A "code fixer" in C++ refers to tools or scripts that help identify and correct errors or inefficiencies in C++ code, improving its functionality and readability.
Here's a simple example of a code fixer that corrects a common mistake in C++:
#include <iostream>
int main() {
int num1 = 10; // Fixed variable initialization
int num2 = 20; // Fixed variable initialization
int sum = num1 + num2;
std::cout << "The sum is: " << sum << std::endl; // Fixed output formatting
return 0;
}
Understanding C++ Code Issues
Common C++ Errors
Errors in C++ can largely be classified into two categories: syntax errors and logic errors. Understanding these types will help in formulating effective solutions with your C++ code fixer.
Syntax Errors occur when the code violates the grammatical rules of the C++ language. An example of a syntax error is forgetting to use a semicolon at the end of a statement.
Example Code Snippet:
int main() {
cout << "Hello World" // Missing semicolon
}
Here, the compiler throws an error, preventing the program from compiling until the missing semicolon is added.
Logic Errors, on the other hand, arise when the syntax is correct but the output or the behavior of the program is not what the programmer intended. For instance, a simple mathematical operation can easily go wrong:
Example Code Snippet:
int add(int a, int b) {
return a - b; // Incorrect operation
}
In this case, the function is meant to add two integers but mistakenly subtracts them instead, leading to unexpected results.
The Importance of Code Quality
Maintaining good code quality is essential not only for achieving optimal performance but also for the long-term maintainability of the software. High-quality code is easier to read, understand, and debug, making it particularly valuable in collaborative environments. Prioritizing quality early on will save time and resources later.
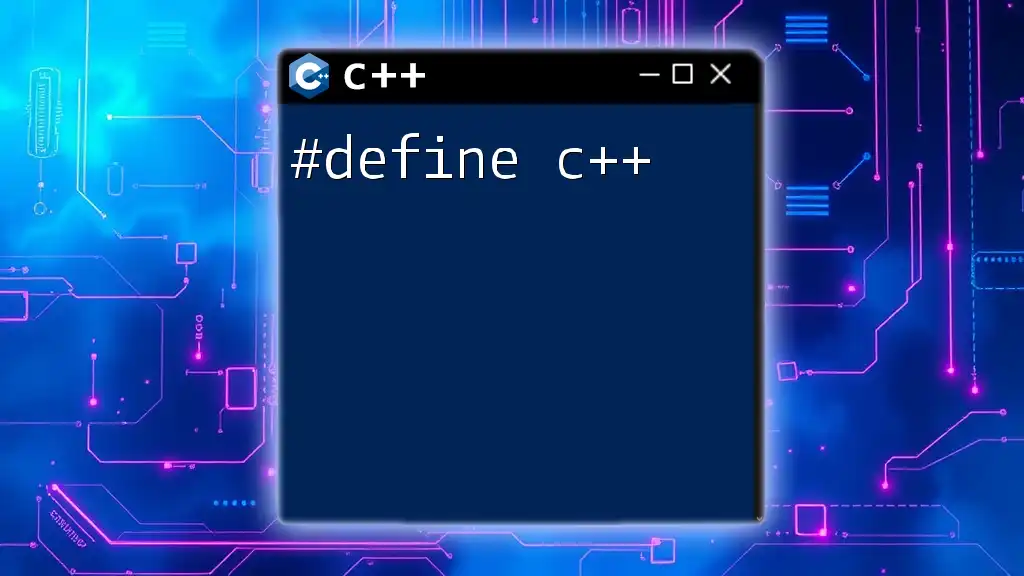
Introducing the Code Fixer C++
What is a Code Fixer?
A C++ code fixer is a tool or software designed to automatically identify and rectify coding errors within C++ programs. The main goals of a code fixer revolve around enhancing code reliability, improving performance, and promoting best practices in coding.
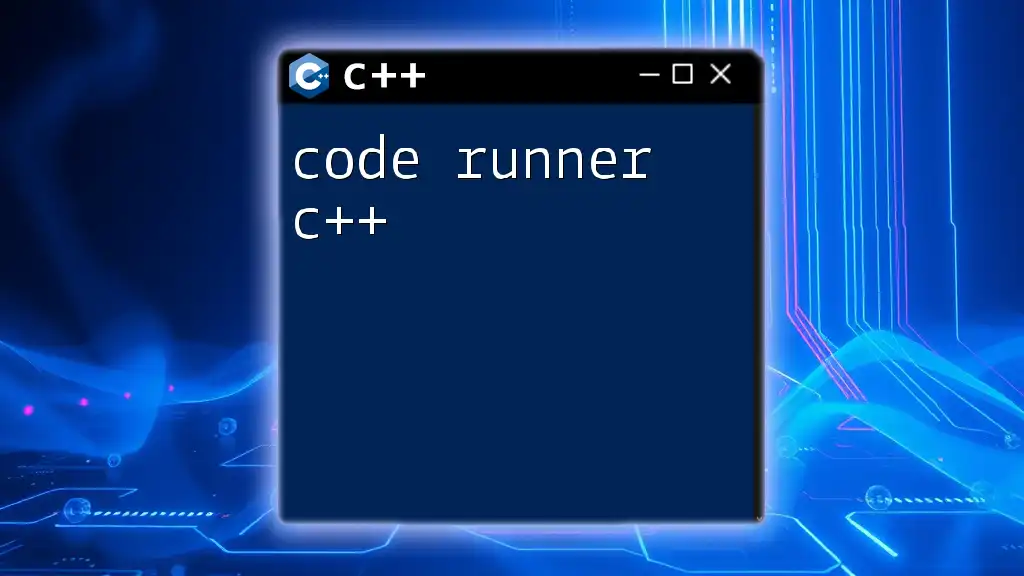
Build Your Own Basic C++ Code Fixer
Setting Up the Environment
Before developing your own code fixer, it is vital to establish a suitable environment. This requires choosing an appropriate Integrated Development Environment (IDE) such as Visual Studio, Code::Blocks, or Eclipse. Additionally, ensure you have a suitable compiler like GCC or Clang installed on your system.
Writing the Core Functionality
The essence of a code fixer lies in its ability to detect errors efficiently.
Function to Detect Syntax Errors
To create a function that detects syntax errors, you'll need to parse the C++ code. This involves checking for common syntax issues, such as missing semicolons, unmatched parentheses, or incorrect declarations.
Example Code Snippet:
bool hasSyntaxError(const std::string& code) {
// Pseudocode: Implementation of syntax checking logic
// 1. Traverse each character in the code
// 2. Monitor open and close brackets
// 3. Check for missed semicolons
// 4. Return true if any errors are detected
}
Function to Detect Logic Errors
Detecting logic errors can be more challenging since it typically requires deeper analysis of the code's intent. One effective strategy is to implement unit tests that validate the expected behavior of the code. A test framework, such as Google Test, can help automate this process and catch discrepancies.
Enhancing the Code Fixer
Integrating Autocomplete Features
To improve the usability of your code fixer, consider integrating autocomplete features. By leveraging libraries such as `libclang`, you can provide suggestions as users type, thereby facilitating quicker and more error-free coding.
Implementing User Feedback Mechanism
An effective code fixer thrives on continuous improvement, largely through user feedback. Establish a simple mechanism for users to report issues or suggest enhancements. Analyzing this input can guide future updates and bolster user satisfaction.
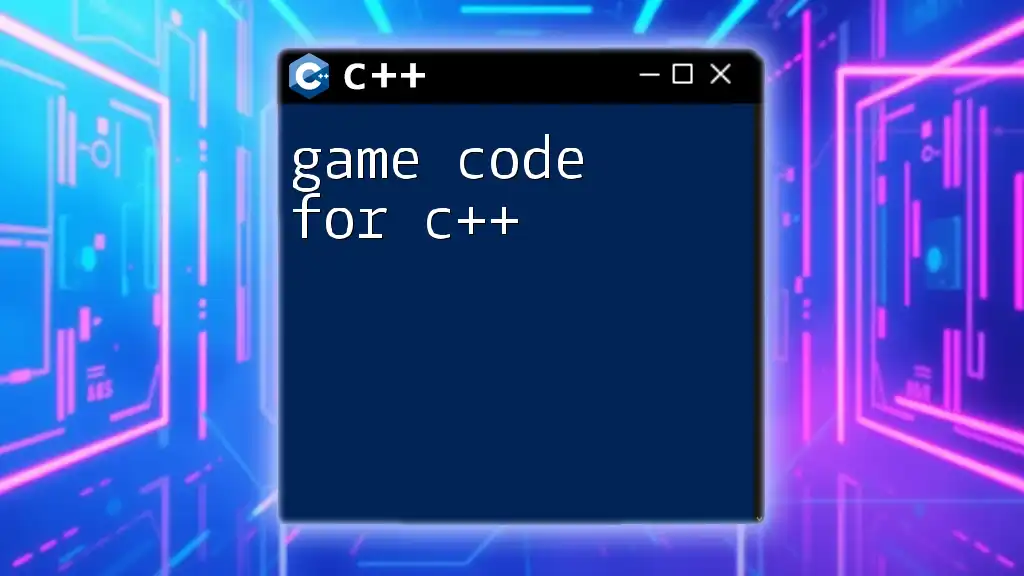
Utilizing Existing Tools for C++ Code Fixing
Popular Code Analysis and Fixing Tools
There are several well-established tools available to aid in C++ code fixing, including:
- Clang-Tidy: An essential tool for linting C++ code, focusing on static analysis and offering suggestions for code improvements.
- CPPCheck: A static analysis tool that identifies various coding issues including memory management problems.
- Visual Studio Code Extensions: Several plugins enhance C++ development by automatically identifying syntax and logic issues.
How to Use These Tools Effectively
To make the most of these tools, start by installing them according to the documentation provided by their respective developers. Use them regularly during the development process to catch errors early.
Practical Example: For instance, if using Clang-Tidy, run it against your project with:
clang-tidy your_code.cpp -- -std=c++11
This command checks your C++ file and applies any suggested fixes.
Comparison of Tools
It is crucial to evaluate the strengths and weaknesses of each tool based on your specific needs. Clang-Tidy is great for quick fixes, while CPPCheck offers deeper analysis of more complex logic errors. Your project requirements and team familiarity with the tools will guide your choice.
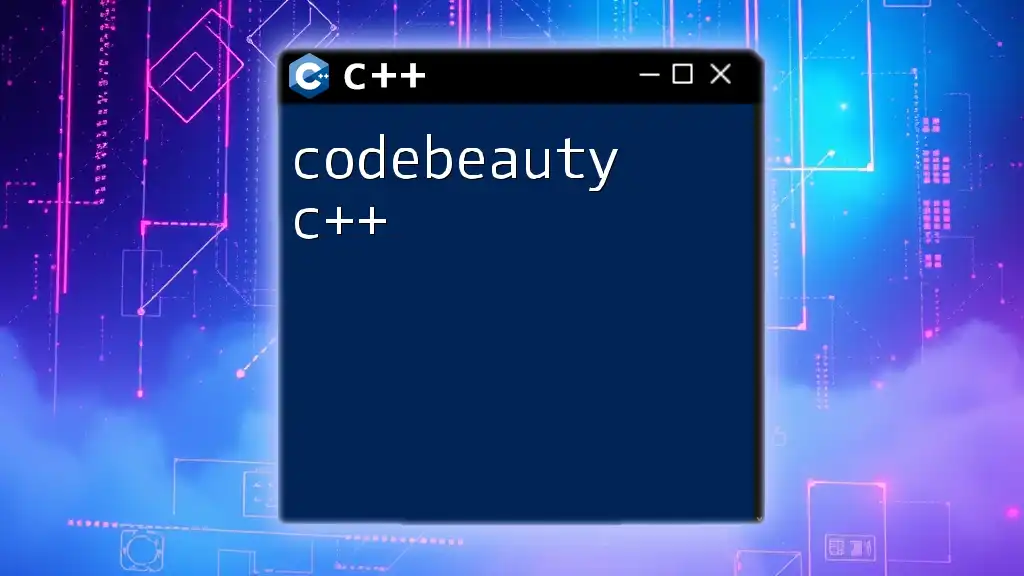
Best Practices for Writing Fixable C++ Code
Code Structure and Organization
A well-structured codebase is foundational for effective fixing. Adopt modular programming techniques by organizing your code into logical units (i.e., classes, functions, and namespaces). This approach makes it easier to isolate and correct errors when they occur.
Documentation and Comments
Incorporate clear documentation and comments to explain your code's functionality. This practice not only aids in future code fixing but also helps others understand your logic. Use comments responsibly, focusing on complex parts to clarify intent.
Example:
// This function adds two integers
int add(int a, int b);
Regular Code Reviews and Refactoring
Conducting routine code reviews is vital to maintaining quality. Peer reviews help spot potential errors and improve the codebase. Additionally, embrace the practice of refactoring code regularly; it helps simplify and optimize your code, making it less error-prone.
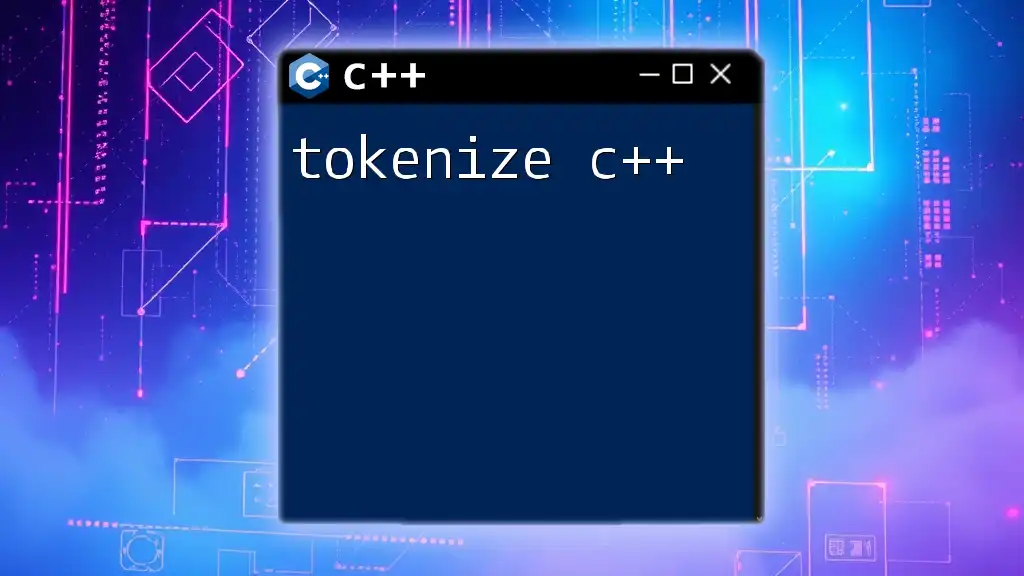
Conclusion
Recap of the Importance of a Code Fixer
In summary, the effectiveness of a code fixer in C++ cannot be overstated. By automating error detection and encouraging best practices, you create a pathway to more reliable and maintainable software.
Resources for Further Learning
For those eager to delve deeper into C++, numerous resources are available, including online courses, books, and community forums. These can enrich your understanding and mastery of C++ programming.
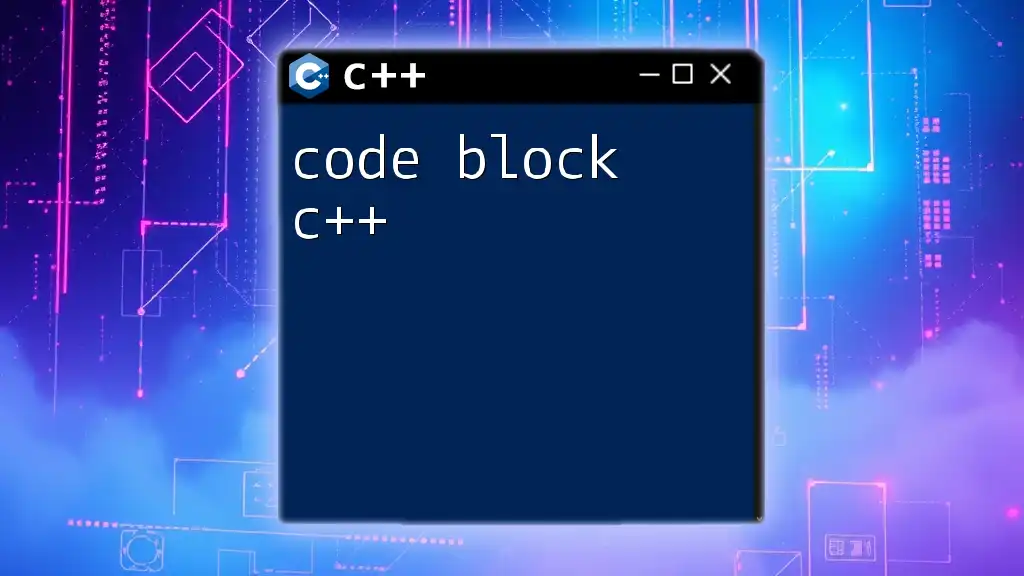
Call to Action
Share your experiences and any tips you've discovered along your coding journey! Engage with the community and explore how our offerings can enhance your skills in crafting error-free C++ code.