The `getopts` utility in C++ is used for parsing command-line options in a concise manner, allowing developers to handle user input efficiently.
#include <iostream>
#include <getopt.h>
int main(int argc, char *argv[]) {
int opt;
while ((opt = getopt(argc, argv, "a:b:")) != -1) {
switch (opt) {
case 'a':
std::cout << "Option a: " << optarg << std::endl;
break;
case 'b':
std::cout << "Option b: " << optarg << std::endl;
break;
default:
std::cerr << "Usage: " << argv[0] << " [-a value] [-b value]" << std::endl;
return EXIT_FAILURE;
}
}
return EXIT_SUCCESS;
}
Understanding Command-Line Arguments
Command-line arguments play a crucial role in many applications, allowing users to pass information directly to programs upon execution. When utilizing C++, command-line arguments are typically processed through the `argc` and `argv` parameters in the `main()` function.
- `argc`: Represents the number of command-line arguments passed, including the program's name.
- `argv`: An array of C-style strings representing the individual arguments.
For example, executing a program like `./my_program arg1 arg2` would yield an `argc` of 3, with `argv[0]` containing `./my_program`, `argv[1]` containing `arg1`, and `argv[2]` containing `arg2`.
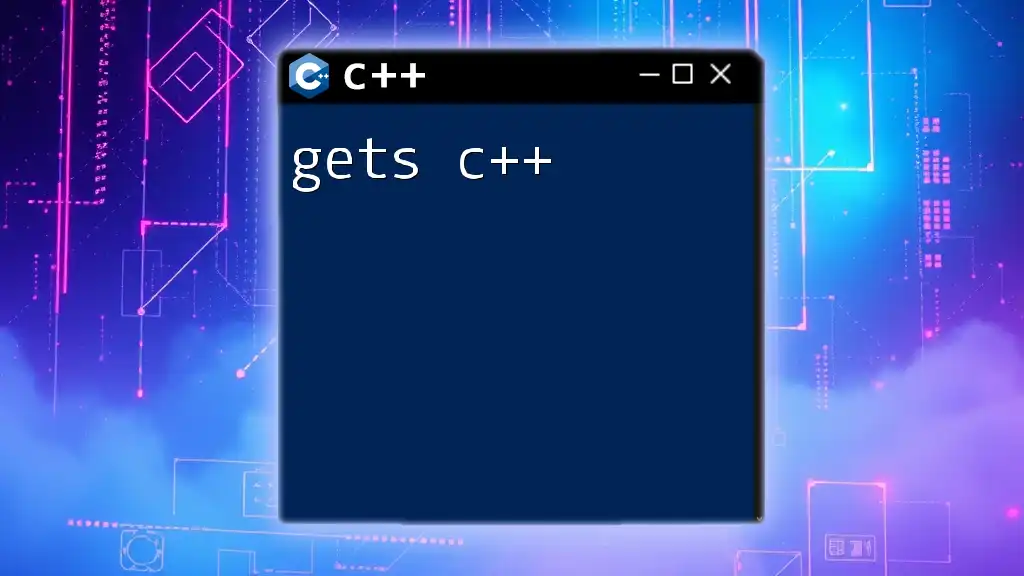
The Getopts Function Explained
What is Getopts?
The `getopt` function is a standard C library function used to parse command-line options. In the context of C++, it provides a straightforward way to handle both short (e.g., `-a`) and long options (e.g., `--alpha`) effectively. Using `getopt` can simplify the complexity of parsing command-line input.
Syntax of Getopts
The basic syntax of the `getopt` function follows this structure:
int getopt(int argc, char * const argv[], const char *optstring);
- `argc` and `argv` are provided from the main function.
- `optstring` is a string that specifies the valid options. Each character represents an option; if a character is followed by a colon, it requires an argument.
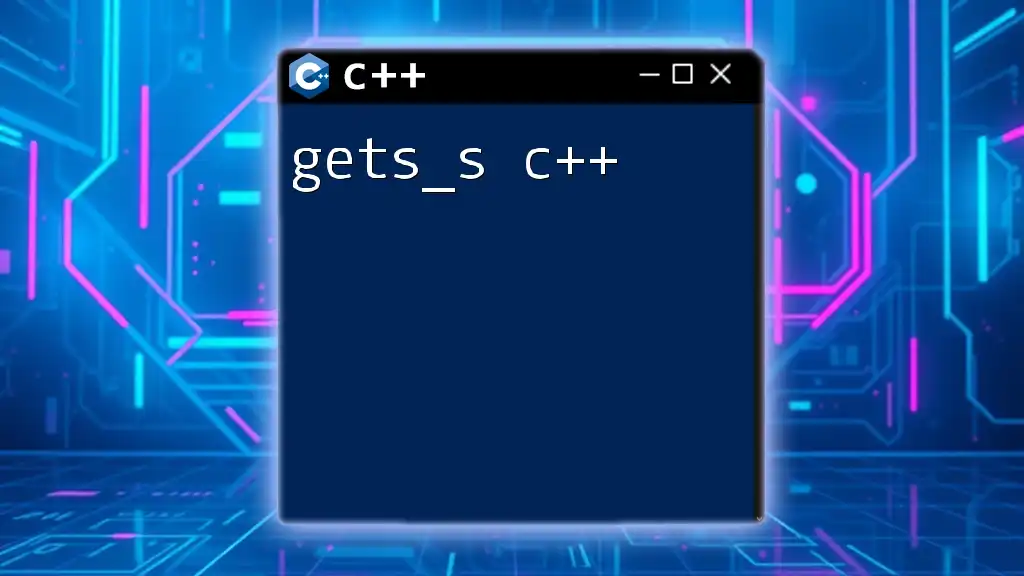
Using Getopts in C++
Setting Up Your Development Environment
To utilize `getopt` in C++, include the `unistd.h` header at the beginning of your source file:
#include <iostream>
#include <unistd.h>
This library provides the `getopt` functionality, making it available for your command-line argument parsing.
Writing a Basic Program Using Getopts
Creating a simple program using `getopt` involves a few steps. Below is an example that demonstrates the fundamental use of `getopt` for parsing command-line options.
#include <iostream>
#include <unistd.h>
int main(int argc, char *argv[]) {
int opt;
while ((opt = getopt(argc, argv, "a:b:c")) != -1) {
switch (opt) {
case 'a':
std::cout << "Option a: " << optarg << std::endl;
break;
case 'b':
std::cout << "Option b: " << optarg << std::endl;
break;
case 'c':
std::cout << "Option c is set." << std::endl;
break;
default:
std::cerr << "Usage: " << argv[0] << " -a <arg> -b <arg> -c" << std::endl;
return EXIT_FAILURE;
}
}
return EXIT_SUCCESS;
}
In this example, the program can receive options `-a`, `-b`, and `-c`. If options `-a` or `-b` are called, the program will print the corresponding arguments. For `-c`, it merely indicates that the option is set.
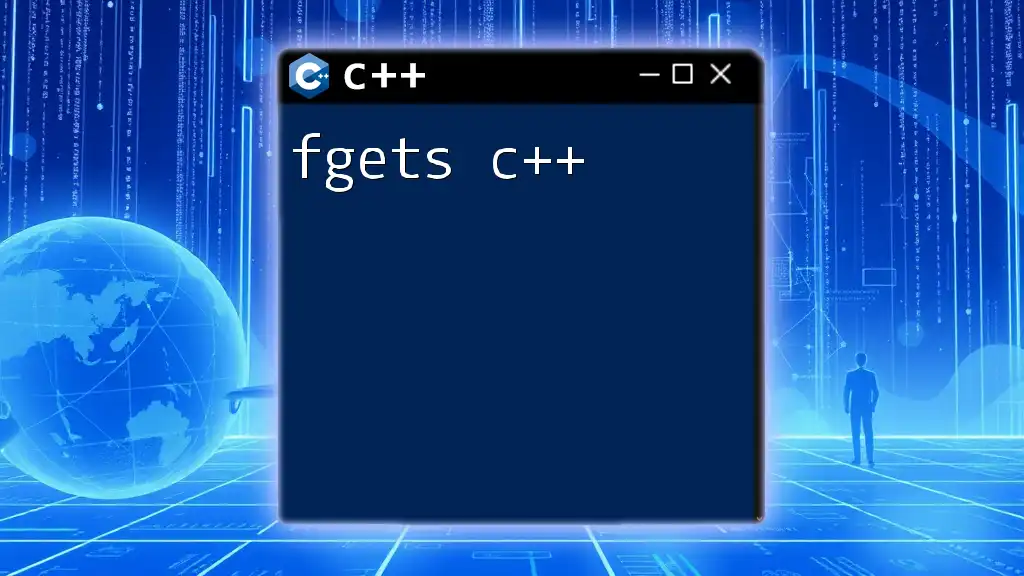
Advanced Getopts Usage
Handling Long Options
In addition to short options, `getopt` can also handle long options using the `getopt_long` function. This function allows for a more user-friendly way to specify options.
To implement long options, you can use the following syntax:
#include <iostream>
#include <getopt.h>
int main(int argc, char *argv[]) {
int opt;
struct option long_options[] = {
{"alpha", required_argument, 0, 'a'},
{"beta", required_argument, 0, 'b'},
{"charlie", no_argument, 0, 'c'},
{0, 0, 0, 0}
};
while ((opt = getopt_long(argc, argv, "a:b:c", long_options, NULL)) != -1) {
switch (opt) {
case 'a':
std::cout << "Long option --alpha: " << optarg << std::endl;
break;
case 'b':
std::cout << "Long option --beta: " << optarg << std::endl;
break;
case 'c':
std::cout << "Long option --charlie is set." << std::endl;
break;
default:
std::cerr << "Usage: " << argv[0] << " --alpha <arg> --beta <arg> --charlie" << std::endl;
return EXIT_FAILURE;
}
}
return EXIT_SUCCESS;
}
This use of `getopt_long` clearly demonstrates how to handle both short and long command-line options effectively.
Error Handling in Getopts
When working with `getopt`, handling errors gracefully is essential. Common pitfalls include missing required arguments or using invalid options. It’s vital to check the output of `getopt` and respond with an appropriate message when an error occurs.
Best practices include:
- Utilizing meaningful error messages to guide users.
- Returning specific exit codes (e.g., `EXIT_FAILURE`) to indicate failure.
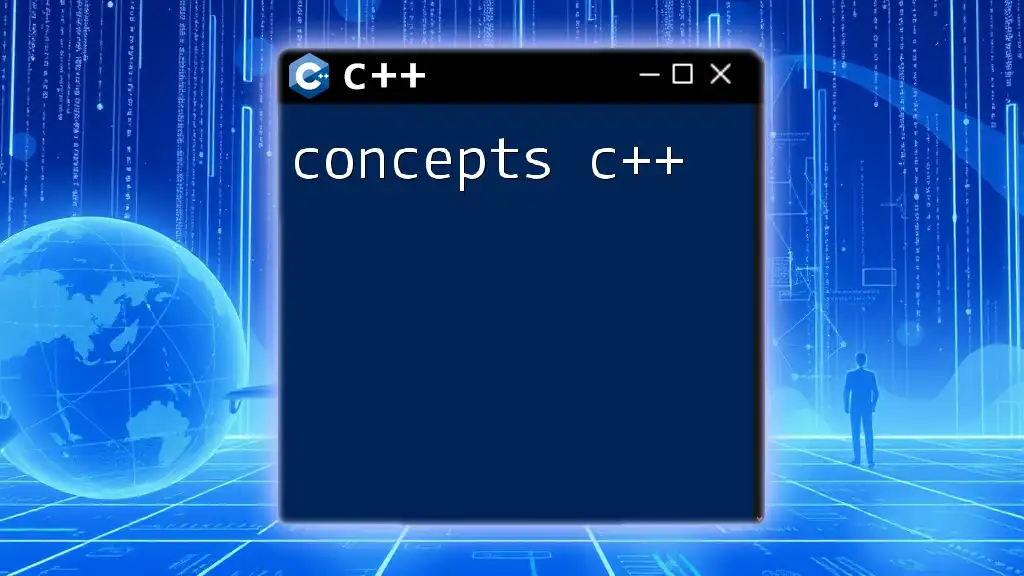
Examples and Use Cases
Practical Applications of Getopts
The versatility of `getopts c++` makes it suitable for various applications, including:
- Command-line utilities that require configuration parameters.
- Automated scripts needing options to customize behavior.
- User-focused tools where optional settings improve usability.
For instance, consider a data processing tool where users can specify input files and output formats using command-line options.
What to Do When Getopts Doesn’t Fit?
While `getopt` is beneficial, it may not be the best fit for every scenario. In cases where more complex parsing is necessary, alternative libraries, such as:
- Boost.Program_options
- cxxopts
- CLI11
These libraries offer enhanced flexibility at the cost of slightly increased complexity and setup.
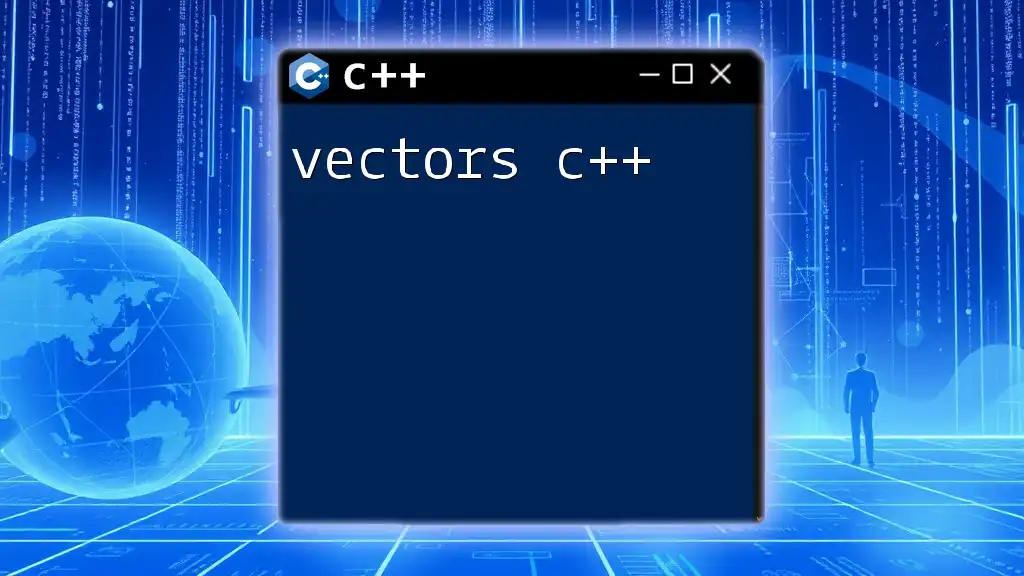
Best Practices for Using Getopts in C++
Code Structure and Organization
To maintain clarity and effectiveness in your code:
- Group related options logically for better organization.
- Use enums or constants to define valid options.
Common Mistakes to Avoid
Some mistakes that programmers frequently encounter with `getopts` include:
- Forgetting to check required arguments.
- Misconfiguring option strings leading to unexpected results.
Be vigilant about testing different combinations of command-line inputs to ensure robustness.
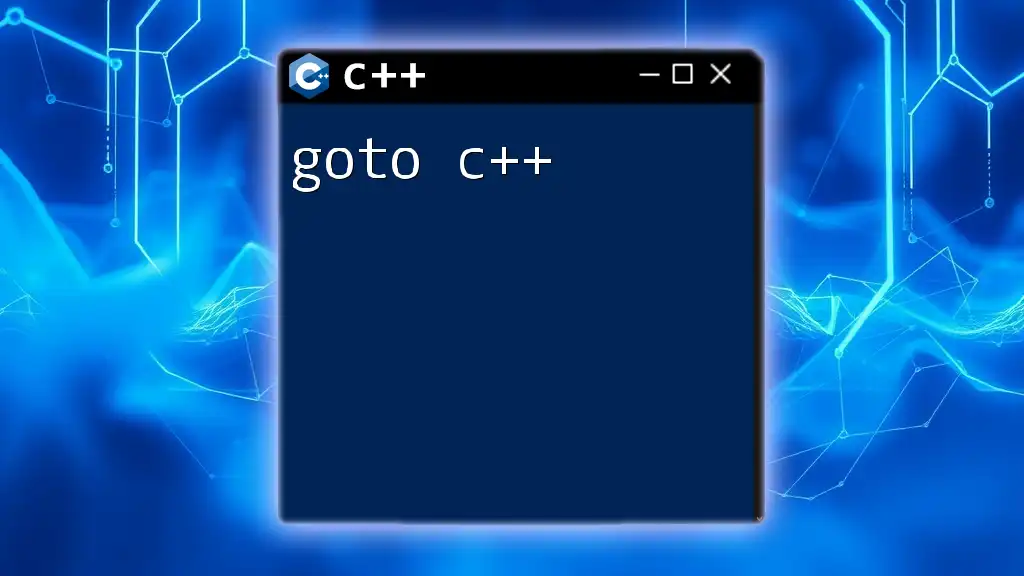
Conclusion
By now, you should have a solid understanding of how to implement getopts c++ to parse command-line arguments effectively. With its straightforward syntax and flexibility, `getopt` is an invaluable tool for C++ developers. Apply the techniques discussed here, and feel free to experiment with more complex scenarios as you grow more comfortable with command-line argument parsing. Don't hesitate to share your experiences or inquire further on the topic!
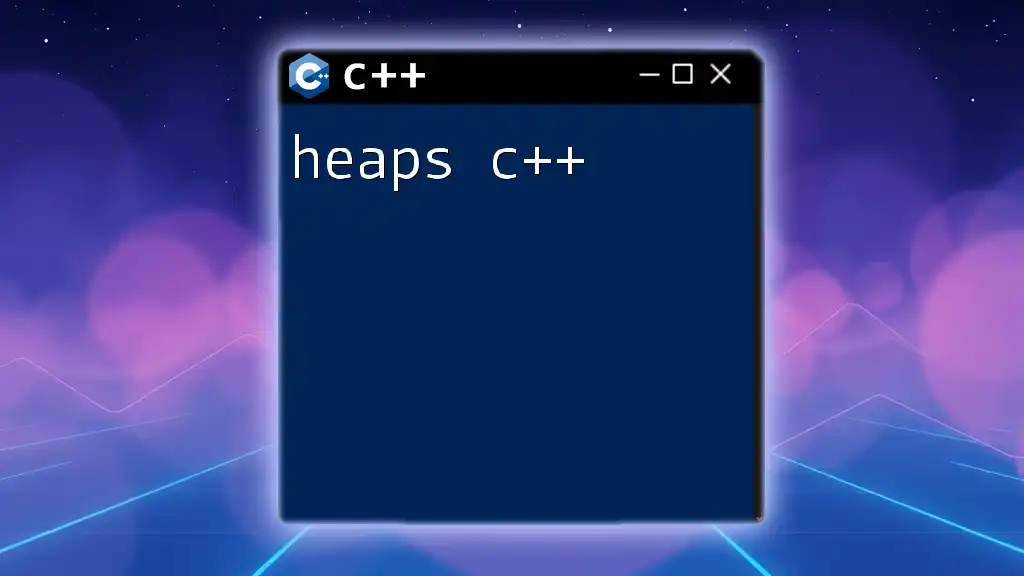
Additional Resources
For further exploration of command-line parsing in C++, consider checking out:
- The official C++ documentation for `getopt`.
- Relevant tutorials and articles highlighting advanced uses and best practices.
- Community forums where developers discuss common issues and solutions.