Go is designed for simplicity and concurrency, making it ideal for modern web services, while C++ offers fine-grained control over system resources and performance, catering to applications that require high efficiency.
Here's a simple comparison of a "Hello, World!" program in both languages:
// Go code
package main
import "fmt"
func main() {
fmt.Println("Hello, World!")
}
// C++ code
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
Language Paradigms
Go Programming Language
Go is designed with a focus on simplicity and efficiency. Primarily procedural, Go emphasizes straightforward programming constructs that make it easier for developers to write concurrent programs. This is largely achieved through goroutines, which allow for lightweight, concurrent execution of functions. Goroutines are scheduled by the Go runtime, enabling developers to manage multiple tasks simultaneously without significant overhead.
C++ Programming Language
In contrast, C++ is a multi-paradigm language that incorporates features from procedural, object-oriented, and even functional programming. This flexibility makes it a powerful choice for a range of applications but also adds complexity to its syntax and paradigms of use. C++ supports various programming styles, allowing developers to leverage features like classes, inheritance, and operator overloading to design sophisticated systems.

Syntax Comparison
Simplicity vs Complexity
Go’s Simplicity
One of the attractive features of Go is its clean and succinct syntax. For example, defining a function in Go is straightforward:
func add(a int, b int) int {
return a + b
}
This simplicity reduces the learning curve for newcomers and enhances readability for experienced developers.
C++’s Rich Syntax
On the other hand, C++ features a more complex syntax that can be daunting for beginners. Although this complexity provides more power and flexibility, it can also lead to potentially convoluted code. Here’s how to declare a function in C++:
int add(int a, int b) {
return a + b;
}
While succinct, the rich syntax of C++ offers a vast array of tools that advanced users can leverage to craft intricate solutions.
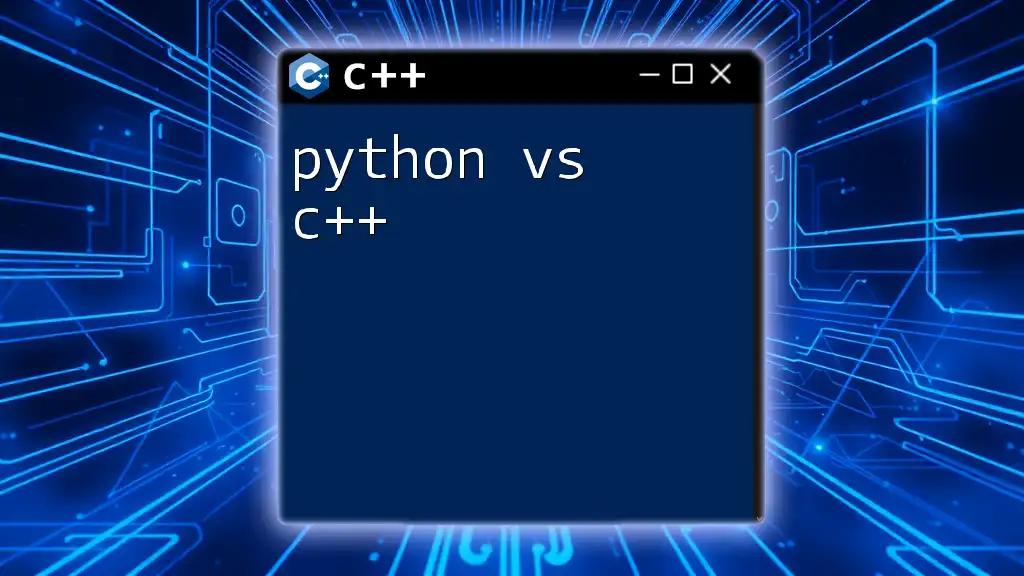
Memory Management
Automatic vs Manual
Go's Garbage Collection
Memory management in Go is handled through garbage collection, which automatically frees up memory that is no longer in use. This feature significantly simplifies the development process, as developers do not need to manually deallocate memory, reducing the risk of memory leaks. However, garbage collection can introduce latency during the execution of programs, especially for applications requiring real-time performance.
C++'s Manual Memory Management
In contrast, C++ requires developers to manage memory manually, offering powerful capabilities through pointers and dynamic memory allocation. While this gives developers fine control, it also places the burden of memory management on them. Below is an example of how to allocate and deallocate memory dynamically in C++:
int* array = new int[10];
delete[] array;
This manual approach can lead to errors, such as memory leaks or dangling pointers, if not handled with care.
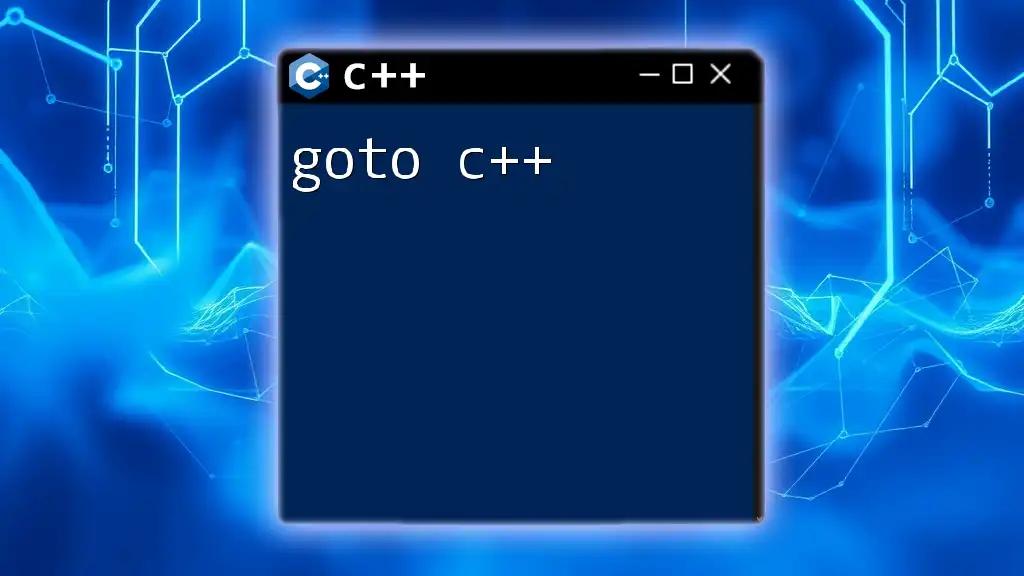
Performance
Benchmarking Go and C++
Speed and Efficiency
In performance-critical applications, C++ often outperforms Go due to its ability to produce highly optimized code. Furthermore, C++ allows for low-level memory management, which can be an advantage in scenarios where performance is paramount, such as in game engines or systems programming.
However, Go excels in scenarios requiring concurrency, such as handling multiple connections for a web server. The built-in goroutines allow developers to efficiently manage concurrent tasks without a significant performance hit.
Example: Performance Comparison
To illustrate performance, consider a simple sorting algorithm implemented in both languages. In Go, sorting a slice is as straightforward as:
sort.Ints(slice)
In C++, the Standard Template Library offers a similar function:
std::sort(slice.begin(), slice.end());
Both languages provide efficient sorting, but the underlying implementations and performance may vary depending on the maturity of the standard library and the characteristics of the data being sorted.
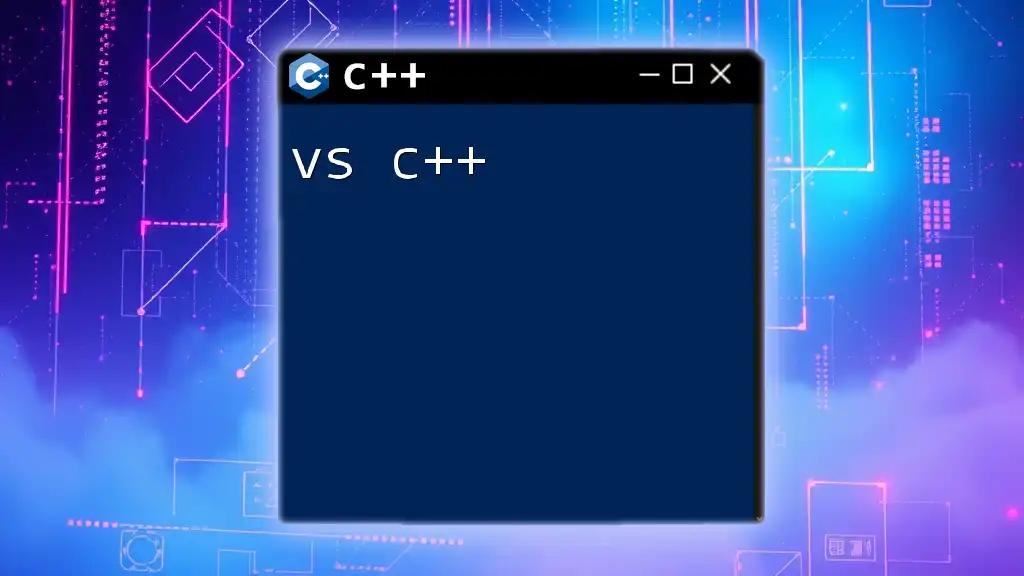
Standard Library and Ecosystem
Go's Standard Library
Rich Yet Lightweight
Go's standard library is expansive yet maintains a lightweight, unobtrusive design. It provides a rich collection of packages that cater to various programming needs, including networking, cryptography, and web development. For instance, creating a simple HTTP server in Go can be accomplished with minimal effort:
http.HandleFunc("/", handler)
http.ListenAndServe(":8080", nil)
This ease of use significantly accelerates development speeds for backend services and microservices.
C++'s Standard Library
Comprehensive and Powerful
Conversely, C++ features an extensive standard library known as the Standard Template Library (STL). The STL includes a variety of data structures and algorithms that provide developers with robust tools for complex problem-solving. Here’s an example of how to utilize vectors in C++:
std::vector<int> v{1, 2, 3};
The STL is incredibly powerful, but its complexity can sometimes be overwhelming for new developers.
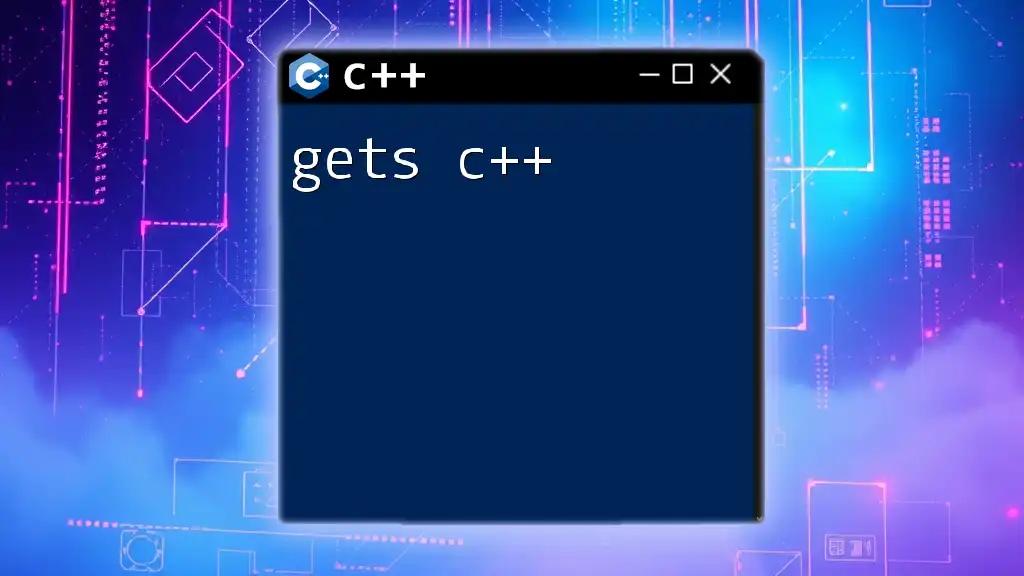
Concurrency
Concurrency in Go
Goroutines and Channels
Go’s approach to concurrency is designed with simplicity in mind. Goroutines are lightweight threads that can be easily spawned, and channels allow for seamless communication between them. The ease of handling concurrency is one of Go’s greatest strengths:
c := make(chan string)
go func() { c <- "Hello, Go!" }()
fmt.Println(<-c)
This code snippet demonstrates how goroutines can work together with channels to maintain order and communication, making concurrent programming more accessible.
Concurrency in C++
Threads and Future
C++ supports concurrency through its standard library features like `std::thread`. This capability allows developers to create multiple threads for parallel execution of tasks, albeit with more complexity and manual control. Here is an example of creating a thread in C++:
std::thread t([] { std::cout << "Hello, C++" << std::endl; });
t.join();
While powerful, managing threads in C++ often requires careful consideration of thread safety and resource handling, adding complexity to the development process.
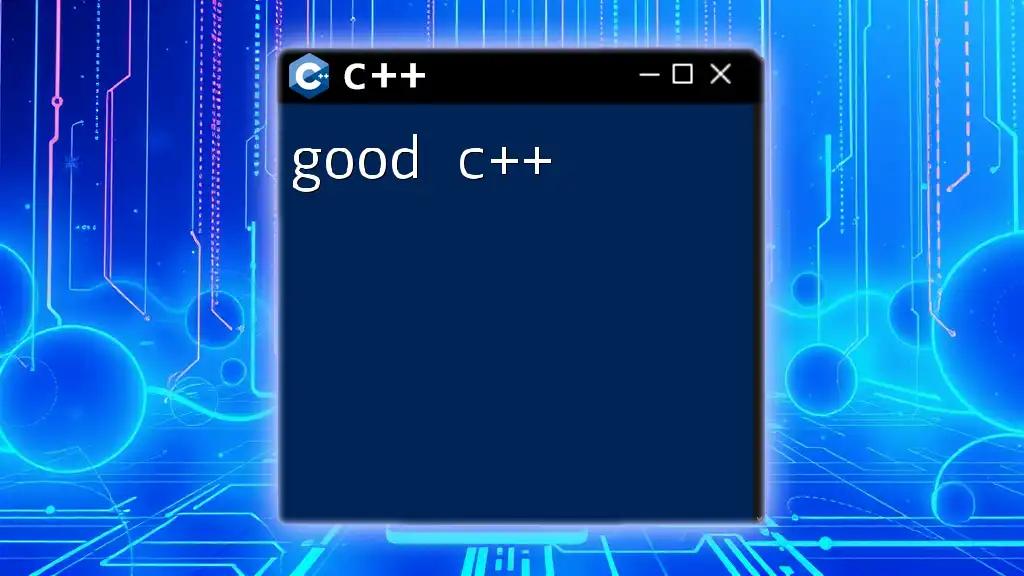
Community and Support
Growing Go Community
Resources and Documentation
Go is rapidly gaining popularity, and its community is vibrant and supportive. Numerous resources—including documentation sites, forums, and tutorials—exist for developers to learn and share knowledge about Go.
Established C++ Community
Maturity and Legacy
C++ has been around for decades, and its community comes with a wealth of established resources and expertise. Various forums, online courses, and extensive documentation provide support for developers across all skill levels.
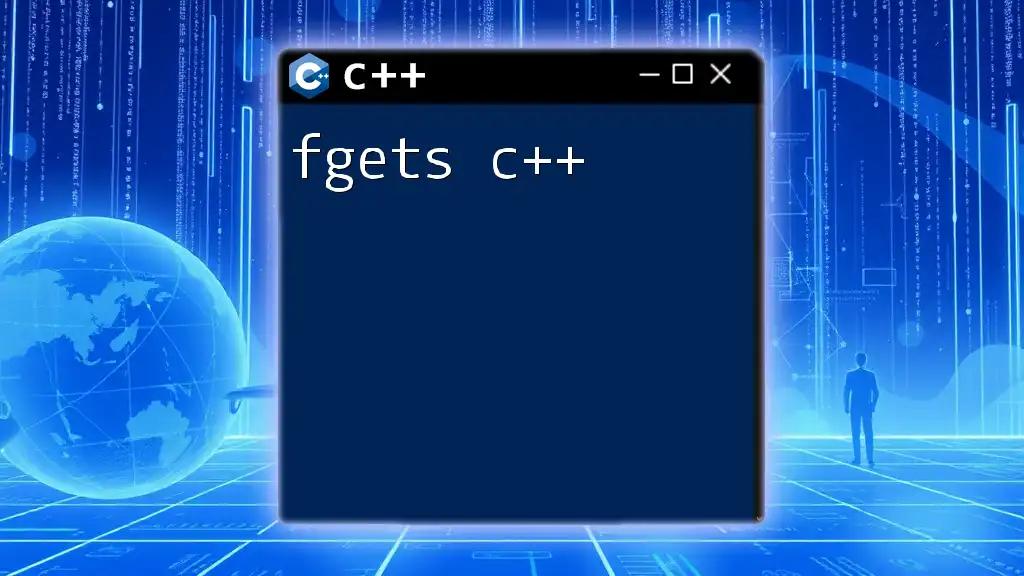
Use Cases and Industry Applications
When to Use Go
Ideal Scenarios
Go is particularly well-suited for backend services, microservices, and cloud applications due to its efficiency in handling concurrency and simplicity. Companies like Google and Dropbox leverage Go for its performance benefits and ease of use in network programming.
When to Use C++
Ideal Scenarios
C++ shines in areas like game development, system programming, and performance-critical applications. Organizations requiring fine control over system resources and optimized performance often turn to C++. Real-time systems, gaming engines, and applications that require interfacing with hardware are best developed using C++.
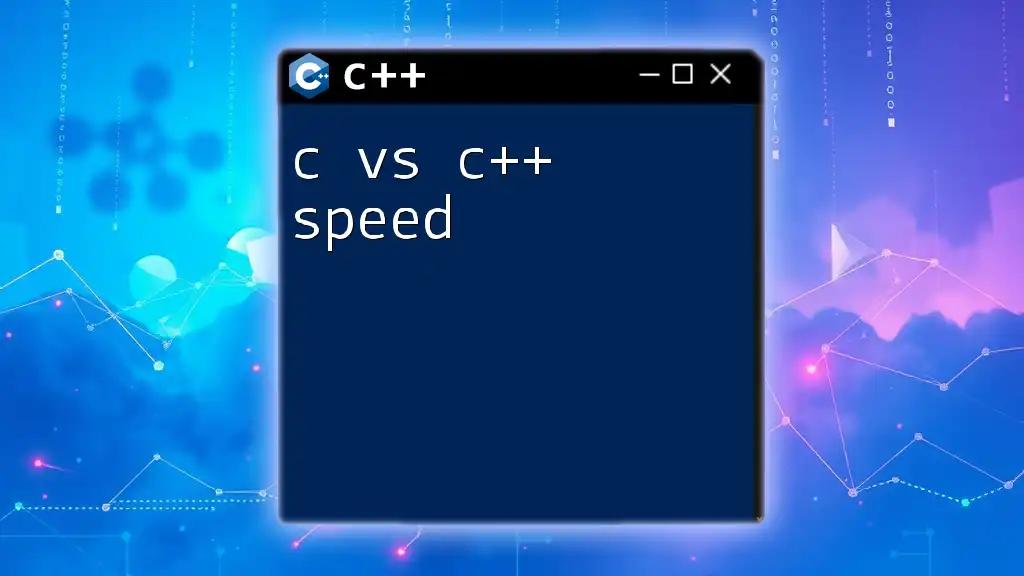
Conclusion
In conclusion, both Go and C++ serve distinct purposes in the programming landscape. While Go excels in concurrency and simplicity, C++ offers depth, flexibility, and performance. The choice between these two languages ultimately depends on the specific needs of a project, the skill set of the development team, and the desired outcomes. By carefully considering these factors, developers can select the language that best fits their goals in this "go vs c++" landscape.
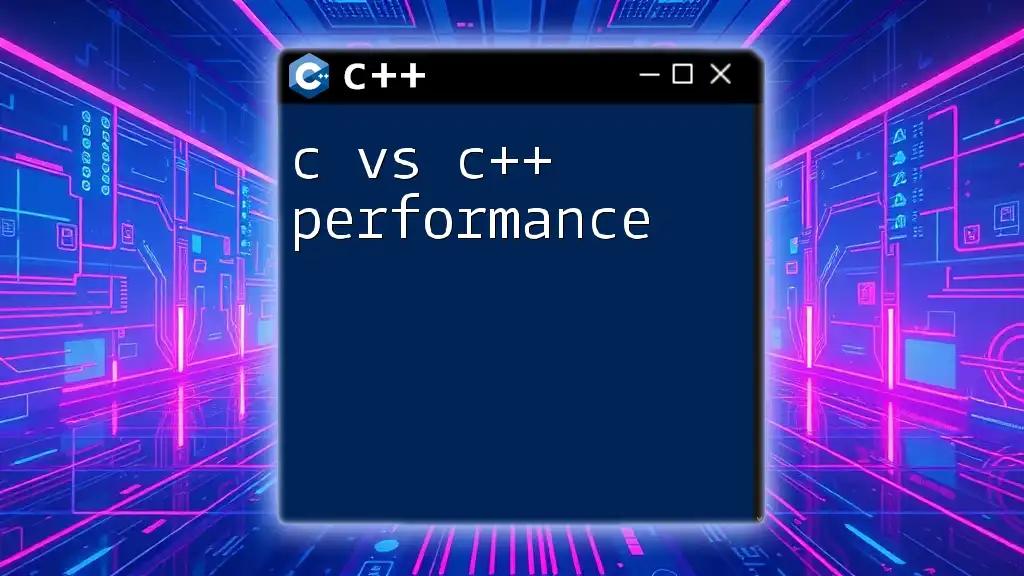
Call to Action
We invite you to share your thoughts on this discussion! What experiences do you have with Go and C++? Which language do you prefer, and why? Join the conversation in the comments section below!