In C++, an "undefined reference to" error occurs when the compiler cannot find the definition of a function or variable that has been declared, often due to missing implementations or incorrect linkage.
// Example of an undefined reference error
#include <iostream>
void myFunction(); // Declaration, but no definition
int main() {
myFunction(); // This will cause an "undefined reference" error
return 0;
}
What is "Undefined Reference to" in C++?
The "undefined reference to" error is a common issue that occurs during the linking stage of C++ compilation. This error indicates that the linker cannot find the definition for a function, variable, or object that has been declared but not implemented. When a developer encounters this error, it means that, while the compiler may have successfully compiled the source files, it is unable to generate the executable since it cannot resolve all references.
Understanding this error is essential as it highlights the importance of differentiating between declaration and definition in C++.

Common Causes of "Undefined Reference to" Errors
Missing Function Implementations
One of the most straightforward causes of the cpp undefined reference to error is the omission of a function's implementation. When you declare a function in a header file but fail to provide the necessary body in your corresponding source file, the linker will throw an error.
Example:
// Header file Example.h
void myFunction(); // Declaration
// Main file main.cpp
#include "Example.h"
int main() {
myFunction(); // Causes undefined reference error
return 0;
}
In this case, the linker cannot find the implementation for `myFunction()`, leading to an undefined reference error.
Incorrect Use of Namespaces
Another frequent source of this error arises from namespace mismatches. If a function is defined within a namespace, it must be invoked with the proper namespace scope. Forgetting this can result in unresolved references.
Example:
// Namespace Example
namespace MyNamespace {
void myFunction() {}
}
// Main file main.cpp
#include "Example.h"
int main() {
MyNamespace::myFunction(); // Correct usage
myFunction(); // Causes undefined reference error
return 0;
}
In the snippet above, while the first call is correctly qualified, the second call fails because it lacks the proper namespace context.
Mismatched Function Signatures
An “undefined reference” error can also arise when there’s a mismatch between the declared function signature and the signature used in the function's definition. This discrepancy prevents the linker from associating the function calls with their implementations.
Example:
// Incorrect function signature
void myFunction(int); // Declaration
// Implementation with different signature
void myFunction(double) {} // Causes undefined reference error
int main() {
myFunction(5); // Result: undefined reference
return 0;
}
In this case, the linker is unable to match the call to `myFunction(5)` with any available implementation because it expects an integer parameter based on the declaration, while the definition uses a double.
Missing Object Files or Libraries
When you compile a program consisting of multiple translation units or external libraries, failing to link all necessary object files or libraries can result in undefined references.
Example:
# Command line example
g++ main.cpp -lMissingLibrary // Missing linked library
Here, you might receive an undefined reference error for functions that are supposed to be resolved by the `MissingLibrary`. This situation emphasizes the need to ensure that all dependencies are correctly linked during the compilation.

Troubleshooting "Undefined Reference to" Errors
Using Compiler Flags
Utilizing compiler flags can be immensely helpful in diagnosing linking issues. The `-g` flag enables debugging symbols, which allows you to track down the source of the errors more effectively.
Example commands:
g++ -o output main.cpp -g -Wl,--no-as-needed
Inserting the `-Wl,--no-as-needed` allows you to discover whether all referenced libraries are being linked appropriately.
Checking Linker Errors
Carefully examining the linker error messages provides valuable clues about what's causing the undefined reference. These messages typically specify the function or variable that the linker cannot resolve, enabling you to pinpoint the exact problem.
Correct Build Order in Makefiles
For projects that utilize Makefiles, ensuring a correct build order is vital. If a file that provides a necessary implementation is built after a file relying on it, the linker will be unable to resolve references.
Example of a simple Makefile:
all: main.o library.o
g++ -o output main.o library.o
main.o: main.cpp Example.h
g++ -c main.cpp
library.o: library.cpp Example.h
g++ -c library.cpp
In this example, the targets `main.o` and `library.o` can be compiled independently, but they both need to be correctly linked to output the final executable without errors.
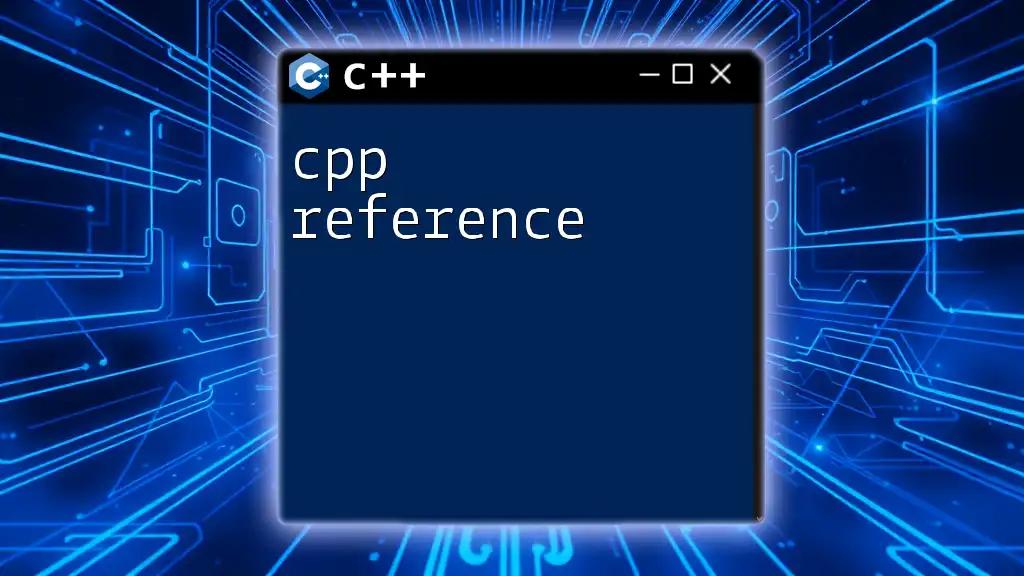
Best Practices to Avoid "Undefined Reference to" Errors
Consistent Headers and Implementation
One of the best practices to avoid the undefined reference to error is maintaining consistency between function declarations in header files and their definitions in source files. Always verify that function signatures match exactly.
Example of maintaining clean header files:
// Example.h
#ifndef EXAMPLE_H
#define EXAMPLE_H
void myFunction();
#endif // EXAMPLE_H
// Example.cpp
#include "Example.h"
void myFunction() {
// Implementation here
}
Use of Inline Functions
Using inline functions when appropriate can help avoid unresolved references. Inline functions are defined directly in header files, so they eliminate the confusion over whether a function has been defined.
Example:
// Inline Function
inline void myFunction() {
// Implementation here
}
This approach ensures that every translation unit that includes the header finds the function definition without triggering linker errors.
Comprehensive Testing and Debugging
Regular testing of your code will help catch issues before they lead to linking errors. Using tools like gdb or integrated development environments (IDEs) with debugging capabilities can significantly aid in identifying the source of errors.

Conclusion
In conclusion, understanding the causes and implications of the cpp undefined reference to error is essential for C++ developers. By being aware of common pitfalls, utilizing debugging techniques, and adhering to best practices, you can navigate this error with ease and efficiency. Embracing these strategies will enhance your C++ programming experience and improve your coding skills overall.

Additional Resources
For further reading on error handling in C++, you may consider exploring official C++ documentation, advanced C++ books, or online tutorials focused on C++ programming best practices and debugging techniques. Engaging with these resources can deepen your understanding and equip you with the tools necessary to tackle C++ challenges confidently.