"CPP learn" focuses on providing quick and concise tutorials for mastering C++ commands and syntax efficiently.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl; // A simple program that outputs a greeting
return 0;
}
What is C++?
C++ is a powerful, high-level programming language that has shaped modern software development since its inception at Bell Labs in the early 1980s. It was designed as an enhancement to the C programming language, providing not just low-level computational ability but also higher-level functionality. One of C++'s defining features is its ability to support both procedural and object-oriented programming paradigms, allowing developers to choose a style that suits their needs.
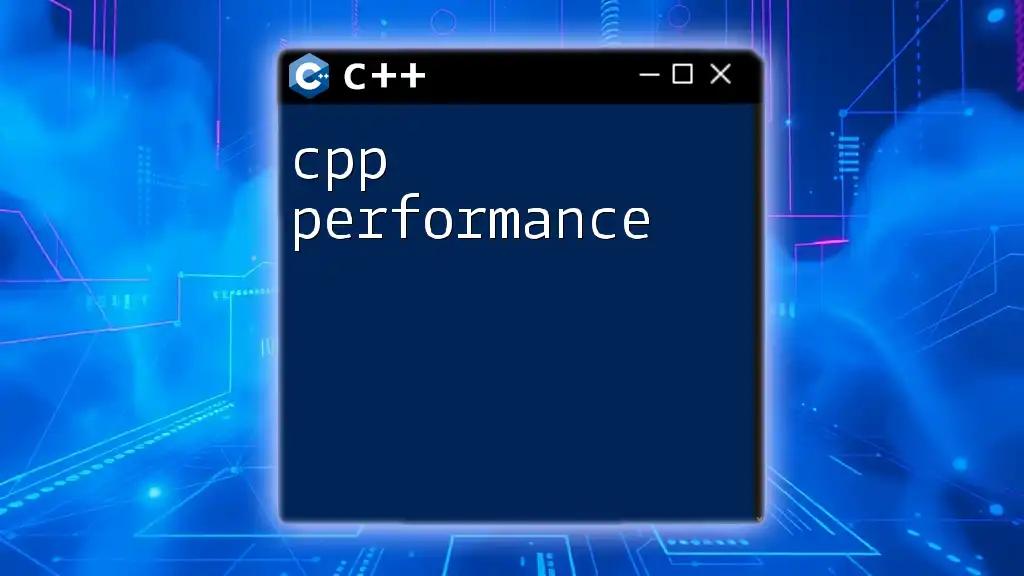
Why Learn C++?
Learning C++ is essential for several reasons. Its versatility allows it to be used in various applications ranging from system software to game development and financial modeling. Understanding C++ also provides a strong foundation for grasping other programming languages, as many concepts in C++ exist in languages like Java, Python, and C#. Furthermore, C++ is widely used in industries that require high-performance applications, making it a valuable skill for aspiring developers.
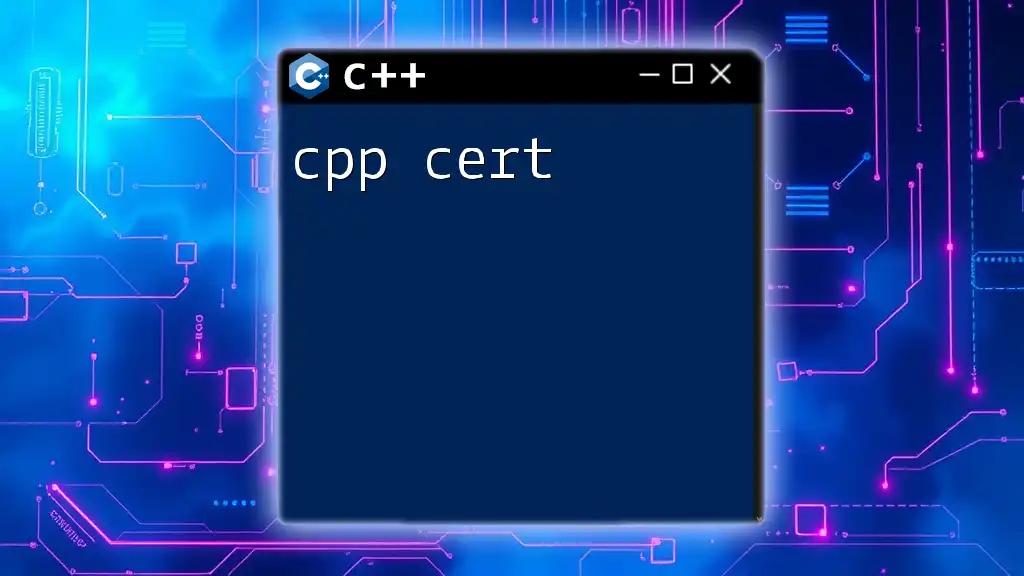
Getting Started with C++
Setting Up Your Environment
To jump into C++ programming, you need to set up your development environment. This typically involves choosing an Integrated Development Environment (IDE) as well as installing a C++ compiler. Popular IDEs for C++ include:
- Visual Studio: A feature-rich IDE suitable for all levels.
- Code::Blocks: A lightweight, cross-platform IDE that is easy to use.
- CLion: A powerful IDE for C++ that offers excellent code navigation features.
Once you've selected your IDE, the next step is to install a C++ compiler. Common choices include GCC (GNU Compiler Collection) and Clang. These tools will allow you to compile your C++ code into executable programs.
Basic Concepts in C++
Syntax and Structure of a C++ Program
A C++ program consists of various elements that come together to produce functional code. At its simplest, it includes headers, the main function, and executable statements. Here’s a basic example of a C++ program that prints "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
This program demonstrates the syntax involved in a simple C++ application. The `#include <iostream>` directive allows the usage of the input-output stream library, while the `main` function is where the execution begins.
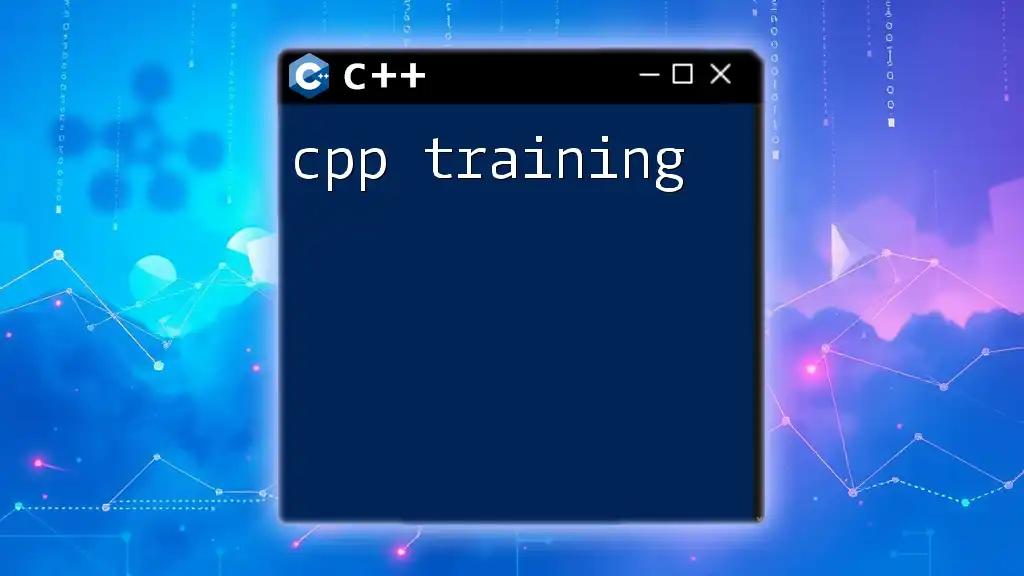
Core Concepts of C++
Variables and Data Types
Understanding variables and data types is fundamental in C++. Variables are used to store data that can change during the execution of a program, while data types define the kind of data a variable can hold. Common data types in C++ include:
- int: Used for integer values (e.g., `int age = 30;`)
- float: For floating-point numbers (e.g., `float height = 5.9;`)
- char: Represents individual characters (e.g., `char grade = 'A';`)
This initial understanding of data types equips beginners with the tools needed to manipulate data effectively.
Control Structures
C++ uses control structures like conditional statements and loops to direct the flow of a program.
Conditional Statements
The basic form of a conditional statement in C++ is the `if` statement. Here’s a simple implementation to check if someone is an adult:
if (age >= 18) {
std::cout << "Adult";
}
Loops
Loops allow code to be executed repeatedly based on certain conditions. For instance, a `for` loop can iterate a block of code a specified number of times. The following code prints numbers from 0 to 4:
for (int i = 0; i < 5; i++) {
std::cout << i << std::endl;
}
The ability to use loops and conditional statements effectively can significantly enhance your programming capabilities.
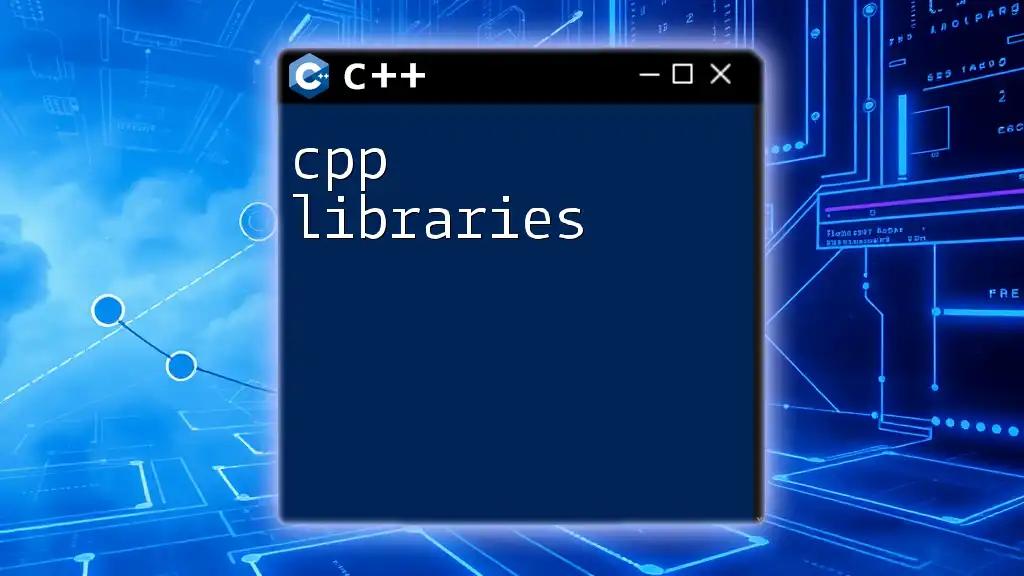
Diving Deeper into C++
Functions in C++
Functions are reusable blocks of code that perform a specific task. In C++, a function can take parameters and return a value. Here’s a simple function that adds two integers:
int add(int a, int b) {
return a + b;
}
In this example, `add` is a function that takes two `int` parameters and returns their sum. Using functions helps to keep code organized and reduces redundancy.
Object-Oriented Programming (OOP) Principles
C++ supports object-oriented programming, which is an essential paradigm for organizing complex software. Key OOP principles include:
-
Classes and Objects: C++ uses classes as blueprints to create objects. An example class is provided below:
class Car { public: std::string model; void honk() { std::cout << "Honk! Honk!"; } };
In this code, `Car` is a class with a public attribute `model` and a method `honk`.
-
Inheritance: This allows a class to inherit attributes and methods from another class, promoting code reusability.
-
Polymorphism: Polymorphism enables methods to do different things based on the object calling them.
-
Encapsulation: This principle restricts access to some of an object’s components, promoting a clearer interface.

Tips for Effective Learning
Structured Learning Resources
To effectively learn C++, consider utilizing a variety of resources. Recommended books include "C++ Primer" by Lippman and "Effective C++" by Scott Meyers. Online courses through platforms like Coursera and Udemy can also provide structured learning. Additionally, engaging in coding challenges on websites such as HackerRank or LeetCode can enhance practical skills.
Best Practices in C++ Coding
Adopting best practices in C++ coding is vital for writing clean, maintainable code. Here are some fundamentals:
- Code Readability: Use meaningful variable names and comments to describe complex logic.
- Documentation: Consistently document your methods and classes to aid future reference.
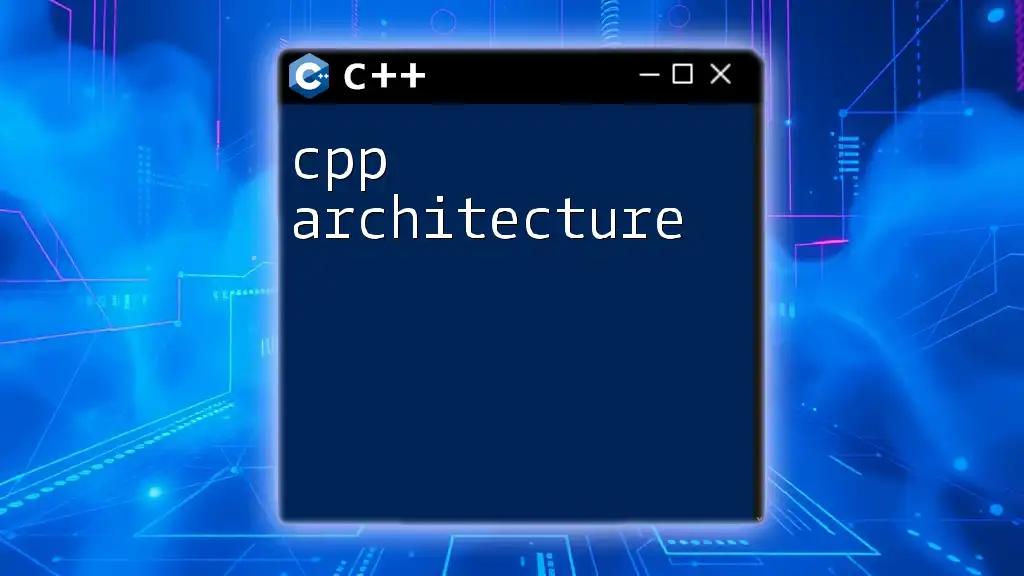
Troubleshooting and Debugging
Common Errors in C++ and How to Fix Them
When learning C++, you're likely to encounter errors. It’s important to understand the difference between compilation errors (issues that occur when code is being compiled) and runtime errors (issues that arise when the compiled program is executed). An example of a common compilation error might be missing a semicolon.
Using Debugging Tools
Most IDEs come with built-in debugging tools to assist in tracking down issues in your code. Familiarizing yourself with these tools will streamline your learning process, as you’ll be able to inspect variable states and control execution flow.
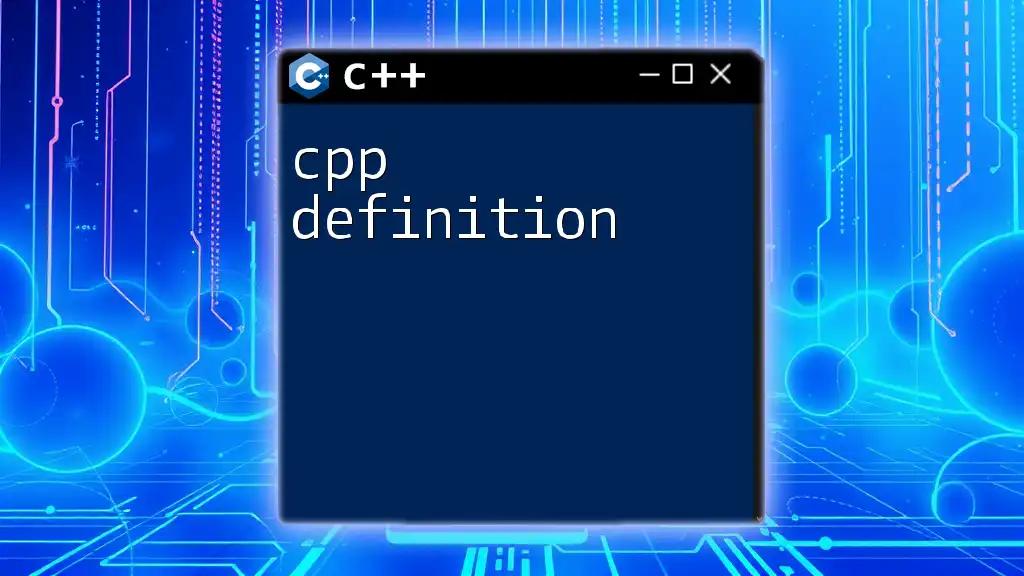
Real World Applications of C++
C++ in Software Development
C++ is widely used in software development due to its high performance and cross-platform capabilities. It is the foundation of many popular operating systems, such as Microsoft Windows, and is frequently employed in applications where speed and efficiency are crucial.
Game Development with C++
C++ plays a significant role in game development, especially in industry-leading game engines like Unreal Engine. Its capabilities allow developers to create complex gameplay mechanics, high-performance graphics, and efficient resource management. If you are passionate about game development, learning C++ will open up numerous avenues in this exciting field.
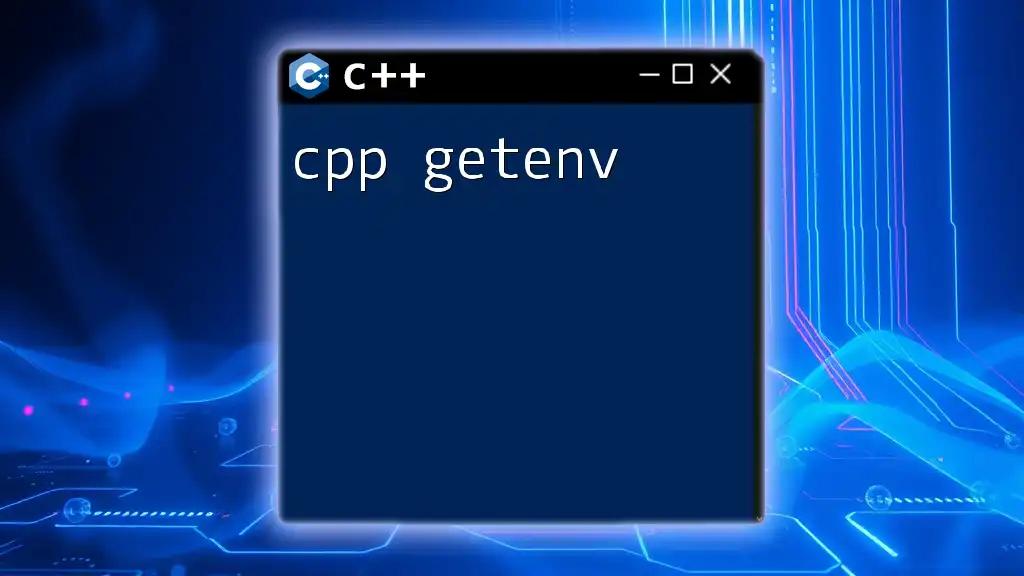
Conclusion
The journey of learning C++ opens up a plethora of opportunities in technology and software development. By understanding its syntax, mastering its core concepts, and applying best practices in coding, you will build a solid foundation that can propel you into more advanced programming challenges. The key is consistent practice and exploration—don't hesitate to dive deeper into the realm of C++ and enhance your skills further.
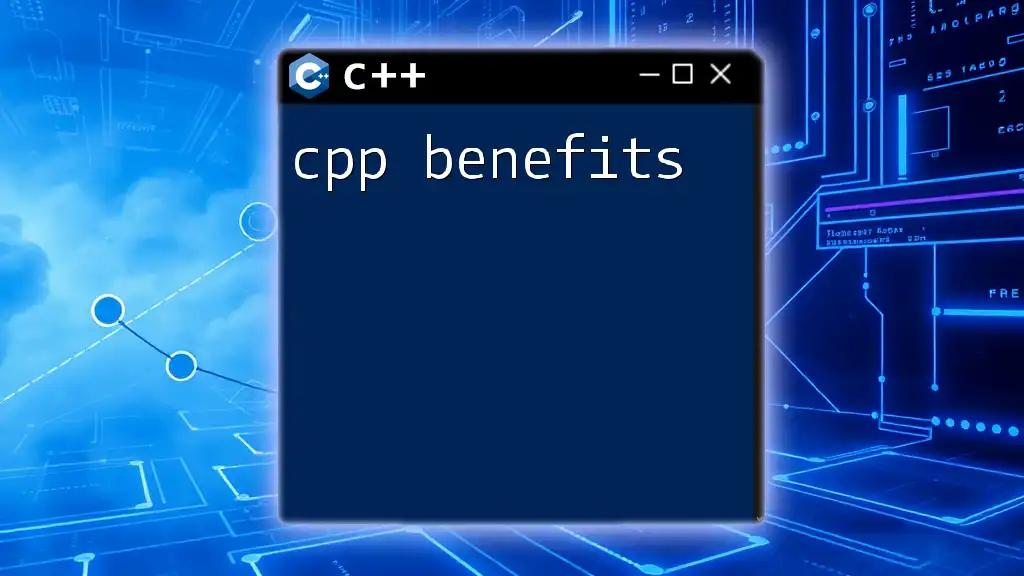
Additional Resources
To support your C++ learning journey, consider joining online communities such as Stack Overflow or Reddit's r/cpp. These platforms can provide not just answers to your questions but also networking opportunities with fellow learners and experienced developers. Further reading and tutorials can also facilitate your mastery of advanced topics, including the Standard Template Library (STL) and templates. By actively engaging in these resources, you enhance not only your knowledge but also your practical skills in C++.