In C++, exit codes indicate the status of a program's termination, where a code of 0 typically indicates success and any non-zero code indicates an error or unexpected behavior.
#include <iostream>
#include <cstdlib> // For exit()
int main() {
// Perform some operations
if (/* operation was successful */) {
std::cout << "Operation completed successfully." << std::endl;
return EXIT_SUCCESS; // Exit code 0
} else {
std::cerr << "An error occurred." << std::endl;
return EXIT_FAILURE; // Exit code 1
}
}
What Are Exit Codes?
Definition of Exit Code
An exit code in C++ is a numerical value returned by a program at its termination. This value conveys the success or failure of the program to the operating system. The exit code is critical for indicating if the program executed as intended or if an error occurred during its execution.
Purpose of Exit Codes
The primary purpose of exit codes is to facilitate communication between the executed program and the operating system. Exit codes enable the OS to understand whether the program executed successfully or encountered an issue. This is particularly valuable during automated tasks, such as in scripting or batch processing, where actions depend on previous outcomes. Additionally, exit codes are invaluable for debugging, helping developers diagnose where issues lie in their code.
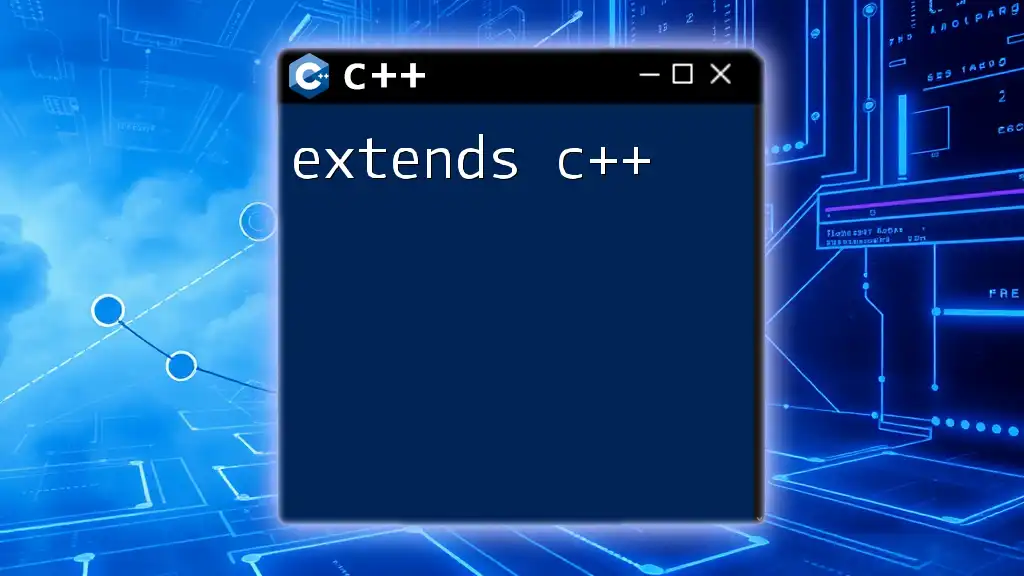
Default Exit Codes in C++
The `main()` Function and Exit Codes
In C++, the entry point of any program is the `main()` function. This function serves a dual purpose: it executes code and returns an exit code. By convention, when the `main()` function completes successfully, it returns 0, indicating success. If it does not return an exit code explicitly, 0 is the implicit return value that the compiler provides.
Common Exit Codes in C++
The most commonly used exit codes in C++ are:
- 0: Indicates that the program has executed successfully without any error.
- Non-zero values: Commonly indicate different types of errors. For example, a program might return `1` for a general error or `-1` for an unexpected condition.
The use of exit codes is not just a formality; it plays a significant role in how other programs, scripts, or the operating system react to the executed program.
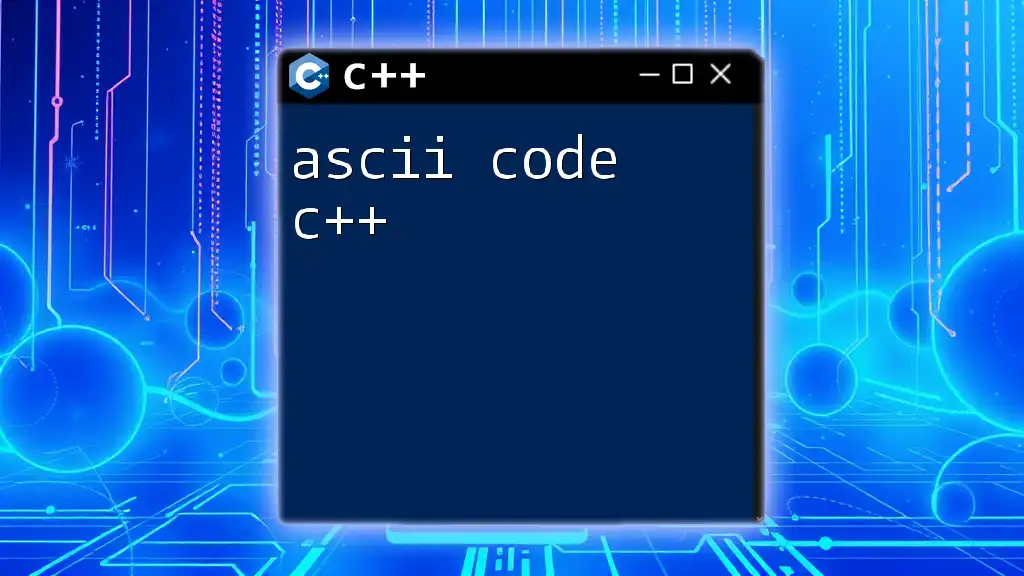
Custom Exit Codes
Creating Custom Exit Codes
In many complex programs, it's essential to define custom exit codes to represent specific errors. This customizability allows developers to implement unique codes for varying failure conditions, facilitating easier debugging and handling of errors.
Example: Custom Exit Codes
Here’s an example that demonstrates how to implement custom exit codes in a C++ program:
#include <iostream>
int main() {
int error_code;
// Simulating a division by zero error
int divisor = 0;
if (divisor == 0) {
std::cerr << "Error: Division by zero" << std::endl;
error_code = 1; // Custom error code for division error
} else {
std::cout << "Result: " << (10 / divisor) << std::endl;
error_code = 0; // Success
}
return error_code;
}
In this example, if the divisor is zero, the program prints an error message and assigns a custom error code of `1`. If the division is successful, it returns `0`. This tailored approach makes it clear what type of error occurred.
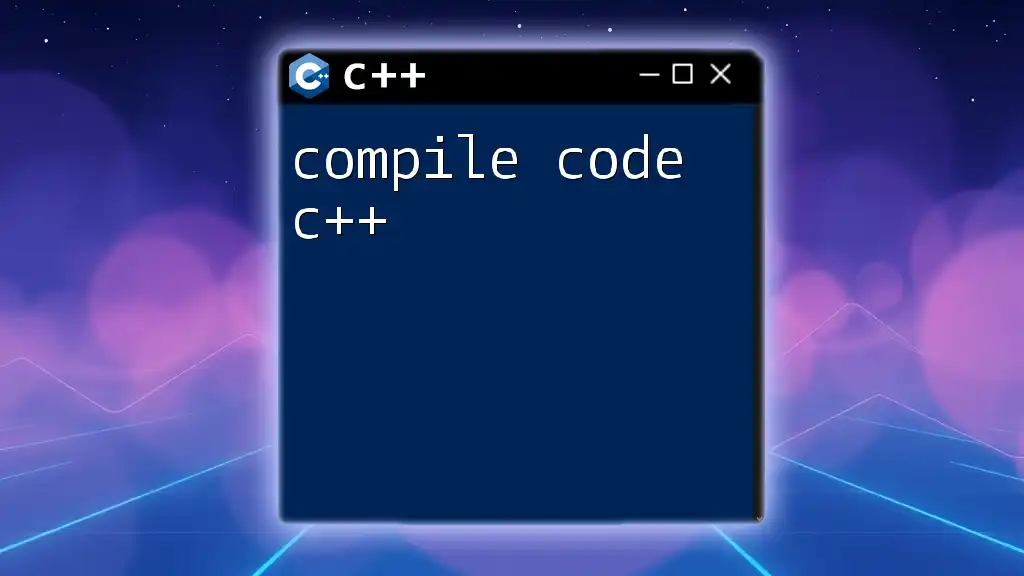
Checking Exit Codes
Using Exit Codes to Determine Program Flow
Checking exit codes is critical, especially in scripts or batch processes where the execution of subsequent commands depends on the success of the previous ones. If a C++ program ends with a non-zero exit code, it usually indicates that something went wrong, which can dictate different flows of execution in a script or application.
Example: Checking Exit Codes in Bash
In a shell script, the exit code of the last executed command can be checked using the `$?` variable. Here’s an example:
./my_cpp_program
if [ $? -ne 0 ]; then
echo "Program terminated with an error!"
else
echo "Program completed successfully."
fi
In this example, the script executes the C++ program and checks the exit code immediately afterward. If the exit code is non-zero, it indicates that the program encountered an issue, prompting an appropriate response.
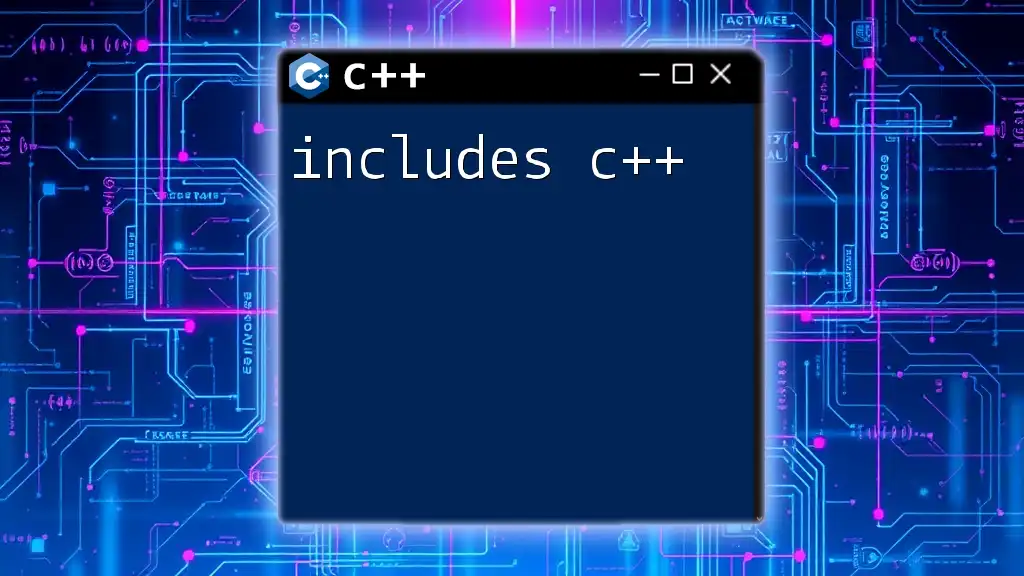
Best Practices for Using Exit Codes
Standardizing Exit Codes
To improve the maintainability and readability of programs, it's essential to establish a standard convention for exit codes. By doing so, developers can ensure consistency across applications, making it easier for other developers (and future you) to understand what different exit codes signify.
Documenting Exit Codes
Proper documentation of exit codes is crucial for both users and maintainers of the code. Providing a clear description of what each code represents can save a lot of headaches down the line. Consider adding this information in more accessible locations like README files, documentation comments within the code, or dedicated documents outlining error handling procedures.
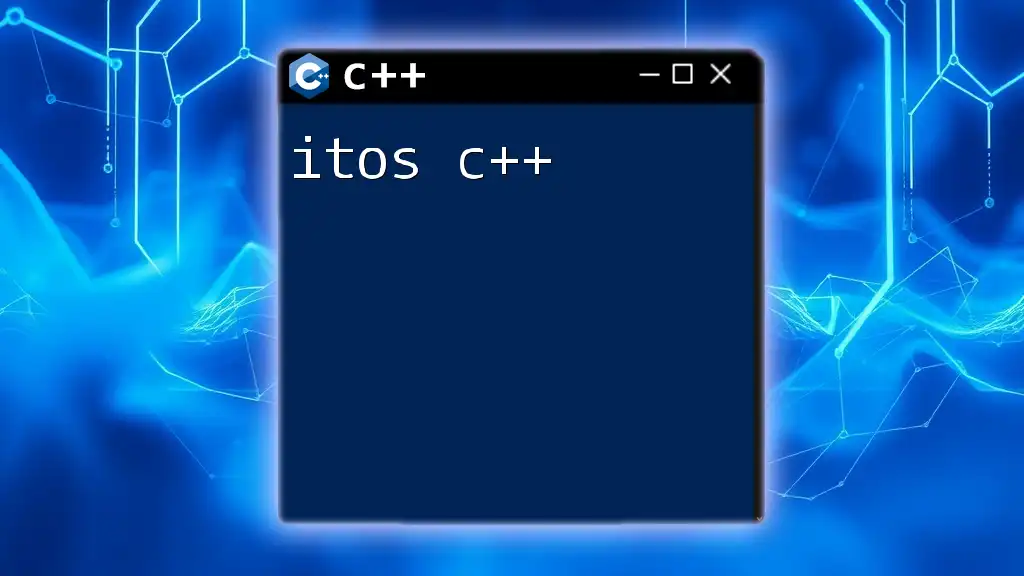
Troubleshooting Common Exit Code Issues
Common Errors and Their Exit Codes
Being aware of common exit codes and their meanings can significantly aid in debugging. For example, many segmentation faults may result in an exit code of `139`, while missing files could return a custom exit code of `2`. Understanding these standard codes allows developers to identify and fix issues more efficiently.
Example:
- Segmentation Fault: Results in exit code `139`, suggesting an access violation error.
- File Not Found: Typically represented by a custom exit code such as `2` to signal that the specified file isn't available.
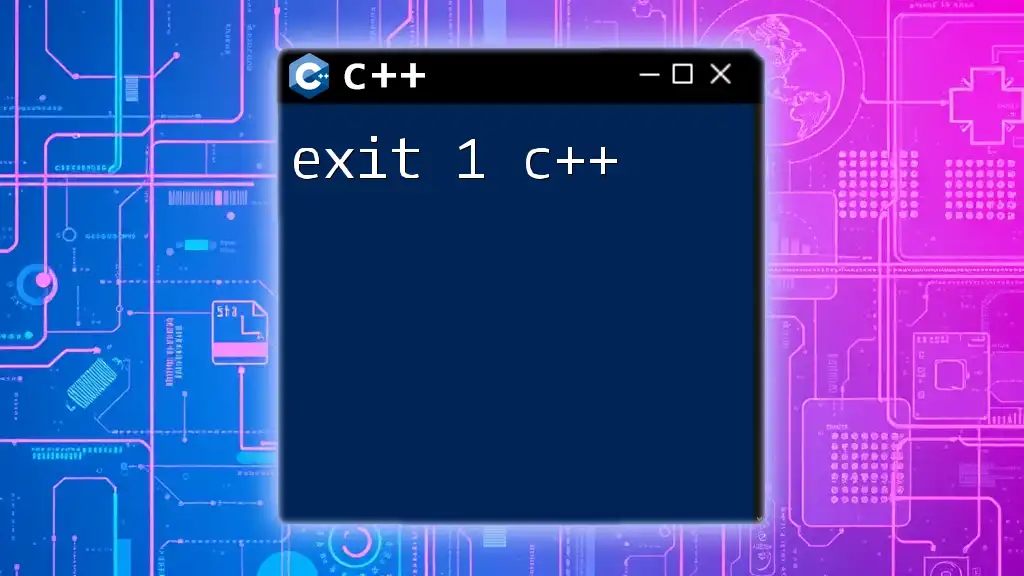
Conclusion
In C++, exit codes are far more than just values returned at the end of a program. They are crucial for indicating the success or failure of a program, assisting in debugging, and serving as a communication mechanism with the operating system. By incorporating custom exit codes, standard practices, and thorough documentation, developers can significantly enhance both the robustness and maintainability of their applications.
Embrace these best practices to foster better error handling and to ensure smoother program execution in your C++ projects!