AddressSanitizer is a powerful memory error detector for C++ that helps identify issues like buffer overflows and use-after-free errors during runtime by instrumenting the code at compile time.
Here’s a simple example of how to compile a C++ program with AddressSanitizer enabled:
// Example C++ code that may have a memory error
#include <iostream>
void causeError() {
int *arr = new int[5];
delete[] arr;
std::cout << arr[0]; // Use-after-free error
}
int main() {
causeError();
return 0;
}
To compile this code with AddressSanitizer, you would use the following command:
g++ -fsanitize=address -g example.cpp -o example
What is Address Sanitizer?
Address Sanitizer (ASan) is a powerful memory error detector that helps C++ developers identify and debug memory-related issues. It functions by instrumenting the code at compile time, allowing it to track memory allocations, detect overruns and illegal accesses, and pinpoint memory leaks. By using Address Sanitizer, developers can significantly improve the reliability and stability of their C++ applications by catching common bugs early in the development stage.
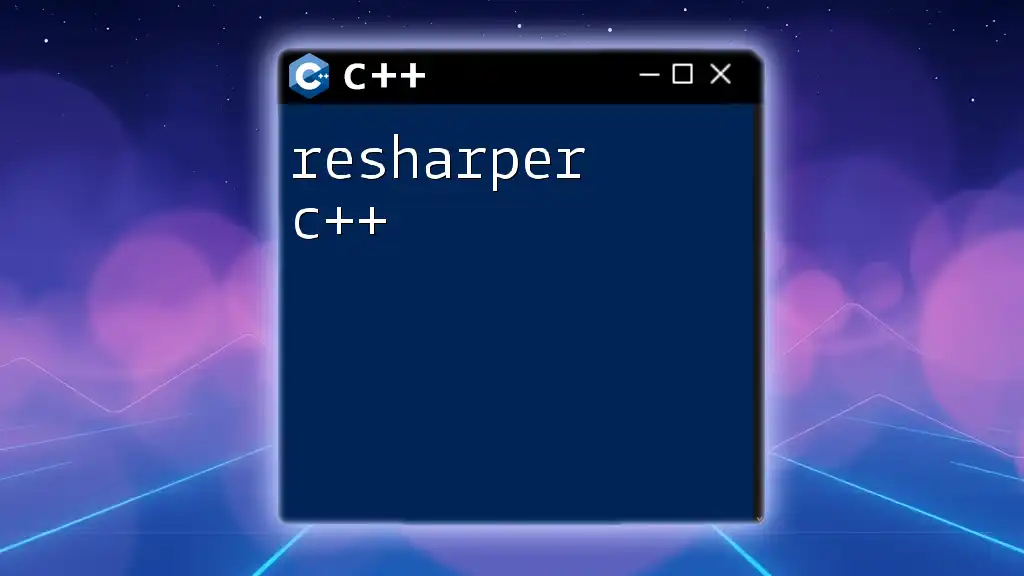
Setting Up Address Sanitizer
Compiler Requirements
Address Sanitizer is supported by major C++ compilers such as GCC and Clang. To make full use of ASan, ensure that you are using a version of these compilers that supports the feature, as ASan implementation may vary between versions.
Enabling Address Sanitizer
To enable Address Sanitizer, you need to compile your code with specific flags. Here’s a straightforward guide for various platforms:
-
Linux and macOS: You can enable Address Sanitizer by adding the following flag to your compilation command:
g++ -fsanitize=address -g my_program.cpp -o my_program
-
Windows: The installation of Clang along with an appropriate build system will allow you to use ASan similarly:
clang++ -fsanitize=address -g my_program.cpp -o my_program
Using the `-g` flag enables debugging information, which is essential for interpreting ASan's error reports effectively.
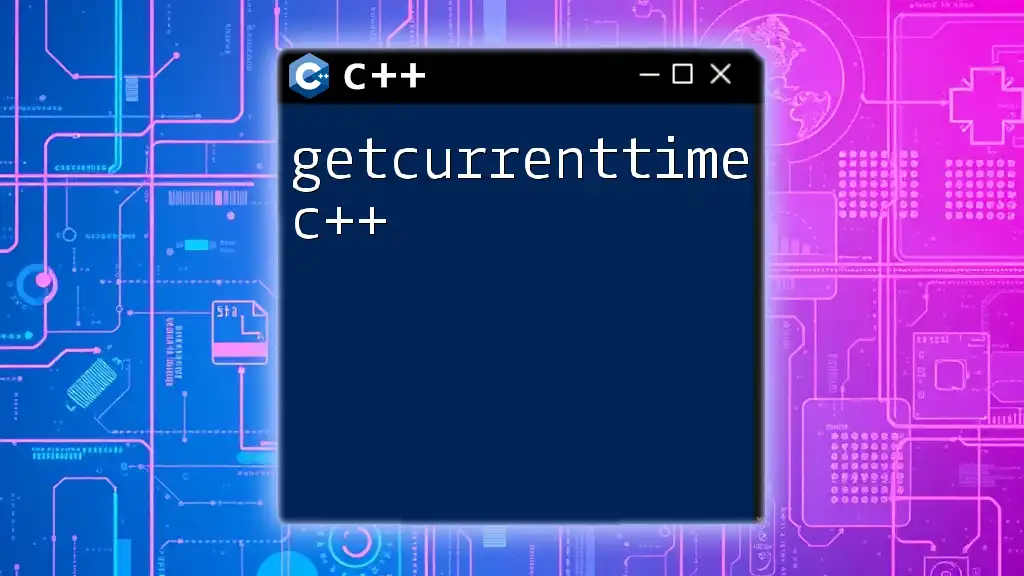
How Address Sanitizer Works
Memory Layout and Shadow Memory
At the core of ASan’s functionality is shadow memory. When you enable Address Sanitizer, it allocates a shadow memory region where it tracks the status of memory blocks. This shadow memory helps in maintaining a mapping between application memory and its status (e.g., allocated, freed, or in-use).
This approach allows ASan to detect errors like buffer overflows or use-after-free occurrences by monitoring the integrity of the memory accessed by your program.
Instrumenting Code
To provide robust memory error detection, ASan instruments the binary at compile time. This means that each memory allocation and deallocation is wrapped in ASan’s custom handlers, which log relevant metadata. While this instrumentation is powerful, it comes with a trade-off: the overhead can result in slower execution speed when running under ASan.
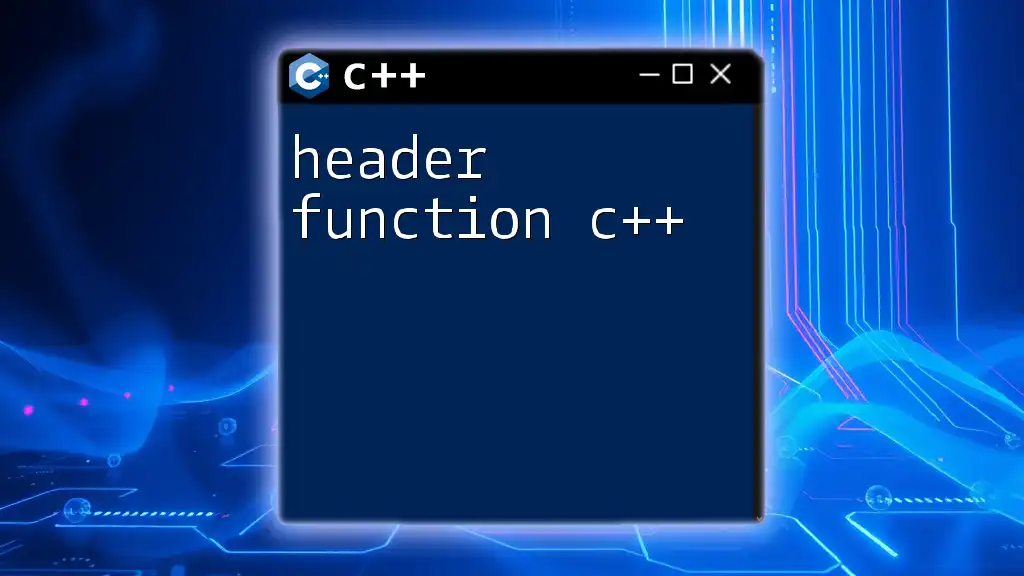
Common Errors Detected by Address Sanitizer
Buffer Overflows
A buffer overflow occurs when a program writes more data to a buffer than it is allocated. ASan can detect these kinds of errors effectively.
Example of a Buffer Overflow:
void bufferOverflow() {
char buffer[10];
for (int i = 0; i < 15; i++) {
buffer[i] = 'A'; // This will lead to an overflow
}
}
When this code is run with Address Sanitizer enabled, it will alert you about the overflow and provide details on where it occurred.
Use After Free
Use After Free errors happen when a program continues to use a pointer after the memory it points to has been released. ASan helps by detecting these accesses.
Example of Use After Free:
void useAfterFree() {
int* ptr = new int(42);
delete ptr;
*ptr = 100; // Use after free
}
If you run this code with ASan, it will notify you that you're accessing memory that has already been freed.
Memory Leaks
Memory leaks occur when allocated memory is not freed, leading to unnecessary memory consumption. ASan makes it easier to identify memory leaks during development.
Example of a Memory Leak:
void memoryLeak() {
int* leak = new int[100]; // Memory allocated but never freed
}
Once executed with Address Sanitizer, it will report the leak, helping you identify and rectify the issue before it becomes a problem in production.
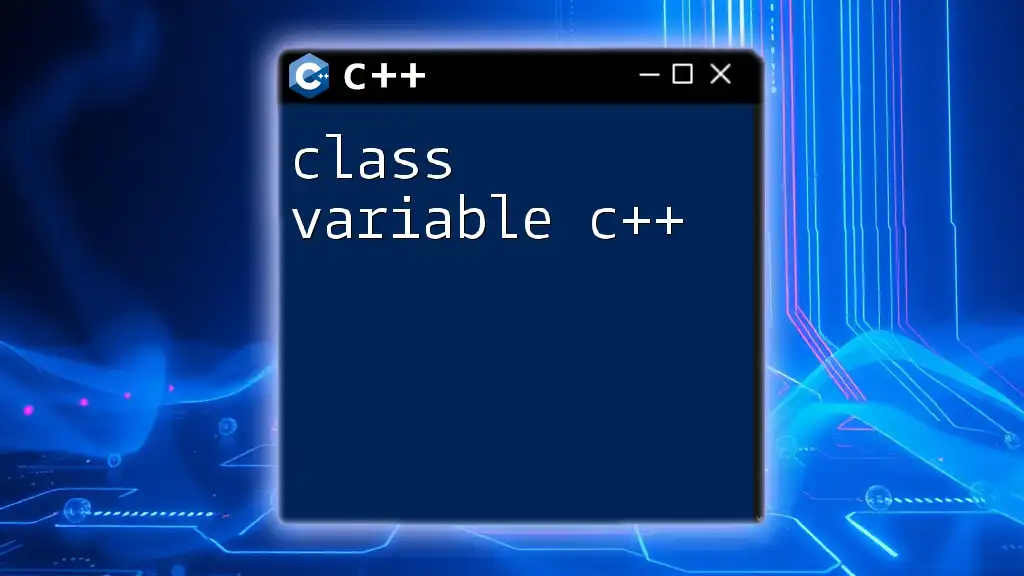
Interpreting Address Sanitizer Output
Understanding the output generated by ASan is crucial for effective debugging.
Understanding Error Messages
When ASan detects an error, it provides a detailed report that includes:
- Type of error (e.g., buffer overflow, use after free)
- Memory address involved
- Stack trace leading up to the error
For instance, a typical ASan output for a detected error may look like this:
==12345==ERROR: AddressSanitizer: stack-buffer-overflow on address 0x...
This output highlights the exact location in your code where the issue occurred, making it easier to track down and fix the problem.
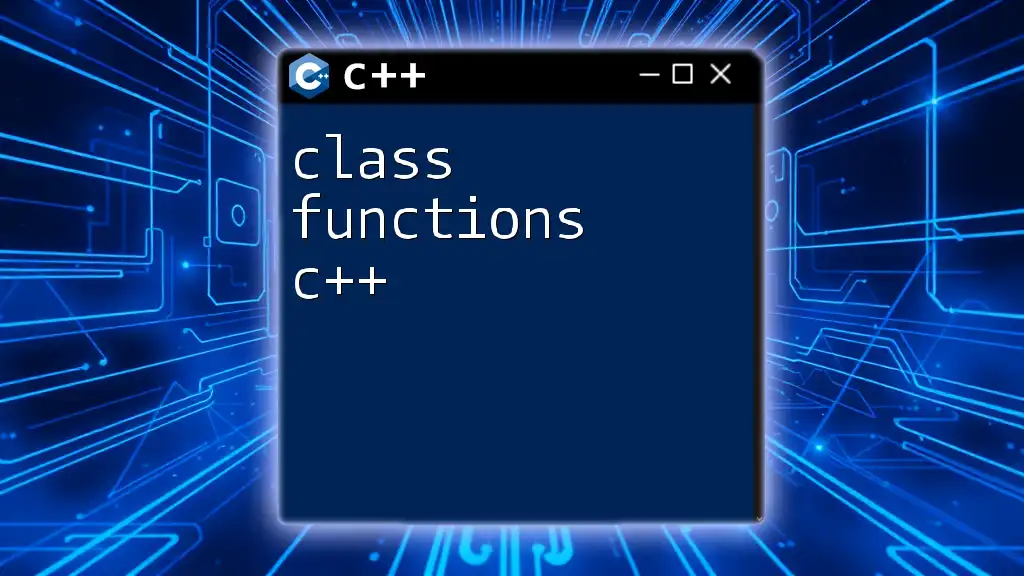
Best Practices for Using Address Sanitizer
To maximize the benefits of Address Sanitizer, consider integrating it regularly into your development workflow. Run your tests with ASan enabled to catch memory issues early. When issues are reported:
- Analyze the reports provided by ASan thoroughly.
- Implement fixes and retest to ensure that the problems are resolved.
- Combine ASan with other tools like Valgrind for a comprehensive debugging experience.
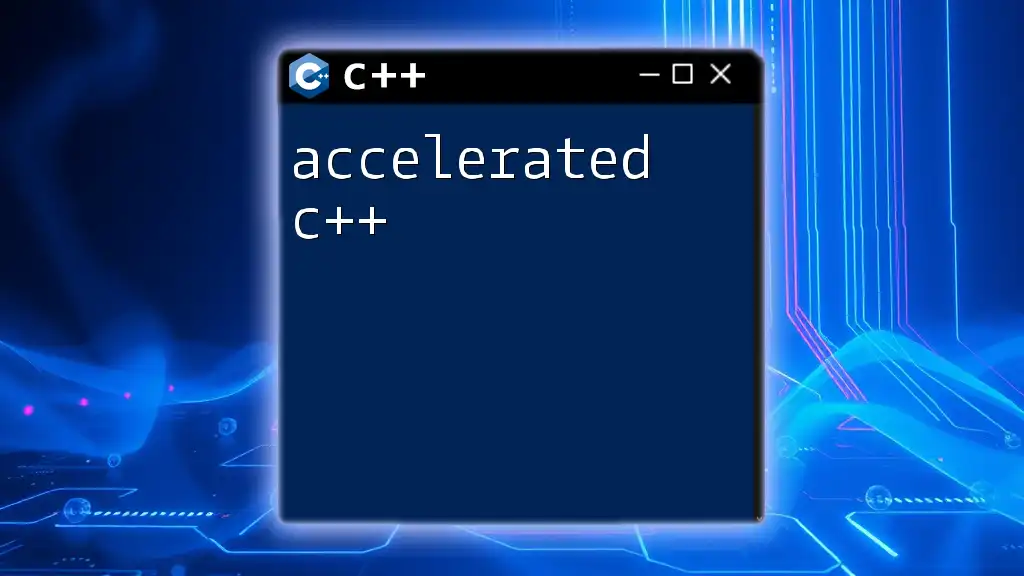
Limitations of Address Sanitizer
Even though Address Sanitizer is a powerful tool, it is important to note its limitations:
- Not a Replacement: ASan does not replace other debugging tools. It is best used in conjunction with them.
- Performance Overhead: The instrumentation can slow down your application, so it is recommended to use it primarily during the testing phase.
- Multi-threading Issues: In multi-threaded applications, ASan may exhibit less reliable results, so caution is needed.
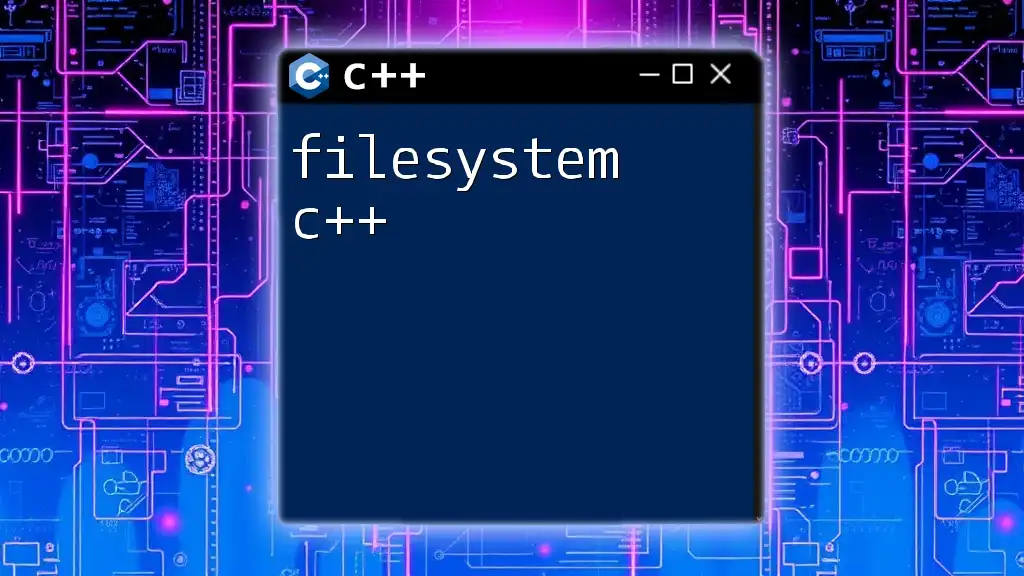
Conclusion
Address Sanitizer offers a robust solution for detecting and debugging memory errors in C++ applications. By integrating ASan into your regular development routine, you can enhance your code's reliability, prevent memory-related bugs, and improve overall application performance. Emphasizing memory safety in your software development process will significantly contribute to high-quality, maintainable code.
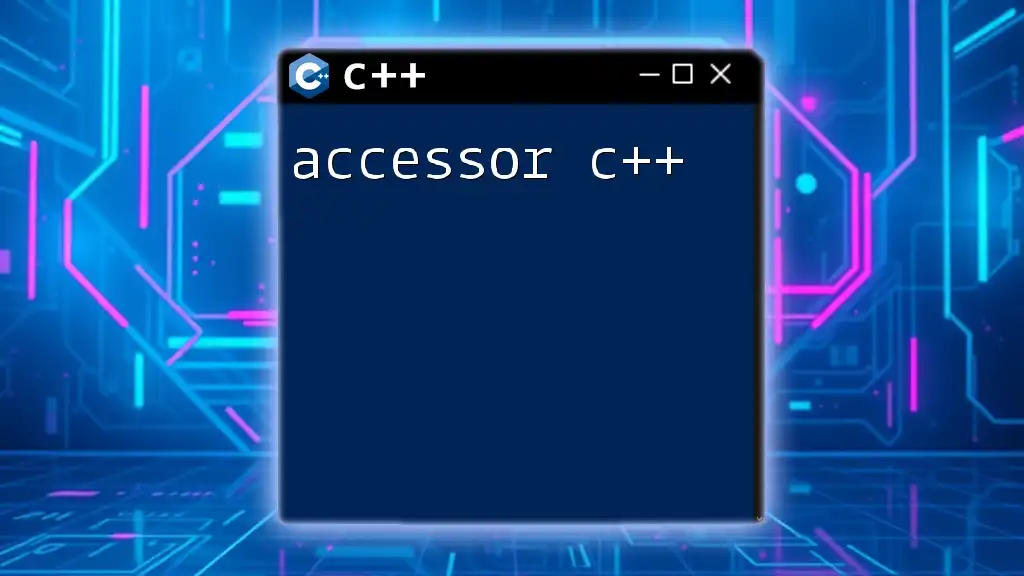
Additional Resources
To further enhance your understanding of Address Sanitizer and memory management in C++, explore the following resources:
- Visit the official documentation for Address Sanitizer on LLVM's website.
- Check out community tutorials and courses focused on C++ memory management techniques.