In C++, the `return` statement is used to exit a function and optionally return a value to the calling function.
int add(int a, int b) {
return a + b; // Returns the sum of a and b
}
Understanding Function Return Types
What is a Return Type?
The return type of a function signifies what type of value a function is expected to return after execution. Specifying the return type is crucial in C++ as it informs the compiler about the kind of data the function will provide to its caller. This helps not only in type safety but also in maintaining the clarity and functionality of the code.
Common Return Types in C++
In C++, functions can return a variety of data types. The most common ones include:
-
Basic Data Types: These include `int`, `float`, `char`, and `bool`. Each type serves specific purposes and holds values that correspond to different data representations in a program.
-
User-defined Types: C++ allows the use of user-defined types, such as structures and classes. This capability enables developers to create complex data structures that can enhance code organization.
-
Pointers and References: Functions can also return pointers, which are variables that hold the memory address of another variable, and references, which are aliases for another variable. This can be particularly useful for dynamic memory management and object manipulation.

Using the `return` Statement
Basic Syntax of the `return` Statement
The syntax of the `return` statement is straightforward. It is followed by the expression to be returned. Here’s a simple function that demonstrates the usage of the `return` statement:
int add(int a, int b) {
return a + b;
}
In this example, the `return` statement takes the sum of `a` and `b` and sends it back to the function caller.
When to Use `return`
The `return` statement is indispensable in scenarios where you need to provide output from a function, but it is also useful for terminating function execution early. For example, a function might return a value before reaching its natural end due to certain conditions being met.
Returning Values
Returning Primitive Data Types
Returning primitive data types is straightforward. Here’s an example where a function multiplies two numbers and returns the result:
float multiply(float x, float y) {
return x * y;
}
In this case, the multiplication result is returned to wherever the function was called.
Returning User-Defined Types
When working with user-defined types, the `return` statement is just as effective. Here’s how you can return an instance of a class:
class Point {
public:
int x, y;
Point(int x, int y) : x(x), y(y) {}
};
Point getOrigin() {
return Point(0, 0);
}
The `getOrigin()` function creates and returns a `Point` object, illustrating how the `return` statement can facilitate the use of complex types.
Returning Pointers and References
Returning Pointers
While powerful, returning pointers can be risky due to issues like dangling pointers. Here’s an example function that returns a pointer to a dynamically allocated array:
int* createArray(int size) {
return new int[size];
}
In this case, it is critical to manage the memory properly to avoid leaks. The caller is responsible for freeing this memory.
Returning References
Returning references can be more efficient, especially in cases where performance is a concern. Here’s an example of returning a reference to an element in an array:
int& getElement(int* array, int index) {
return array[index];
}
This allows the caller to modify the original array element directly. However, caution is advised to ensure the reference remains valid for its intended use.
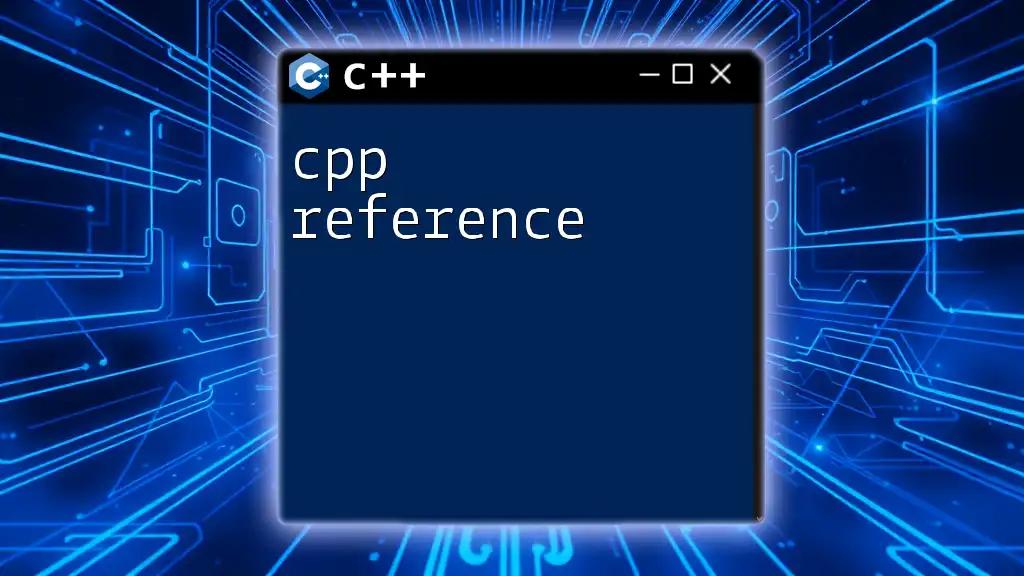
Implicit and Explicit Returns
Implicit Returns in C++
C++ allows for implicit returns, meaning that if you do not explicitly specify a return value, a function will return an automatic value based on the final expression evaluated. However, being clear with your returns is best practice. For example:
int square(int n) {
return n * n; // returns the square value
}
Explicit Returns
Explicit returns should be used in functions, especially void functions, to clarify the intent. Although optional, using `return` can enhance readability:
void displayMessage() {
std::cout << "Hello, World!" << std::endl;
return; // optional for clarity
}
This practice can significantly improve code maintainability.
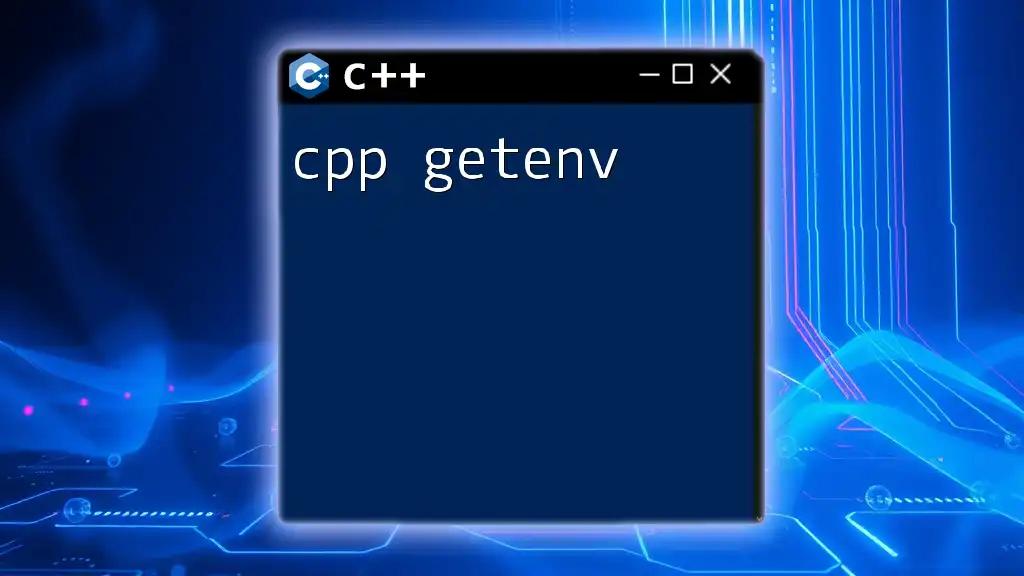
Returning from Main
Using `return` in `main()`
The function `main()` serves as the entry point for a C++ program and also makes use of the `return` statement. By convention, returning `0` indicates successful execution:
int main() {
return 0; // Indicates successful execution
}
Exiting with Error Codes
Returning non-zero values in `main()` indicates that an error occurred. This convention is essential for operating systems and other programs that might launch your C++ application and need status information.
int main() {
if (someErrorCondition) {
return 1; // Indicates error
}
return 0;
}
This clear distinction helps in debugging and managing application behavior efficiently.

Common Mistakes and Best Practices
Mistakes Related to `return`
One common mistake is forgetting to use `return` in functions that are expected to return a value. This can lead to undefined behavior. Another risk is inadvertently returning a variable that goes out of scope, which might cause dangling references.
Best Practices for Using `return`
When writing functions, it’s essential to use `return` statements effectively. Aim for clarity to make your intentions explicit. Consider using early returns to decrease nesting in complex functions, thus improving readability and maintainability.

Conclusion
The `return` statement in C++ is crucial for providing output and managing control flow within functions. By understanding its syntax, function types, and common practices, you can enhance your coding skills and write more robust, effective C++ programs. Practical applications and careful management of return types will lead to cleaner and more efficient code. For further exploration, developers are encouraged to engage in real-world coding scenarios to consolidate their understanding of the `cpp return`.