C++ events typically refer to the mechanisms used for handling user interactions or specific occurrences in a program, often implemented through callback functions or event listeners.
Here's a simple code snippet demonstrating an event-like mechanism using a callback function:
#include <iostream>
#include <functional>
// Define a type for the event callback
using EventCallback = std::function<void(int)>;
// Function to simulate an event trigger
void triggerEvent(EventCallback callback) {
int eventData = 42; // Sample data associated with the event
callback(eventData); // Trigger the event
}
// Example callback function
void onEvent(int data) {
std::cout << "Event triggered with data: " << data << std::endl;
}
int main() {
// Triggering the event and passing the callback
triggerEvent(onEvent);
return 0;
}
Understanding the C++ Event System
Concept of Events
In C++, events are fundamental constructs that allow the design of responsive systems by signaling that something has occurred. Events can be considered as notifications that something significant has happened within an application, and other parts of the system can respond accordingly. This is particularly important in graphical user interfaces (GUIs), real-time systems, and applications that require inter-component communication.
Event-driven Programming
Event-driven programming is a paradigm centered around the concept of events. This approach is especially useful when dealing with asynchronous operations where the flow of the program is determined by events such as user actions. Advantages of event-driven programming include:
- Improved Responsiveness: Programs can remain responsive to user inputs while executing background tasks.
- Modularity: Different parts of the application can operate independently, simplifying maintenance and enhancing flexibility.
- Scalability: Easily add new event listeners without significant changes to existing code.

Implementing Events in C++
Basic Event Implementation
Creating a simple event class serves as the foundation for building robust event systems in C++. An event class encapsulates the behavior expected when an event occurs.
class Event {
public:
virtual void trigger() = 0; // Pure virtual function
};
In this code snippet, we define a base class `Event` with a pure virtual function `trigger()`. This sets up the expectation that any derived class will implement its own specific event-triggering logic.
Event Listeners
Event listeners are crucial components that respond to events. They define `onEvent()` functions that dictate how the program reacts to each event occurrence.
class EventListener {
public:
virtual void onEvent() = 0; // Pure virtual function
};
By creating an interface with the `onEvent()` function, we ensure that any class implementing an event listener will conform to this structure.
Registering and Unregistering Listeners
Managing listener subscriptions is the next important step in building an event system. This involves adding and removing listeners from an event emitter.
class EventEmitter {
private:
std::vector<EventListener*> listeners;
public:
void addListener(EventListener* listener) {
listeners.push_back(listener);
}
void removeListener(EventListener* listener) {
listeners.erase(std::remove(listeners.begin(), listeners.end(), listener), listeners.end());
}
};
In this code, `addListener()` allows us to register new listeners for events, while `removeListener()` is responsible for unsubscribing listeners, avoiding memory leaks and maintaining a clean event system.

Building an Event Management System
Step-by-Step Guide
Creating an event emitter enables the dispatching of events to registered listeners. The `emitEvent` function iterates over all the listeners and calls their `onEvent()` methods.
void emitEvent() {
for (auto listener : listeners) {
listener->onEvent();
}
}
Through this mechanism, we efficiently notify all registered listeners whenever an event occurs.
Handling Multiple Events
Extending functionality to include multiple event types can greatly enhance your application’s responsiveness to different stimuli. This can be done using enums to define the types of events you want to handle.
enum EventType {
OnClick,
OnHover
};
class ClickListener : public EventListener {
public:
void onEvent() override {
// Code for handling click event
std::cout << "Button clicked!" << std::endl;
}
};
Through the flexible use of enums and listener classes, you can manage various events within your application, making the design more adaptable.
Practical Example: A Button Click Event
To bring the event system together practically, we can create a button class that emits click events and registered listeners can take action upon these clicks.
class Button : public EventEmitter {
public:
void click() {
emitEvent(); // Triggers OnClick event
}
};
class MyClickListener : public EventListener {
public:
void onEvent() override {
std::cout << "Button clicked!" << std::endl;
}
};
In this implementation, the `Button` class extends `EventEmitter` and invokes `emitEvent` during the `click()` method, while `MyClickListener` specifies the action upon receiving a button click event.

Best Practices for Using Events in C++
Performance Considerations
When critically assessing performance in event-driven systems, keep in mind that emitting events can introduce overhead, especially when many listeners are registered. To ensure that your application remains responsive, provide mechanisms to debounce events, reducing the frequency of event handling when high interactivity occurs.
Avoiding Memory Leaks
Proper memory management is vital in complex applications that utilize event systems. Always ensure that listeners are properly unregistered when they are no longer needed. This prevents memory leaks and ensures efficient resource management.
Debugging Event Systems
Event systems can be challenging to debug due to their asynchronous nature. Common issues include listeners that do not fire or memory leaks from unregistered listeners. Implement logging at key points using print statements to trace the flow of events through your system. This can help identify if and when events are triggered or missed.
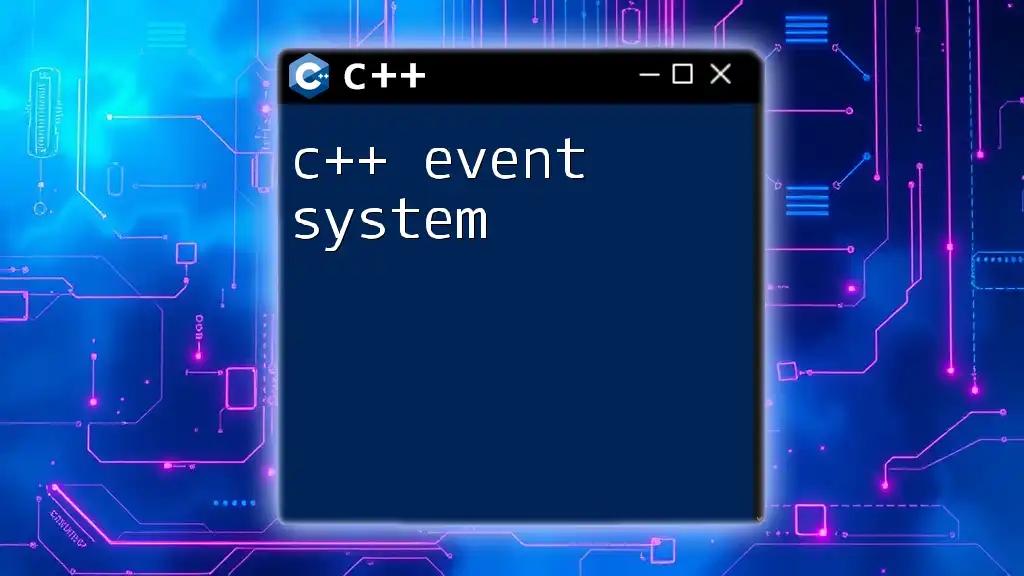
Conclusion
By understanding and implementing cpp events, developers can create more responsive, maintainable, and flexible applications. The concepts of events and event-driven programming provide powerful ways to handle interactions in software design.
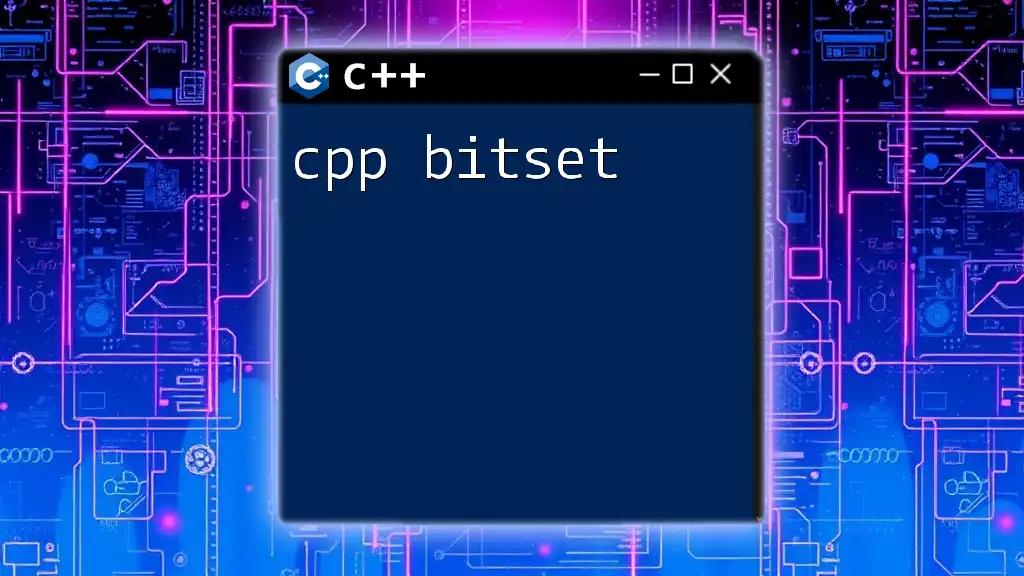
Frequently Asked Questions (FAQ)
What is the difference between events and signals?
While both events and signals signify occurrences in a system, events are more commonly used in C++, where they are explicitly triggered and handled by listeners. Signals, on the other hand, may refer to broader asynchronous notifications.
How can events improve user interface responsiveness?
By leveraging events, user interfaces can remain responsive to user actions, such as clicks and hovers, while background processes complete. This separation allows for a smooth user experience where the application remains interactive and fluid.
Are there available libraries for handling events in C++?
Yes, various libraries, such as Boost.Signals2 and Qt, offer robust implementations of event systems, streamlining the process of creating event-driven applications. These libraries handle many complexities associated with event handling, allowing developers to focus on building features rather than managing event flow.