The C++ programming language (cpp) defines the syntax and structure for writing C++ programs, while g++ is a specific compiler used to convert C++ code into executable programs.
Here’s a simple code snippet demonstrating how to compile a C++ program using g++:
// hello.cpp
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
To compile this file using g++, you would run:
g++ hello.cpp -o hello
Executing `./hello` would then print "Hello, World!" to the console.
What is CPP?
CPP, or C++, is a powerful, high-level programming language that adds object-oriented features to the programming paradigm established by its predecessor, the C programming language. C++ was designed with performance and efficiency in mind, which makes it widely used in various application domains, such as systems software, game development, embedded programming, and large-scale enterprise applications.
Key features of C++ include:
- Object-Oriented Programming (OOP): C++ allows developers to create classes and objects, enabling modularity and code reuse.
- Strong Type Checking: C++ enforces stricter data type checks compared to C, reducing potential runtime errors.
- Standard Template Library (STL): C++ comes with a rich set of generic classes and functions that help manage collections of data.
The importance of C++ in the programming world cannot be understated. It combines the power of low-level programming with the flexibility of high-level languages, making it a favorite among developers. C++ has a large community, extensive resources, and a vast ecosystem of libraries and frameworks that speed up development processes.

Understanding G++
G++ is a specific implementation of the GNU Compiler Collection (GCC) for compiling C++ code. As a compiler, G++ translates the human-readable C++ source code into machine code or an executable format that the computer can understand. In essence, G++ serves as a crucial step in turning C++ ideas and logic into runnable software.
The advantages of using G++ are substantial:
- Open-source: G++ is freely available and continuously improved by the global community, ensuring that it remains updated and reliable.
- Cross-platform compatibility: G++ can be run on various operating systems, including Windows, Linux, and macOS. This flexibility helps developers maintain consistent development workflows across different environments.
- Optimizations: G++ includes numerous optimization techniques that improve the performance and speed of the generated executable code, enabling developers to create efficient applications.

CPP vs G++
Fundamental Differences
The terms CPP and G++ represent different aspects of the C++ programming workflow. While CPP refers to the actual programming language and the code that developers write, G++ refers to the compiler that converts this code into an executable program.
Understanding these distinctions is crucial for any aspiring C++ programmer:
- What CPP includes: CPP consists of .cpp files containing C++ source code—the syntactical code you write to implement your logic and functionalities.
- What G++ does: G++ compiles the CPP code into machine code, ultimately producing a binary output (executable file) that can be run on your computer.
Detailed Comparison
Functionality
To illustrate the functionality differences between CPP and G++, consider the following C++ code snippet:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Here, the code defines a simple program that outputs "Hello, World!" When executed, this process involves two distinct stages:
- Code Writing: You write the C++ code in a .cpp file (like `hello.cpp`).
- Compiling: This .cpp file is fed into G++ for compilation. The output will be an executable file (such as `hello`), which can be run to see the result.
The key point is that CPP represents the design and logic, while G++ turns that design into a functioning program.
Output Formats
When you write CPP code, you are creating the blueprint. In contrast, when G++ processes this code, it produces an executable format that is ready to run.
This transition from written code to an executable format bridges the gap between programming and practical application, showcasing the vital role of the compiler.
Use Cases for CPP and G++
Understanding when to utilize CPP or G++ in your workflow can enhance your development process significantly.
When to Use CPP
Use CPP primarily when writing the actual code. This includes:
- Developing modular code using classes and functions
- Implementing complex algorithms and data structures
- Writing unit tests and integrating larger systems
In this phase, you'll focus on syntax, logic, and structuring your code effectively.
When to Use G++
G++ should be employed when compiling your C++ code into executable formats. This is particularly important when:
- Working on large-scale projects that require efficient building
- Implementing automated scripts to streamline deployment
- Testing and debugging code in different environments
For example, to compile your `hello.cpp` code into an executable named `HelloWorld`, you would run:
g++ -o HelloWorld hello.cpp
This command instructs G++ to create an executable so you can run your application.
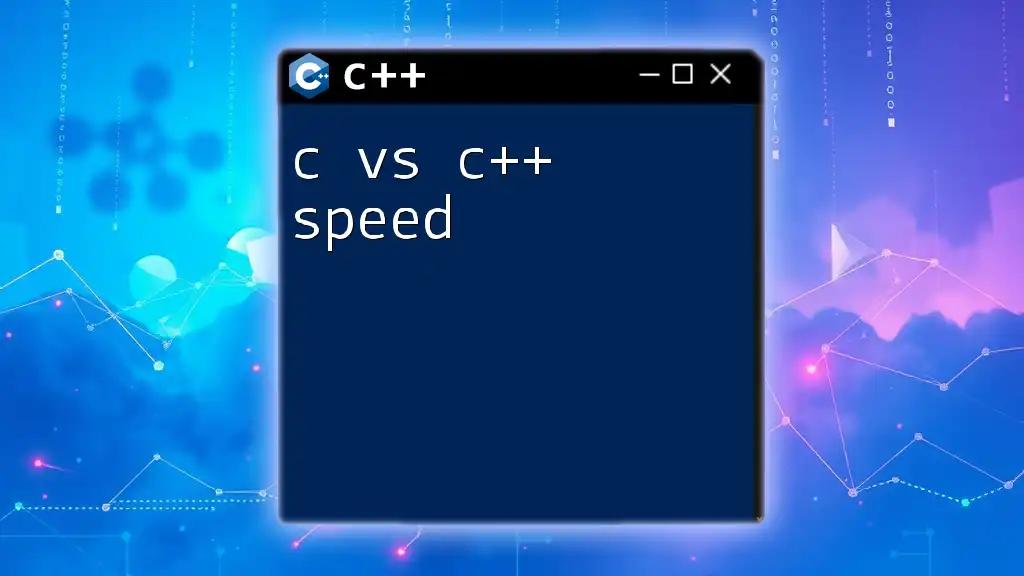
Best Practices
Efficient Coding in CPP
When coding in CPP, adhere to best practices that can enhance code readability and maintainability:
- Organize your code into functions and classes for better structure.
- Follow consistent naming conventions for variables and functions.
- Include comments and documentation to explain your code logic for future reference.
Compiling with G++
To efficiently use G++, familiarize yourself with commonly used command-line flags and options, such as:
- `-Wall`: Activates all compiler's warning messages to promote cleaner code.
- `-O2`: Enables optimizations that improve speed without significant code size increase.
For example, use G++ with warnings and optimization flags as follows:
g++ -Wall -O2 -o OptimizedProgram main.cpp
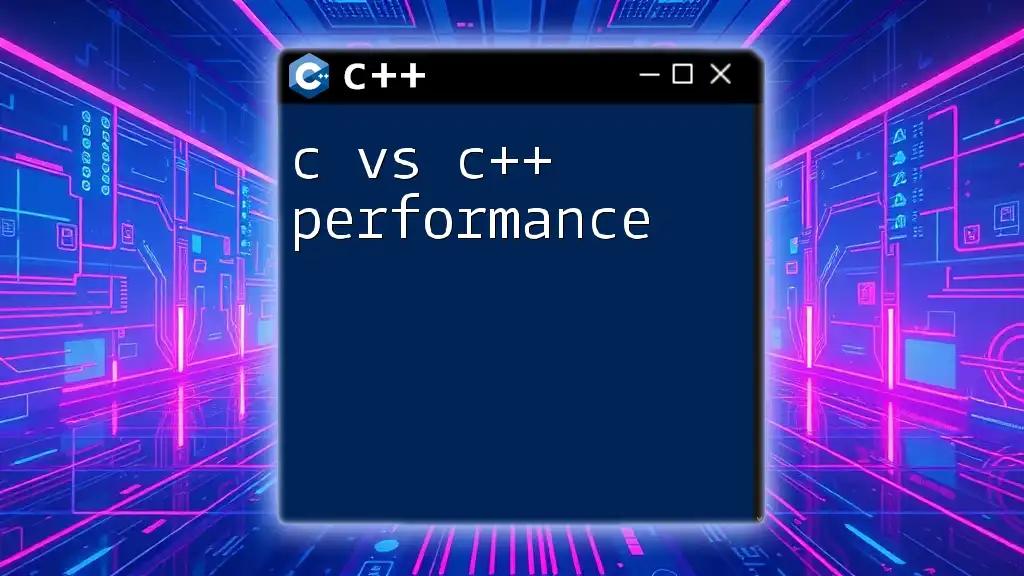
Troubleshooting Common Issues
When developing in C++ and using G++, you may encounter common errors that need addressing.
CPP Code Errors
Common syntax and logic errors might plague your CPP code. It’s important to be vigilant and test your code iteratively. Typical mistakes include:
- Mismatched parentheses or braces
- Incorrect variable types being used
G++ Compilation Errors
When compiling code, you may face various errors. Some common ones include:
- Linker errors: These occur if the G++ cannot find the definitions for declared functions or classes. Here's an example of a compilation command that may produce a linker error:
g++ -o MyProgram MyProgram.cpp
If `MyProgram.cpp` has unresolved symbols, you will see linker errors that need troubleshooting.

Conclusion
In summary, understanding the difference between CPP and G++ is essential for any C++ programmer. CPP represents the fundamental language and code you write, while G++ serves as the indispensable tool that compiles that code into executable forms. Each plays a unique role in the development lifecycle, and mastering both is critical for successful programming in C++.
As you embark on your C++ journey, remember to practice regularly, explore both coding and compiling aspects, and engage with the vibrant community around C++. With determination and practice, you'll become proficient in the language and its tools.
Don't hesitate to share your experiences with CPP and G++ in the comments, and consider exploring our classes and resources to deepen your understanding further!