C++ exercises are practical tasks designed to help learners master C++ syntax and commands through hands-on coding experience.
Here's a simple example that demonstrates the use of a for loop to print numbers from 1 to 5:
#include <iostream>
int main() {
for (int i = 1; i <= 5; i++) {
std::cout << i << std::endl;
}
return 0;
}
Understanding C++ Exercises
C++ exercises are practical tasks designed to help individuals learn and enhance their programming skills in the C++ language. These exercises range from basic syntax and logic to more complex algorithms and data structure implementations. The practice is crucial for reinforcing concepts, enhancing problem-solving skills, and building confidence to tackle real-world programming challenges.
Why Focus on C++ for Beginners?
C++ is a versatile language widely used in various domains, including software development, game programming, and systems engineering. With its ability to manage low-level system resources while also supporting high-level programming paradigms, C++ serves as a foundational language for many aspiring developers. By focusing on C++, beginners can learn essential programming concepts that are transferable to other languages.
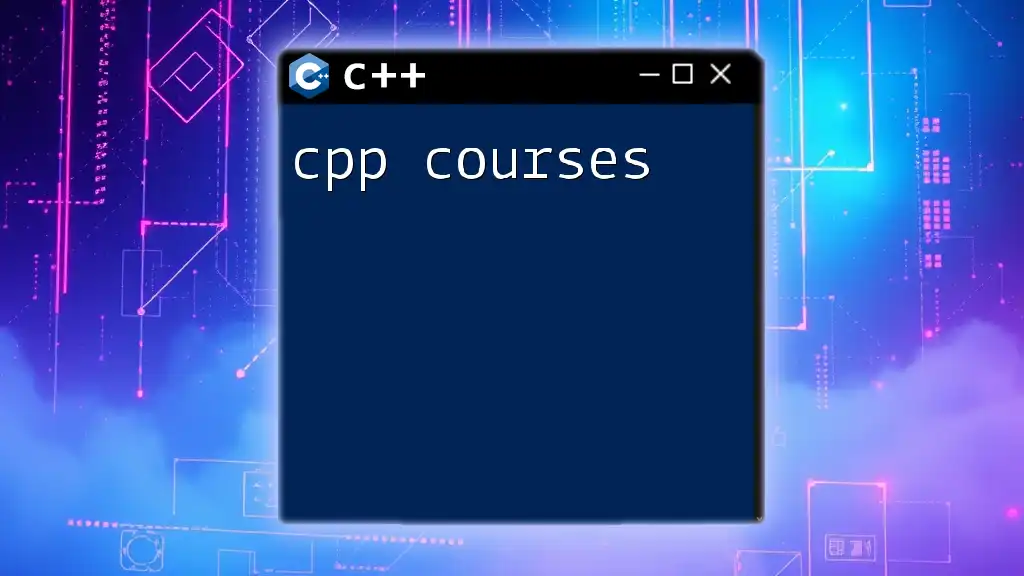
Getting Started with C++ Exercises
Key Concepts Every C++ Beginner Should Know
Before diving into C++ exercises, it’s important for beginners to grasp fundamental concepts:
-
Variables: These serve as storage locations in a program that hold data values. Understanding data types such as integers, floats, and characters is essential.
-
Control Structures: These include conditionals (like `if` and `switch`) and loops (such as `for` and `while`). Mastery of these constructs is vital for controlling the flow of programs.
Setting Up Your C++ Environment
To start practicing, you'll need a C++ development environment. Choose an integrated development environment (IDE) such as:
- Visual Studio
- Code::Blocks
- CLion
Once you've installed an IDE, create your first C++ program to verify that everything is set up correctly. This simple test will help you familiarize yourself with the environment and the process of compiling and executing a C++ program.
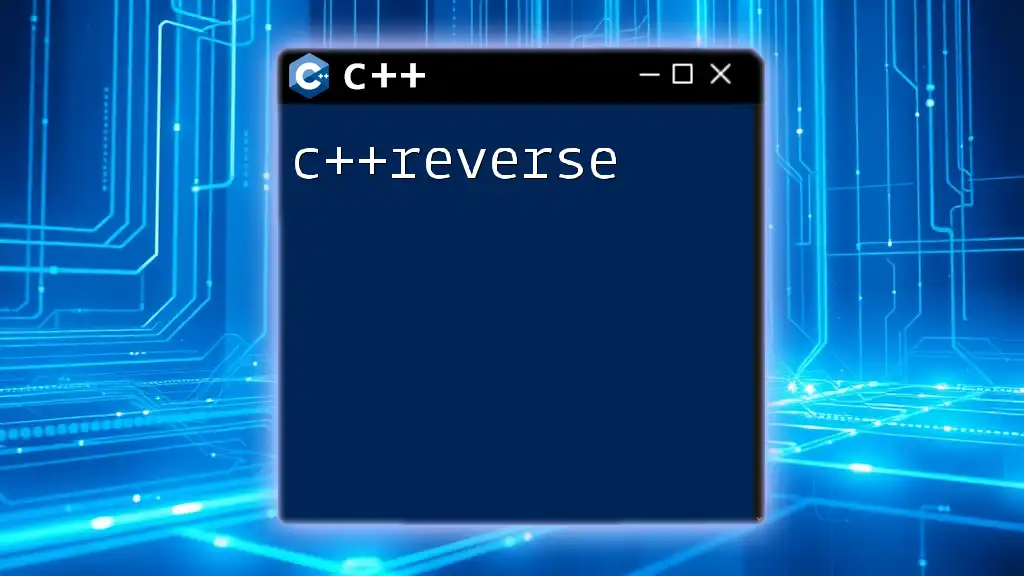
Types of C++ Exercises
Beginner C++ Exercises
When starting your journey, focusing on beginner-friendly exercises is crucial. These exercises often emphasize core syntax and simple logic, allowing new programmers to build confidence in their coding abilities.
C++ Beginner Practice Problems
A great starting point for practice is the "Hello World" program, which introduces the basic structure of a C++ application.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Explanation: This code snippet prints "Hello, World!" to the console, showcasing the use of the `iostream` library and the `main` function.
Another beginner exercise is to create a program that calculates the sum of two numbers:
#include <iostream>
int main() {
int a, b;
std::cout << "Enter two numbers: ";
std::cin >> a >> b;
std::cout << "Sum: " << a + b << std::endl;
return 0;
}
Explanation: This example demonstrates user input and output, as well as arithmetic operations, which are fundamental skills for any budding programmer.
C++ Coding Exercises for Beginners
As you progress, it's essential to tackle more engaging challenges, such as calculating the factorial of a number using loops. This not only reinforces your understanding of loops but also enhances your problem-solving skills:
#include <iostream>
int main() {
int n, factorial = 1;
std::cout << "Enter a positive integer: ";
std::cin >> n;
for(int i = 1; i <= n; ++i) {
factorial *= i;
}
std::cout << "Factorial: " << factorial << std::endl;
return 0;
}
Explanation: In this code, we use a `for` loop to multiply numbers sequentially, demonstrating the concept of iteration in a practical way.
A classic coding challenge for beginners is the FizzBuzz problem, which reinforces decision-making using conditionals:
#include <iostream>
int main() {
for(int i = 1; i <= 100; i++) {
if (i % 15 == 0)
std::cout << "FizzBuzz\n";
else if (i % 3 == 0)
std::cout << "Fizz\n";
else if (i % 5 == 0)
std::cout << "Buzz\n";
else
std::cout << i << "\n";
}
return 0;
}
Explanation: This program prints numbers from 1 to 100, but for multiples of 3, it prints "Fizz" instead, and for multiples of 5, it prints "Buzz." For numbers that are multiples of both 3 and 5, it prints "FizzBuzz."
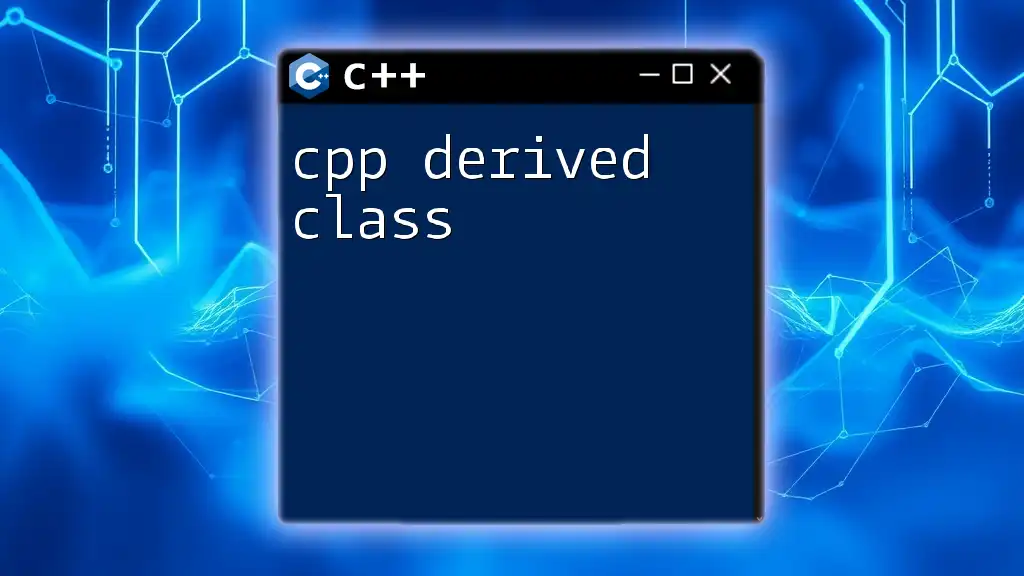
Advanced C++ Programming Exercises
Transitioning from Beginner to Intermediate
Once you are comfortable with basic exercises, it's beneficial to challenge yourself with intermediate programming problems. These exercises generally require combining multiple concepts and can significantly improve your skills.
Examples of Intermediate Programming Exercises
A prime example of an intermediate exercise is checking whether a number is prime:
#include <iostream>
bool isPrime(int n) {
if(n <= 1) return false;
for(int i = 2; i <= n/2; i++) {
if(n % i == 0) return false;
}
return true;
}
Explanation: This function checks if a number `n` is prime by testing for divisors from 2 to `n/2`. This reinforces concepts of functions and control structures while also introducing more complex logic.
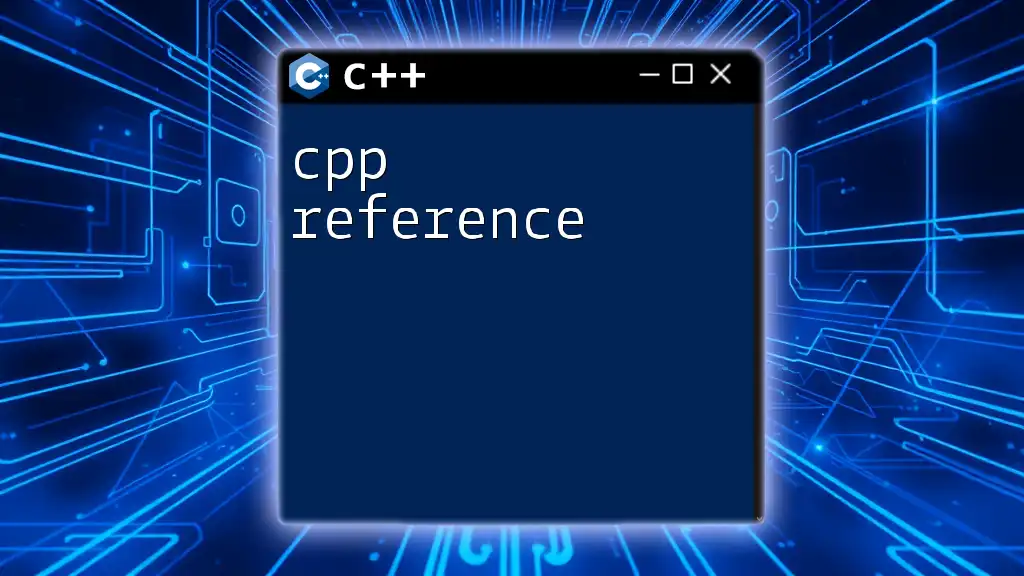
Resources for C++ Programming Practice
Online Platforms to Find C++ Exercises
There are numerous websites where you can find C++ exercises. Some popular options include:
- LeetCode: A platform known for coding challenges that span various difficulty levels.
- HackerRank: Provides practice problems and competitions that focus on various programming skills.
- Codecademy: Offers interactive courses that include C++ programming exercises.
Books and Materials for Further Learning
Investing in good literature can greatly enhance your learning experience. Recommended books for beginners include:
- "C++ Primer" by Stanley B. Lippman
- "Programming: Principles and Practice Using C++" by Bjarne Stroustrup
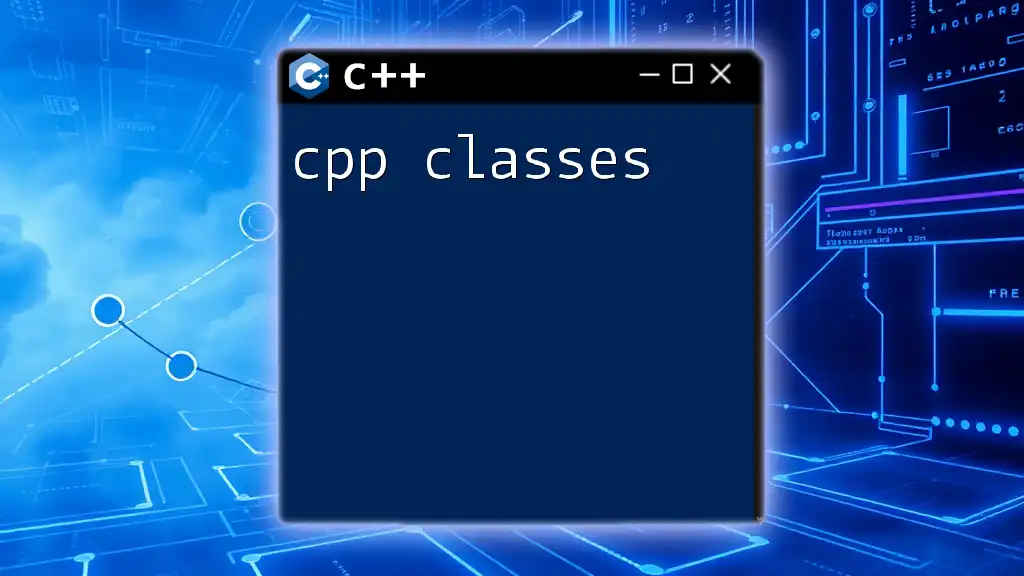
Tips for Effective Practice
How to Approach C++ Exercises for Maximum Benefit
Consistency is crucial in mastering C++. Set aside regular time weekly to practice these exercises.
Methodical Practice Techniques: Structure your practice sessions to focus on specific concepts each time. For instance, dedicate one session to loops, another to arrays, and so forth.
Review and Reflect: After completing exercises, revisit your code. Ask yourself if there are more efficient solutions. Refactoring your code can provide deeper insights into best practices.
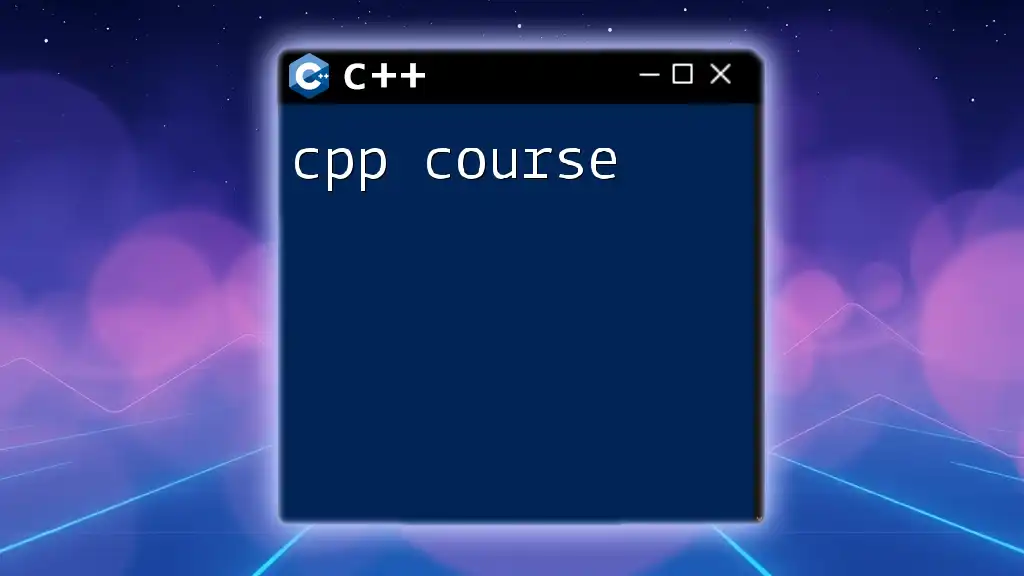
Conclusion
Engaging in C++ exercises not only enhances your coding skills but also builds a strong programming foundation. As you work through exercises, remember that programming is a journey. Continuous practice, exploration of more difficult problems, and learning from mistakes is key to becoming a proficient C++ programmer.
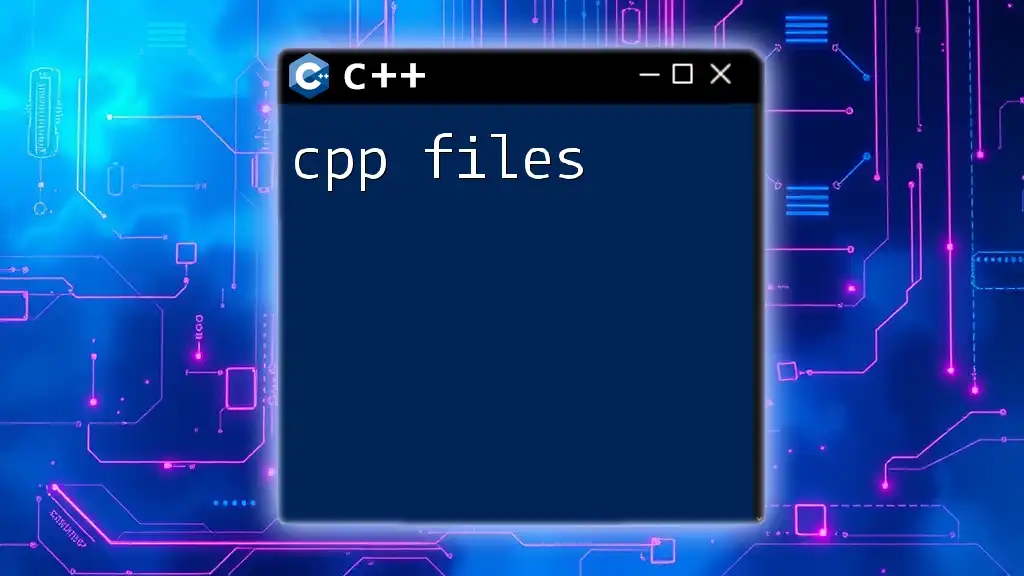
Call to Action
Join our community of aspiring C++ programmers! Share your progress, seek assistance, and explore additional resources to enhance your skills even further. Let's embark on this journey together and master C++ programming!