STL (Standard Template Library) containers in C++ are essential data structures that manage collections of objects, allowing for efficient storage, retrieval, and manipulation of data.
Here's a simple example demonstrating the use of a vector, one of the most commonly used STL containers:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (int num : numbers) {
std::cout << num << " ";
}
return 0;
}
Introduction to STL Containers
The C++ Standard Library is a powerful set of tools and components that every C++ developer should understand. At its core lies the Standard Template Library (STL), which includes various functionalities, where containers play a vital role.
STL containers are foundational elements that allow developers to manage collections of data efficiently. By using STL containers, programmers can save time and effort, as these built-in structures come with a wealth of functionalities that make data handling seamless.
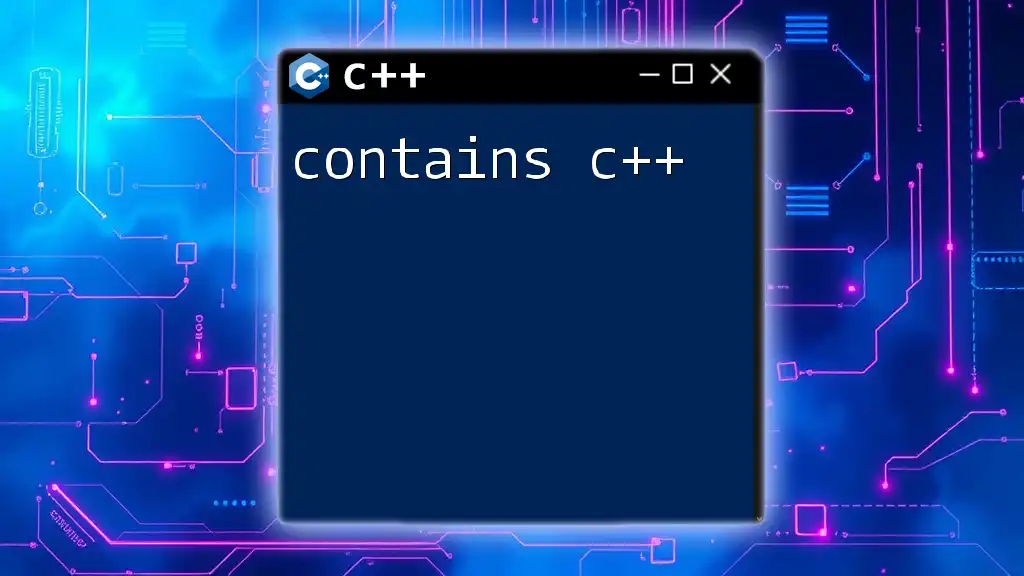
Types of STL Containers
Container Categories
Sequence Containers
Sequence containers are designed to store a collection of data elements in a specific order. They allow access, insertion, and removal of elements in ways that reflect that order.
- Common Sequence Containers:
- `vector`
- `deque`
- `list`
Each of these containers has its unique characteristics that make them suitable for various applications.
Example: Basic operations with `vector`
#include <iostream>
#include <vector>
int main() {
std::vector<int> v = {1, 2, 3, 4, 5}; // Initializing a vector
v.push_back(6); // Adding an element to the end
for (int i : v) std::cout << i << " "; // Iterating through the vector
return 0;
}
In the example above, we create a `vector` that holds integers. The `push_back` function adds a new element to the end of the vector, showcasing the dynamic size and flexibility of STL containers in C++.
Associative Containers
Associative containers provide a mechanism to access elements based on a key. They store elements in a sorted order and use specific keys for such retrieval.
- Common Associative Containers:
- `set`
- `map`
- `multiset`
- `multimap`
Example: Using `map` for key-value storage
#include <iostream>
#include <map>
int main() {
std::map<std::string, int> m; // Initializing a map
m["apple"] = 10; // Inserting key-value pairs
m["banana"] = 20; // Inserting another pair
for (const auto &pair : m) // Iterating through the map
std::cout << pair.first << ": " << pair.second << std::endl;
return 0;
}
In this example, a `map` is used to associate string keys with integer values, providing efficient data retrieval based on the key.
Unordered Associative Containers
Unordered associative containers operate similarly to associative containers but do not sort the elements. They leverage hashing for fast lookups, insertions, and deletions.
- Common Unordered Containers:
- `unordered_set`
- `unordered_map`
Example: Fast lookups using `unordered_map`
#include <iostream>
#include <unordered_map>
int main() {
std::unordered_map<std::string, int> um; // Initializing an unordered map
um["dog"] = 4; // Assigning key "dog" the value 4
um["cat"] = 9; // Assigning key "cat" the value 9
std::cout << "Dog has: " << um["dog"] << " legs" << std::endl; // Accessing value
return 0;
}
This example shows how `unordered_map` allows quick access to a value based on its key, illustrating the versatility and efficiency of STL containers in C++.
Container Adapters
Container adapters provide a restricted interface to one or more underlying containers. They allow specific operations tailored to certain data handling scenarios.
- Common Container Adapters:
- `stack`
- `queue`
- `priority_queue`
Example: Using `stack` for Last-In-First-Out (LIFO) behavior
#include <iostream>
#include <stack>
int main() {
std::stack<int> s; // Initializing a stack
s.push(1); // Adding elements to the stack
s.push(2);
std::cout << "Top element: " << s.top() << std::endl; // Accessing the top element
s.pop(); // Removing the top element
std::cout << "Top element after pop: " << s.top() << std::endl; // Accessing the new top
return 0;
}
The `stack` is an essential container adapter that exemplifies LIFO behavior, making it ideal for applications such as function call management and backtracking algorithms.
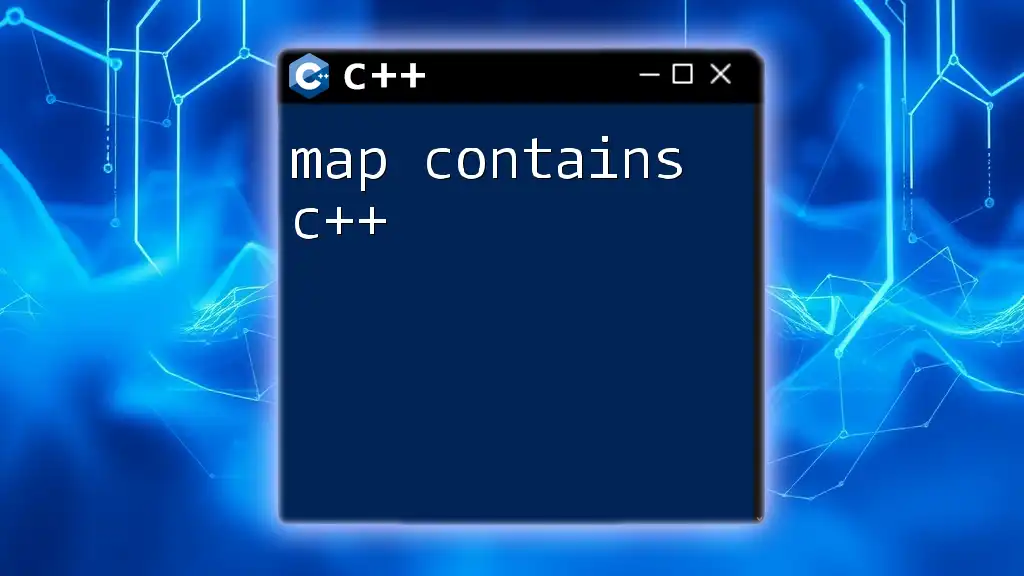
Working with STL Containers
Container Operations
Every STL container in C++ supports a variety of operations. These include inserting elements, erasing them, and searching for specific values. The high-level abstraction provided by these operations allows for flexibility in managing and processing collections of data.
Iterators and STL Containers
Iterators are a crucial part of STL containers that allow users to traverse the elements stored within a container. Depending on the type of container, iterators can be categorized as:
- Random access (e.g., `vector`)
- Bidirectional (e.g., `list`, `deque`)
- Forward (e.g., `forward_list`)
Using iterators is simple yet powerful, as they decouple the algorithm from the container implementation.
Code examples showing how to iterate over containers
#include <iostream>
#include <vector>
int main() {
std::vector<int> v = {10, 20, 30, 40};
for (auto it = v.begin(); it != v.end(); ++it) // Using iterator to access each element
std::cout << *it << " ";
return 0;
}
This code demonstrates how to iterate through a `vector` using an iterator, highlighting the importance of flexibility in container interaction.
Using Algorithms with STL Containers
The STL also includes a wide range of algorithms that can be applied to containers, making data manipulation easier. Common algorithms include:
- `sort`: sorts elements in the container
- `find`: locates an element in the container
- `accumulate`: computes the sum (or other aggregate function) of elements
Example: Sorting a vector
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> v = {4, 1, 3, 2};
std::sort(v.begin(), v.end()); // Sorting the vector in ascending order
for (int i : v) std::cout << i << " "; // Outputting sorted vector
return 0;
}
The use of `std::sort` showcases how STL algorithms can simplify code, improving readability while enhancing performance.
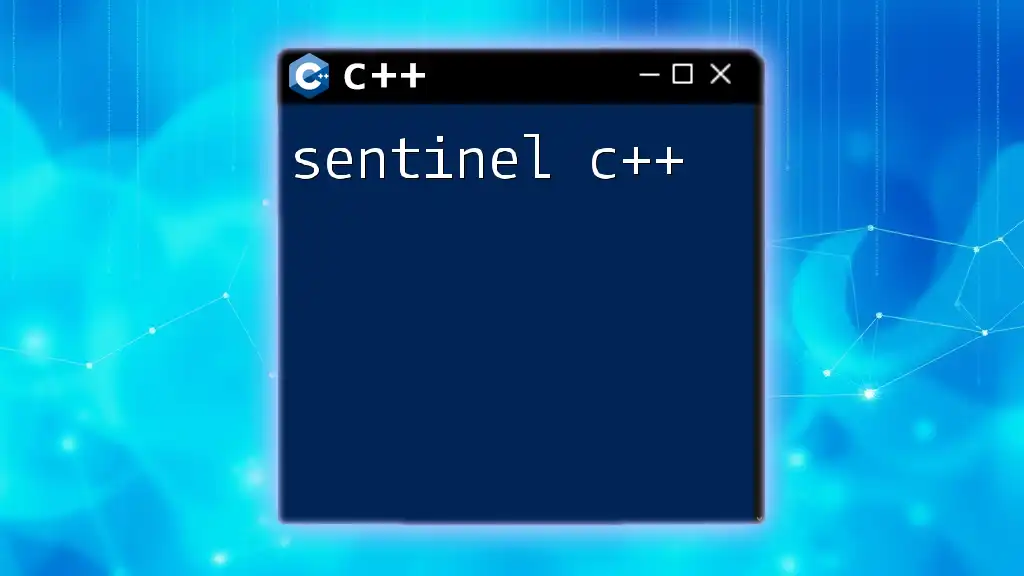
Best Practices for Using STL Containers
Choosing the Right Container for Your Needs
When utilizing STL containers, it is crucial to select the right type of container based on your requirements. Considerations include time complexity and intended operations. For instance:
- Use `vector` when you need contiguous storage and fast access.
- Opt for `list` if frequent insertions and deletions are required without regard to order.
- Prefer `map` or `unordered_map` for associative operations.
Common Pitfalls and Mistakes to Avoid
Developers should be cautious of misusing container types or not fully understanding their behavior, which can lead to inefficient code. A common mistake involves assuming that certain operations are as efficient across all containers, which is not the case. Always consider the lifespan of pointers or references to container elements, especially when dealing with dynamically allocated memory.
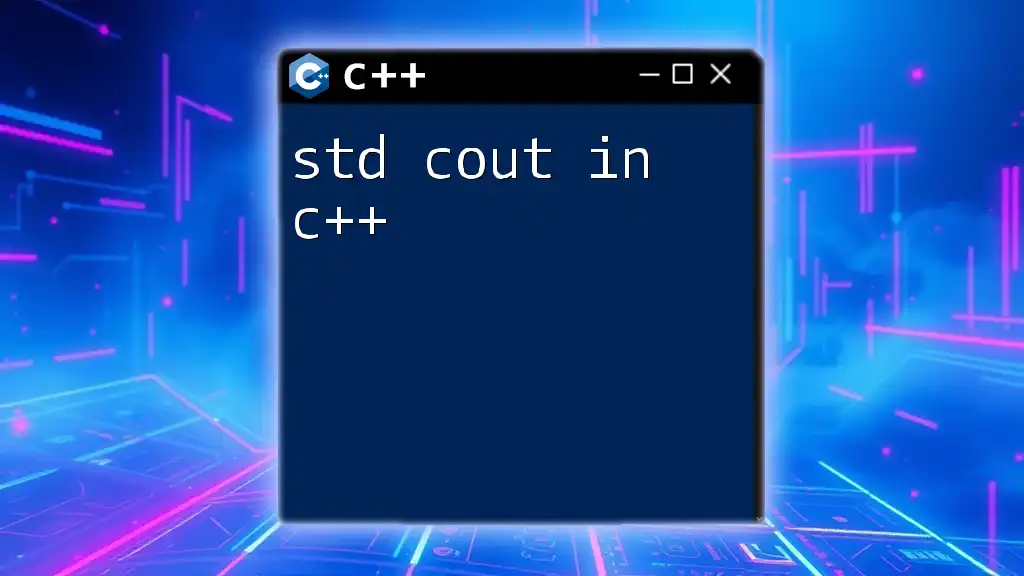
Conclusion
STL containers are an essential aspect of modern C++ programming. Understanding and leveraging their capabilities can significantly enhance your data management skills and overall code efficiency. I encourage you to explore and practice with these containers, as they represent a powerful tool in the C++ Standard Library.
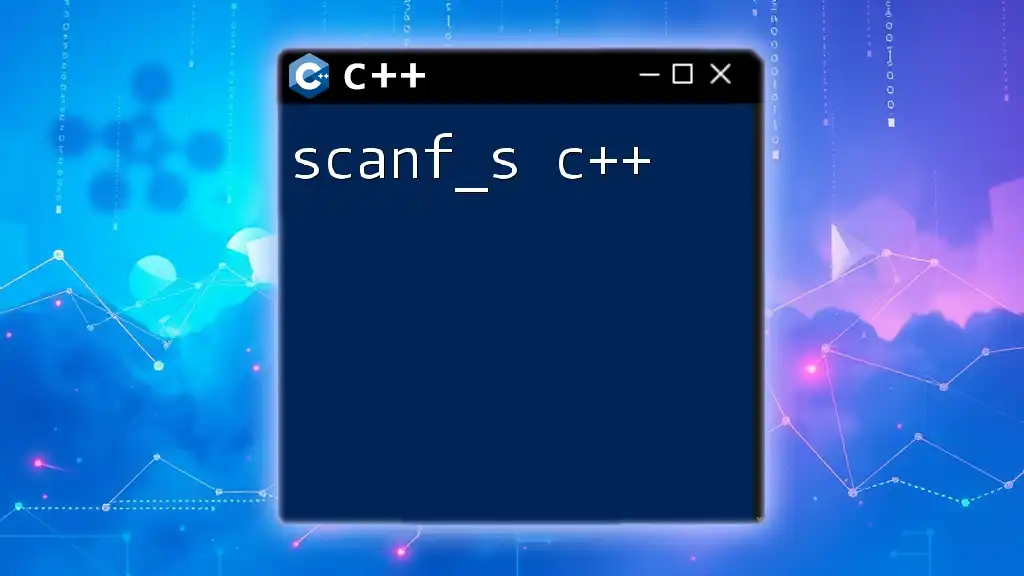
Additional Resources
To deepen your understanding of STL containers in C++, I recommend exploring various books and online resources dedicated to advanced C++ programming. Engaging with communities and forums can also provide valuable insights and practical tips from experienced developers.