In C++, an "undefined symbol" error occurs when the linker cannot find the definition for a variable or function that has been declared but not defined, as illustrated in the following example:
#include <iostream>
void myFunction(); // Declaration
int main() {
myFunction(); // Call to undefined function
return 0;
}
// Missing definition of myFunction() leads to an "undefined symbol" error during linking.
Understanding Undefined Symbols in C++
Undefined symbols in C++ refer to identifiers that the linker cannot find a definition for. When you compile a C++ program, it goes through several stages, including preprocessing, compiling, assembling, and linking. The linker is responsible for combining the various pieces of object files into a single executable. If it encounters any symbols that do not have corresponding definitions in the linked files, it raises an undefined symbol error.
Common scenarios that can lead to undefined symbols include:
- Declaring a function in a header file but failing to provide an implementation.
- Forgetting to link against a necessary library.
- Using functions or variables that were meant to be private or scoped within a particular file.
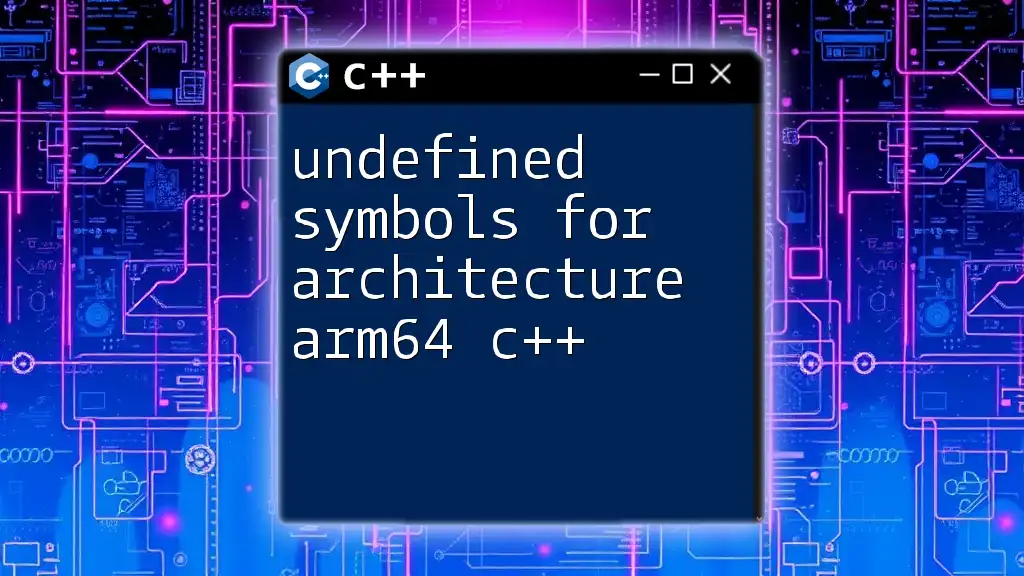
Causes of Undefined Symbol Errors
Linker Issues
The linking process is critical in C++. It combines all the object files generated during the compilation stage into an executable. An undefined symbol error often arises from:
- Missing object files: If a required object file is not included during linking, any symbols defined within that file will be undefined.
- Incorrect order of object files: The order in which object files are specified can also affect symbol resolution.
For instance, if you have two files, `main.cpp` and `utils.cpp`, where `utils.cpp` contains the implementation of a function called `helper()`, the linker needs to see `utils.o` when linking `main.o`.
Missing Function Implementations
One of the most common pitfalls in C++ programming is declaring a function without providing an implementation. This can easily result in an undefined symbol error:
// functions.h
void helper(); // Declaration
// main.cpp
#include "functions.h"
int main() {
helper(); // Calling an undeclared function
return 0;
}
If you compile `main.cpp` without a corresponding `helper()` implementation in `functions.cpp`, you will encounter an undefined symbol error when linking.
Incorrect Namespace Usage
Namespaces in C++ help avoid naming collisions; however, using them incorrectly can lead to undefined symbols. If you declare a function within a namespace but later attempt to call it without qualifying the namespace, the linker will fail to resolve it:
// utils.h
namespace MyNamespace {
void helper();
}
// main.cpp
#include "utils.h"
int main() {
helper(); // Undeclared function
return 0;
}
To resolve this, you must specify the namespace when calling the function:
MyNamespace::helper();
Static and External Linkage
C++ offers static and external linkage which dictate the visibility of variables and functions. If a function is declared as `static`, it can only be used within the file it is defined in. Attempting to call it from another file results in an undefined symbol error:
// file1.cpp
static void helper() {
// Implementation
}
// file2.cpp
void callHelper() {
helper(); // Undefined symbol error
}
In the above example, `helper()` is not accessible from `file2.cpp`, leading to an undefined symbol error.
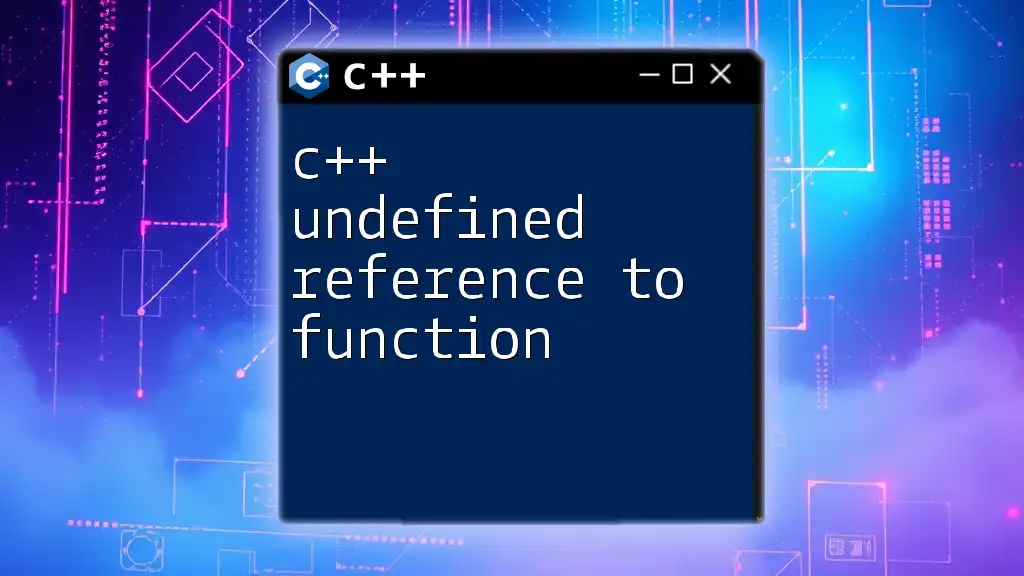
Diagnosing Undefined Symbol Errors
Interpreting Compiler and Linker Error Messages
Understanding compiler and linker error messages is vital. When you receive an undefined symbol error, the message typically includes the name of the symbol and the object files being linked. This information can help pinpoint the source of the issue. For example:
undefined reference to 'helper()'
This indicates that the linker is looking for the definition of `helper()` but cannot find it.
Using Debugging Tools
Debugging tools such as `gdb` and `nm` can help identify undefined symbols.
- Using `nm`: This command lists symbols from object files.
nm main.o
If `helper()` is not listed in the output, you know it isn’t defined in `main.o` or any linked files.
- Using `gdb`: You can run your program within `gdb`, which will provide backtrace and more context around where things go wrong.
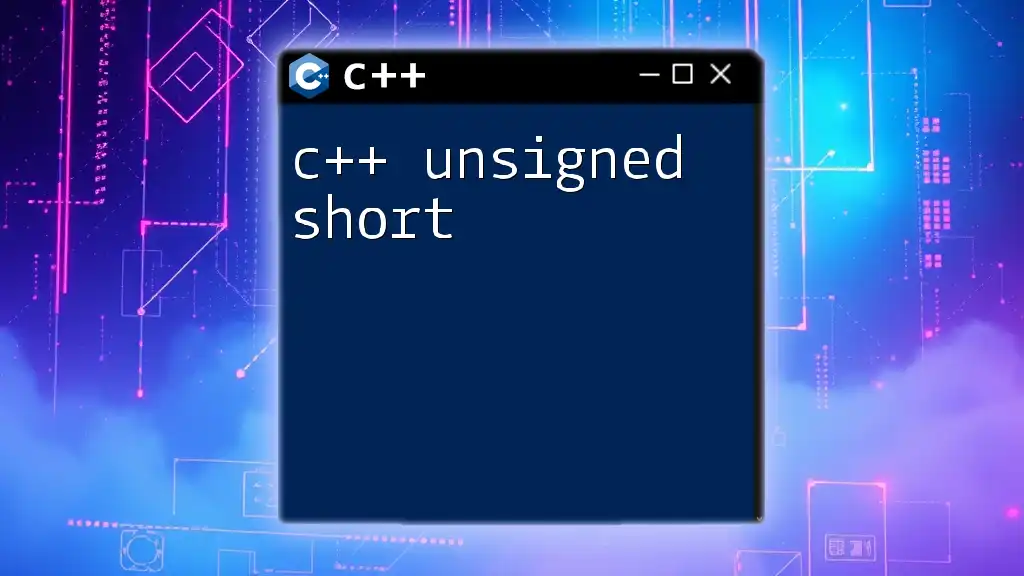
Solutions to Undefined Symbol Errors
Defining Missing Functions
The most straightforward solution is to ensure that all declared functions are defined. Verify that:
// functions.cpp
#include "functions.h"
void helper() {
// Implementation goes here.
}
This definition provides the linker with the implementation it needs.
Correctly Linking Libraries
If your program depends on external libraries, ensure that they are properly linked during compilation. Use:
g++ main.o utils.o -o my_program -lmylib
Ensure `-lmylib` correctly points to the relevant library that contains the required symbols.
Namespace Management
To mitigate issues related to namespace usage, always confirm the namespace context when calling functions or accessing variables. You can also use:
using namespace MyNamespace;
to eliminate the need for repetitive namespace qualification, but use it cautiously to avoid confusion with symbols of the same name.
Using `extern` Keyword
When declaring global variables that need to be shared across multiple files, use the `extern` keyword properly:
// file1.cpp
extern int globalVar;
// file2.cpp
int globalVar = 42; // Definition
This ensures that the variable's visibility is appropriately managed, preventing undefined symbol errors.
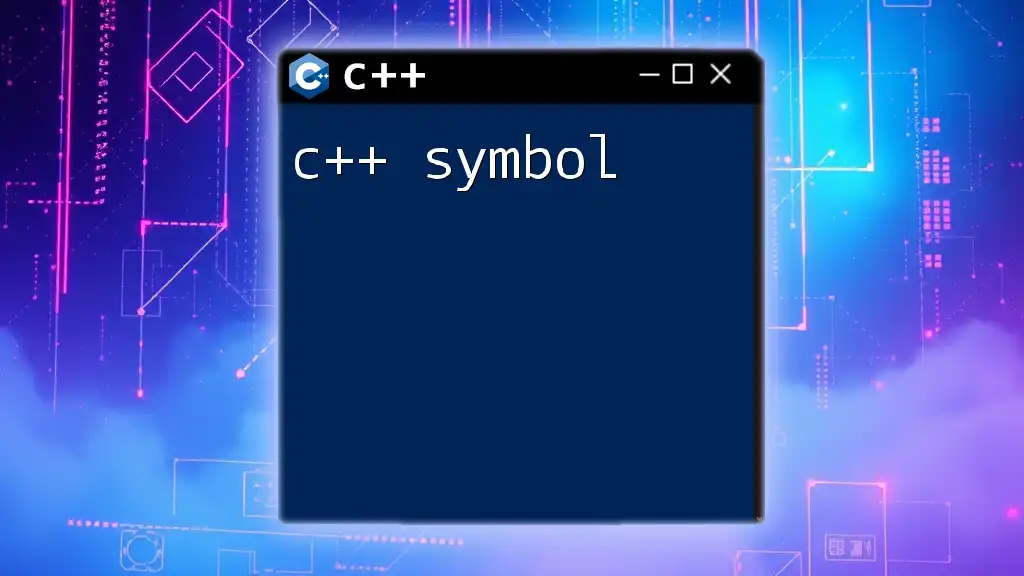
Best Practices to Avoid Undefined Symbol Errors
Consistent Naming Conventions
Adopt consistent naming conventions across your project. This decreases the likelihood of unexpected name collisions that can lead to undefined symbols.
Documentation and Comments
Keep thorough documentation and comments outlining function declarations and implementations. This way, any developer maintaining the code can swiftly identify what needs to be defined.
Unit Testing and Build Automation
Implement unit tests to catch errors early in the development cycle. Additionally, consider using build automation tools like CMake or Makefiles to streamline the build process and reduce human error during compilation and linking.
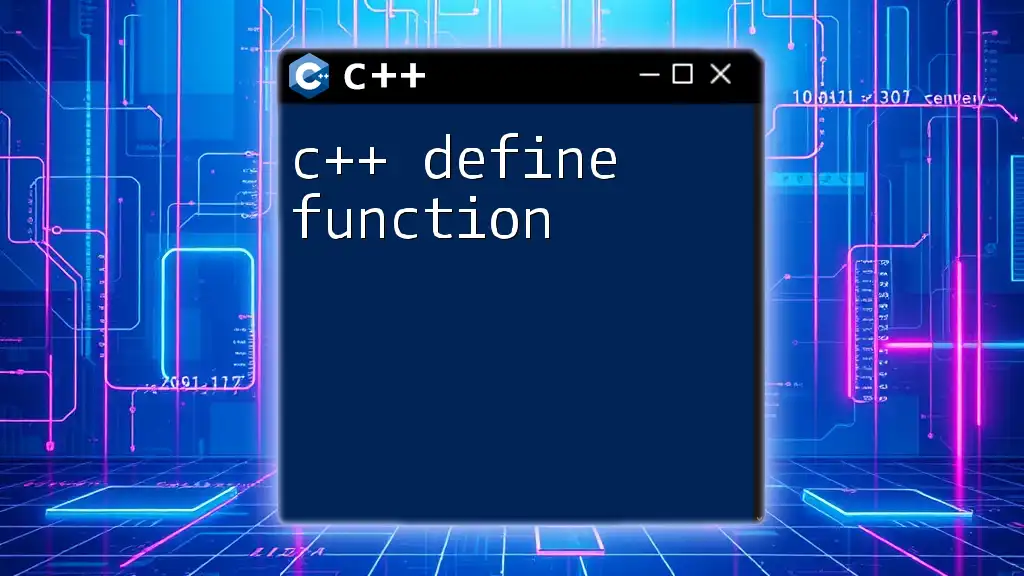
Conclusion
Understanding C++ undefined symbols is crucial for any programming endeavor. It not only helps in debugging but also fosters stronger coding practices. By following the guidelines and best practices outlined in this article, you can efficiently manage and resolve undefined symbol errors for a smoother C++ development experience.
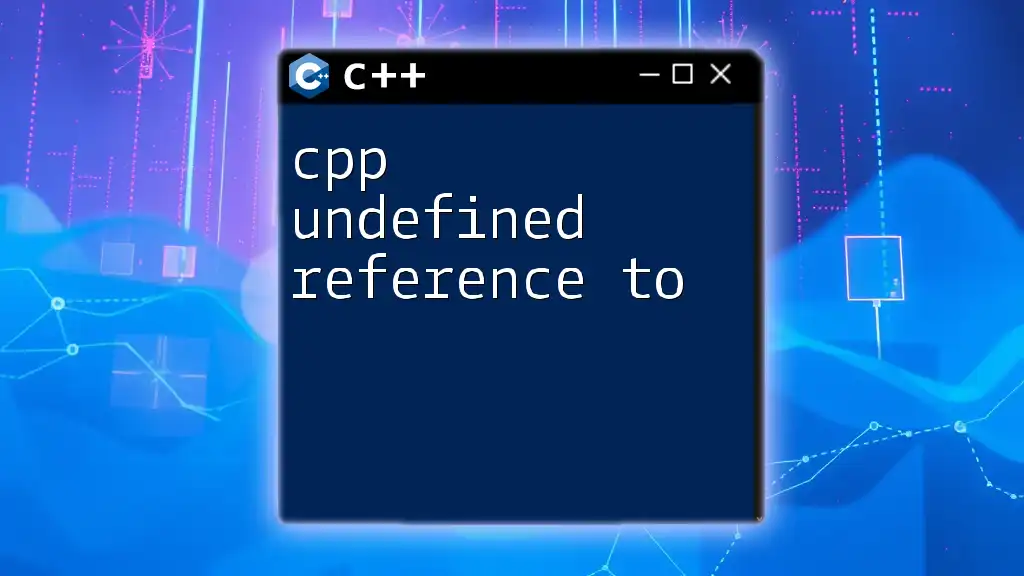
Additional Resources
To continue your learning journey, explore programming books dedicated to C++, participate in online forums, and engage with the community on platforms like Stack Overflow, where discussions often revolve around common C++ challenges. These resources will enhance your understanding and proficiency in C++ programming.
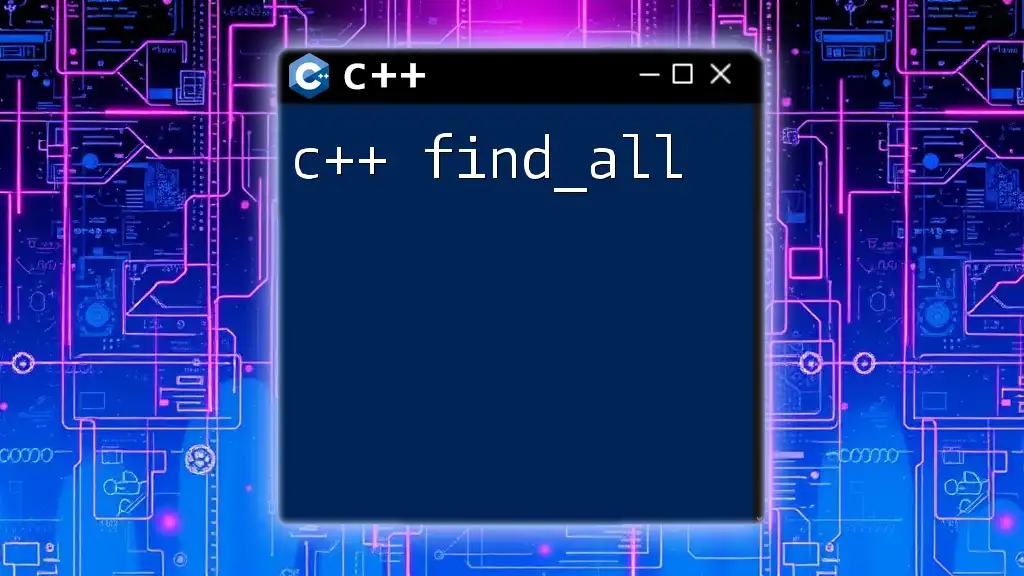
Glossary of Terms
- Linker: A tool that combines various object files into an executable.
- Static Linking: Refers to the embedding of library routines in the executable.
- Namespace: A declarative region that provides a scope to the identifiers inside it, preventing name conflicts.