The "cpp extension" refers to the file extension used for C++ source code files, which enables developers to write and compile programs using this powerful programming language.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++ Extension Concepts
What is an Extension in C++?
In the realm of C++ programming, an extension refers to any addition or enhancement that allows the language to function more robustly. This encompasses various resources such as libraries and frameworks that offer pre-built functionalities which developers can leverage in their applications. By utilizing such extensions, programmers can save time and effort that would otherwise be spent building common functionalities from scratch.
The Role of C++ Extensions in Programming
C++ extensions play a pivotal role in promoting code modularity. This modular approach allows developers to build applications in a more organized manner, where complex functions can be divided into manageable parts. Additionally, extensions facilitate collaboration among developers by keeping codebases clean and maintainable.
The benefits of employing C++ extensions include:
- Enhanced Efficiency: Extensions provide developers with the tools needed to accelerate development cycles.
- Pre-tested Components: By using established extensions, developers can reduce the likelihood of introducing bugs, as these components have been vetted by the community.
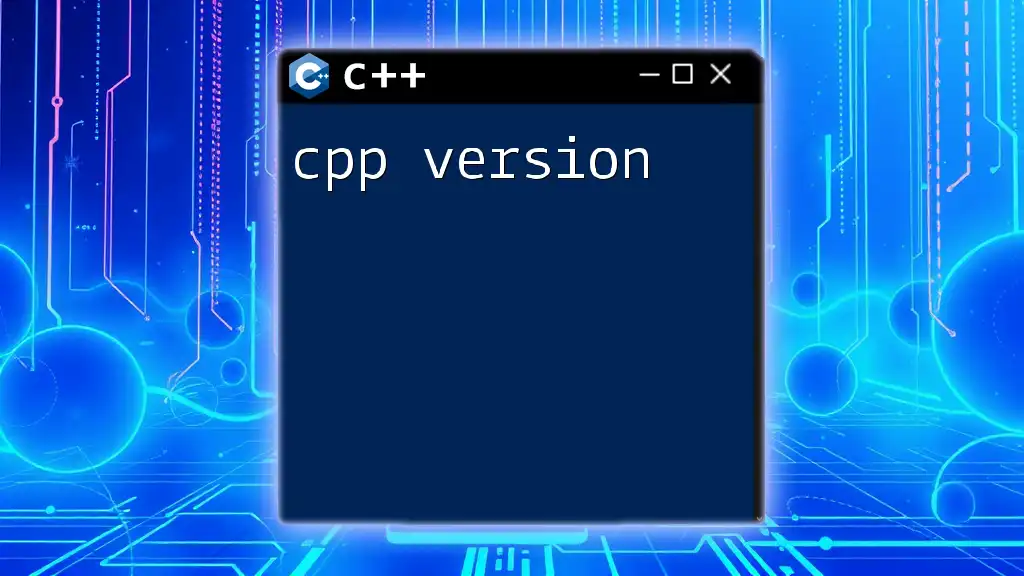
Popular C++ Extensions and Their Uses
Overview of Common C++ Extensions
Several C++ extensions have become industry standards due to their extensive features and functionalities. Familiarizing oneself with these tools can significantly enhance the quality of software development.
Boost Libraries
Boost is one of the most recognized C++ extension libraries that offers a wealth of functionalities ranging from algorithms, data structures, to file system management. It is lauded for its robust design and extensive support.
One key advantage of using Boost is its ease of integration. Here is a simple example demonstrating how to use the Boost library to manipulate strings:
#include <boost/algorithm/string.hpp>
int main() {
std::string s = "Boost Libraries";
boost::to_upper(s);
// s now contains "BOOST LIBRARIES"
return 0;
}
In the example, the `to_upper` function transforms all characters in the string to uppercase, showcasing Boost's straightforward usage.
Qt Framework
Qt is another powerful extension primarily used for building graphical user interfaces. It supports cross-platform development, enabling developers to write code once and deploy it on various operating systems without significant modifications.
Using Qt can simplify the creation of rich UI applications. Here’s a basic example of a Qt application:
#include <QApplication>
#include <QPushButton>
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
QPushButton button("Hello, World!");
button.show();
return app.exec();
}
In this snippet, we create a simple button labeled "Hello, World!" The code illustrates the ease with which developers can create a user interface with Qt.
OpenCV - Computer Vision Library
OpenCV stands out in the sphere of image processing and computer vision. It is an open-source library that provides numerous algorithms for processing images and performing machine learning tasks.
For instance, loading and displaying an image with OpenCV can be accomplished with just a few lines of code:
#include <opencv2/opencv.hpp>
int main() {
cv::Mat image = cv::imread("image.jpg");
if (!image.empty()) {
cv::imshow("Displayed Image", image);
cv::waitKey(0);
}
return 0;
}
This example illustrates how effortlessly OpenCV allows you to handle image files. The function `imread` loads an image, and `imshow` presents it in a window.
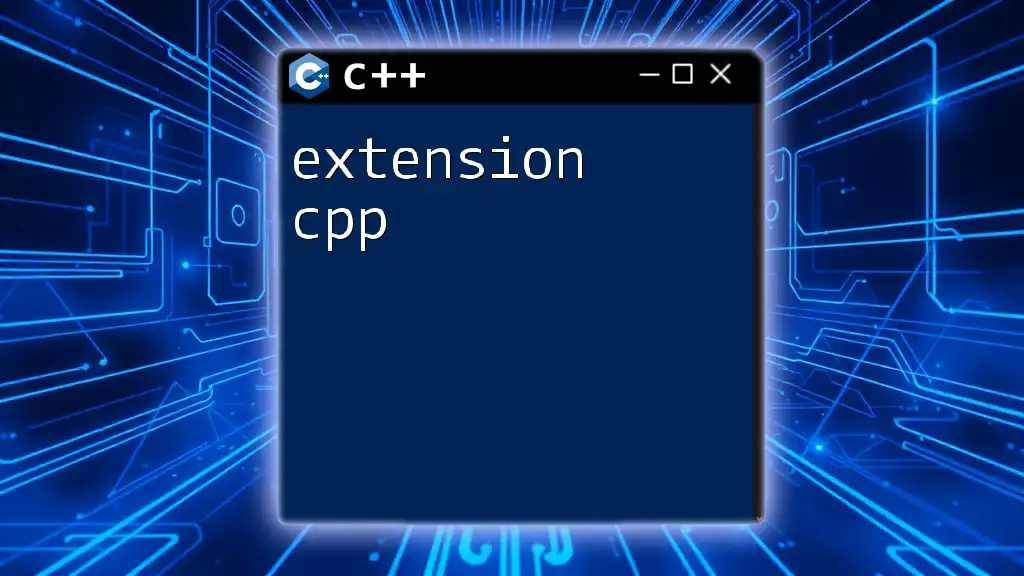
How to Implement C++ Extensions in Your Projects
Setting Up Your Development Environment
Before diving deep into using C++ extensions, it is essential to correctly set up your development environment. Popular IDEs like Visual Studio or Code::Blocks can greatly simplify this process. Additionally, using package managers such as vcpkg or Conan allows for automated management of dependencies, making it easier to install and update C++ libraries.
Installing C++ Extensions
Installing a C++ extension often involves downloading the library and configuring your project files accordingly. For example, when installing Boost, you would typically:
- Download Boost from the official website.
- Extract the files to a specified directory.
- Configure your project settings to include the Boost header files and link against the Boost libraries.
Linking Extensions in Your Project
Linking libraries in a C++ project can vary depending on the IDE or build system being used. Understanding the difference between static and dynamic linking is crucial:
- Static Linking: This involves copying all used libraries into the final executable. It increases the size of the executable but simplifies deployment since no external library files are required.
- Dynamic Linking: In this case, external library files are referenced at runtime, reducing the executable size but requiring these files to be present on the system where the program is executed.
Knowing when to use each method involves evaluating your project requirements and the distribution model.
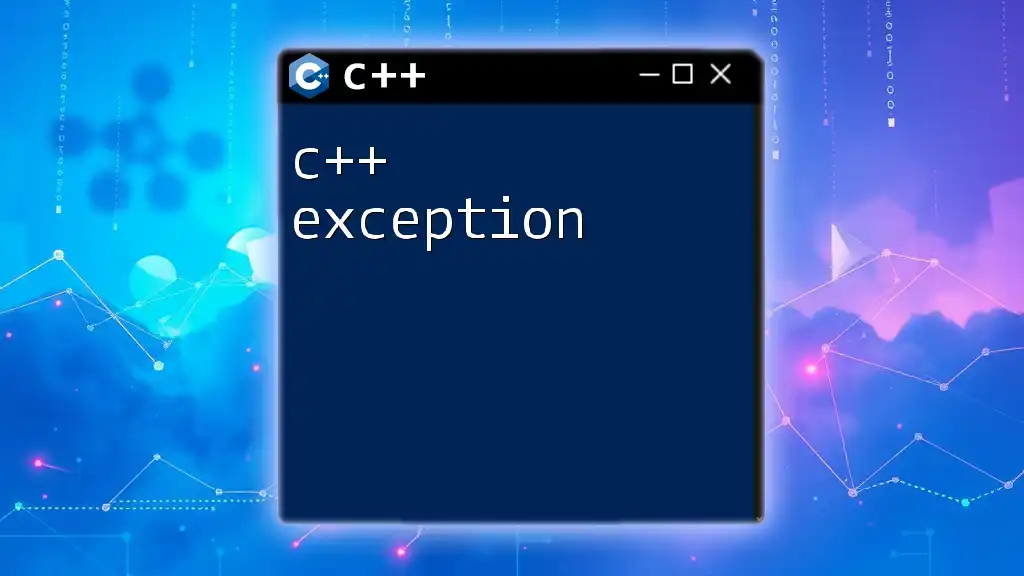
Best Practices for Using C++ Extensions
Choosing the Right Extension for Your Project
Selecting an appropriate C++ extension can significantly impact the success of your project. Consider the following factors:
- Functionality: Ensure the extension meets the specific needs of your project.
- Documentation: Look for well-maintained documentation, as it is invaluable for troubleshooting and learning.
- Community Support: An active community can provide helpful resources and solutions to common problems.
Version Compatibility
Version compatibility is a critical concern when using C++ extensions. It is essential to ensure that the extensions you are using will work seamlessly with the specific version of C++ you are compiling against. Additionally, you should always be cautious about updating extensions, as newer versions may introduce breaking changes.
Troubleshooting Common Issues
Working with C++ extensions can sometimes lead to challenges. Common issues may include missing dependencies, version mismatches, or integration problems. A systematic approach to troubleshooting— such as consulting documentation, community forums, and using debugging tools—can effectively resolve most issues.
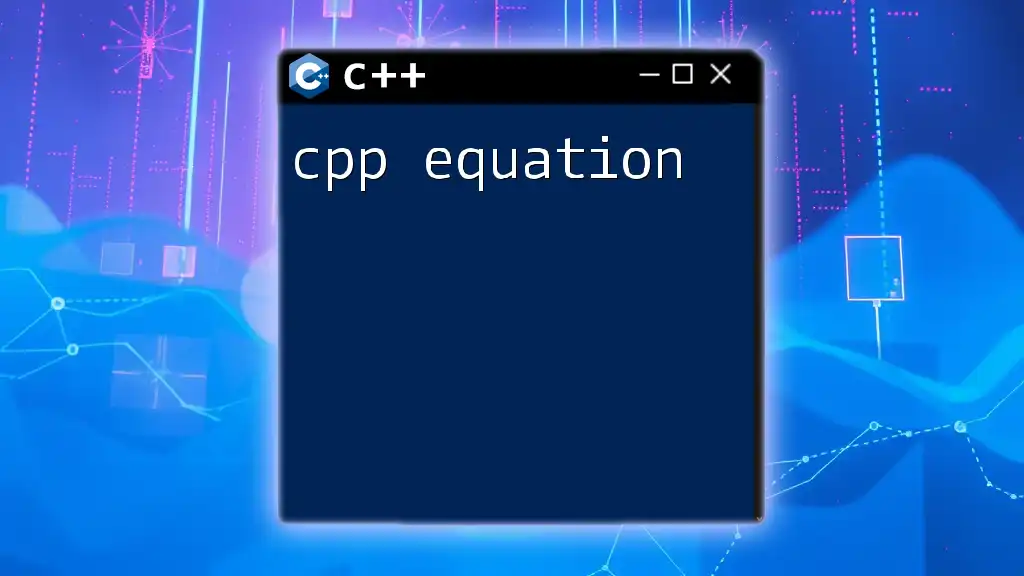
Conclusion
Incorporating C++ extensions into your projects not only enhances development efficiency but also elevates the quality of your software. By embracing these specialized tools, you empower yourself to create robust, maintainable, and feature-rich applications. As you explore different extensions, don’t hesitate to experiment and see which combinations yield the best results for your specific programming needs.
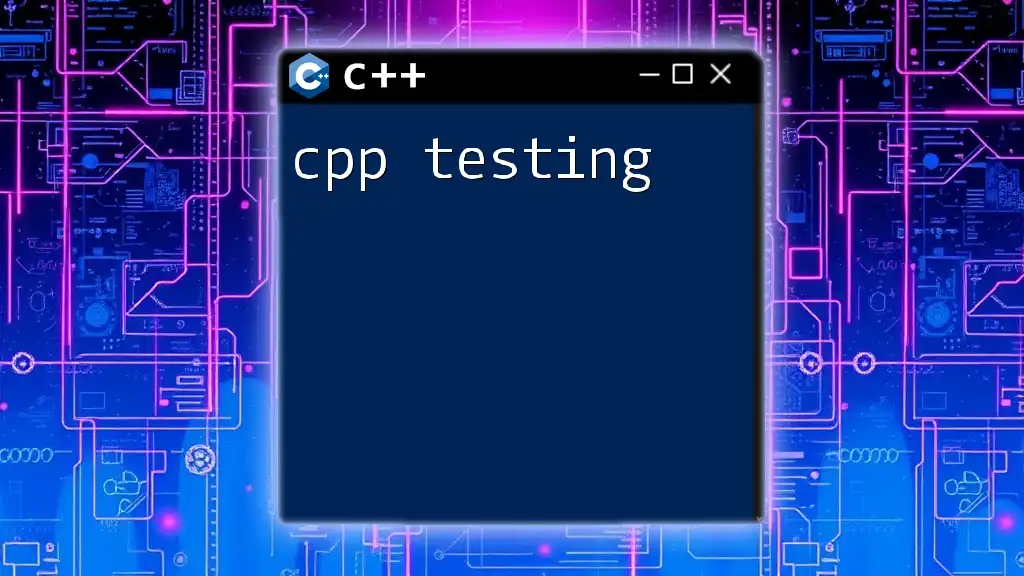
Further Reading and Resources
For those eager to expand their knowledge of C++ extensions, consider exploring recommended books, websites, and forums dedicated to C++ development. Additionally, ensure you check out the official documentation for popular extensions like Boost, Qt, and OpenCV. These resources can provide deeper insights and further enhance your programming skills.