In C++, a pointer is used to store the memory address of a variable, allowing for direct access and manipulation of the variable's value at that address.
int main() {
int x = 10; // Declare an integer variable
int* ptr = &x; // Create a pointer and store the address of x
std::cout << *ptr; // Output the value at the address stored in ptr
return 0;
}
Understanding Addresses in C++
In C++, an address refers to a specific location in memory where data is stored. Every variable in C++ has a memory address, which is crucial for understanding how data is accessed and manipulated in programs.
When you declare a variable, the operating system allocates a block of memory for it, and this block can be identified by its address. Understanding addresses can help you manage memory more effectively and grasp how data types interact within your applications.

Pointers: The Key to Addresses
What are Pointers?
A pointer is a variable that stores the address of another variable. This allows developers to efficiently manipulate memory and create powerful data structures. Pointers are an integral part of C++ programming, enabling dynamic memory management and the creation of complex data types like linked lists and trees.
Declaring and Initializing Pointers
Declaring a pointer involves specifying the data type that the pointer will point to, followed by an asterisk (`*`) to indicate that it is a pointer.
int x = 10;
int *ptr = &x; // Pointer to x
In this example, `ptr` is a pointer that holds the address of the variable `x`. The `&` operator is used to retrieve the address of `x`. Understanding this concept is foundational, as it leads to the next crucial area of pointers: dereferencing.
Dereferencing Pointers
Dereferencing a pointer means accessing the value stored at the address held by the pointer. It is done using the asterisk (`*`).
cout << *ptr; // Outputs: 10
This line of code shows how to retrieve the value that `ptr` points to, which is `10`. Dereferencing is essential because it allows you to manipulate the variable indirectly through its pointer.
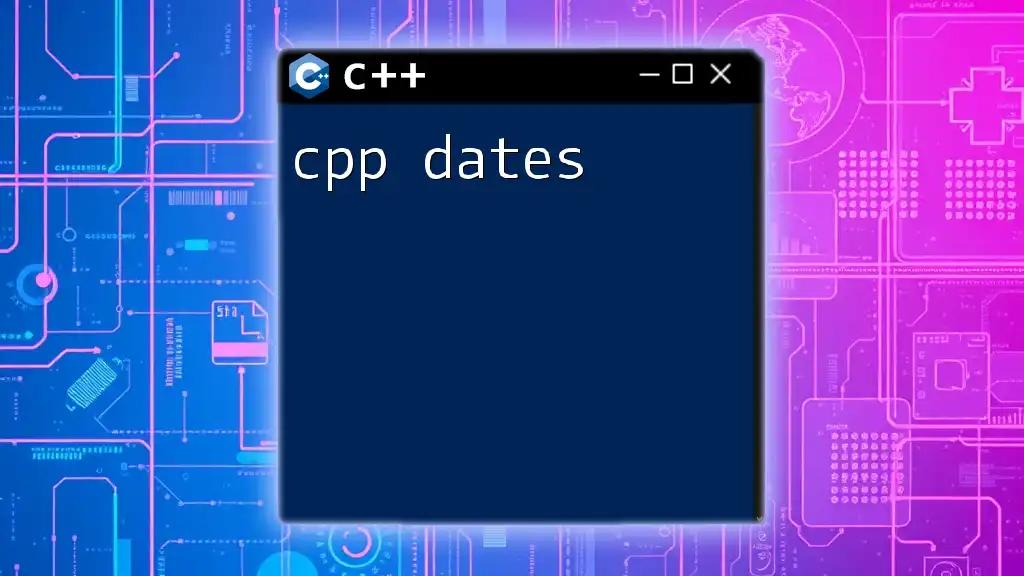
Memory Management
Dynamic Memory Allocation
Dynamic memory allocation is used when you do not know the size of a data structure at compile time. This is common in scenarios where data structures must be flexible in size, such as arrays or linked lists.
Using `new` and `delete`
In C++, you can allocate memory dynamically using the `new` keyword. After using this memory, it’s essential to deallocate it using the `delete` keyword to prevent memory leaks.
int *arr = new int[5]; // allocating array of size 5
delete[] arr; // deallocating memory
In this code snippet, `arr` points to an array of five integers. We then use `delete[] arr` to deallocate the memory block. This practice is critical for effective memory management in your applications.
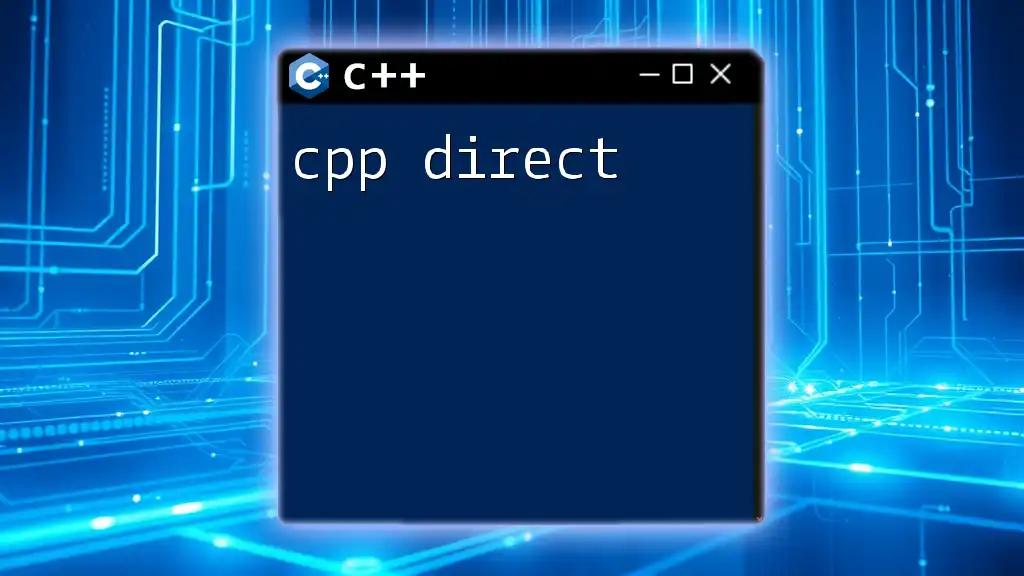
Understanding Memory Addresses and Their Types
Stack vs. Heap Memory
In C++, memory can be allocated on two major types of storage: the stack and the heap.
- Stack Memory: Memory is automatically managed, and variables declared within a function's scope are stored here. When the function exits, the memory is automatically released.
- Heap Memory: Memory is manually managed by the programmer. Since allocations here remain until explicitly freed, this allows for greater flexibility in memory usage.
Choosing between stack and heap memory is a fundamental decision in C++ programming, depending on the requirements of your application.
Address of Operators
The `&` operator is vital in obtaining the address of a variable. This operator can be used wherever you need to know the memory location of a variable.
cout << "Address of x: " << &x << endl;
This code will print the memory address where `x` is stored. Understanding how to use the address operator effectively can help debug memory-related issues in your applications.

Address Calculation and Manipulations
Pointer Arithmetic
Pointer arithmetic involves performing arithmetic operations on pointers, allowing you to traverse through arrays or data structures directly through their memory addresses.
int arr[5] = {1, 2, 3, 4, 5};
int *p = arr; // Pointing to the first element
p++; // Now points to the second element
In this snippet, defining pointer `p` to point to the array `arr` allows you to increment it to point to the next element. Understanding pointer arithmetic can significantly enhance your programs' efficiency and logic.

References: An Alternate Address
What Are References?
A reference in C++ is an alias for another variable, allowing for direct manipulation of that variable without using pointers. References are generally easier to use than pointers because they don't require dereferencing.
Using References Effectively
Here's an example demonstrating the use of references:
void increment(int &num) { num++; }
int a = 5;
increment(a); // a now becomes 6
In this code, `increment` is a function that accepts a reference to an integer. When `a` is passed to this function, its value is directly modified to `6`. Understanding references is vital as they offer an alternative method to work with memory addresses while simplifying code management.
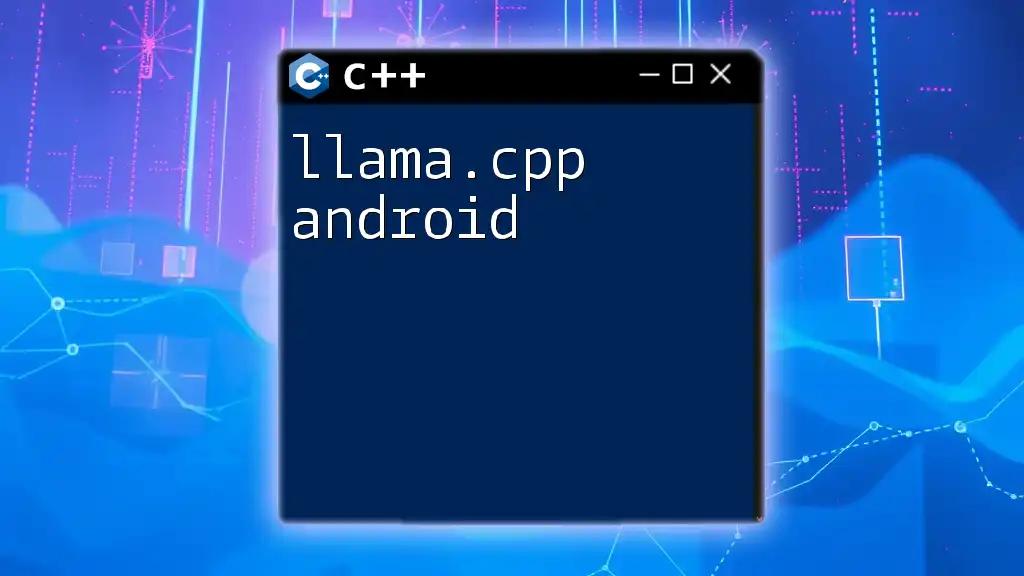
Best Practices and Tips for Handling Addresses
When working with addresses in C++, there are several best practices to follow:
- Avoid Dangling Pointers: Ensure that pointers do not reference memory that has already been deallocated.
- Prevent Memory Leaks: Always pair `new` with `delete` to free dynamically allocated memory.
- Use Smart Pointers: In modern C++, consider using smart pointers like `std::unique_ptr` or `std::shared_ptr` to manage memory automatically and minimize errors.
Implementing these practices can improve the robustness and reliability of your C++ applications.

Real-World Applications of Addresses in C++
Addresses play a critical role in numerous applications, particularly in systems programming and game development. They allow developers to manipulate memory directly for performance optimization and to implement data structures that require dynamic memory. Understanding how to work with these addresses can yield significant performance gains and lead to effective resource management in your applications.

Conclusion
In summary, grasping the concept of `cpp address` is crucial for effective C++ programming. From understanding pointers and memory management to utilizing references, every aspect contributes to developing robust and efficient applications. I encourage you to practice these concepts to gain a deeper comprehension and enhance your programming skills.

Additional Resources
For further learning, consider exploring the official C++ documentation, joining online forums, or enrolling in C++ courses. Engaging with community resources can deepen your knowledge and support your development journey as you master C++.