The `reserve` function in C++ is used to preallocate memory for a vector, optimizing performance by reducing the need for multiple reallocations as elements are added.
#include <vector>
#include <iostream>
int main() {
std::vector<int> myVector;
myVector.reserve(10); // Reserves space for 10 integers
std::cout << "Capacity: " << myVector.capacity() << std::endl; // Outputs: Capacity: 10
return 0;
}
Understanding `std::vector`
In C++, a vector is a dynamic array that can resize itself when elements are added or removed. This powerful container offers several features that make it suitable for a wide range of applications. Unlike fixed-size arrays, vectors can grow and shrink in size, providing flexibility that allows developers to create more robust solutions.
Vectors are part of the C++ Standard Library and are found in the `<vector>` header. They are particularly useful when we don't know the number of elements to be stored in advance. Compared to other containers like arrays and lists, vectors provide a compelling combination of performance and ease of use.
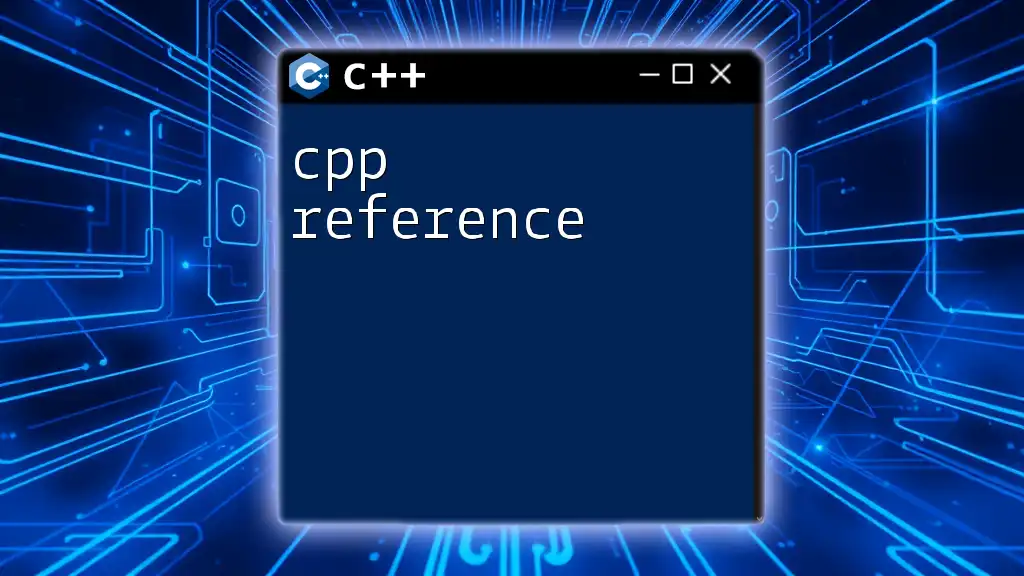
The `reserve()` Function
The `reserve()` function is crucial for optimizing the memory management of a vector. Its main purpose is to allocate enough memory for a specified number of elements ahead of time, thereby reducing the number of reallocations and copying of existing elements when the vector grows.
Syntax of `reserve()`
The syntax of the `reserve()` function is straightforward:
void reserve(size_type new_cap);
Where `new_cap` is the new capacity to reserve for the vector. It is important to note that using `reserve()` does not change the current size of the vector; it only ensures that enough memory is allocated.
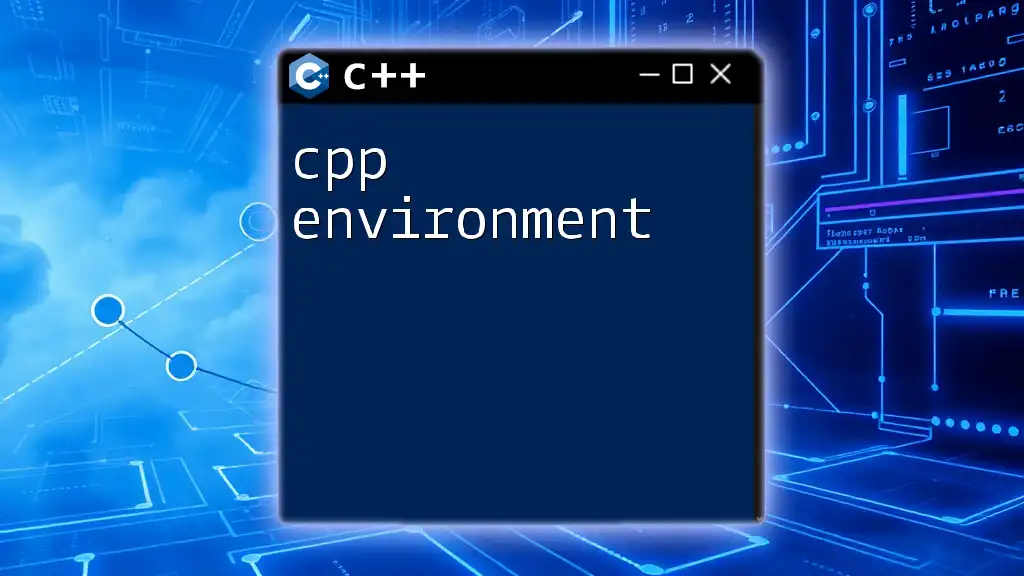
Importance of Reserving Space
Reserving space in a vector can significantly improve performance, especially when handling large amounts of data. Here are some of the key benefits:
- Avoiding multiple reallocations: Each time a vector grows beyond its current capacity, it must allocate new memory and copy existing elements to this new location. By using `reserve()`, you can allocate all the needed space in a single call, thereby minimizing the overhead that comes with multiple reallocations.
- Performance: Efficient memory allocation reduces fragmentation and speeds up access times. This is especially important in scenarios where latency is critical, such as real-time data processing applications.
Benefits of using `reserve()`
By calling `reserve()`, developers can ensure that their vectors operate efficiently when dealing with larger datasets. The most significant advantages include:
- Improved performance when adding elements: When you know the final size of the vector in advance, reserving memory can lead to substantial reductions in time spent on memory management.
- Reduced likelihood of fragmentation in memory: Fewer allocations mean that memory is more likely to remain contiguous, which can lead to better cache performance.
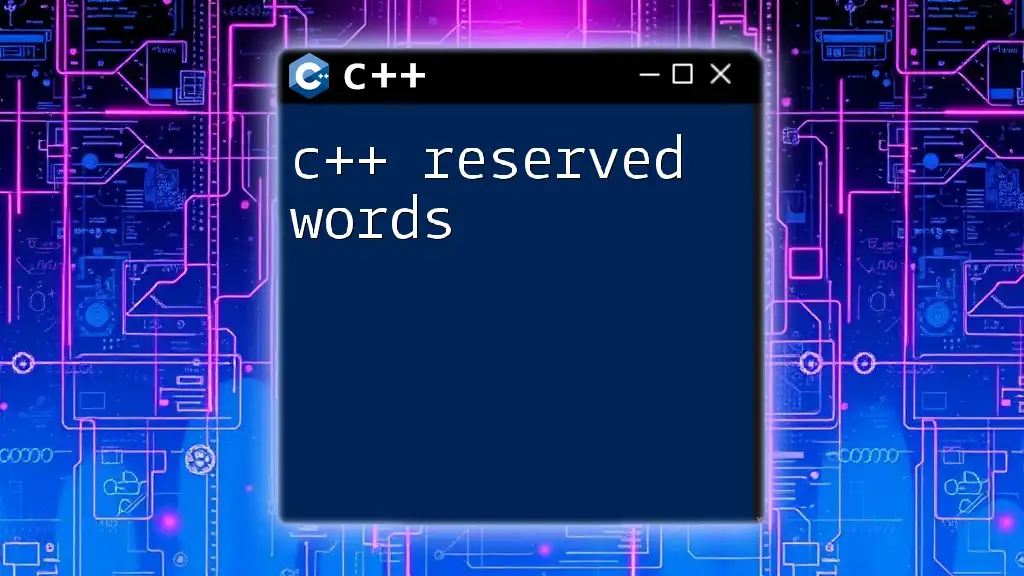
When to Use `reserve()`
There are specific scenarios where reserving space is not just beneficial but essential:
- Handling large datasets: If you expect a vector to grow significantly in size, it's wise to call `reserve()` at the onset.
- Real-time data processing applications: In such cases, any delays caused by memory allocation can be detrimental.
Examples of Good Practices
A simple yet effective use of `reserve()` can be seen in the following code snippet:
std::vector<int> vec;
vec.reserve(1000); // Reserve space for 1000 integers
This code snippet demonstrates a proactive measure: allocating memory for a known number of elements prevents costly reallocations during runtime.
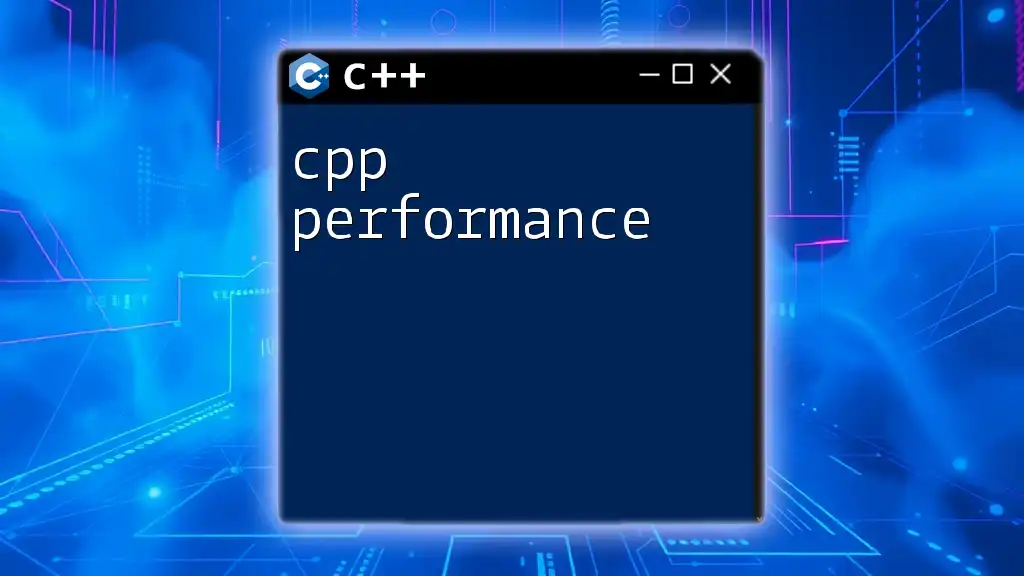
Code Snippets Demonstrating `reserve()`
Basic Example
To understand `reserve()` better, let's take a look at a basic implementation:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec;
vec.reserve(5); // Reserve space for 5 integers
for (int i = 0; i < 5; ++i) {
vec.push_back(i);
}
std::cout << "Size: " << vec.size() << ", Capacity: " << vec.capacity() << std::endl;
return 0;
}
In this example, we reserve space for five integers before adding them to the vector. The output will reveal that the size of the vector matches the number of elements inserted, and the capacity reflects the space we allocated ahead of time.
Demonstrating Efficiency
To illustrate the benefits of `reserve()`, let's compare two functions, one using `reserve()` and the other not:
#include <iostream>
#include <vector>
#include <chrono>
void add_elements_without_reserve() {
std::vector<int> vec;
for (int i = 0; i < 2000; ++i) {
vec.push_back(i);
}
}
void add_elements_with_reserve() {
std::vector<int> vec;
vec.reserve(2000); // Optimal performance
for (int i = 0; i < 2000; ++i) {
vec.push_back(i);
}
}
int main() {
auto start1 = std::chrono::high_resolution_clock::now();
add_elements_without_reserve();
auto end1 = std::chrono::high_resolution_clock::now();
auto start2 = std::chrono::high_resolution_clock::now();
add_elements_with_reserve();
auto end2 = std::chrono::high_resolution_clock::now();
std::cout << "Time without reserve: "
<< std::chrono::duration_cast<std::chrono::microseconds>(end1 - start1).count() << "µs\n";
std::cout << "Time with reserve: "
<< std::chrono::duration_cast<std::chrono::microseconds>(end2 - start2).count() << "µs\n";
return 0;
}
In this snippet, we time the execution of two functions: one that adds elements without using `reserve()` and one that does. The result clearly underscores the performance gains when using `reserve()`.
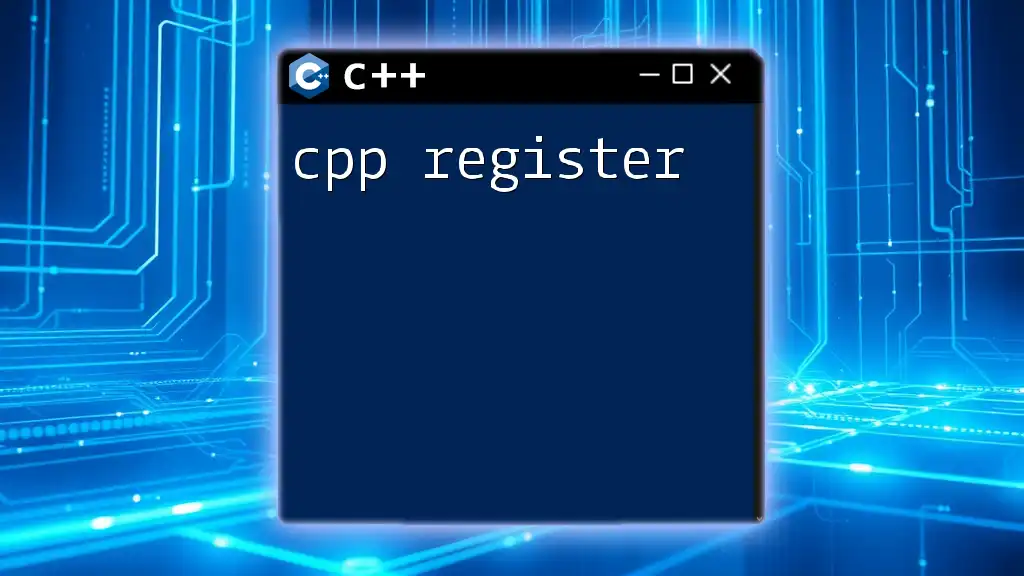
Common Pitfalls when Using `reserve()`
Misunderstandings of `capacity()`
One common misunderstanding among newcomers is the distinction between capacity and size. The `capacity()` function tells you how many elements the vector can hold before needing to increase that space, while `size()` tells you the number of actual elements present in the vector.
Scenario when the vector size exceeds capacity
If elements are added to a vector beyond its reserved capacity, a reallocation will automatically occur, which negates some of the advantages provided by reserving space.
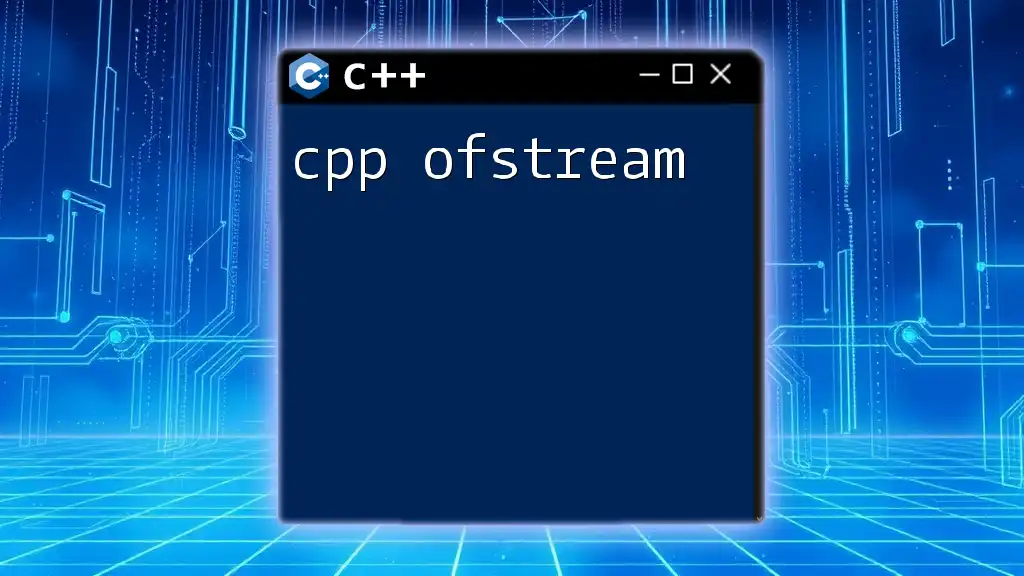
Advanced Concepts
How `reserve()` interacts with other operations
Understanding how `reserve()` interacts with other vector operations is key to using it effectively. For instance, if you call `resize()` after reserving space, you may inadvertently change the current size of the vector, leading to unintended consequences if you're not careful.
Custom Allocators and `reserve()`
If you're using custom memory allocators, it's essential to note how `reserve()` can affect performance. When working with non-standard memory management, the benefits of reserving space may vary, and it may require additional considerations to ensure optimal performance.
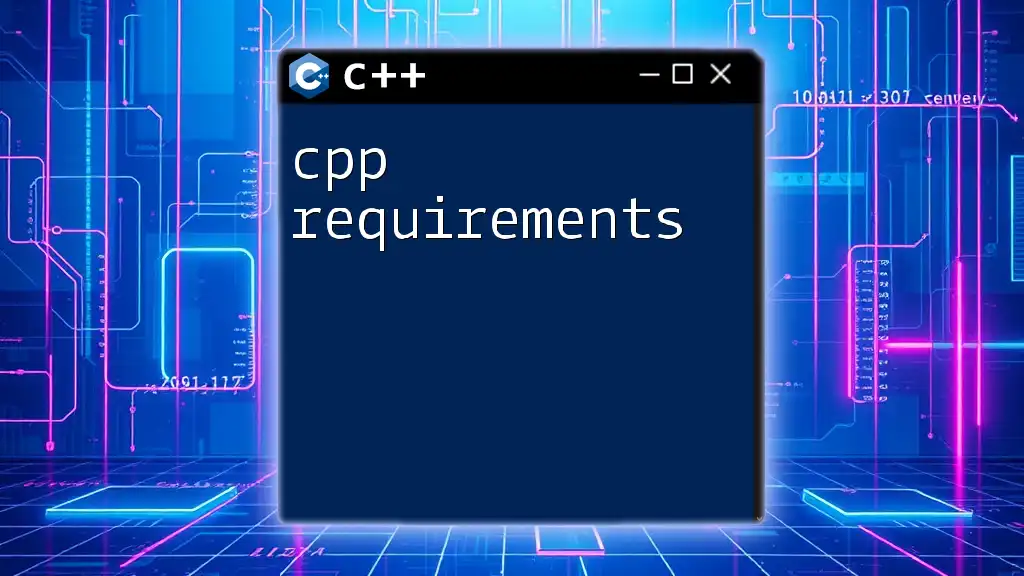
Conclusion
In conclusion, mastering the use of `cpp reserve room` can yield significant performance improvements when working with vectors in C++. By understanding not just how to use `reserve()` but when and why to apply it, developers can create more efficient and responsive applications.
Utilizing `reserve()` is a simple yet critical technique that can elevate the performance of your C++ applications, especially in scenarios demanding sophisticated data handling. With the insights gained from this guide, you're well-equipped to leverage `reserve()` effectively in your programming endeavors.
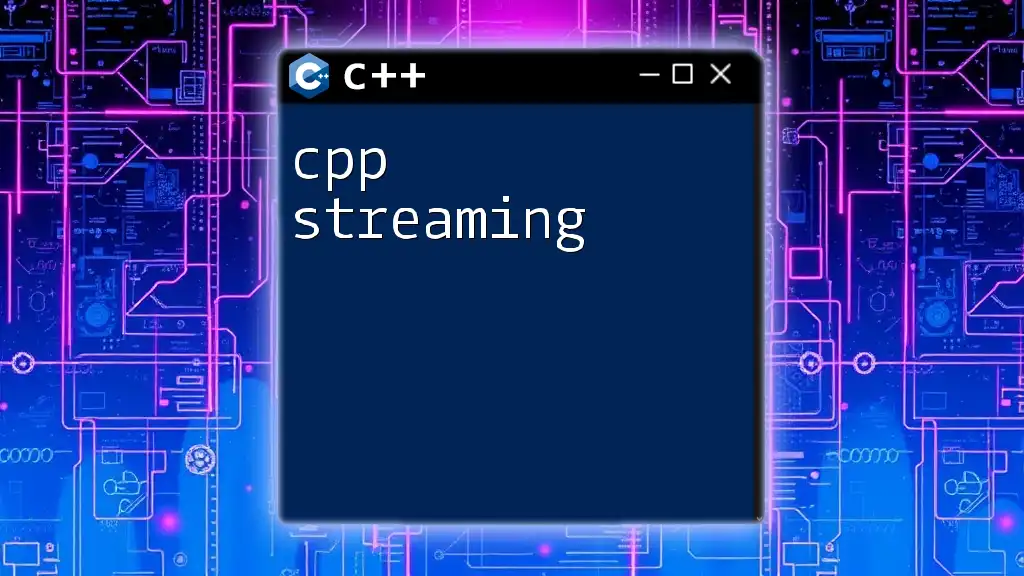
Additional Resources
For those looking to deepen their understanding of C++ and its powerful containers, checking reputable online forums, community discussions, and literature on C++ memory management is highly recommended. Continuing to learn and engage with other C++ developers can also provide valuable insights and practical experiences to refine your skills further.