The `gets` function in C++ is a legacy command used to read a string from standard input until a newline character is encountered, though it is recommended to use safer alternatives due to security concerns.
Here's an example of its usage, though note that it's generally advisable to use `std::getline` instead:
#include <iostream>
using namespace std;
int main() {
char str[100];
cout << "Enter a string: ";
gets(str); // Warning: gets is unsafe and should be avoided.
cout << "You entered: " << str << endl;
return 0;
}
What is `gets`?
The `gets` function is a standard input function in C and C++. It is used to read a line of text from the standard input, typically the keyboard, into a character array. Historically, `gets` was prevalent for simple input handling, providing a straightforward way for programmers to capture user inputs quickly. However, its simplicity comes with significant caveats, particularly regarding security.
How `gets` Works
When you use `gets`, it reads characters from the input until it encounters a newline character (`\n`) or EOF (end of file). This means it captures all characters, including spaces, until the user hits Enter. However, it does so without performing any checks for buffer overflow, leading to potentially dangerous situations where data outside of the intended storage area can be overwritten.
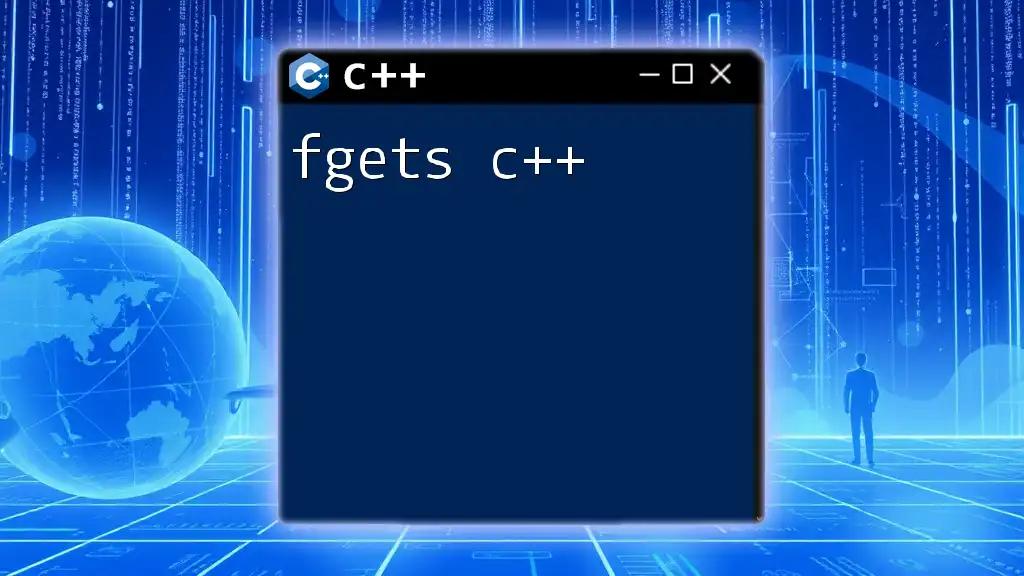
Syntax of `gets`
The syntax for using `gets` is quite simple:
char* gets(char* str);
- Parameter: It takes a single argument, which is a pointer to the character array (or buffer) where the input will be stored.
- Return Value: On success, it returns the pointer to the input string, while on failure, it returns a `NULL` pointer.
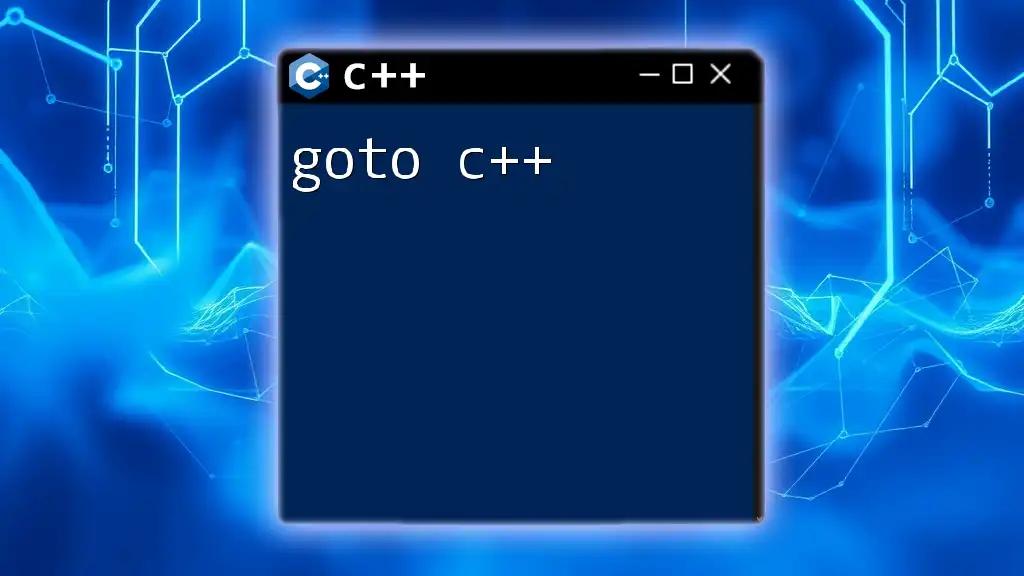
Why was `gets` Deprecated?
Security Issues
The primary reason `gets` has been deprecated in the C11 and C++ standards is due to its inherent security risks. Because `gets` does not check the size of the buffer, if a user inputs more characters than the buffer can hold, it leads to a buffer overflow. This can be exploited by attackers to overwrite memory and inject malicious code, leading to vulnerabilities in applications.
Alternatives to `gets`
Given these risks, safer alternatives were introduced. Two notable substitutes are `fgets` and `getline`, which provide more robust handling of input strings.
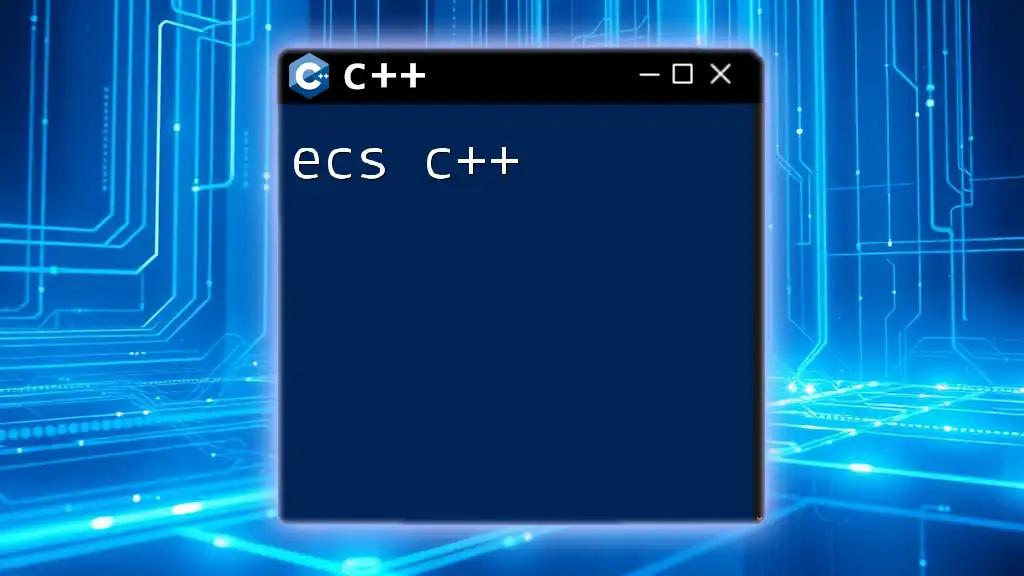
Practical Examples using `gets`
Example Code Snippet
#include <iostream>
#include <cstdio>
int main() {
char str[100];
std::cout << "Enter a string: ";
gets(str); // Unsafe usage example
std::cout << "You entered: " << str << std::endl;
return 0;
}
In the code snippet above, a buffer of 100 characters is declared to hold the user’s input. While it may seem harmless at first glance, this usage of `gets` poses a significant risk. If a user enters a string longer than 99 characters (the last character being reserved for the null terminator), it will overflow the buffer, potentially causing instability in the program or exposing it to security vulnerabilities.
Safe Alternatives in Practice
Using `fgets`
To mitigate the risks associated with `gets`, you can use `fgets`, which limits the number of characters read and checks the buffer size:
#include <iostream>
#include <cstdio>
int main() {
char str[100];
std::cout << "Enter a string: ";
fgets(str, sizeof(str), stdin);
std::cout << "You entered: " << str << std::endl;
return 0;
}
In this example, `fgets` reads at most `sizeof(str) - 1` characters including the null terminator, ensuring that you do not overflow the buffer. If you enter more than what can be stored, it will simply stop reading further input, thus safeguarding your program.
Using `getline`
Another alternative is the C++ way, which involves the `getline` function, a part of the STL (Standard Template Library):
#include <iostream>
#include <string>
int main() {
std::string str;
std::cout << "Enter a string: ";
std::getline(std::cin, str);
std::cout << "You entered: " << str << std::endl;
return 0;
}
Using `getline` is not only safer but also more convenient, as it automatically manages the memory for the input string and does not require you to worry about buffer sizes. It reads an entire line, including spaces, and handles dynamic allocations, which makes it an excellent choice for more extensive input handling.

Common Mistakes with `gets`
Misunderstanding Buffer Sizes
One of the most common mistakes when using `gets` is underestimating the input size and buffer allocation. Programmers may declare a buffer size only to run into issues when a user inputs a much longer string. This not only leads to crashes but can impose serious security vulnerabilities as well.
Using `gets` with Non-Character Arrays
Another common error is attempting to use `gets` on non-character arrays. This can lead to unexpected behavior and must be avoided for robust applications. The expectation that `gets` can handle various data types is misleading; it is strictly meant for character arrays.
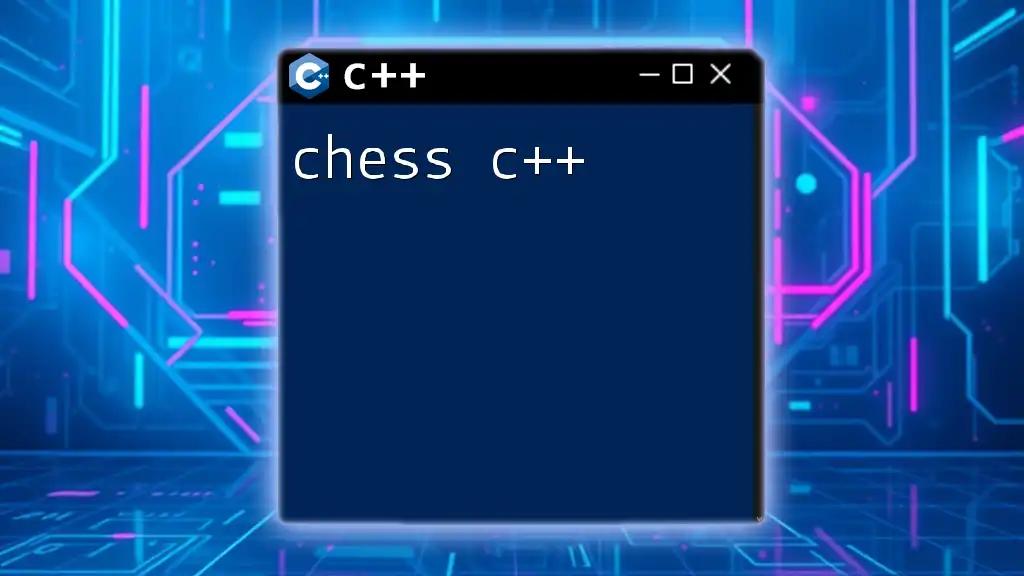
Conclusion
Understanding `gets` in C++ is crucial for any programmer, but it's imperative to recognize its limitations and the associated risks. While it may still be found in legacy code, programmers are strongly discouraged from using `gets` due to its security vulnerabilities. Instead, adopting safer alternatives like `fgets` and `getline` is essential for ensuring the robustness and security of applications.
In coding, being aware of safe practices is paramount. C++ offers many features to help manage input properly; it is a programmer's responsibility to incorporate these features into their work. As programming continues to evolve, embracing safety and security in our code becomes ever more crucial.