The "contains" functionality in C++ can be exemplified by using the `std::string` method `find()` to check if a substring exists within a string.
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, World!";
std::string to_find = "World";
if (str.find(to_find) != std::string::npos) {
std::cout << "The string contains '" << to_find << "'." << std::endl;
} else {
std::cout << "The string does not contain '" << to_find << "'." << std::endl;
}
return 0;
}
What Does "Contains" Mean in C++
In the context of programming, particularly in C++, the term contains refers to the ability to check for the existence of an element within a collection. This is a common requirement in various programming scenarios, such as verifying if a value exists in a list, checking for a key in a map, or determining whether an object is present in a set. Understanding how to implement and utilize the contains functionality is crucial for efficient data handling and manipulation in your C++ applications.
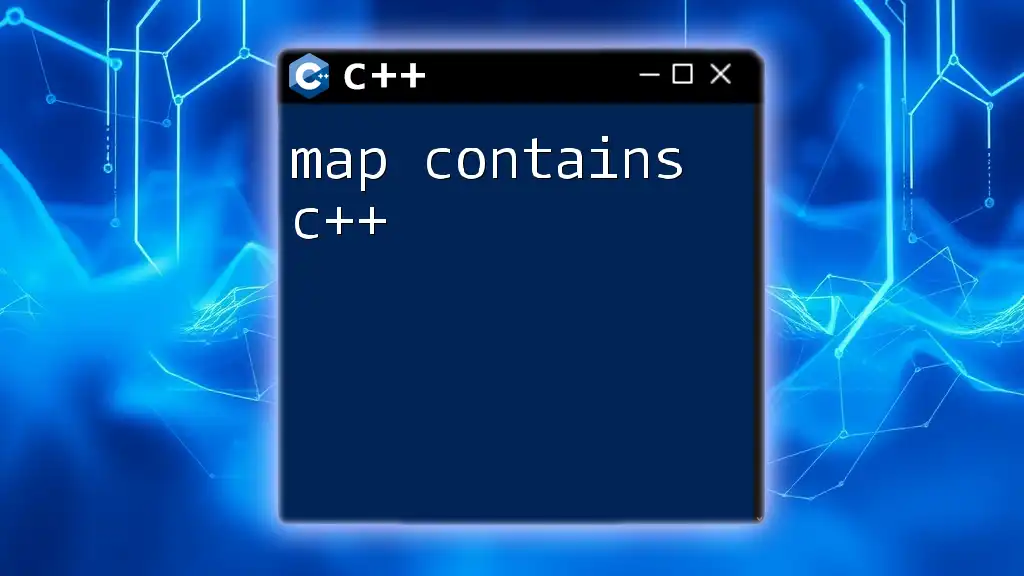
C++ Standard Library Overview
The C++ Standard Library is a powerful and versatile collection of classes and functions that simplify complex programming tasks. It includes various containers such as vectors, lists, sets, and maps, each with unique characteristics and use cases.
- Vectors are dynamic arrays that can grow in size and store elements contiguously in memory.
- Sets store unique elements in a sorted manner, ensuring no duplicates.
- Maps are key-value pairs, where each key is unique and maps to a corresponding value.
Understanding how these containers work allows you to effectively utilize the contains mechanism within your code.
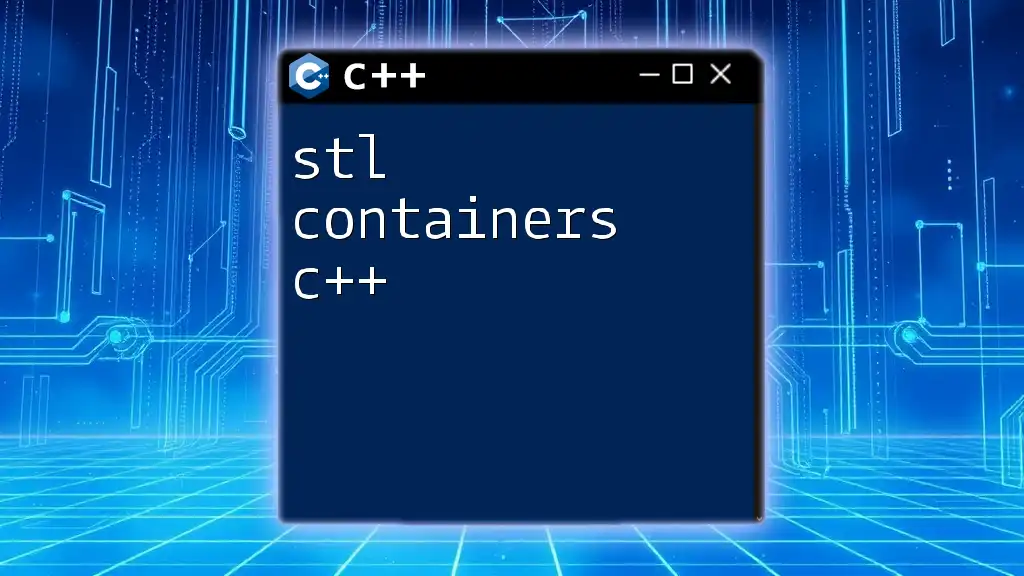
Using Containers to Check "Contains"
When it comes to checking for the presence of an element, different containers provide various methods to achieve this. Let's explore how to use the most common containers for checking if they contain a specific item.
Vectors
Vectors are an excellent choice for collections needing dynamic resizing. To check if a vector contains a specific value, you can use the `std::find` function from the `<algorithm>` header.
Here's how you can implement this:
#include <vector>
#include <iostream>
#include <algorithm>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
int value = 3;
if (std::find(numbers.begin(), numbers.end(), value) != numbers.end()) {
std::cout << "Contains " << value << "\n";
}
return 0;
}
In this code snippet, `std::find` iterates through the vector from the beginning to the end, checking for the specified value. If it finds it, it returns an iterator pointing to the value; otherwise, it returns an iterator pointing to the end of the vector.
Sets
Sets provide a more efficient way of checking for the contains functionality, as they are designed to store unique elements and perform lookups in logarithmic time complexity due to their underlying tree structure.
Here’s how to use a set to check if it contains an element:
#include <set>
#include <iostream>
int main() {
std::set<int> numbers = {1, 2, 3, 4, 5};
int value = 3;
if (numbers.find(value) != numbers.end()) {
std::cout << "Contains " << value << "\n";
}
return 0;
}
In this snippet, the `find` method efficiently checks if the set contains the specified value. If the value exists, it returns an iterator to the element; if not, it returns `end()`.
Maps
For scenarios where you need to check for the presence of a key-value pair, maps are an ideal choice. They store unique keys associated with their corresponding values.
Here’s how to determine if a map contains a specific key:
#include <map>
#include <iostream>
int main() {
std::map<int, std::string> myMap = {{1, "One"}, {2, "Two"}, {3, "Three"}};
int key = 2;
if (myMap.find(key) != myMap.end()) {
std::cout << "Contains key " << key << "\n";
}
return 0;
}
Using the `find` method in this context checks whether the specific key exists in the map. This method is extremely useful for data retrieval and management in key-value pair scenarios.
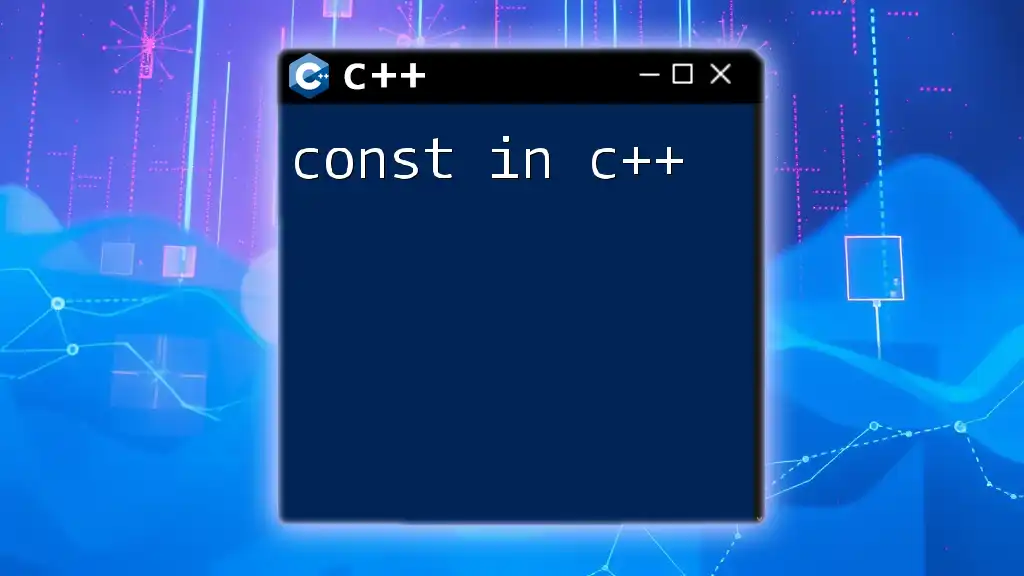
Custom Classes and Contains Methods
Creating a contains method in user-defined classes is a valuable way to implement custom data handling solutions. Below is an example of how you can create a simple container class with a contains method:
#include <vector>
#include <iostream>
#include <algorithm>
class MyContainer {
private:
std::vector<int> items;
public:
void add(int item) { items.push_back(item); }
bool contains(int item) {
return std::find(items.begin(), items.end(), item) != items.end();
}
};
int main() {
MyContainer container;
container.add(1);
container.add(2);
// Checking for item
if (container.contains(2)) {
std::cout << "Container contains 2\n";
}
return 0;
}
In this example, `MyContainer` manages a vector of integers. The contains method employs `std::find` to identify the presence of a given item within its stored elements. This custom approach allows flexibility and control over the data while encapsulating the logic within the class.
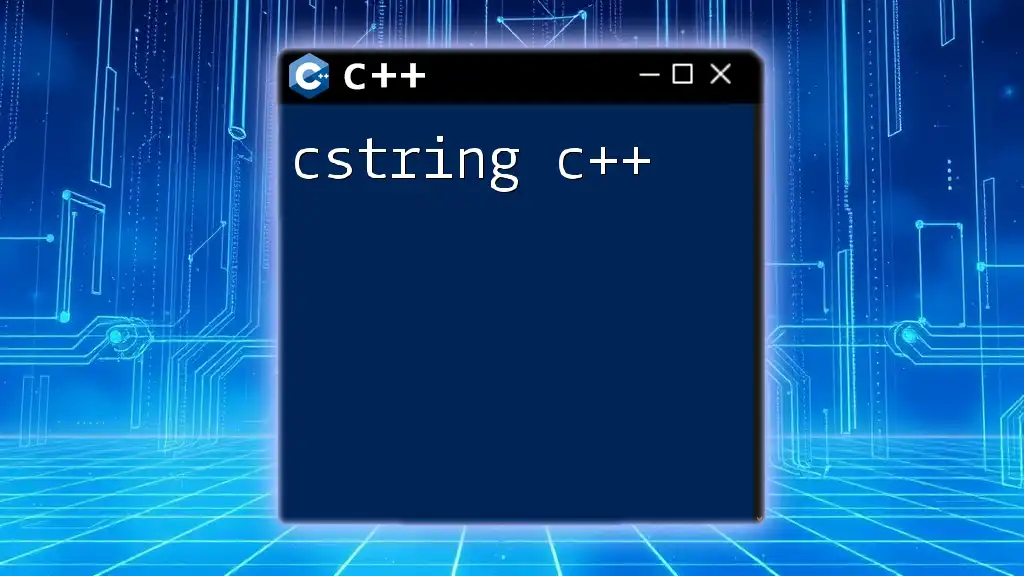
Conclusion
Mastering how to use the contains functionality in C++ is essential for effective data management. Whether you are using built-in containers from the Standard Library or defining your own classes, understanding how to implement checks for membership enhances your programming capabilities. By practicing these concepts in real-world programming scenarios, you can harness the power of C++ effectively and efficiently.
For further resources and to deepen your understanding of C++, we encourage you to explore additional books and online courses that focus on advanced uses of C++ commands and best practices.