A C++ vector is a dynamic array that can resize itself automatically when elements are added or removed, allowing for efficient storage and manipulation of a collection of elements.
Here’s a simple code snippet demonstrating how to declare, add elements to, and access elements in a C++ vector:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers; // Declare a vector of integers
numbers.push_back(1); // Add elements to the vector
numbers.push_back(2);
numbers.push_back(3);
// Access and print elements in the vector
for (int num : numbers) {
std::cout << num << " ";
}
return 0;
}
What is a Vector in C++?
A vector is a part of the Standard Template Library (STL) in C++, which provides a dynamic array feature. Unlike traditional arrays, which have a fixed size, vectors can grow and shrink in size automatically as elements are added or removed. This adaptability makes vectors a preferred choice for handling data where the number of elements isn't predetermined.
When comparing vectors to arrays, it’s essential to highlight several benefits of using vectors:
- Dynamic Sizing: Vectors can change size without the need for manual memory management.
- Safety Features: Vectors offer functions like `.at()`, providing bounds-checking during element access.
- Built-in Functions: Vectors come with a range of built-in functions, making various operations simpler and more efficient.
Additionally, vectors allocate memory dynamically, reducing the risk of memory overflow and allowing for better memory management.

Basic Operations on Vectors
Declaration and Initialization
You can declare a vector using the following syntax:
std::vector<int> myVector; // Declare a vector of integers
Vectors in C++ can be initialized in several ways:
-
Empty Vector: Declared without initializing any elements.
std::vector<std::string> emptyVector;
-
Filled Vector: You can initialize it with a set number of elements.
std::vector<int> filledVector(5, 10); // A vector of size 5, filled with 10
-
Using an Array: You can also create a vector from an array.
int arr[] = {1, 2, 3, 4}; std::vector<int> vectorFromArray(arr, arr + sizeof(arr) / sizeof(arr[0]));
Adding Elements
Vectors allow you to add elements using the `push_back()` method. This method appends an element to the end of the vector.
myVector.push_back(10); // Adds 10 to the end of the vector
This flexibility means you can continuously add elements without being constrained by predefined sizes.
Accessing Elements
Accessing elements in a vector can be done in two primary ways:
-
Using Array-like Indexing: This is similar to accessing array elements.
int firstElement = myVector[0]; // Access first element
-
Using the `at()` Function: This method provides safety by checking bounds.
int secondElement = myVector.at(1); // Accesses the element safely
The `at()` method is generally preferred for its safety features, especially in larger programs where bounds checking helps avoid runtime errors.
Removing Elements
To remove the last element of a vector, you can use the `pop_back()` function.
myVector.pop_back(); // Removes the last element
For removing specific elements, the `erase()` function comes into play. This method lets you delete elements at particular indices.
myVector.erase(myVector.begin() + 1); // Removes the second element
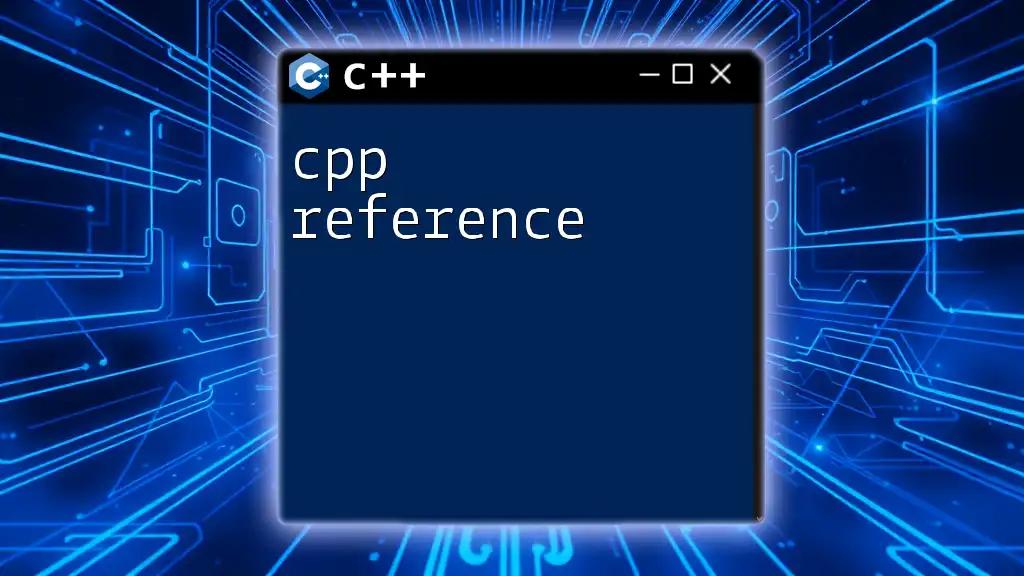
Vector Properties and Functions
Size and Capacity
Understanding size and capacity is crucial when working with vectors. Size refers to the number of elements currently stored in a vector, while capacity refers to the amount of space allocated.
You can check the size and capacity of a vector using these functions:
std::cout << "Size: " << myVector.size() << ", Capacity: " << myVector.capacity() << std::endl;
These functions help gauge how much data your vector is managing, allowing for optimal performance.
Resizing Vectors
To resize a vector when its current size doesn’t serve your needs, you can use the `resize()` method. This is particularly helpful for ensuring your vector size aligns with your data needs.
myVector.resize(5); // Resize the vector to contain 5 elements
If you reduce the size, any elements beyond that index are removed. Conversely, if you increase it, new elements are by default initialized.
Clearing and Swapping
To remove all elements from a vector, you can use the `clear()` method. Though the vector remains, it will be empty.
myVector.clear(); // Removes all elements
Swapping contents between two vectors can be efficiently done using the `swap()` method, allowing efficient data manipulation in your program.
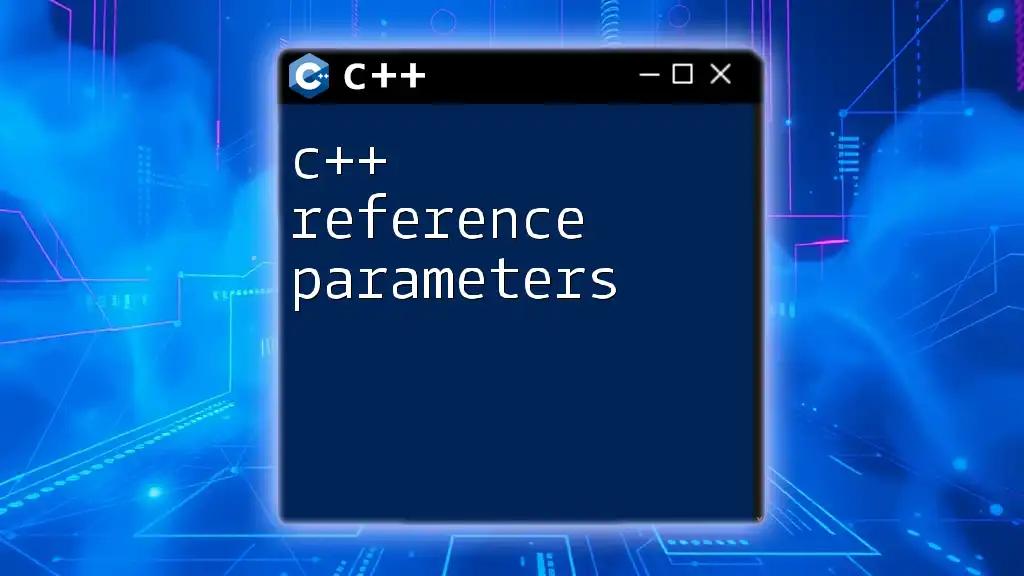
Iterating through Vectors
Using for Loop
The traditional for loop is a straightforward way to iterate through a vector.
for (size_t i = 0; i < myVector.size(); ++i) {
std::cout << myVector[i] << " ";
}
This approach provides a clear understanding of the index and its elements.
Using Range-based for Loop
If you are using C++11 or later, the range-based for loop gives a more elegant way to iterate:
for (const auto& element : myVector) {
std::cout << element << " ";
}
This method eliminates the need for indexing, simplifying the code.
Using Iterators
Iterators provide a more generalized approach for traversing containers. You can use `begin()` and `end()` functions to iterate.
for (auto it = myVector.begin(); it != myVector.end(); ++it) {
std::cout << *it << " ";
}
This iterator-based approach is versatile and can be integrated with various STL algorithms.
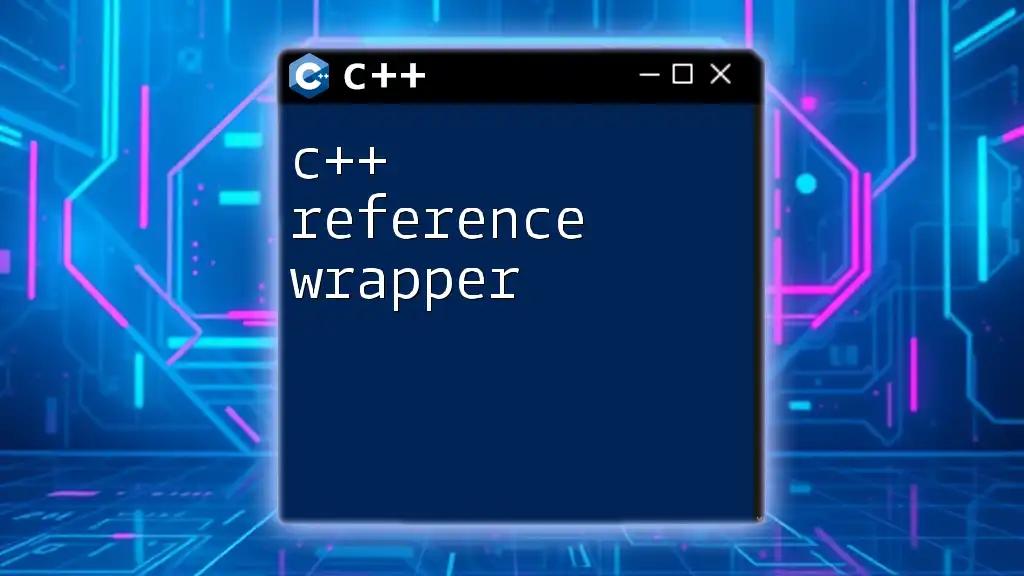
Common Use Cases for Vectors
Vectors are ideal for situations where the data size is dynamic and can change frequently, such as:
- Managing lists where items can be added or removed (e.g., shopping lists, event registrations).
- Storing objects, such as customer records or inventory items, allowing for administrative features like sorting and searching.
The flexibility of vectors aligns well with various applications in real-world programming scenarios.

Performance Considerations
When working with vectors, it's essential to understand the time complexity of vector operations. For example:
- Accessing elements using indexing is O(1).
- Adding elements with `push_back()` can be O(1) on average but may be O(n) in specific instances, such as when reallocation occurs.
To maintain optimal performance, consider preallocating memory with the `reserve()` function when the number of elements is known beforehand:
myVector.reserve(100); // Preallocates memory for 100 elements
This initial reservation helps avoid repeated reallocations as your vector grows.
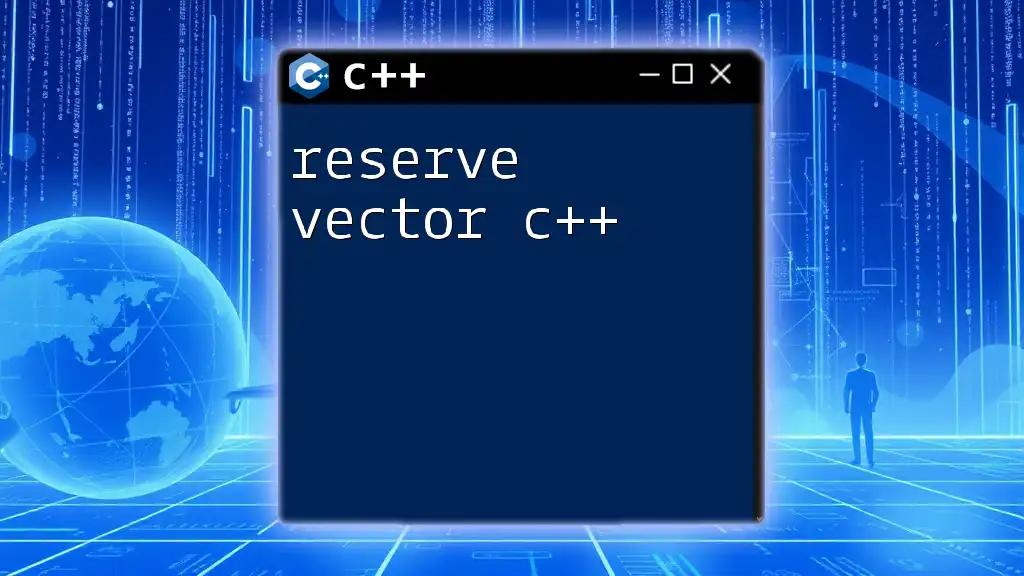
Conclusion
In this comprehensive guide, we've explored the features and functionalities of the C++ reference vector. From initialization and basic operations to best practices and performance considerations, understanding vectors equips you with essential tools for efficient C++ programming. Engage in practice with the examples provided to solidify your knowledge and expand your programming acumen.

Frequently Asked Questions (FAQs)
What happens when a vector runs out of capacity?
When a vector reaches its maximum capacity, it automatically allocates more memory (usually doubling the capacity) to accommodate additional elements.
Can vectors hold objects of custom classes?
Yes, vectors can store objects of any data type, including custom classes, as long as those classes are copy-constructible.
What are the limitations of vectors?
Vectors can lead to higher memory usage due to dynamic resizing and may not be as efficient as other data structures for frequent insertions and deletions at the beginning or the middle of the container.