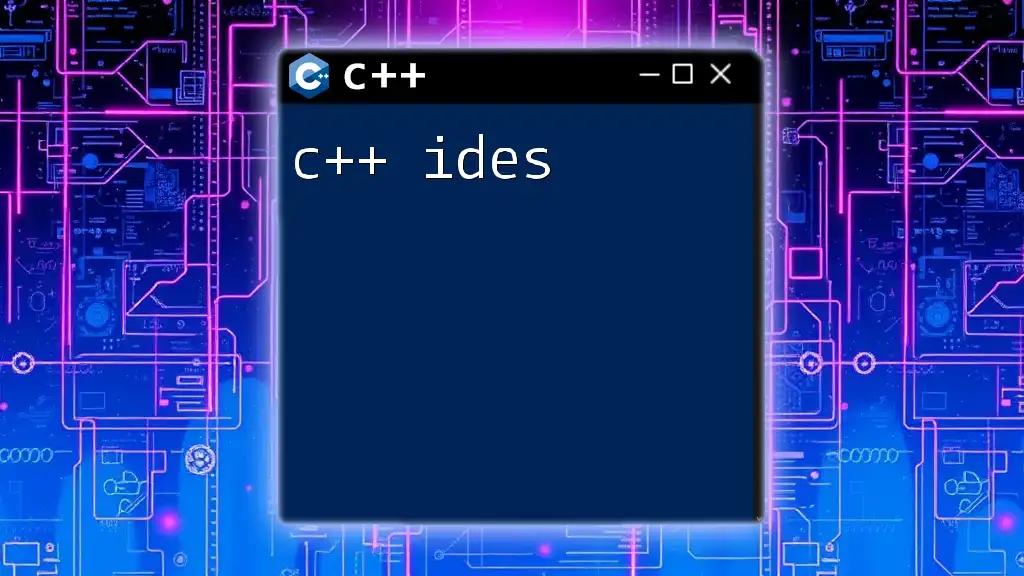
Arrays and Indexing in C++
What are Arrays?
Arrays are among the simplest data structures in C++, providing a collection of elements of the same type. They can be single-dimensional or multi-dimensional, each accessing elements via an integer index that starts from zero.
Indexing in Arrays
Array indexing allows for direct access to its elements. Here's how it works:
int arr[5] = {1, 2, 3, 4, 5};
// Access elements
std::cout << "Element at index 0: " << arr[0] << std::endl; // Output: 1
In this example, `arr[0]` retrieves the first element of the array. It's crucial to remember that accessing an index outside the declared size of the array can result in undefined behavior.
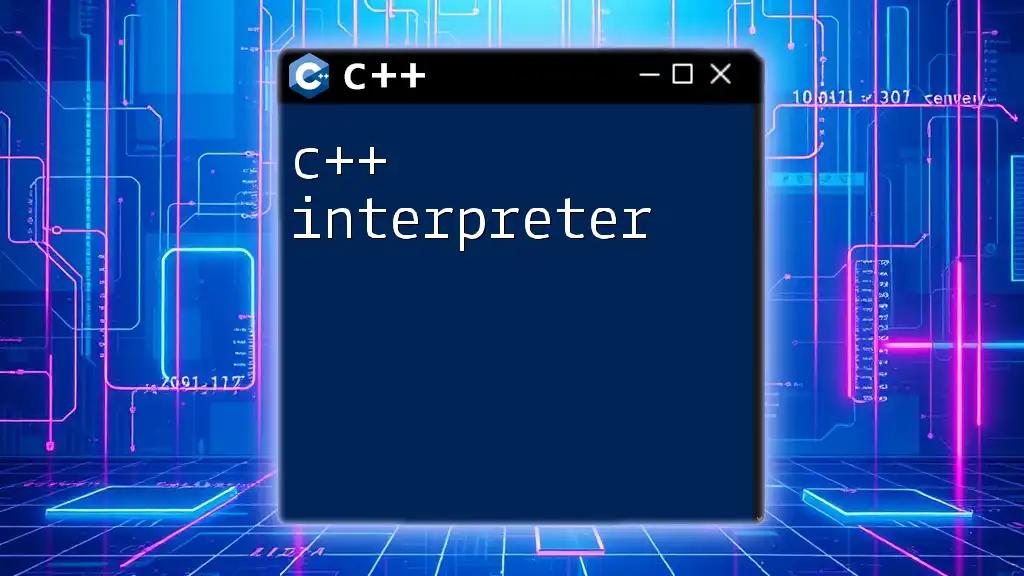
Strings and Indexing in C++
Understanding C++ Strings
C++ strings can be represented by C-style character arrays or as `std::string` objects. The latter is preferred for its ease of use and extensive functionality.
Accessing Characters via Indexing
Just like arrays, strings can be indexed to access individual characters:
std::string str = "Hello";
std::cout << "Character at index 1: " << str[1] << std::endl; // Output: e
In this case, `str[1]` retrieves the second character of the string. Understanding this is vital for string manipulation tasks.

Vectors and Indexing in C++
Introduction to Vectors
Vectors are dynamic arrays, automatically managing their size. This adaptability allows for efficient data handling in applications where the size of the dataset is unknown at compile time.
Indexing with Vectors
You can access vector elements just as you would with an array:
std::vector<int> vec = {10, 20, 30};
std::cout << "Element at index 2: " << vec[2] << std::endl; // Output: 30
This code demonstrates how straightforward it is to access elements in a vector, giving programmers flexibility and control over their data.

Maps and Indexing in C++
What are Maps?
Maps are associative containers that store elements formed by a combination of key-value pairs. This structure allows for fast retrieval based on a key, making it essential in many applications.
Accessing Values using Keys
Using maps, indexing is performed through keys rather than numeric indices:
std::map<std::string, int> age;
age["Alice"] = 25;
std::cout << "Alice's age: " << age["Alice"] << std::endl; // Output: 25
Here, `age["Alice"]` accesses the value associated with the key "Alice," highlighting how maps use indexing differently from arrays.

C++ Indexing Techniques
Privileges and Limitations of Indexing
Benefits of Indexing include:
- Speed of Access: Indexing allows for rapid data retrieval.
- Ease of Use: Indexing makes code intuitive and simplifies data handling.
However, Limitations include potential out-of-bounds errors and performance issues, especially in large datasets or with inefficient data structures.
Choosing the Right Data Structure
Each data structure has its strengths and weaknesses. For instance, use arrays for fixed-size collections and maps when you need quick lookups based on keys. Adopting the most suitable data structure is integral to effective indexing.
Algorithmic Indexing Strategies
Understanding algorithms like binary search can enhance indexing efficiency. Binary search relies on sorted data and dramatically reduces the time complexity of finding an element.

Common Issues in C++ Indexing
Out-of-Bounds Access
Out-of-bounds access occurs when trying to access an index not present in the data structure. It can lead to undefined behavior, crashes, or corrupted data.
How to Avoid Out-of-Bounds Errors
Best practices include:
- Always validate indices before accessing elements.
- Use methods like `at()` in vectors and `std::string` to perform bounds checking, which throw exceptions when an invalid index is accessed.
Performance Bottlenecks
Efficient indexing is critical, as poor performance can slow down applications significantly. Indexing issues can arise from:
- Non-optimized data structures.
- Inefficient algorithms that perform excessive calculations.
Optimizing Index Access
Consider using:
- Caching mechanisms to store frequently accessed data.
- Choosing appropriate data structures that reduce access time.
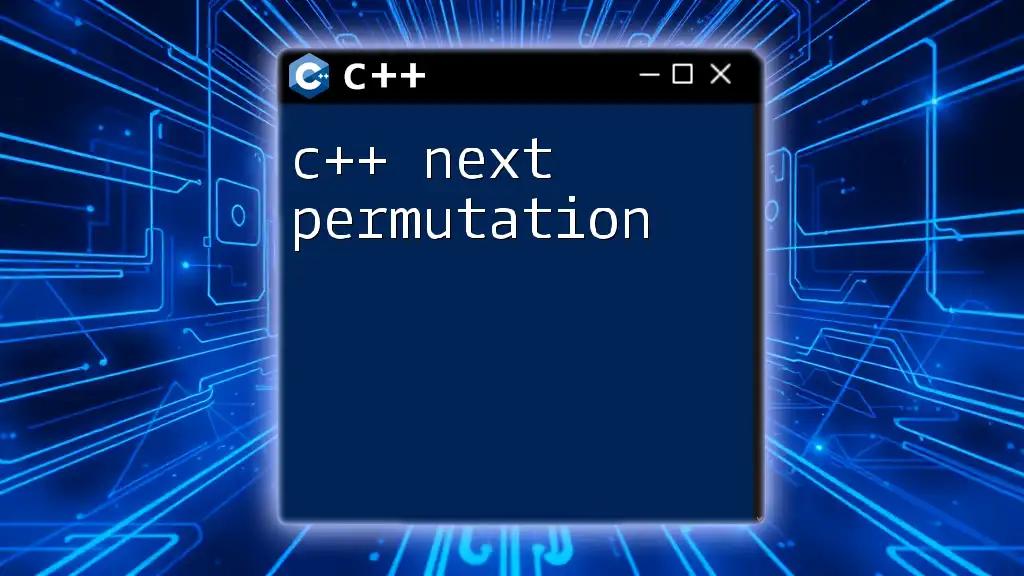
Advanced Concepts in C++ Indexing
Multidimensional Arrays and Indexing
Multidimensional arrays allow for complex data organization, akin to tables or matrices. Accessing elements requires understanding multiple indices:
int matrix[2][3] = {{1, 2, 3}, {4, 5, 6}};
std::cout << "Element at (1, 2): " << matrix[1][2] << std::endl; // Output: 6
This example emphasizes how to access specific elements based on their row and column indices.
Sparse Data Structures and Indexing
Sparse data structures only store non-empty elements, making them ideal for cases where data density is low. Efficient indexing methods in sparse arrays can optimize both memory and performance, maintaining access speed while conserving space.

Conclusion
In summary, the C++ index plays a vital role in determining how effectively you can access and manipulate data within your programs. By grasping the nuances of different data structures, employing efficient indexing strategies, and recognizing potential issues, you can harness the full power of C++ indexing for optimal application performance. As you continue to deepen your understanding, delve into advanced topics and practical coding exercises to solidify your skills.